Jump to a key chapter
Python Indexing Definition
Python Indexing refers to the method used to access specific elements from a sequence type like a list, tuple, or string. In Python, indexing helps you retrieve and modify the data elements, offering a powerful tool to navigate and manipulate structured data.
The Basics of Python Indexing
The concept of indexing in Python is fundamental for accessing elements within data structures such as lists, strings, and tuples. Indexing uses 0-based numbering, meaning the first element is at index 0, the second at index 1, and so on.
Consider the list fruits = ['apple', 'banana', 'cherry']. To access the first element, use fruits[0]. Similarly, fruits[1] and fruits[2] will give you 'banana' and 'cherry', respectively.
Negative indexing is another feature, where -1 refers to the last element, -2 to the second last, and so on. Therefore, fruits[-1] will retrieve 'cherry'.
Example:
fruits = ['apple', 'banana', 'cherry']# Accessing the first elementprint(fruits[0]) # Output: apple# Accessing the last elementprint(fruits[-1]) # Output: cherry
In Python, out-of-bound index access will lead to an IndexError. Ensure your index is valid for the length of the sequence.
Slicing with Python Indexing
Slicing extends the functionality of indexing by enabling retrieval of a range of elements from a sequence. The syntax sequence[start:stop] is used, where 'start' is inclusive, and 'stop' is exclusive.
This means that fruits[0:2] will return the list ['apple', 'banana']. If you omit 'start', it defaults to the beginning of the sequence. Likewise, omitting 'stop' defaults to the end. Thus, fruits[:2] also results in ['apple', 'banana'].
Step is an additional parameter in slicing, formatted as sequence[start:stop:step]. This allows you to access elements at regular intervals. For example, fruits[::2] will give every second element, returning ['apple', 'cherry'].
Deep Dive:
The power of slicing isn't just limited to Python's built-in data structures. It can also extend to your own classes by implementing the __getitem__ method. This allows for customized sequence behavior unique to your application's needs.
For instance, you can design a class that behaves like a list yet performs additional operations like logging every element access or transforming data on-the-fly. By leveraging slicing and indexing, Python offers an elegant and powerful way to navigate your data structures.
Understanding Python Indexing
Python Indexing is a key concept that helps you access elements from within a sequence. It's crucial in working with lists, strings, and tuples. Indexing is straightforward yet powerful, providing the tools needed to navigate and manipulate data effectively.
The Basics of Python Indexing
Indexing in Python uses a zero-based system, meaning you start counting from 0. For example, in the list numbers = [10, 20, 30, 40], the first element is accessed with numbers[0] and is 10, while numbers[3] accesses 40.
Python also supports negative indexing, which starts from the end of the sequence. Here, numbers[-1] would yield 40, while numbers[-4] would return 10.
- Zero-based index starts from the beginning.
- Negative index starts from the end.
Example of Indexing:
numbers = [10, 20, 30, 40]# Positive indexingprint(numbers[0]) # Output: 10# Negative indexingprint(numbers[-1]) # Output: 40
Remember, attempting to access an index beyond the sequence range will cause an IndexError.
Slicing with Python Indexing
Slicing grants you the ability to access a sub-list of a sequence by specifying a range. The syntax used is sequence[start:stop], where the 'stop' index is non-inclusive.
An empty value for 'start' defaults to the beginning, and an empty 'stop' defaults to the end. For instance, given colors = ['red', 'green', 'blue', 'yellow'], colors[1:3] returns ['green', 'blue'].
You can specify a 'step' with sequence[start:stop:step], which controls the interval at which elements are accessed. For example, colors[::2] would get every second element, yielding ['red', 'blue'].
Deep Dive:
The customization power of Python’s slicing can extend beyond lists and cover your own data structures. By customizing the __getitem__ method in your classes, you can define unique behaviors for indexing and slicing.
This allows for intricate data manipulations or on-the-fly transformations, making Python highly versatile in processing structured data.
Python Indexing Technique
Python Indexing Technique is crucial for selecting precise elements from a list, string, or tuple. As you delve into data manipulation, understanding indexing will empower you to efficiently access and modify various data types.
