Jump to a key chapter
Understanding Python Data Types
In the world of computer programming, data types play a fundamental role in ensuring that your code runs smoothly and correctly. Python, a popular programming language, offers a wide range of data types to help you manage and manipulate different kinds of data in your programs.
The Importance of Python Data Types in Computer Programming
In computer programming, data types are essential because they determine how data is stored, processed, and presented to the users. Proper understanding and use of Python data types allow you to write efficient, error-free code, and achieve the desired output. Let's explore some key reasons why Python data types are so crucial:
- Memory Allocation: Data types determine the amount of memory assigned to a variable or constant. Different data types have different memory requirements, and using the correct data type helps in optimal memory utilization.
- Operations: Certain operations are specific to particular data types. For example, arithmetic operations can be performed on numeric data types, whereas string operations can be applied to text data. Using suitable data types allows you to perform relevant operations without errors.
- Type Checking: Python is a dynamically typed language, which means it automatically identifies the data type of a variable during runtime. Understanding data types can help avoid common issues, such as type mismatch or unexpected outputs, in your Python programs.
- Code Readability: Choosing the appropriate data type makes your code more readable and easier to understand. This is particularly important when working on large projects or collaborating with other developers.
Note: Data types are the building blocks of any programming language. Although Python is a high-level and flexible language that automatically handles many aspects of data types, understanding their fundamentals is crucial for writing efficient and error-free programs.
Overview of Primitive and Built-in Data Types in Python
Python data types can be broadly classified into two categories: primitive and built-in data types. Primitive data types consist of basic types like numbers and characters, whereas built-in data types include more complex structures such as lists, dictionaries, sets, and tuples. Let's dive into more detail about each of these data types.
Primitive Data Types: These are the most basic data types in Python, consisting of numbers (integers, floating-point numbers), and characters (strings). The primary primitive data types in Python are int, float, and str.
Data Type | Description |
int | Represents integer numbers, i.e., whole numbers without decimal points. E.g., 42 |
float | Represents floating-point numbers, i.e., real numbers with decimal points. E.g., 3.14 |
str | Represents a sequence of characters enclosed within single or double quotes. E.g., 'Hello, World!' |
Built-in Data Types: These data types are more complex and versatile structures provided by Python, which can store and manipulate collections of data. The primary built-in data types are list, tuple, dictionary, and set.
Data Type | Description |
list | Represents an ordered, mutable collection of items enclosed within square brackets [ ]. Items can be of different data types. |
tuple | Similar to a list but enclosed within parentheses ( ). Tuples are ordered but immutable, meaning their elements cannot be modified after creation. |
dictionary | Represents an unordered collection of key-value pairs, enclosed within curly braces { }. Dictionaries are mutable, and elements can be accessed using their keys. |
set | Represents an unordered collection of unique items, enclosed within curly braces { }. Sets are mutable, but unlike lists and dictionaries, do not allow duplicate values. |
Here's an example illustrating the use of built-in data types in Python: # List example fruits = ['apple', 'banana', 'orange'] print(fruits[0]) # Output: apple # Tuple example coordinates = (40.7128, 74.0060) print(coordinates[1]) # Output: 74.0060 # Dictionary example student = {'name': 'John', 'age': 25, 'grade': 'A'} print(student['name']) # Output: John # Set example unique_numbers = {1, 2, 3, 2, 1, 3} print(unique_numbers) # Output: {1, 2, 3}
Python Data Types List: An Extensive Guide
To write efficient and error-free code in Python, it is crucial to thoroughly understand its data types. In the following sections, we will delve into the details of both primitive and built-in data types, including integers, floating-point numbers, complex numbers, strings, booleans, lists, tuples, sets, dictionaries, and arrays.
Exploring Primitive Data Types in Python
Primitive data types in Python are the most basic types, including numbers (integers, floating-point numbers, complex numbers) and characters (strings). Additionally, Python provides the boolean data type for true/false values. Let's delve deeper into these data types.
Integers, Floating point numbers, and Complex numbers
Python supports three types of numeric data: integers, floating-point numbers, and complex numbers. These numeric data types allow you to perform mathematical operations in your programs.
- Integers: Integers are whole numbers without decimal points. In Python, integers can be positive, negative, or zero. They are represented by the 'int' data type. Python automatically handles very large integer values, and there is no maximum limit for integer values.
