Jump to a key chapter
What Is a Runtime System? Understanding the Definition
Exploring the concept of a runtime system unlocks a fundamental aspect of computer science that is vital for the execution of software applications. This introduction serves as your gateway to understanding the mechanics behind program execution and the environment facilitating it.
The Basic Definition of a Runtime System
A runtime system, at its core, represents the collection of software services provided to an application while it is running. It acts as an intermediary between the running application and the underlying operating system, managing responsibilities such as memory allocation, handling system calls, and ensuring the smooth operation of the software during execution.
Runtime System: A framework providing program execution services, which includes memory management, input/output operations, and invoking system calls, among others.
Consider a simple Python program that prints "Hello, World!" to the console. The Python runtime system, in this case, interprets the script, allocates memory for the program's execution, and manages the output to display the message.
print("Hello, World!")
How a Runtime System Differs From a Compiler
Understanding the distinction between a runtime system and a compiler is crucial for grasping the broader picture of how programs come to life. While both play pivotal roles in the software development lifecycle, they serve fundamentally different purposes.
A compiler transforms source code written in a high-level programming language into machine language, creating an executable program. This process occurs before the program is run. On the other hand, a runtime system provides necessary services and support to the executable program during its execution. Essentially, the compiler readies the program, and the runtime system manages its life during execution.
Deep Dive into Compiler Operations:A compiler undergoes several steps to transform source code into an executable format. These steps include lexical analysis, parsing, semantic analysis, optimisation, and code generation. Each step plays a critical role in understanding the source code, optimising its performance, and ultimately producing a machine-readable file.
Source Code -> Lexical Analysis -> Parsing -> Semantic Analysis -> Optimisation -> Code Generation -> Executable
It's fascinating to note that some programming environments blend aspects of runtime systems and compilers, such as Java, which uses a 'Just-In-Time' compiler within its runtime environment to enhance performance.
Exploring Runtime System Examples in Daily Computing
When delving into runtime systems, examples from widely-used programming languages like Java and Python provide illuminating insights into how applications execute in real-world scenarios.
Runtime System in Java: A Common Example
The Java runtime system, also known as the Java Runtime Environment (JRE), is a quintessential example of a runtime system piecing together various components essential for Java applications to run. This encompasses the Java Virtual Machine (JVM), core libraries, and other necessary components.
An example of Java code running on a runtime system looks like this:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Upon execution, the JRE invokes the JVM to interpret the bytecode generated from the compiled Java source code. The JVM then allocates memory, performs necessary optimisations, and manages the execution environment to print "Hello, World!" to the console.
The versatility of the JVM allows Java programs to run on any device that has the JRE installed, making Java a highly portable language.
Runtime System for Python: How It Works
Python's runtime system differs from Java's, as Python is an interpreted language and does not require separate compilation to bytecode. The Python runtime system involves the Python interpreter and standard libraries that provide a rich set of functionalities during program execution.
Consider a sample Python script:
def greet(): print("Hello, World!") greet()
In this case, the Python interpreter reads and executes the script line by line. It manages memory allocations, function calls, and the execution stack to display "Hello, World!" on the console.
Understanding Python's Memory Management:Python’s runtime system includes an intricate memory management system that handles memory allocation, garbage collection, and the Python object lifecycle. This system ensures efficient memory use and aids in the smooth execution of Python scripts. For instance, the automatic garbage collector recycles memory that is no longer in use, preventing memory leaks.
Unlike Java, Python's easy-to-read syntax and dynamic typing make it an incredibly popular choice for beginners and experts alike, with its runtime system playing a key role in its versatility and widespread use.
Delving Into Runtime System Memory Management
Memory management is a pivotal aspect of runtime systems that ensures the efficient allocation and deallocation of memory during the execution of programs. It stands as the backbone for maintaining application performance and stability.
Understanding Garbage Collection in Runtime Systems
Garbage collection is a form of automatic memory management found in runtime systems. It helps to reclaim memory occupied by objects that are no longer used by a program, thus preventing memory leaks and optimizing the allocation of memory resources.
