Jump to a key chapter
What are Comparison Operators in Python?
Comparison operators in Python are the symbols that allow you to make comparisons between two or more values. They enable you to evaluate expressions based on certain conditions, and the output of these comparisons is always a boolean value (either True or False). These operators are an essential feature in programming as they make it possible to create complex condition checks, branching structures, and loops.
Comparison Operators: Symbols used to compare two or more values in programming languages, resulting in a boolean value (True or False).
Python Comparison Operators Types and Functions
Python provides a variety of comparison operators, each with a specific purpose and function. Understanding these operators, their syntax, and usage, will help you write more efficient and readable code. Here are the most common Python comparison operators:
Operator | Name | Description |
== | Equal to | Checks if the values on both sides of the operator are equal. |
!= | Not equal to | Checks if the values on both sides of the operator are not equal. |
> | Greater than | Checks if the value on the left side of the operator is greater than the one on the right. |
< | Less than | Checks if the value on the left side of the operator is less than the one on the right. |
>= | Greater than or equal to | Checks if the value on the left side of the operator is greater than or equal to the one on the right. |
<= | Less than or equal to | Checks if the value on the left side of the operator is less than or equal to the one on the right. |
Python comparison operators can be used with various data types, such as integers, floats, and strings. When comparing values, Python will try to implicitly convert them to a common data type. For example, when comparing an integer with a float, the interpreter will implicitly convert the integer into a float value.
Example: Let's compare two integers using the "greater than" operator:
5 > 3 # True
Now, let's compare an integer and a float using the "equal to" operator:
10 == 10.0 # True, as the integer 10 is implicitly converted to the float 10.0
In some cases, comparison operators can also be used with collections like lists, tuples, and strings. These comparisons are done in a lexicographical order, meaning the comparison is decided based on the order of elements.
Deep Dive: When comparing strings, Python compares the Unicode code point of each character. For this reason, comparison between uppercase and lowercase letters can produce different results, as the code points of these characters differ.
Exploring Python Class Comparison Operators
Python comparison operators are not limited to primitive data types like integers, floats, and strings. You can also utilise them within custom classes for easy object comparisons. Python allows you to define how these operators behave within your custom classes by implementing special methods known as "magic methods" or "dunder methods."
Magic methods (or dunder methods): Special methods in Python custom classes that define how certain operators and functionality behave within the class.
To use Python comparison operators within a custom class, you must implement the following magic methods:
Magic Method | Operator | Description |
__eq__(self, other) | == | Defines the behaviour for the 'equal to' operator. |
__ne__(self, other) | != | Defines the behaviour for the 'not equal to' operator. |
__gt__(self, other) | > | Defines the behaviour for the 'greater than' operator. |
__lt__(self, other) | < | Defines the behaviour for the 'less than' operator. |
__ge__(self, other) | >= | Defines the behaviour for the 'greater than or equal to' operator. |
__le__(self, other) | <= | Defines the behaviour for the 'less than or equal to' operator. |
When implementing these magic methods, remember to define the logic for how to compare the instances of your custom class.
Example: Let's create a custom class called 'Person' and implement the magic methods that correspond to the 'equal to' and 'not equal to' operators:
class Person:
def __init__(self, age):
self.age = age
def __eq__(self, other):
if isinstance(other, Person):
return self.age == other.age
return NotImplemented
def __ne__(self, other):
result = self.__eq__(other)
if result is NotImplemented:
return NotImplemented
return not result
person1 = Person(25)
person2 = Person(30)
person3 = Person(25)
print(person1 == person2) # Outputs False
print(person1 != person3) # Outputs False
Best practices for Class Comparison Operator usage
When working with class comparison operators, following these best practices will ensure your code is efficient, flexible, and easy to understand:
- Return NotImplemented: When implementing a comparison method, if the function cannot determine how to compare two objects, return NotImplemented instead of raising an exception. This allows Python to try calling the comparison method on the other object.
- Fallback to Comparison Methods: When implementing a magic method such as __ne__(self, other) or __gt__(self, other), use the corresponding comparison method, like __eq__(self, other) or __lt__(self, other), as a starting point. This will ensure that the logic defined in one method is consistent with the logic in the other method.