- Floating-point numbers: Floating-point numbers or floats represent real numbers with decimal points. They are represented by the 'float' data type. Python supports floating-point numbers with different levels of precision, depending on the available system memory.
- Complex numbers: Python also supports complex numbers, which consist of a real part and an imaginary part. The real part is a floating-point number, and the imaginary part is a floating-point number followed by the letter 'j'. Complex numbers are represented by the 'complex' data type.
Here are a few examples of numeric data types in Python: integer_number = 42 # int float_number = 3.14 # float complex_number = 2+3j # complex
Strings and Booleans: An Introduction
In addition to numeric types, Python offers data types to handle text and logical values: strings and booleans.
- Strings: Strings represent a sequence of characters enclosed within single or double quotes. They are represented by the 'str' data type. In Python, strings are immutable, meaning their content cannot be altered after creation. You can, however, concatenate and slice strings using various string operations.
- Booleans: Booleans represent true or false values and are represented by the 'bool' data type. They are often used in conditional statements to control the flow of your program. In Python, boolean values are case sensitive and must be written as 'True' and 'False' (capitalized).
Here are a few examples of string and boolean data types in Python: my_string = "Hello, World!" # str my_boolean = True # bool
Built-in Data Types in Python Explained
Beyond primitive data types, Python offers more complex and versatile built-in data types, including lists, tuples, sets, dictionaries, and arrays. These data types provide a powerful and flexible way to store and manipulate collections of items in your programs.
Lists, Tuples, and Sets: Essential Python Data Types
Lists, tuples, and sets in Python are used to store and manipulate collections of data. Each of these data types has distinct characteristics:
- Lists: A list is an ordered, mutable collection of items enclosed within square brackets [ ]. Items can be of different data types, and you can access, insert, or delete elements using various list operations. Lists also support slicing, which allows you to extract a portion of the list.
- Tuples: A tuple is similar to a list but is enclosed within parentheses ( ). Tuples are ordered but immutable, meaning their elements cannot be altered after creation. Because of their immutable nature, tuples are often faster than lists in certain scenarios.
- Sets: A set is an unordered collection of unique items enclosed within curly braces { }. Sets are mutable and do not allow duplicate values. They are particularly useful for removing duplicates from a list or performing mathematical set operations such as unions, intersections, and differences.
Here are a few examples of lists, tuples, and sets in Python: my_list = [1, 2, 3] # List my_tuple = (4, 5, 6) # Tuple my_set = {1, 2, 2, 3, 3} # Set (output: {1, 2, 3})
Dictionaries and Arrays: Working with Key-Value Pairs
Dictionaries and arrays are important Python data types that allow you to store data using key-value pairs. Here, we'll discuss dictionaries and a variant of the list called an array:
- Dictionaries: A dictionary is an unordered collection of key-value pairs enclosed within curly braces { }. They are mutable, and elements can be accessed, inserted, or deleted using their keys. Dictionaries are particularly useful for representing structured data, such as JSON data, in a more human-readable format.
- Arrays: An array is a variant of lists that can store items of a single data type (integers, floats, or characters). Although the array data type is not built into the core of Python, you can use the 'array' module to access and manipulate arrays. Arrays are often preferred over lists for numerical calculations, as they are more memory-efficient and allow you to perform element-wise operations.
Here are a few examples of dictionaries and arrays in Python: my_dict = {'key1': 'value1', 'key2': 'value2'} # Dictionary import array my_array = array.array('i', [1, 2, 3]) # Array (typecode 'i' represents signed integers)
Learning to Check Data Types in Python
When working with Python, it is essential to understand how to check and validate data types to ensure the smooth execution of your programs. This can help prevent errors caused by mismatched or incompatible data types. In this section, we will explore how to use the 'type()' function to identify Python data types and discuss how to validate input data types in your Python programs.
The 'type()' Function: Identifying Python Data Types
In Python, the built-in 'type()' function is used to determine the data type of a given object. By passing an object as an argument to the 'type()' function, you can obtain its data type as output. This is particularly useful when dealing with variables where the data type is uncertain or when you need to ensure that specific objects have the expected data types.
Features of the 'type()' function include:
- One required argument: The object whose data type you want to determine.
- Output: The data type of the object passed as an argument.
- Compatibility: Works with both primitive and built-in data types.
Keep in mind that the 'type()' function returns the actual data type of the object, not a string representation. So, when comparing the output of 'type()' to a specific data type, use the data type itself rather than its string representation.
