Jump to a key chapter
Introduction to R Programming Language
The R Programming Language is a powerful tool for data analysis and statistical computing. It has gained immense popularity in various fields due to its flexibility and dedicated statistical functionalities.
What is R as a Programming Language?
R is a language and environment specifically designed for statistical computing and graphics. Developed in the early 1990s, R offers a variety of statistical techniques, including linear and nonlinear modeling, time-series analysis, classification, clustering, and more. Unlike some other programming languages that require extensive libraries to perform complex analyses, R integrates these capabilities inherently within its base environment.The code structure in R is flexible, enabling easy integration with other technologies and systems. Here's an example of a simple R code to create a basic plot:
plot(cars)In this example, the cars dataset, which comes built into R, is used to generate a scatter plot.Moreover, R supports a wide range of data types and data structures:
- Vectors - One-dimensional arrays that store data of the same type.
- Data Frames - Two-dimensional data structures equivalent to tables, where different columns can contain different types of data.
- Lists - A versatile data type that can hold elements of various types.
R Programming Language: An open-source language primarily used for statistical analysis and visualizing data, known for its extensive statistical functionalities and graphical capabilities.
R is particularly popular among statisticians, data scientists, and academia due to its straightforward syntax and vivid visualization tools.
Benefits of Learning the R Programming Language
There are numerous benefits to learning R, particularly if you're interested in data analysis or statistics. Below are some significant advantages:
- Open Source: R is free to download and use, making it accessible for anyone with a computer.
- Comprehensive Packages: R offers thousands of packages to extend its functionalities, specifically in statistical and graphical analysis. Popular packages include ggplot2 for data visualization and dplyr for data manipulation.
- Community Support: With a large user base, R boasts plenty of forums, tutorials, and guides for learning and problem-solving.
- Data Visualization: R provides rich visual tools that are essential for data analysis and interpretation.
model <- lm(mpg ~ wt + hp, data=mtcars)Here, a linear regression model is created to understand the relationship between miles per gallon (mpg), vehicle weight (wt), and horsepower (hp). This simple implementation exemplifies R's capability to apply complex statistical algorithms effortlessly.
While R is highly targeted at statistical analysis, it is also capable of connecting with databases, performing data wrangling, and communicating with other programming languages like Python and C++. This interdisciplinary compatibility enhances its applicability in various industries.R's aptness comes from its focus on three core features:
- Computation: Handling a massive volume of data, thanks to strong statistical capabilities.
- Visualization: Supporting high-quality visualizations to aid data interpretation.
- Reproducibility: Offering tools that help ensure your results and analyses are consistent and replicable over time.
Fundamentals of R Programming
R Programming is essential for anyone delving into data analysis. It is recognized for its powerful statistical capabilities and user-friendly graphical interface. Let's explore the basic syntax and the essential data types that form the foundation of programming in R.
Basic R Programming Syntax and Functions
Understanding the basic syntax of R is crucial for manipulating data and executing commands effectively. R's syntax is known for being straightforward and intuitive. Here are some fundamental components:
- Variable Assignment: In R, you can assign values to variables using the
<-
operator.x <- 10
- Comments: Use the
#
symbol to include comments in your code.# This is a comment
- Printing Output: Use the
print()
function to display output.print(x)
- mean(): Computes the average of a set of numbers.
- sum(): Calculates the sum of values.
- seq(): Generates sequences of numbers.
result <- mean(c(10, 20, 30, 40))print(result)This simple use of the mean() function demonstrates how concise R code can be for statistical computing.
Example: To generate a sequence of numbers from 1 to 10 in R, you can use the seq() function:
sequence <- seq(1, 10)print(sequence)
Remember, R is case-sensitive. Therefore, VariableName and variablename would be treated as distinct names.
Essential Data Types and Structures in R
R's data types and structures are diverse, allowing the handling of complex datasets with ease. Below are the principal data types you will encounter:
- Numeric: Represents numbers, including integers and decimals.
- Character: Represents text strings.
- Logical: Represents TRUE or FALSE.
- Vectors: Homogeneous data structures that store elements of the same type.
- Data Frames: Two-dimensional, tabular data structures that allow storage of different types of data in columns.
- Lists: Can contain elements of various types, making them very flexible.
my_data <- data.frame( Name = c("Alice", "Bob", "Carol"), Age = c(25, 30, 35), Score = c(90, 85, 88))print(my_data)This will produce a table-like structure where names, ages, and scores are organized into columns.
Data Frame: A table or a two-dimensional array-like structure in R that can store different types of data across columns.
R's data handling capabilities also extend to matrix operations. Unlike data frames, matrices store elements of the same type in a two-dimensional format. They are particularly useful in mathematical calculations and linear algebra. To illustrate:
matrix_example <- matrix( c(1, 2, 3, 4, 5, 6), nrow = 2, ncol = 3)print(matrix_example)Here, matrix() creates a 2x3 matrix filled by the numbers 1 to 6 in a column-major order, demonstrating how R can efficiently handle and perform calculations on matrix data.