- Python built-in methods: Use Python built-in functions like super() or functools.total_ordering when possible to simplify custom class comparisons.
- Code Refactoring: Keep the logic for comparisons within the corresponding magic methods, and avoid cluttering your class with unnecessary helper methods related to comparisons.
Following these best practices can significantly impact the readability, efficiency, and maintainability of your Python code when implementing comparison operators in custom classes.
Python Comparison Operators Overloading Explained
In Python, overloading refers to the ability to redefine the behaviour of certain operators or functions so they can be used with custom classes. In the context of comparison operators, overloading allows you to compare objects of your custom classes using standard comparison operators like ==, !=, >, <, >=, and <=.
To overload comparison operators in Python, you have to define the corresponding magic methods within your custom class. As mentioned earlier, each comparison operator is associated with a distinct magic method which needs to be implemented in the class.
Example: Overloading the equal to (==) comparison operator in a custom class called 'Book' that compares the title of two book instances:
class Book:
def __init__(self, title):
self.title = title
def __eq__(self, other):
if isinstance(other, Book):
return self.title.lower() == other.title.lower()
return NotImplemented
book1 = Book("Python Programming")
book2 = Book("python programming")
print(book1 == book2) # Outputs True, since the titles are the same (ignoring case)
Use Cases and Benefits of Overloading Comparison Operators
Overloading comparison operators in Python is essential for numerous use cases. Here are some common scenarios where overloading comparison operators plays a significant role:
- Sorting and Comparing Custom Objects: Comparison operators overloading allows objects of custom classes to be easily sorted and compared using built-in functions like sorted(), max(), and min(). This can be particularly useful when working with lists or other collections of custom objects.
- Data Structures: Overloading comparison operators enable the development of complex data structures, such as priority queues and binary search trees, requiring custom comparisons between objects.
- Logical Conditions: Implementing comparison operators for custom classes simplifies logical conditions in control structures like if statements and while loops.
- Testing and Debugging: Overloading comparison operators can be helpful during testing and debugging by enabling direct object comparison, making it easier to analyse and identify discrepancies in your code.
Additionally, overloading comparison operators offer several benefits that can improve the overall quality of your code:
- Flexibility: Comparison operators overloading adds more flexibility to your code, allowing you to adapt the behaviour of these operators to fit your specific requirements.
- Readability: Overloading comparison operators enhances code readability by providing a standard way of comparing objects, making the code more intuitive and easier to understand.
- Maintainability: Implementing overloaded comparison operators within custom classes ensures that the logic for object comparison is encapsulated within the class, making it easier to maintain or update your code.
In conclusion, overloading comparison operators is an essential tool for working with custom classes in Python, providing numerous benefits such as improved flexibility, readability and maintainability of your code. Mastering comparison operators overloading will help you tackle complex programming tasks more effectively and efficiently.
Python List Comparison Operators
Python list comparison operators play an important role in working with lists, a versatile and widely-used data structure within the language. By implementing Python comparison operators for lists, you can compare elements within the data structure, making data manipulation tasks such as sorting and searching easier to perform.
How to Implement Python List Comparison Operators
To compare lists in Python, you can utilise the same built-in comparison operators as those used for other data types, such as ==, !=, >, <, >=, and <=. These operators allow you to make comparisons between different lists or even elements within the same list.
Here are some key points to keep in mind when using Python list comparison operators:
- Comparison operators work on lists element-wise, meaning Python will compare each corresponding element of both lists starting from the first index.
- When a comparison is made between elements of different data types within the same list, Python follows its type hierarchy for comparisons (e.g., an integer is deemed less than a float).
- To compare multiple list elements, you can combine comparison operators and logical operators such as "and" and "or".
- To implement custom comparisons within lists of custom classes, ensure that your class contains the appropriate magic methods for comparison operators.
Applying Python list comparison operators can be useful in numerous real-life scenarios, particularly for data analysis, where comparing and sorting elements within lists is a common task.
