Jump to a key chapter
Introduction to Quicksort
Quicksort is a highly efficient sorting algorithm, known for its speed and simplicity in sorting large datasets with impressive time complexity. This technique divides the data into partitions and optimizes the sorting process by reducing unnecessary comparisons.
Understanding the Basics
When you delve into the world of algorithms, understanding Quicksort is crucial. It works through a mechanism called 'divide and conquer,' where the list is partitioned into smaller sections. This method ensures that sorting is performed only within the necessary segments, leading to higher efficiency.
Quicksort is a sorting algorithm that utilizes a divide and conquer approach to sort elements by partitioning arrays into smaller sub-arrays.
Consider a simple list: [7, 2, 1, 6]. By implementing Quicksort, the list undergoes partitioning several times until it becomes [1, 2, 6, 7], sorted in ascending order.
Quicksort can be implemented both recursively and iteratively, with recursion being more common for simpler and concise code.
Implementing Quicksort in Python
Implementing Quicksort in Python involves creating a function that calls itself recursively to sort the list. Python's flexibility with lists makes it an ideal language for demonstrating this algorithm. Below, you'll find a basic implementation.
In a standard Quicksort implementation, the performance and speed optimization come from choosing the right 'pivot'. The pivot is the element from which partitioning begins. If chosen poorly, it can lead to an imbalanced tree structure, resulting in higher time complexity. Ideally, the pivot should divide the array into two ideally equal parts.
def quicksort(arr): if len(arr) <= 1: return arr else: pivot = arr[0] less_than_pivot = [x for x in arr[1:] if x <= pivot] greater_than_pivot = [x for x in arr[1:] if x > pivot] return quicksort(less_than_pivot) + [pivot] + quicksort(greater_than_pivot)array = [10, 7, 8, 9, 1, 5]print(quicksort(array)) # Output will be [1, 5, 7, 8, 9, 10]
In this implementation, each pivot divides the list into two parts: those less than and greater than the pivot. These parts are then recursively sorted until the array is completely ordered. Note the use of Python’s list comprehension for efficient creation of sub-lists.
Remember, Quicksort's average time complexity is O(n log n), but in the worst case, it can degrade to O(n2). Choosing a good pivot can help mitigate this risk.
Quicksort Algorithm Python
The Quicksort algorithm is an essential tool in computer science for efficient data sorting. Its design is based on the divide-and-conquer technique, providing speed and reliability for handling large datasets. Quicksort operates by partitioning an array into smaller sub-arrays and recursively sorting them.
Understanding Quicksort Fundamentals
The Quicksort algorithm works by selecting a 'pivot' element from the array, around which the other elements are rearranged. Here is a quick breakdown of its process:
- Choose a pivot element from the array.
- Reorder the array to ensure all items below the pivot come before it, and all items above come after it.
- Recursively apply the above steps to the sub-arrays formed on either side of the pivot.
To delve deeper, the choice of pivot can greatly influence performance. Ideally, a well-chosen pivot subdivides the array into two equal parts, driving the algorithm towards an average time complexity of \(O(n \log n)\). However, in the worst-case scenario where partitions are highly unbalanced, the complexity degrades to \(O(n^2)\).
# Example of Quicksort in Pythondef quicksort(arr): if len(arr) <= 1: return arr else: pivot = arr[0] less_than_pivot = [x for x in arr[1:] if x <= pivot] greater_than_pivot = [x for x in arr[1:] if x > pivot] return quicksort(less_than_pivot) + [pivot] + quicksort(greater_than_pivot)array = [12, 4, 5, 6, 7, 3, 1, 15]print(quicksort(array)) # Output will be [1, 3, 4, 5, 6, 7, 12, 15]
In practice, choosing the median value of the array as the pivot can help in improving the performance of Quicksort.
The power of Quicksort lies in its flexibility and adaptability. Python simplifies the implementation of Quicksort using list comprehensions and recursion, allowing an elegant and concise expression of this algorithm.
Quicksort in Python can be succinctly implemented using recursion and list comprehensions to effectively sort elements by organizing them around a pivot and recursively sorting the partitions.
Quicksort Implementation Python
Implementing Quicksort in Python allows for efficient sorting of elements through recursive partitioning. Python's intuitive syntax makes it an excellent choice for demonstrating this algorithm.