R Programming Concepts Explained
The R Programming Language is renowned for its data analysis and visualization capabilities. In this section, you'll explore its core concepts and techniques, which are fundamental for effectively utilizing R for statistical computations.
Key R Programming Concepts and Techniques
To make the most of R Programming, understanding its key concepts and techniques is vital. Here are some of the fundamental elements:
- Data Manipulation: R provides several packages like dplyr and tidyverse to reshape and filter data efficiently.
- Data Visualization: The ggplot2 package is one of R’s most powerful tools for creating high-quality graphs and plots.
- Statistical Analysis: R is equipped with numerous functions for statistical tests and predictive modeling, such as lm() for linear modeling.
- Programming Functions: Custom functions can be created to enhance the reusability of code in R.
library(dplyr)filtered_data <- my_data >%>% filter(Age > 25) select(Name, Score)In this code snippet, filter() is used to extract entries where Age is greater than 25, while select() filters specific columns like Name and Score. This showcases how dplyr simplifies data manipulation.
dplyr Package: An R package that provides a consistent set of functions to solve common data manipulation challenges.
R’s extensibility allows the integration of multiple packages to perform complex analyses with ease. A significant feature is the piping operator %>%
introduced by tidyverse, which streamlines chaining commands. This operator strengthens readability by reducing the nesting of functions, leading to more intuitive code.
result <- my_data >%>% group_by(Category) >%>% summarize(Total = sum(Value))In this example, data is grouped by Category and summarized to calculate total Value across each group. Such piping enhances the clarity of data analysis processes.
Embrace the use of R packages. They are continuously being updated and expanded by the R community to meet diverse analytical needs.
Understanding R Programming Environment
Navigating the R programming environment effectively is crucial for productivity in data analysis. The R environment includes a variety of components and utilities:
- R Console: The command-line interface where R commands are executed. It is ideal for quick calculations and small scripts.
- RStudio: An integrated development environment (IDE) for R that provides user-friendly tools and features to write scripts, debug, plot, and manage packages.
- R Scripts: Text files containing sequences of R commands that can be executed together. This is useful for projects and reproducible research.
- Package Management: R allows easy installation, updating, and management of packages through commands like install.packages().
install.packages("ggplot2")This command installs the ggplot2 package, adding advanced plotting capabilities to your R session.
RStudio's extensive visualization and debugging tools significantly expedite the development process in R.
R Programming Language Course
Embarking on a course in the R Programming Language offers a structured way to gain proficiency in a tool that is essential for data science and statistics. This course is designed to provide you with the foundational knowledge needed to perform data analysis and graphical representations effectively using R.
Learning Path and Recommended Resources
To master the R Programming Language, it’s beneficial to follow a structured learning path coupled with reliable resources. Here’s a step-by-step guide:
- Start with the basics: Understand R syntax, variables, and basic operations like arithmetic computations and logical conditions.
- Data Structures: Learn about vectors, lists, matrices, and data frames to handle complex datasets.
- Data Manipulation: Explore data reshaping techniques using packages such as dplyr and tidyr.
- Data Visualization: Practice creating visually appealing graphs with ggplot2.
- Statistical Analysis: Implement basic statistical tests and models through built-in functions.
- Practice through projects: Engage in real-world projects to apply your skills in data analysis.
Example Resource: Consider using online learning platforms like Coursera or DataCamp, which provide a structured curriculum and hands-on projects for practice.
Join R programming communities such as R-Bloggers or Stack Overflow for peer support and insights.
R Programming offers a diverse ecosystem of packages, enabling learners to expand their skillset significantly. Throughout your learning path, make a conscious effort to explore:
- Specialized packages for industry-specific applications (e.g., quantmod for finance data analysis).
- Interactivity with other programming languages, like connecting Python scripts using the reticulate package.
- Machine Learning packages such as caret for predictive modeling tasks.
Practical Applications in R Programming Language
R Programming excels in various practical applications, especially in fields requiring rigorous data analysis and visualization. Understanding and implementing these applications will provide a comprehensive grasp of R's capability and flexibility.
Key applications of R Programming include:
- Data Analysis: R is adept at statistical analysis and handling large datasets, making it a staple in fields like research, academia, and healthcare.
- Data Visualization: With packages like ggplot2, R allows users to create detailed and publication-quality plots and graphs.
- Financial Modeling: Financial analysts frequently use R for forecasting, risk management, and quantitative modeling.
Data Analysis: The process of inspecting, cleansing, transforming, and modeling data with the goal of discovering useful information, conclusions, and supporting decision-making.
R's adaptability extends to bioinformatics, where it's utilized to analyze genomic data, and epidemiology, which benefits from its statistical prowess. The interoperability of R with databases and other languages like SQL for data extraction enhances its application scope.One advanced use of R is in text mining and natural language processing. Using packages like tm and wordcloud, R can process and extract insights from textual data. Here's a snippet illustrating the creation of a word cloud:
library(tm)library(wordcloud)docs <- Corpus(VectorSource(text_data))tdm <- TermDocumentMatrix(docs)m <- as.matrix(tdm)wordcloud(words = names(m), freq = rowSums(m))Through this kind of application, R demonstrates its capability to uncover patterns and trends from diverse data types, showcasing its versatility and essential role in modern data science practices.