Examples of Using 'type()' to Check Data Types in Python
The following examples demonstrate how to use the 'type()' function to check the data types of various objects in Python:
integer_number = 42 data_type = type(integer_number) print(data_type) # Output: my_string = "Hello, World!" data_type = type(my_string) print(data_type) # Output: my_list = [1, 2, 3] data_type = type(my_list) print(data_type) # Output:
As seen in the examples above, using 'type()' enables you to determine the data type of various objects, including integers, strings, and lists. This is valuable in situations where you need to confirm that specific variables or inputs in your programs have the correct data types.
Validating Input Data Types in Python Programming
In Python programming, it is crucial to validate input data types to avoid errors and ensure the expected functionality of your code. Validating input data types can be helpful when handling user input, processing data from external sources (such as files or APIs), or even minimizing errors caused by incorrect data types within your program.
To validate input data types in Python, you can make use of the 'type()' function or the 'isinstance()' function. The 'type()' function allows you to check the data type of an object, while the 'isinstance()' function compares the object with a specified class (or classes) to determine if the object is an instance of a particular class (or a subclass thereof).
The following steps can be used to validate input data types in Python programming:
- Obtain the data type of the input object using the 'type()' function.
- Compare the obtained data type with the expected data type(s).
- If necessary, use the 'isinstance()' function to check if the object is an instance of a specific class or subclass.
- Implement appropriate error handling or data conversion based on the validation results.
By following these steps, you can ensure that your Python programs are robust and error-free, capable of handling data with varying data types.
Here is an example demonstrating how to validate input data types in Python using both 'type()' and 'isinstance()' functions: user_input = input("Enter a number: ") # Check using 'type()' function if type(user_input) != int: print("Invalid input: Please enter an integer.") # Check using 'isinstance()' function if not isinstance(user_input, int): print("Invalid input: Please enter an integer.")
Keep in mind that using 'isinstance()' is considered more versatile since it also works with subclasses and allows you to check for multiple data types simultaneously. On the other hand, 'type()' is more suitable when you need to check for an exact data type match.
Practical Python Data Types Examples
In this section, we will explore practical examples that help gain a deeper understanding of Python data types in action. Specifically, we will focus on hands-on exercises for working with primitive data types, as well as real-world examples using built-in data types. These examples will provide you with the knowledge and confidence to tackle challenges using Python data types in your own projects.
Hands-on Exercises for Working with Primitive Data Types
Let's start with some hands-on exercises that will allow you to practice working with Python's primitive data types, such as integers, floating-point numbers, strings, and booleans. These exercises are designed to illustrate the basic properties of primitive data types and demonstrate their practical applications in various programming scenarios.
- Integers and Floating-point numbers: Create a program that reads two numbers provided by the user, performs arithmetic operations like addition, subtraction, multiplication, and division, and prints the results.
- Strings: Create a program that takes a string input from the user and performs string manipulations such as reversing the string, converting it to uppercase, and counting the number of vowels present in the string.
- Booleans: Create a program that evaluates a given logical expression, such as "not (A and B) or C", where the user inputs the values for A, B, and C as 'True' or 'False'.
Here's an example of the first exercise related to integers and floating-point numbers: # Read input from user number1 = float(input("Enter the first number: ")) number2 = float(input("Enter the second number: ")) # Perform arithmetic operations addition = number1 + number2 subtraction = number1 - number2 multiplication = number1 * number2 division = number1 / number2 # Print results print("Addition:", addition) print("Subtraction:", subtraction) print("Multiplication:", multiplication) print("Division:", division)
Real-World Examples using Built-in Data Types in Python
Now, let's delve into some real-world examples that demonstrate the power and flexibility of Python's built-in data types, such as lists, tuples, sets, and dictionaries. These examples will illustrate practical use cases for built-in data types and how they can be employed to solve various programming challenges that you may encounter in everyday situations.
- Lists: Create a program that reads a list of integers from the user, sorts the list in ascending order, and displays the smallest and the largest element in the list.
- Tuples: Create a program that reads a sequence of numbers separated by commas from the user and converts the sequence into a list and a tuple.
- Sets: Create a program that reads two lists of integers from the user, calculates the union, intersection, and difference of their corresponding sets, and displays the results.
- Dictionaries: Create a simple dictionary-based program that stores student information (name, age, and grade) and allows the user to query the information based on a given student's name.