Basics of Indexing
In Python, indexing follows a zero-based system, which means the first position in a sequence is index 0. Using lists as an example, if fruits = ['apple', 'banana', 'cherry'], then fruits[0] retrieves 'apple'.
Negative indices help you retrieve elements from the end. Therefore, fruits[-1] returns 'cherry'.
- Zero-based Index: Begins at 0.
- Negative Index: Begins from the sequence's end.
Example:
fruits = ['apple', 'banana', 'cherry']# Access the first elementprint(fruits[0]) # Output: apple# Access the last elementprint(fruits[-1]) # Output: cherry
Slicing Techniques
Python slicing allows you to extract parts of a sequence by specifying a range. The syntax [start:stop] lets you retrieve elements from 'start' (inclusive) to 'stop' (exclusive).
Given colors = ['red', 'green', 'blue', 'yellow'], colors[1:3] yields ['green', 'blue'].
An omitted 'start' defaults to 0, and an omitted 'stop' defaults to the sequence's length. Assigning a 'step' extracts elements at regular intervals, as in colors[::2] returning ['red', 'blue'].
Negative step values can reverse sequences, such as sequence[::-1], which results in a reversed sequence.
Deep Dive:
Slicing is not restricted to lists and can be applied to any sequence type, including strings and tuples. Moreover, with the __getitem__ method, you can integrate slicing into custom objects, thereby enhancing their functionality.
This capability is particularly beneficial for developing data structures and algorithms where flexible and efficient data access patterns are required.
Imagine tailoring slicing functions to handle time series data, where indices represent temporal information. Implementing these customized slicing techniques in classes enables potent, time-aware sequence manipulation.
Python List Index Explained
Understanding how Python handles list indexing can significantly enhance your ability to navigate and manipulate lists. Let's delve into what makes indexing a fundamental part of using Python lists effectively.
Python List at Index
Definition: In Python, a list index is a numerical representation that uniquely identifies each element in a list. This allows for retrieval and manipulation of the list’s elements efficiently.
Accessing elements in a Python list uses zero-based indexing, where the first element is indexed at 0. For example, in the list numbers = [10, 20, 30, 40], the element at index 0 is 10, and the element at index 2 is 30.
Negative indexing allows you to access elements from the end of the list, where -1 corresponds to the last element. For numbers[-1], the result would be 40.
- numbers[0] = 10
- numbers[-1] = 40
Example:
numbers = [10, 20, 30, 40]# Accessing elementsprint(numbers[1]) # Output: 20print(numbers[-2]) # Output: 30
Utilizing negative indices can be very useful for accessing elements from the end of any sequence without needing to know its length.
How Python List Indexing Works
Python list indexing works by assigning each element an index for straightforward access. This numeric index can be used to retrieve, modify, or delete an element.
Beyond simple element access, Python supports list slicing to pull segments from lists. The syntax list[start:stop] can create a new list containing elements from 'start' to 'stop' (excluding 'stop'). For example, if colors = ['red', 'green', 'blue', 'yellow'], then accessing colors[1:3] would yield ['green', 'blue'].
Slices can also include a step value, as in colors[::2], which retrieves every second element, producing ['red', 'blue'].
Deep Dive:
The underlying mechanism of indexing relies on a contiguous block of memory. When you use indexes, Python calculates the corresponding memory location, providing direct and fast access, which makes lists a dynamically-scalable data structure.
However, this memory layout also means that while accessing and appending elements is efficient, inserting or deleting elements can become costly as it may require shifting elements. Understanding this trade-off is vital for choosing the right data structure according to use cases.
Advantages of Python Indexing
Python indexing offers several benefits that aid in efficient data handling:
- Fast Access: Immediate access to any element by its index.
- Easy Manipulation: Simple syntax for modifying or retrieving elements.
- Flexibility: Support for negative indexing and slicing for accessing ranges.
- Powerful Slicing: Ability to retrieve subsets of data easily, enhancing readability and ease of use in code.
Example of Slicing:
letters = ['a', 'b', 'c', 'd', 'e']# Slicing the listprint(letters[1:4]) # Output: ['b', 'c', 'd']print(letters[:3]) # Output: ['a', 'b', 'c']
Python Indexing - Key takeaways
- Python Indexing Definition: Method to access specific elements from sequence types like lists, using 0-based numbering.