R Programming Language - Key takeaways
- R Programming Language: An open-source language used primarily for statistical analysis and data visualization, known for its comprehensive statistical and graphical capabilities.
- Fundamentals of R Programming: Understanding R involves mastering its syntax, variable assignment, data types such as vectors, data frames, and lists, as well as utilizing functions like
mean()
andlm()
for statistical computations. - R Programming Concepts Explained: Key concepts include data manipulation with packages like dplyr, data visualization with ggplot2, statistical analysis, and creating programming functions.
- R as a Programming Language: Designed for statistical computing, it inherently includes techniques like linear modeling, time-series analysis, etc., without needing extensive external libraries.
- R Programming Syntax and Functions: R's syntax is straightforward with functions for diverse tasks, aiding in statistical computing and data manipulation, using operators like
<-
for assignment and%>%
for piping. - R Programming Language Course: Courses provide foundational knowledge in R syntax, data structures, manipulation, visualization, statistical analysis, and integration with other languages, often using resources from platforms like Coursera.
Learn faster with the 27 flashcards about R Programming Language
Sign up for free to gain access to all our flashcards.
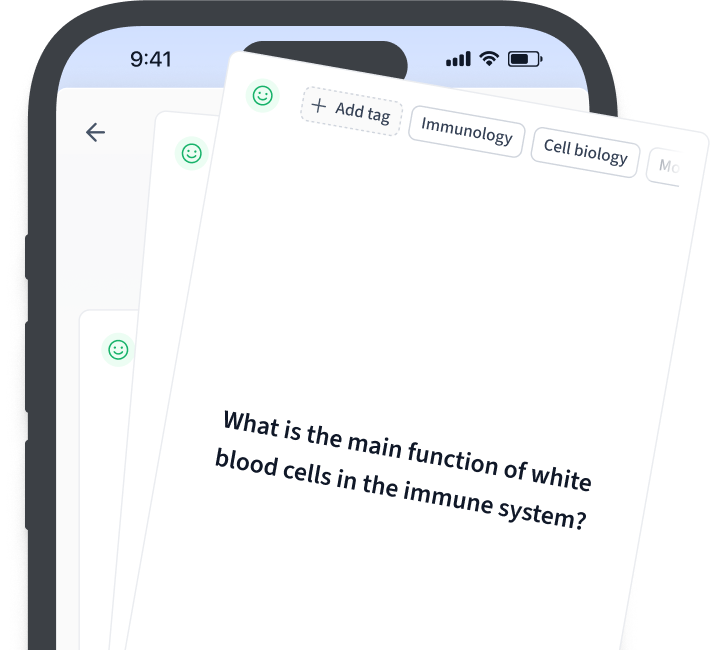
Frequently Asked Questions about R Programming Language
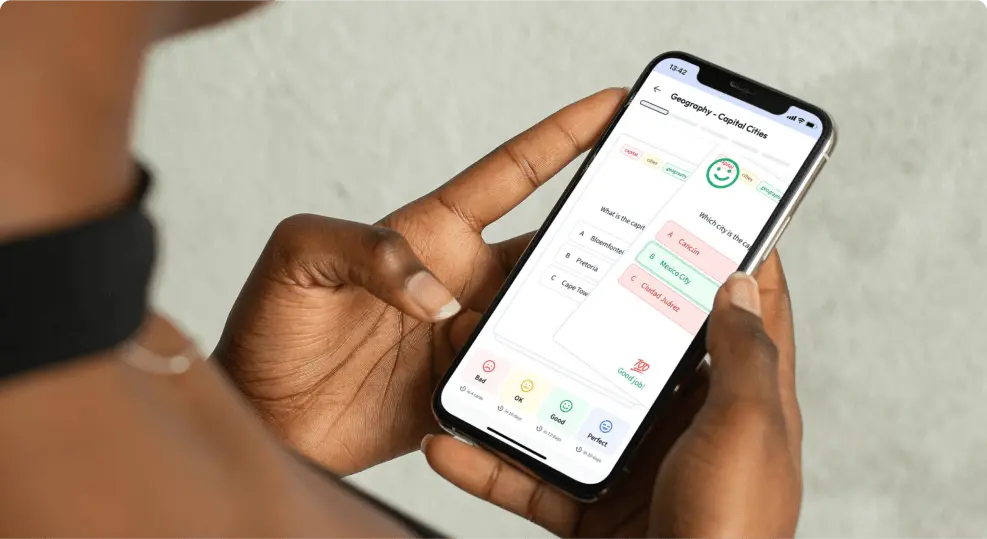
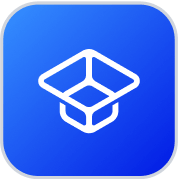
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more