Garbage Collection: An automated process within a runtime system that identifies and frees memory that is no longer in use by the application.
An illustration of garbage collection can be seen in the context of Java:
public class Test { public static void main(String[] args) { String str1 = new String("Hello"); str1 = null; // The reference to "Hello" is null, making "Hello" eligible for garbage collection } }
In this example, the runtime system identifies "Hello"
as no longer needed and reclaims that memory space.
Various runtime systems implement garbage collection differently, with some using reference counting and others sophisticated algorithms like mark-and-sweep or generational collection.
The Role of Memory Management in a Runtime System
Memory management within a runtime system is multifaceted, aiming to efficiently allocate, use, and reclaim memory. It not only involves garbage collection but also encompasses other tasks such as allocation strategies, handling stack and heap memory, and avoiding memory fragmentation.
Effective memory management ensures that applications run smoothly without unnecessary slowdowns or crashes due to memory misuse. This includes:
- Allocating memory space for objects and variables.
- Tracking the lifecycle of each object to identify when they are no longer in use.
- Reclaiming the memory space used by objects that are no longer needed.
Such management is vital for long-running applications, where inefficient memory usage can lead to performance degradation over time.
The intricacies of memory management strategies are profound. For instance, stack memory allocation is fast and managed by the computer's CPU but limited in size and scope. In contrast, heap memory is more flexible but requires explicit allocation and deallocation, often leading to fragmentation if not managed properly. Understanding these distinctions is crucial for developers to optimise their applications' memory usage and performance.
Comparing Runtime vs Compile Time: What’s the Difference?
Understanding the distinctions between runtime and compile time is crucial for grasping how software applications are developed and executed. These phases encompass distinct processes in the software development lifecycle, each playing a vital role in transforming code into a functioning program.
How Runtime System Behaviours Contrast With Compile Time
At compile time, source code is translated into machine code by a compiler, a process essential for preparing a program for execution. This translation happens once, turning high-level language commands into a language that the computer's hardware can understand.
In contrast, runtime concerns itself with the events that occur while the program operates. The runtime system is thus the environment in which a program executes, handling tasks like memory management, input/output operations, and executing compiled code.
Compile Time: The phase in the software development process where source code is converted into executable code by a compiler.
Runtime: The period when a program is executing, involving tasks managed by the runtime system such as memory allocation and execution of code.
Consider a scenario where a Java program is compiled. At compile time, the Java compiler (javac
) takes human-readable code and converts it into bytecode (.class
files), which is a platform-independent code. At runtime, the Java Virtual Machine (JVM) executes this bytecode, managing memory and executing instructions.
The errors detected at compile time typically involve syntax or semantic issues, while runtime errors are often related to logical issues or resources that are only available during execution.
The Impact of Runtime Systems on Program Performance
While compile time sets the stage for a program's operation by translating code, runtime systems directly influence performance during execution. Efficient runtime systems optimise memory usage, manage resources carefully, and ensure the correct execution of operations.
Factors inherent to the runtime system, such as garbage collection, just-in-time (JIT) compilation and resource allocation strategies, have profound effects on program performance. For instance:
- Garbage collection mechanisms can free up memory space, but if not optimised, they can cause performance hitches.
- JIT compilers can significantly speed up program execution by compiling bytecode to machine code on-the-fly.
- Resource allocation strategies affect how efficiently a program utilises system resources.
The performance implications of runtime systems are not only theoretical. For example, Android applications running on the Dalvik virtual machine experienced noticeable performance improvements with the switch to the Android Runtime (ART), which uses ahead-of-time (AOT) compilation to convert bytecode into machine code during application installation rather than at runtime.
Runtime System - Key takeaways
- Runtime System Definition: A runtime system provides program execution services such as memory management, input/output operations, and invoking system calls while the application is running.
- Runtime System Example: In Python, the runtime system interprets the script line by line and manages memory allocations, function calls, and the execution stack.
- Garbage Collection in Runtime Systems: Garbage collection is an automated process that identifies and frees memory no longer in use by the application, preventing memory leaks and optimising memory resource allocation.