Here's an example of the first real-world exercise related to lists: # Read input from user numbers = list(map(int, input("Enter a list of integers separated by spaces: ").split())) # Sort the list numbers.sort() # Find the smallest and the largest element smallest = numbers[0] largest = numbers[-1] # Print results print("Smallest element:", smallest) print("Largest element:", largest)
These examples and exercises will not only give you a better understanding of Python data types and their practical applications but also equip you with essential skills to confidently work with any kind of data structures in your future projects.
Python Data Types - Key takeaways
Python Data Types: int, float, str, list, tuple, dictionary, and set
Primitive data types: int (integer), float (floating-point number), and str (string)
Built-in data types: list (ordered, mutable), tuple (ordered, immutable), dictionary (unordered, key-value pairs), and set (unordered, unique items)
Check Data Types using 'type()' function or 'isinstance()' function
Examples and exercises to strengthen knowledge of working with Python Data Types
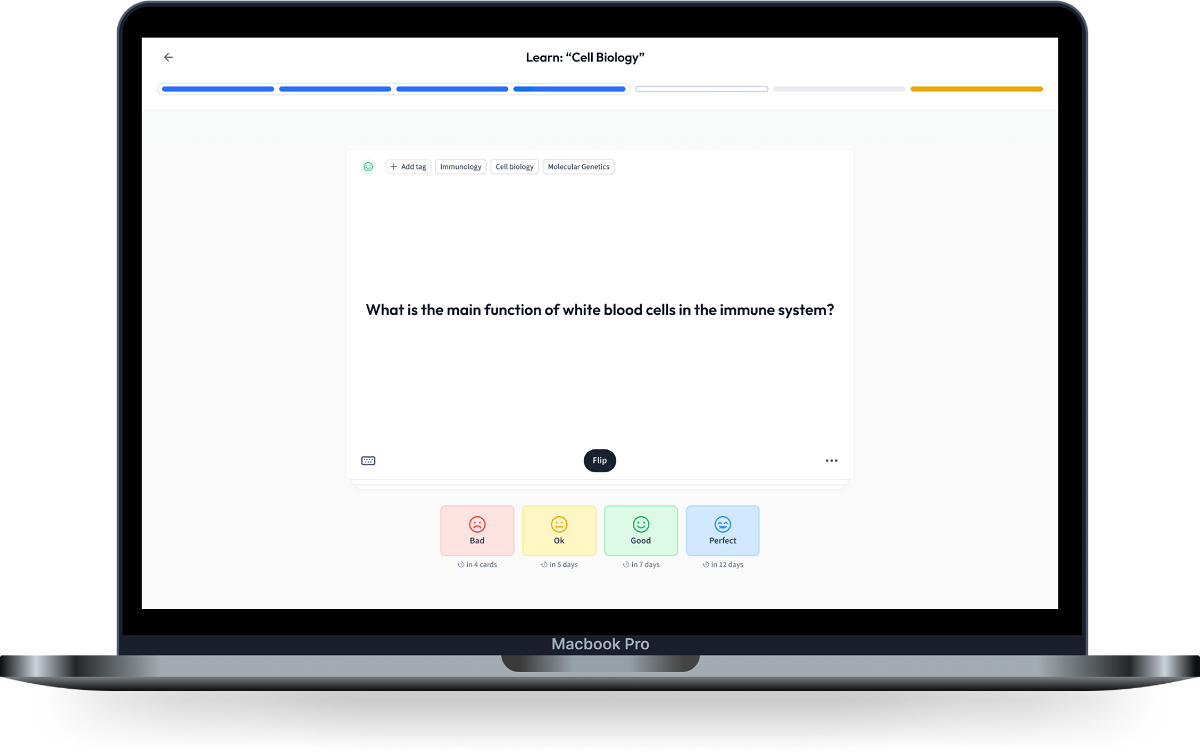
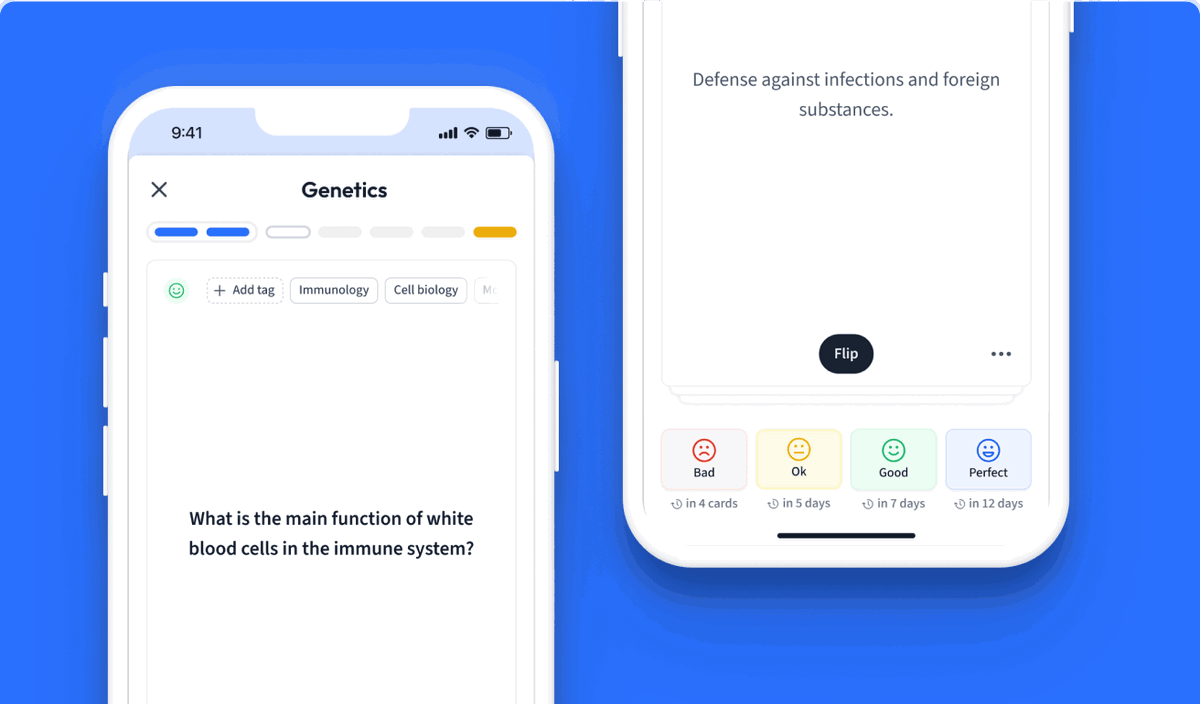
Learn with 23 Python Data Types flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Python Data Types
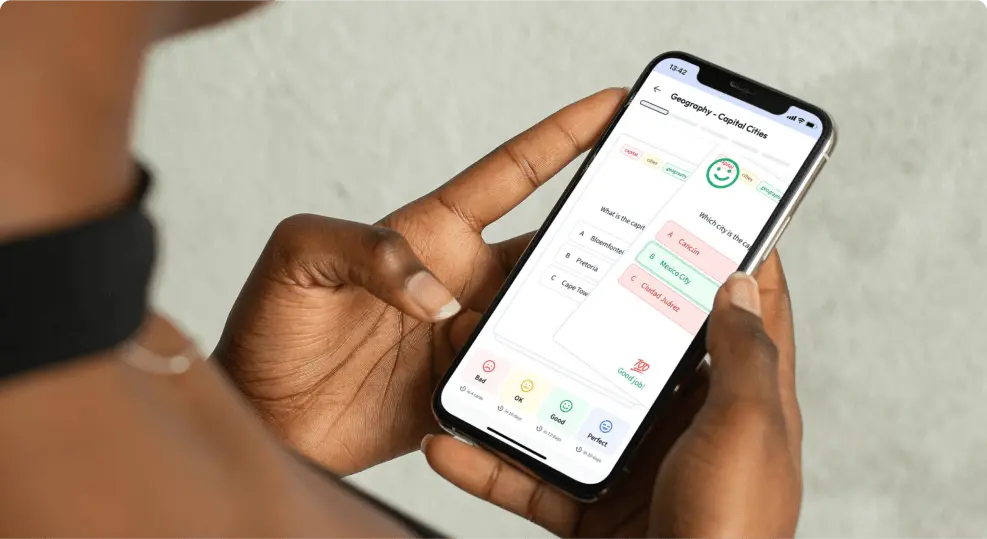
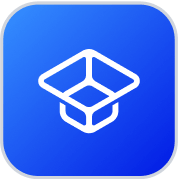
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more