Python Quicksort Method
In Python, Quicksort can be implemented by using a recursive function that segregates the elements around a chosen pivot. Here is a straightforward implementation:
def quicksort(arr): if len(arr) <= 1: return arr else: pivot = arr[0] less_than_pivot = [x for x in arr[1:] if x <= pivot] greater_than_pivot = [x for x in arr[1:] if x > pivot] return quicksort(less_than_pivot) + [pivot] + quicksort(greater_than_pivot)array = [10, 7, 8, 9, 1, 5]print(quicksort(array)) # Output will be [1, 5, 7, 8, 9, 10]
The pivotal operation in Quicksort is critical to its performance. While this implementation chooses the first element as the pivot, there are other strategies such as selecting the median or a random element to further optimize sorting.
The choice of pivot in Quicksort affects the algorithm's efficiency. An ideal pivot results in balanced partitions, pushing time complexity towards \(O(n \log n)\). Conversely, poor pivot choices may lead to \(O(n^2)\) time complexity in cases of highly imbalanced partitions. Advanced techniques like 'median-of-three' help in pivot selection by considering the median of the first, middle, and last elements of the partition.
Recursion can lead to stack overflow if the dataset is too large. Iterative Quicksort variations or setting recursion limits might be necessary.
Quicksort is an algorithm that divides less and more significant elements than the pivot recursively into sub-arrays for sorting.
Python Quicksort Code
Understanding the Quicksort algorithm and its implementation is a fundamental skill in computer science. Its presence in Python programming emphasizes the language's ability to handle complex data operations efficiently. Let's explore how you can implement this effective sorting mechanism in Python.
Quicksort Python Example
A Python implementation of Quicksort leverages the language's expressive power through simple syntax and list comprehension. Here's a typical example of how Quicksort can be implemented:
def quicksort(arr): if len(arr) <= 1: return arr else: pivot = arr[0] less_than_pivot = [x for x in arr[1:] if x <= pivot] greater_than_pivot = [x for x in arr[1:] if x > pivot] return quicksort(less_than_pivot) + [pivot] + quicksort(greater_than_pivot)array = [3, 6, 8, 10, 1, 2, 1]print(quicksort(array)) # Output will be [1, 1, 2, 3, 6, 8, 10]
When choosing a pivot, the first element is often chosen for simplicity, but selecting a median can improve sorting efficiency.
Detailed Steps of Quicksort in Python
The Quicksort algorithm follows a clearly defined process. Below are the steps involved:
- Select a pivot element.
- Partition the array into two subarrays: elements less than the pivot and elements greater than the pivot.
- Recursively apply Quicksort to each subarray.
The elegance of Quicksort lies in partitioning the array around a pivot. This revolves around the divide and conquer strategy, ensuring each partition becomes smaller and easier to sort. The efficiency of the algorithm is generally characterized by its average-case complexity of \(O(n \log n)\), making it favorable for substantial datasets. Still, using additional strategies like dual-pivot Quicksort could potentially enhance performance in specific scenarios.
Benefits of Using Quicksort in Python
Quicksort offers numerous benefits, especially when implemented in Python:
- Efficiency: Best and average-case time complexities of \(O(n \log n)\) make it suitable for many applications.
- In-place sorting: Does not require extra storage, making it space-efficient.
- Flexibility: Easy to implement recursively, making the code clean and readable.
- Versatility: Adaptable to various data types and naturally handles arrays and lists in Python.
Common Mistakes in Quicksort Implementation Python
Implementing Quicksort in Python can lead to some common pitfalls. Here are the typical ones to watch for:
- Choosing a poor pivot: Always selecting the first or last element may result in worst-case time complexity \(O(n^2)\) if not careful.
- Excessive stack depth: Recursive calls may lead to stack overflow with large lists; consider an iterative approach if necessary.
- Incorrect partitioning: Failing to correctly separate elements around the pivot can result in unsorted arrays.
- Not handling duplicates: Ensure the algorithm correctly deals with duplicate elements to avoid infinite recursion.
Quicksort in Python is an efficient sorting algorithm that sorts elements by partitioning them into subarrays recursively, using a pivot point.
Quicksort Python - Key takeaways
- Quicksort is a highly efficient sorting algorithm that uses the 'divide and conquer' technique to sort elements by partitioning arrays into smaller sub-arrays.
