Jump to a key chapter
Basics of Bit Shift Operator in C
In order to fully understand the concept of Shift Operator in C, it's essential to begin by grasping the basics of bitwise operations. A bitwise operation is used to manipulate individual bit patterns, which is a fundamental aspect in C programming. When you have a thorough understanding of bitwise operations, it becomes easier to comprehend the purpose and functionality of the Shift Operator C.
Bit Shift Operators are employed to move a set of positional bits left or right, which can be particularly useful for performing arithmetic operations, such as multiplication or division. These operators have two main categories: the left shift (<>). The shift length depends upon the number of positions you intend to move the bits, and it's essential to be mindful of the operator's behaviour when shifting.
Here are a few basic concepts and operations:
- Left Shift Operator (< The left shift operator moves a binary sequence left by a defined number of places. It shifts the bits to the left and appends zeroes to the vacant positions on the right side. This operation usually implies a multiplication by two for each bit position moved.
- Right Shift Operator (>>): The right shift operator, conversely, moves a binary sequence to the right by a specific number of positions. Depending on the format of the number (signed or unsigned), it can append zeroes or the sign bit (for negative numbers) to the empty positions on the left side. This operation is often used for division by powers of two.
Importance of Binary Shift Operator in C
Binary Shift Operators are essential in various applications within the C programming language, due to their ability to perform specific tasks efficiently. Here are some of the reasons for their importance:
Example: Suppose you need to multiply a number by 8 - instead of using the multiplication operator, you can use the left shift operator to shift the number's bits three positions to the left. This process is significantly faster and consumes less processing power, making it an efficient method for such operations.
- Performance Boost: Bitwise shift operations are more rapid and computationally efficient in comparison to traditional arithmetic operations. Therefore, they are frequently utilized to optimize applications and improve performance.
- Memory and Space Efficiency: Bit manipulation using Shift Operators can reduce the memory usage of certain algorithms by compressing data structures or eliminating the need for additional data storage. This can make a significant difference in terms of memory management and storage.
- Faster Processing: These operators allow programmers to execute arithmetic operations like multiplication and division by powers of two at a faster speed. This swift execution can increase the overall efficiency of a program.
- Hardware-level Control: Shift operators can provide programmers with direct access and control over individual bits. As a result, it becomes easier to employ hardware-level optimizations and interact with low-level devices or hardware components, a crucial aspect in embedded systems and device drivers.
As you can see, the binary Shift Operators in C are essential for optimizing code performance, controlling the hardware level operations, and improving the overall efficiency of a program. As a student of computer science, mastering the usage of these operators is essential for writing optimal code.
Difference Between Shift Operator in C and C++
While both C and C++ programming languages share similarities, particularly in syntax and operations, there are some differences that a developer should be aware of when working with Shift Operators.
The main differences between Shift Operators in C and C++ are in how they handle undefined behaviour and the new features offered by C++ regarding overloading and templates.
- Undefined Behaviour: In C, the behaviour for left-shifting negative numbers is considered undefined. However, C++ follows strict rules for this operation. In C++, left-shifting a negative number by a positive number of positions is well-defined and results in a positive value, as long as the result fits the type.
- Operator Overloading: C++ offers the ability to overload operators, such as the left-shift operator (<
- Templates: C++ features templates that provide support for generic programming, enabling functions and classes to work with multiple data types. When implemented, the left-shift operator can also be used within template functions in C++. C, without this feature, does not offer such flexibility.
Implementing Left Shift Operator in C++ Programming
In C++ programming, the Left Shift Operator (<
Example: A simple use of the Left Shift Operator (< #include
- Arithmetic: Similar to C, the Left Shift Operator in C++ is frequently used for arithmetic operations like multiplication and division by powers of 2. This can be achieved by shifting bits to the left or right for signed and unsigned integer types.
- Operator Overloading: As mentioned earlier, C++ enables overloading of the Left Shift Operator (<
- Templates: Implementing C++ templates allows for generic programming, and the Left Shift Operator can be incorporated within template functions, extending its application and making it more adaptable. This facilitates the creation of reusable code that works seamlessly with various data types.
In conclusion, the Left Shift Operator in C++ provides valuable functionality for arithmetic operations, and with the help of operator overloading and templates, it becomes even more versatile, enabling its use with an assortment of data types and enhancing code readability. As a computer science student, it's important to understand the intricacies of such operators to build efficient and well-structured code.
Practical Shift Operator Examples in Computer Programming
Shift Operators in C programming have a wide range of real-world applications, from microcontroller-based systems to low-level hardware manipulation. To better understand when and how Shift Operators are used, let's delve into some examples and scenarios that showcase their practical usage:
- Bitwise Operations: Many hardware devices and peripherals communicate using bitwise protocols. Shift Operators can help programmers to read and manipulate individual bits efficiently. For example, reading a status bit from a register, or setting/resetting specific bits in a control register without affecting the other bits.
- Sorting Algorithms: In computer programming, sorting algorithms can benefit from Shift Operators. One such sorting algorithm is the Radix Sort, where bits are processed from the least significant digit (LSD) to the most significant digit (MSD). Shift Operators play a crucial role in manipulating and comparing bit positions while sorting.