Sorting and Comparing Python Lists
Sorting and comparing lists in Python is a fundamental operation for data manipulation. There are several ways to perform these operations:
- Using the built-in sorted() function: This function returns a new sorted list from the given iterable. It accepts optional arguments such as 'key' (to provide a custom sorting logic) and 'reverse' (to sort the list in descending order).
- Using the list sort() method: The sort() method is an in-place sorting method that can be called on a list object. Similar to sorted(), it accepts the 'key' and 'reverse' arguments.
- Using comparison operators for element-wise comparison: You can use comparison operators (>, <, ==, !=, >=, <=) between lists or list elements to determine the relation between them. When comparing lists, Python compares them lexicographically, examining elements from left to right.
- Using lambda functions for complex comparison logic: In scenarios where the default sorting and comparison logic do not suffice, you can utilise lambda functions in combination with sorted() or sort(). This allows you to define custom sorting logic on-the-fly.
Example: Comparing and sorting a list of integers:
a = [3, 4, 5]
b = [1, 2, 3]
c = [3, 4, 5]
print(a == b) # Outputs False
print(a == c) # Outputs True
sorted_list = sorted(b)
print(sorted_list) # Outputs [1, 2, 3]
b.sort(reverse=True)
print(b) # Outputs [3, 2, 1]
Mastering the use of list comparison operators and various sorting techniques is essential for efficient and effective data manipulation in Python. It enables you to execute complex tasks with ease, ensuring that your code remains versatile and easy-to-read.
Python Comparison Operators Example
Understanding Python comparison operators is essential for learning to write more effective and efficient code. The best way to master this concept is by reviewing practical examples and hands-on exercises that demonstrate the use of these operators in various scenarios. This way, you'll be better equipped to handle complex tasks and challenges in your coding journey.
Practical Examples of Python Comparison Operators
Here are several practical examples covering different aspects of Python comparison operators. These examples demonstrate how to use these operators and help you understand their real-world applications:
- Integer comparison: Comparing numerical values is often a basic yet important task in programming. Let's compare two integer values, A and B:
A = 10
B = 5
result = A > B # Outputs True because 10 is greater than 5
- String comparison: You can also compare strings using Python comparison operators. This is extremely useful when searching for specific patterns or sorting text data. Here's an example comparing two strings:
str1 = "apple"
str2 = "orange"
result = str1 < str2 # Outputs True because 'apple' comes before 'orange' lexicographically
- List comparison: Lists in Python can be compared directly using comparison operators. The items within the lists are compared element-wise, in a lexicographical order. Let's compare a pair of lists:
list1 = [1, 2, 3]
list2 = [1, 2, 4]
result = list1 < list2 # Outputs True because 3 < 4 (first difference in element-wise comparison)
Hands-on Exercises for Python Comparison Operators
Now that we've covered several practical examples, let's dive deeper into Python comparison operators with the following hands-on exercises:
- Comparing custom objects: Create a custom class 'Student' with attributes 'name' and 'age'. Implement the appropriate magic methods to enable comparison of 'Student' objects using the 'equal to' (==) operator. Compare two 'Student' objects with the same name but different ages.
- Combining comparison and logical operators:Write a Python program that checks if the elements of two lists are equal, considering the following rules:
- A == B – The elements at the same index in both lists are equal.
- A != B – The elements at the same index in both lists are not equal.
- Sorting lists by multiple criteria:Create a list of tuples containing information about books, such as title, author, and publication year. Write a Python program to sort this list using the following criteria:
- Primary sorting should be based on the author's name, in alphabetical order.
- Secondary sorting should be based on the publication year, in ascending order.
Working on these hands-on exercises will help you become more comfortable with using Python comparison operators in a variety of situations. By understanding how these operators work, you can make your coding more efficient and tackle complex programming tasks with ease.
Python Comparison Operators - Key takeaways
Python Comparison Operators: symbols that allow you to compare values, resulting in a boolean value (True or False).
Common types: Equal to (==), Not equal to (!=), Greater than (>), Less than (=), Less than or equal to (<=).