- Positive and Negative Indexing: Positive indexing starts from 0, negative indexing starts from -1 (end of the list).
- Example Usage: Access element in a list using index, e.g.,
fruits[0]
retrieves 'apple'. - Python List Index: Numerical identifier of list elements for easy retrieval and manipulation.
- Slicing Technique: Access ranges in sequences with
[start:stop:step]
syntax. - Advantages of Python Indexing: Fast access, easy manipulation, flexible negative indexing, and slicing capabilities.
Learn faster with the 27 flashcards about Python Indexing
Sign up for free to gain access to all our flashcards.
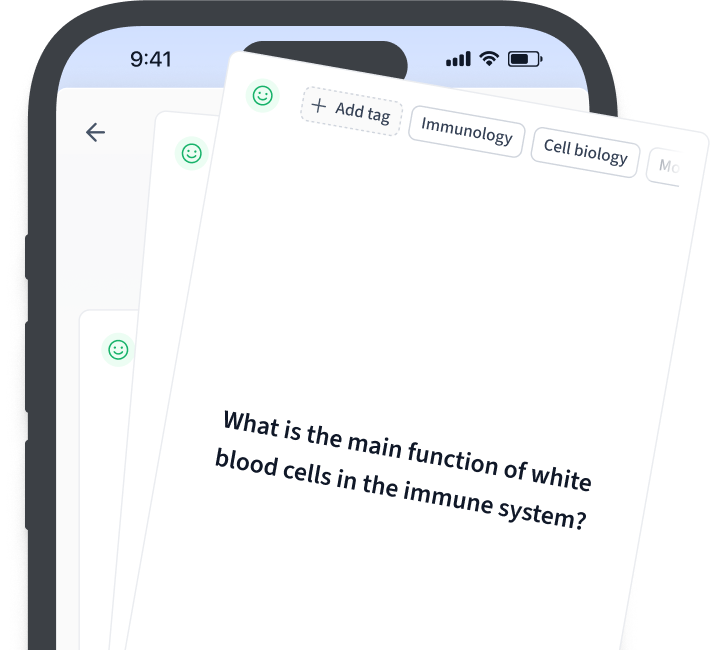
Frequently Asked Questions about Python Indexing
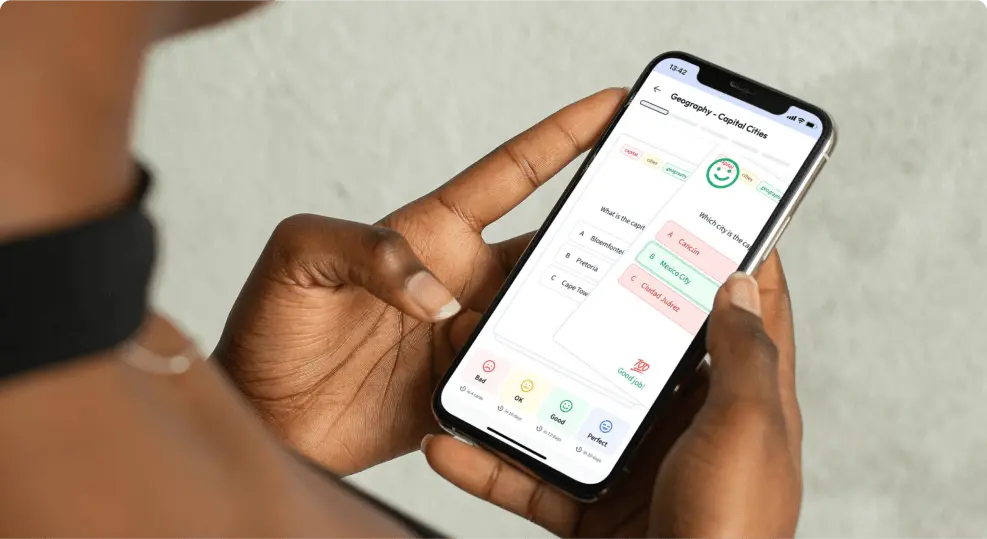
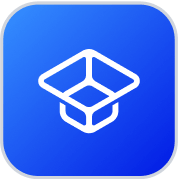
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more