- Bit Manipulation Techniques: Many advanced computer programming techniques involve bit manipulation, such as using bitwise AND, NOR, and XOR operations. Shift Operators provide an efficient way of performing these operations without the need for complex or computationally expensive calculations.
- Hash Functions: Shift Operators are commonly used in hash function implementations, where the goal is to generate unique keys from input data. Using bitwise operations, hash functions can distribute unique keys uniformly across the available key space, providing faster access and improved storage efficiency.
Analysing Shift Operator Example Code and Outputs
To illustrate how Shift Operators work in practice and their effect on binary data, let's analyse some example code snippets and their corresponding outputs:
Example 1: Left Shift Operator/Multiplication by 2³.
#includeint main() { int num = 4; int shift_by = 3; int result = num << shift_by; //This operation will multiply `num` (4) by 2^3 (8) printf("The result is : %d\n", result); //Output: "The result is : 32" return 0; }
In this example, the Left Shift Operator multiplies the value of 'num' (4) by 2 raised to the power of 3 (8), resulting in a value of 32. This illustrates how the Left Shift Operator effectively and quickly multiplies a number by a power of 2.
Example 2: Right Shift Operator/Division by 2².
#includeint main() { int num = 24; int shift_by = 2; int result = num >> shift_by; //This operation will divide `num` (24) by 2^2 (4) printf("The result is : %d\n", result); //Output: "The result is : 6" return 0; }