Python Class Comparison Operators: special magic methods that define how comparison operators behave within custom classes.
Comparison Operators Overloading: redefining the behaviour of comparison operators for custom classes to enable object comparisons.
Python List Comparison Operators: use built-in operators to compare lists or elements within a list, aiding data manipulation tasks.
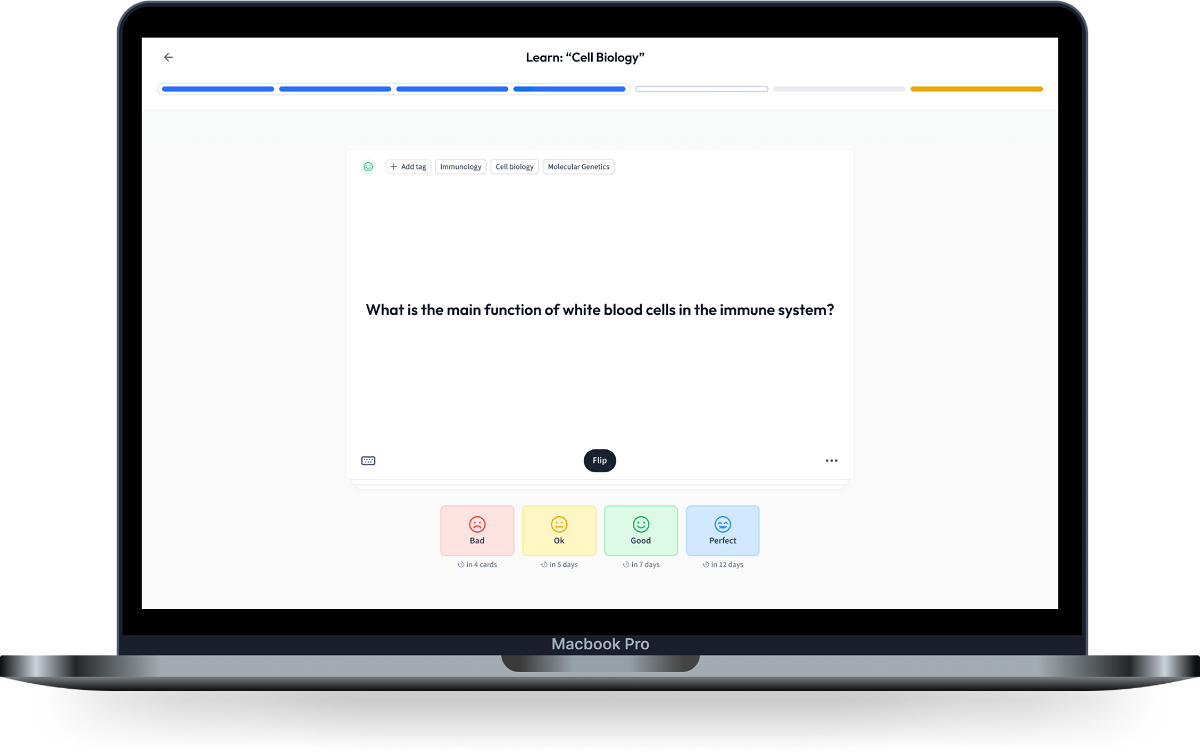
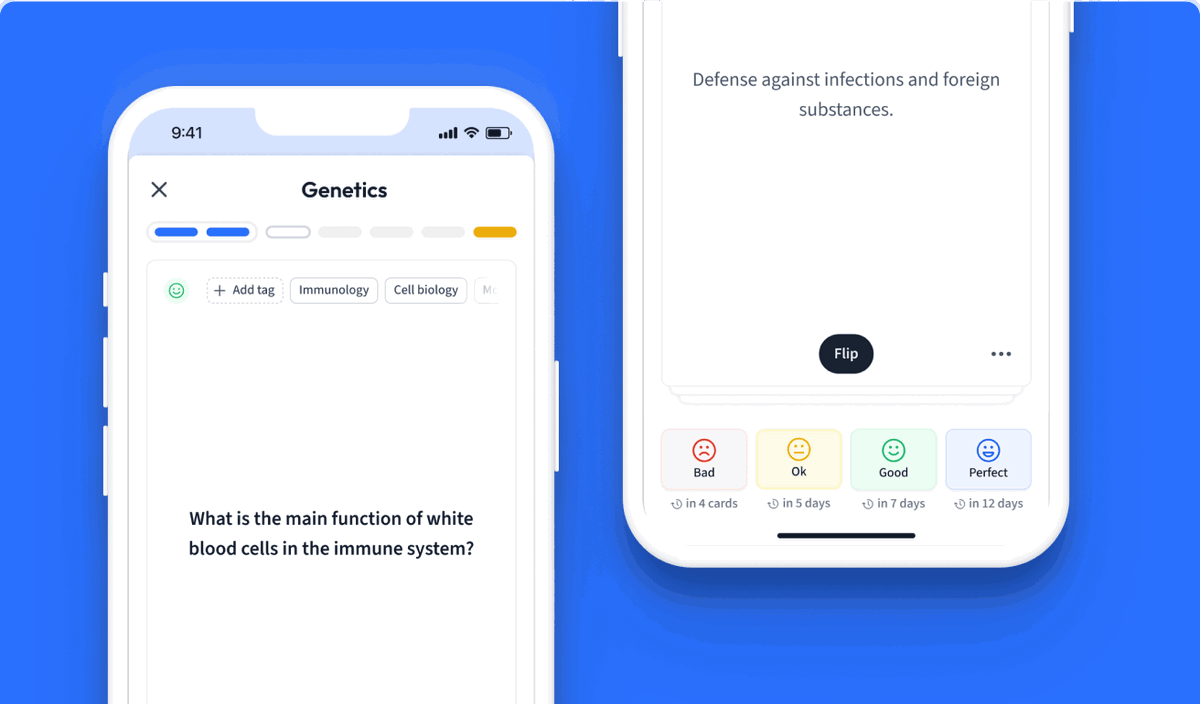
Learn with 24 Python Comparison Operators flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Python Comparison Operators
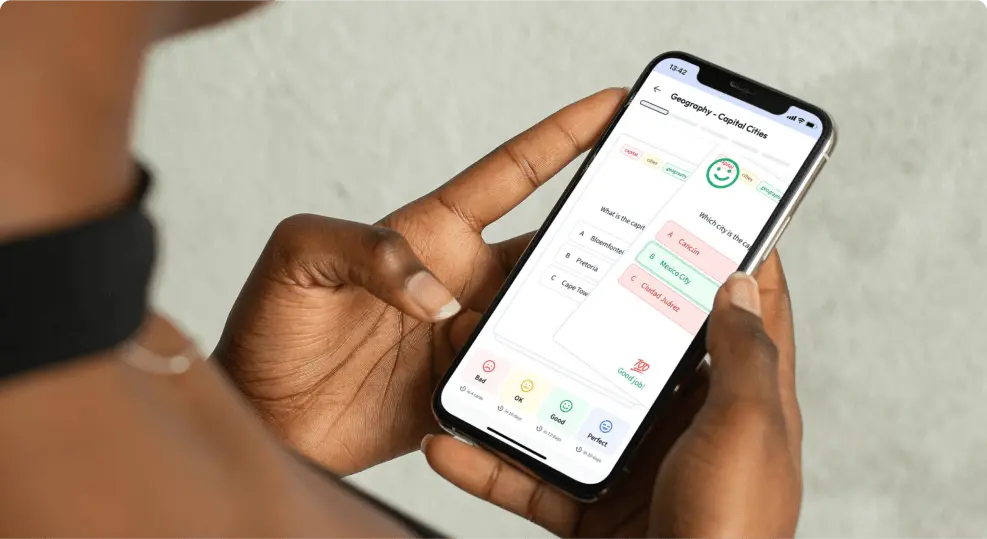
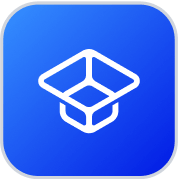
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more