- Runtime System in Java: The Java Runtime Environment (JRE) includes the Java Virtual Machine (JVM) which interprets bytecode, optimises execution, and handles memory for Java applications.
- Runtime vs Compile time: Runtime involves the program's operation and the execution environment provided by the runtime system, while compile time refers to when source code is converted into an executable format by a compiler.
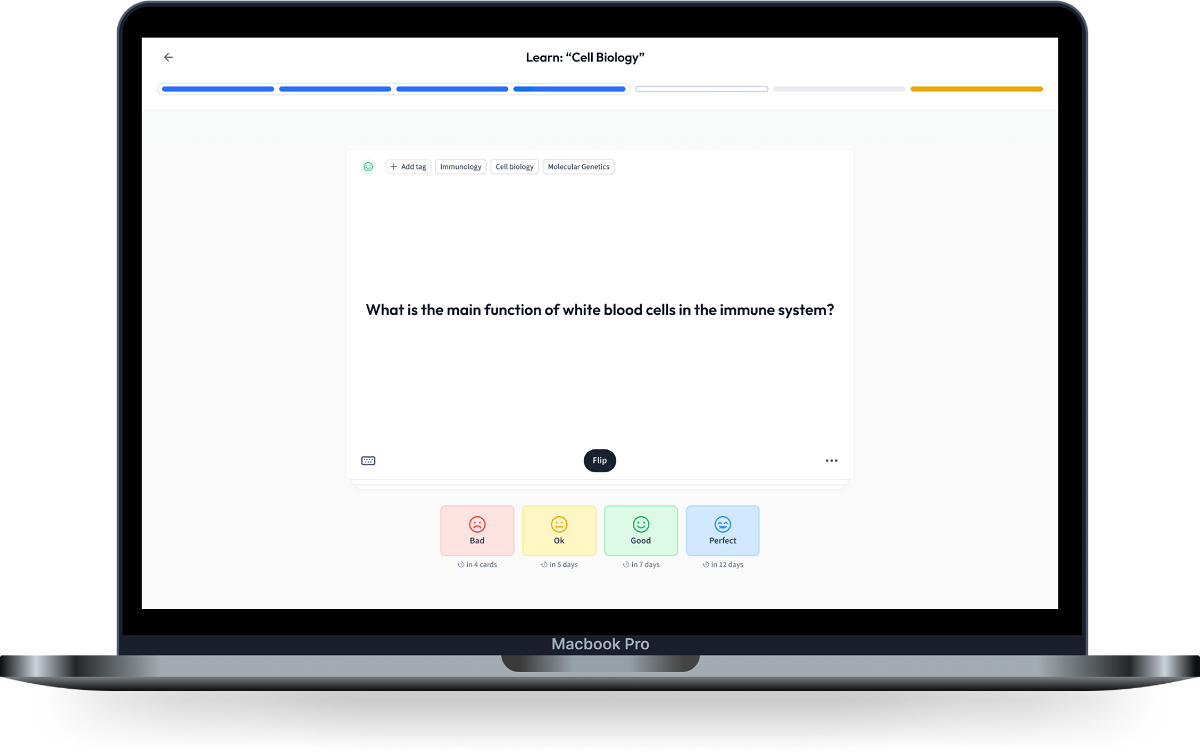
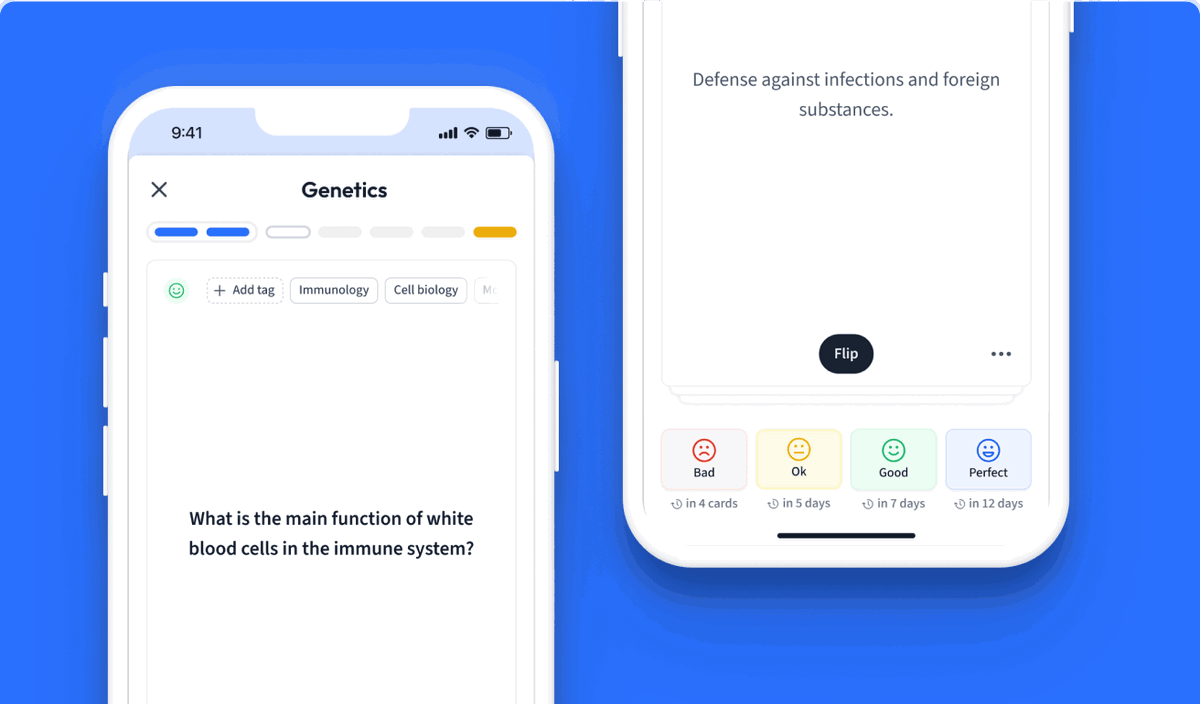
Learn with 27 Runtime System flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Runtime System
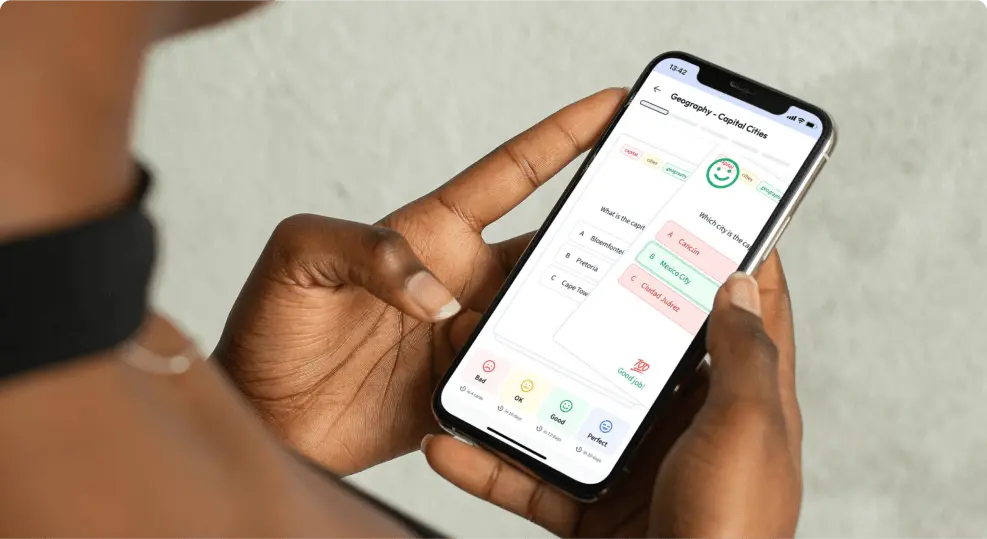
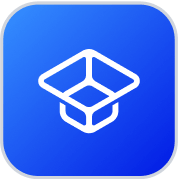
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more