In this second example, the Right Shift Operator divides the value of 'num' (24) by 2 raised to the power of 2 (4), resulting in a value of 6. This demonstrates how the Right Shift Operator effectively and quickly divides a number by a power of 2.
Both examples provide insight into the efficiency and speed of Shift Operators in C when performing arithmetic operations. As a computer science student, understanding the practical implementation and benefits of these operators in real-world scenarios is essential for developing efficient and well-structured code.
Shift Operator C - Key takeaways
Shift Operator C: allows developers to efficiently manipulate data at the bit level with left (<>) shift operators.
Bit Shift Operator in C: commonly used for arithmetic operations, such as multiplication or division by powers of 2.
Left Shift Operator C++: utilized for arithmetic operations, operator overloading, and within template functions for enhanced code versatility.
Shift Operator Example: perform arithmetic operations like multiplication and division, bitwise operations, and use in sorting algorithms and hash functions.
Shift Operator in C explained: efficient method for arithmetic operations, memory management, and low-level hardware control.
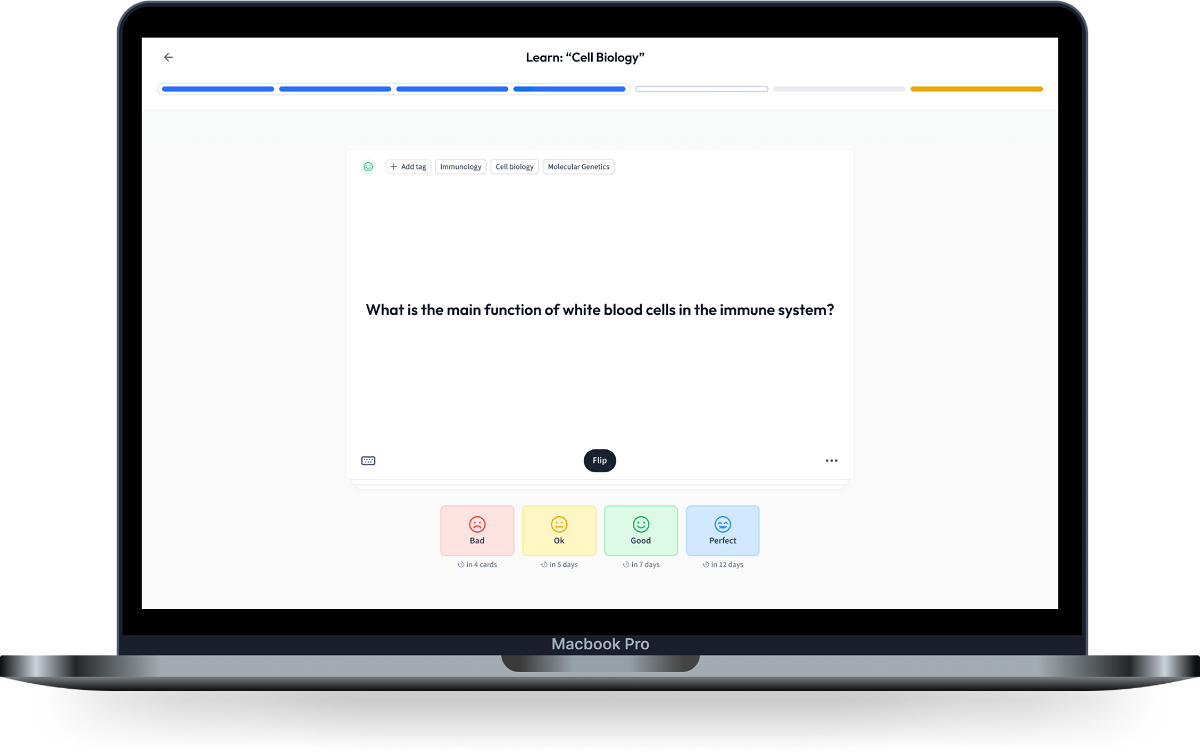
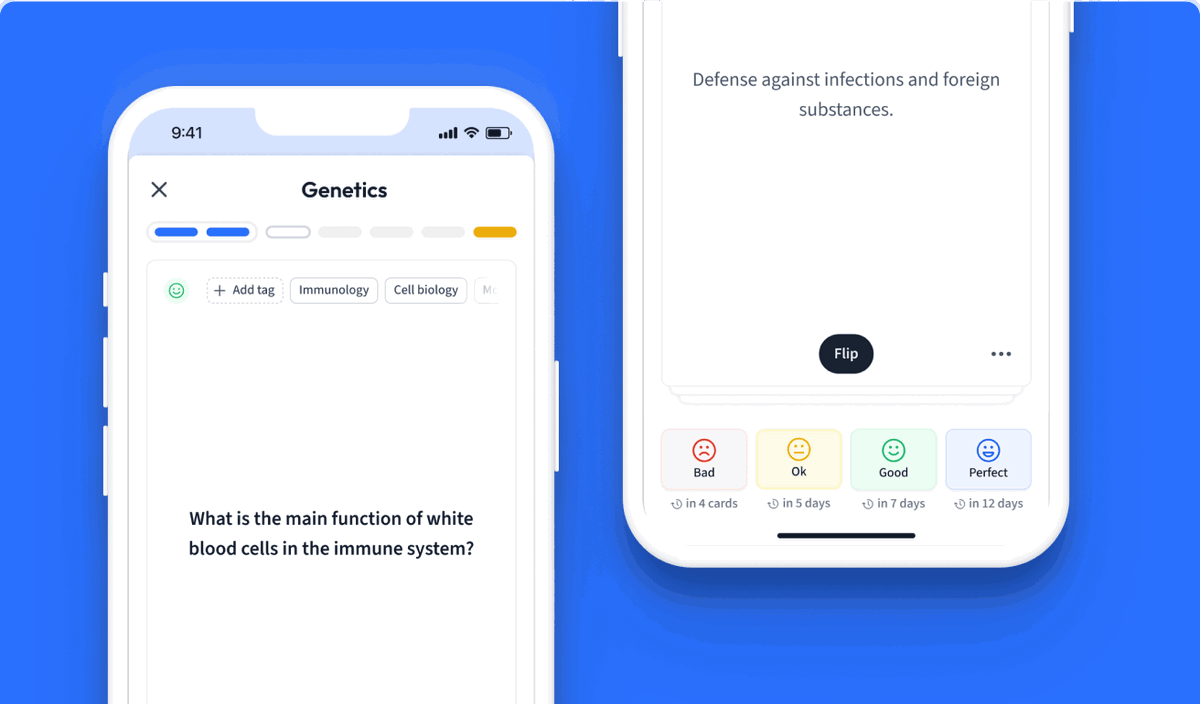
Learn with 15 Shift Operator C flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Shift Operator C
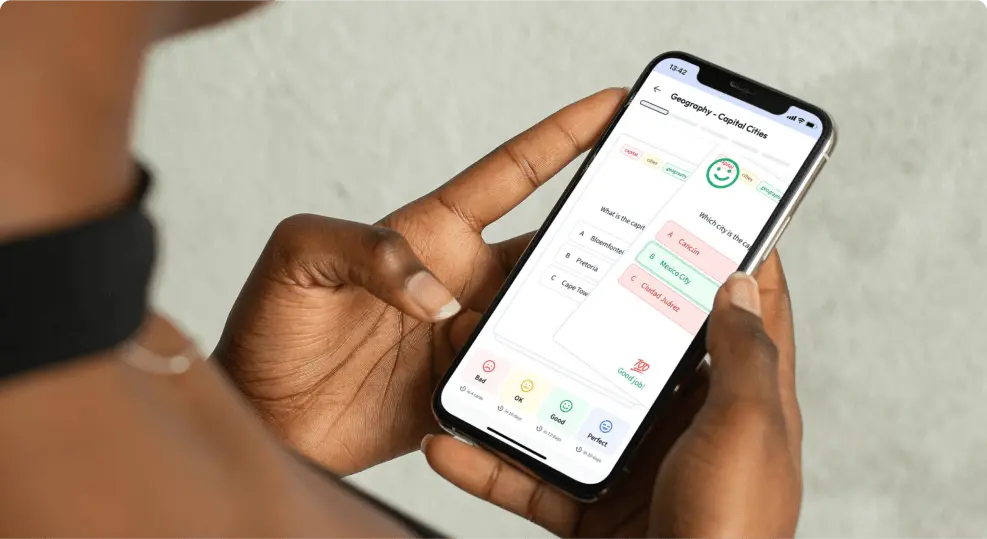
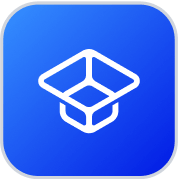
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more