- Quicksort implementation in Python typically involves creating a recursive function that sorts a list by selecting a 'pivot' element, leading to efficient sorting with average time complexity of O(n log n).
- The choice of pivot is critical to Quicksort's performance, influencing whether the algorithm reaches optimal time complexity or degrades to O(n2) in the worst case.
- A basic quicksort Python example involves selecting a pivot, dividing the array into elements less and greater than the pivot, then recursively sorting these partitions.
- Python quicksort code often uses list comprehensions for efficiently creating sub-lists during sorting implementation.
- Quicksort's benefits in Python include space efficiency (in-place sorting), flexibility with various data types, and ease of readability and implementation through recursion.
Learn faster with the 23 flashcards about Quicksort Python
Sign up for free to gain access to all our flashcards.
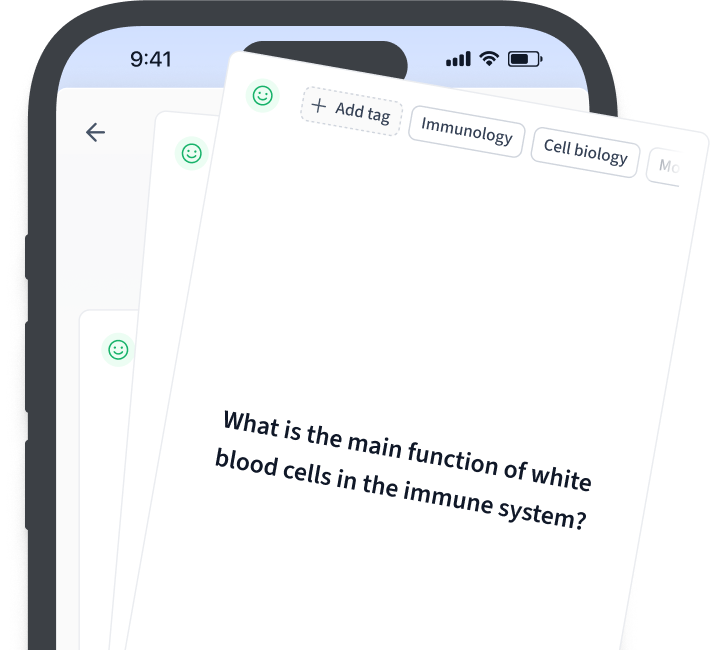
Frequently Asked Questions about Quicksort Python
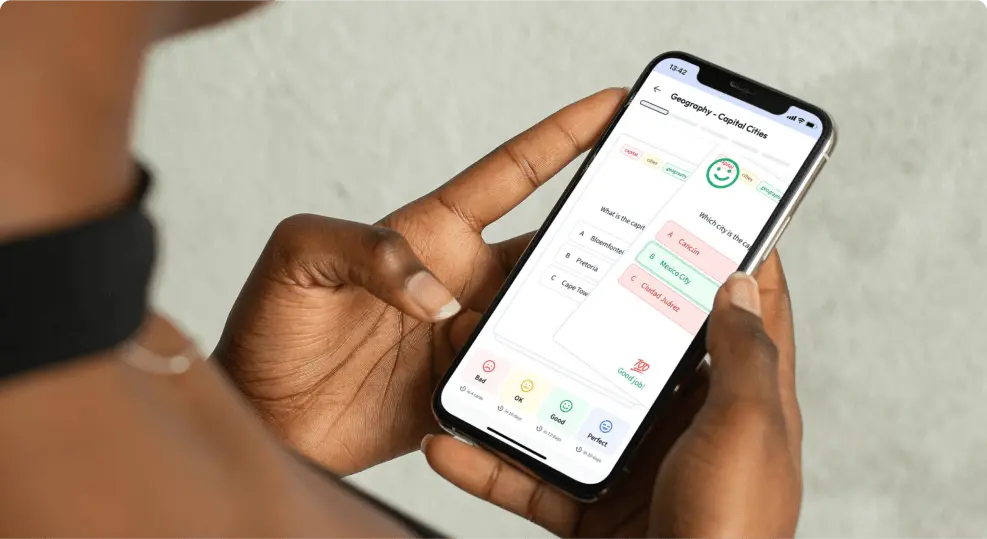
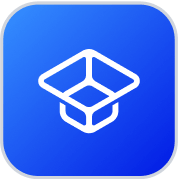
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more