Jump to a key chapter
Definition: What is a Syntax Error?
A syntax error occurs when the source code of a computer program contains mistakes or does not adhere to the rules and grammar of the programming language. These errors prevent the program from compiling and executing properly. Syntax errors are identified by compilers or interpreters when translating the source code into machine code or when executing it.
Common Types of Syntax Errors
There are several common types of syntax errors that programmers often encounter. Some of the most frequently occurring syntax errors include:
- Missing or extra parentheses
- Missing or extra braces
- Mismatched quotes
- Missing or misplaced semicolons
- Incorrectly nested loops or blocks
- Invalid variable or function names
- Incorrect use of operators
Understanding these common errors can help you diagnose and fix syntax errors more quickly and efficiently.
Syntax Error in C Example
Suppose you are writing a C program to calculate the area of a rectangle. An example of a syntax error in this program might be:
int main() {
int length = 5;
int width = 10
int area = length * width;
printf("The area of the rectangle is %d\n", area);
return 0;
}
Here, the syntax error is the missing semicolon after the declaration and assignment of the 'width' variable. The compiler will raise an error, and the program will not compile until the mistake is corrected.
Expression Syntax Error in C
Expression syntax errors can also occur when using arithmetic, logical, or relational operators incorrectly. An example of an expression syntax error in C:
int main() {
int a = 2;
int b = 4;
int result = a ++ * ++ b;
printf("The result is %d\n", result);
return 0;
}
Here, the error is caused by the incorrect use of the increment operator (++). The correct expression should be:
int result = a++ * ++b;
Correcting the error will allow the program to compile and execute successfully.
Declaration Syntax Error in C
Declaration syntax errors occur when a variable or function is improperly declared. An example of a declaration syntax error in C:
int main() {
int a;
int 1b;
a = 5;
1b = 10;
printf("The value of a is %d and the value of 1b is %d\n", a, 1b);
return 0;
}
The syntax error in this example is the naming of the '1b' variable, as variable names must not start with a number. Changing the variable name to a valid identifier will correct the error:
int b;
a = 5;
b = 10;
printf("The value of a is %d and the value of b is %d\n", a, b);
Once the error is corrected, the program will compile and execute without issues.
Identifying Common Syntax Errors in C
When programming in C, it is essential to be aware of common syntax errors to avoid potential issues in your code. This section will provide insights into frequent syntax errors and their identification process, making it easier for you to locate and rectify them.
Tips for Debugging Syntax Errors
Debugging is the process of locating and resolving errors in your code. To make the debugging process more efficient, follow these tips:
- Examine compiler or interpreter error messages: These messages often provide detailed information about the location and nature of the syntax errors.
- Use descriptive variable and function names: This makes it easier to understand the purpose of each code element and helps you identify potential errors in their usage.
- Indent and comment your code: Proper indentation and comments will enable you to grasp the structure and logic of your code quickly, thus making it easier to identify any syntax errors.
- Break complex expressions into smaller parts: This helps in understanding the logic better, making it easier to locate and fix errors.
- Use a debugger tool: Debugger tools step through your code, helping you locate mistakes efficiently and fix them in real-time.
- Test your code frequently: By testing small sections of your code as they are completed, you will be able to resolve syntax errors earlier in the development process and avoid additional issues later on.
Case Study: Syntax Errors in Code Samples
In this case study, we will examine code samples that contain syntax errors, identify the mistakes, and suggest solutions to fix them.
Code Sample 1:
int main() {
int count = 5
while (count <= 10) {
printf("%d\n", count);
count++;
}
return 0;
}
There are two syntax errors in this code sample:
- Missing semicolon after the 'count' variable declaration.
- Missing parentheses around the condition in the 'while' loop.
To fix these errors, insert a semicolon after the declaration of the 'count' variable and add parentheses around the condition in the 'while' loop:
int main() {
int count = 5;
while (count <= 10) {
printf("%d\n", count);
count++;
}
return 0;
}
Code Sample 2:
int main() {
int i;
int sum = 0;
for (i == 0; i < 10; i++) {
sum += i;
}
printf("The sum of the numbers from 0 to 9 is %d\n", sum);
return 0;
}
In this code sample, there is a syntax error within the 'for' loop declaration:
- Incorrect use of the equality operator (==) instead of the assignment operator (=).
To fix the error, change the equality operator (==) to the assignment operator (=) in the 'for' loop declaration:
int main() {
int i;
int sum = 0;
for (i = 0; i < 10; i++) {
sum += i;
}
printf("The sum of the numbers from 0 to 9 is %d\n", sum);
return 0;
}
By examining these code samples and employing the debugging tips discussed earlier, you can quickly and effectively identify and fix syntax errors in your C programs, ensuring a smoother development process.
Learning to Prevent Syntax Errors
While learning to fix syntax errors is essential, avoiding them from the beginning can save time and frustration during the development process. This section will discuss the importance of proper syntax in programming and strategies for writing error-free code.
Importance of Proper Syntax in Programming
Proper syntax is crucial in programming for various reasons. A solid understanding of a programming language's syntax will help you avoid errors, resulting in cleaner, more efficient code. Some of the key benefits of using proper syntax in programming include:
- Code readability: Correct syntax makes your code more accessible and easier to understand, not just for you but also for others who might need to read or maintain it in the future.
- Code efficiency: Properly structured code is generally more efficient in terms of execution time and memory usage, leading to better overall performance of your application.
- Easier debugging: When you follow the syntax rules of a language, you minimize the likelihood of errors, making it easier to debug your code if and when issues do arise.
- Better collaboration: Adhering to the correct syntax practices is crucial when working in a team, as it ensures all members can read and understand each other's code easily.
The importance of proper syntax should not be underestimated, as it plays an essential role in the success and maintainability of your programs.
Strategies for Writing Error-Free Code
To avoid syntax errors and write more efficient, maintainable code, it is essential to adopt strategies that promote error-free programming. The following sections provide tips and techniques for preventing syntax errors and achieving clean, effective programs.
Syntax Error Prevention Techniques
There are several techniques and best practices that can help you prevent syntax errors and improve your overall programming skills:
- Learn the language syntax: Thorough understanding of a programming language's syntax rules is instrumental in preventing errors. Invest time in learning and practicing the syntax rules of the language you are working with.
- Use a code editor with syntax highlighting: Utilising a code editor that offers syntax highlighting not only improves the readability of your code but also makes it easier to spot potential syntax errors as you write.
- Follow a consistent coding style: Adhering to a consistent coding style, such as indentation, naming conventions, and comments, facilitates better readability and reduces the likelihood of syntax errors.
- Divide complex tasks into smaller sub-tasks: Breaking down larger tasks into simpler components allows you to focus on smaller pieces of code, making it easier to spot and fix syntax errors.
- Test your code frequently: Regular testing during the development process helps you identify and correct syntax errors early on, saving time and resources later.
- Peer review and pair programming: Collaborating with peers to review each other's code or working together on a task (pair programming) promotes knowledge-sharing and helps catch syntax errors that might go unnoticed individually.
By adopting these prevention techniques and best practices, you can significantly reduce the occurrence of syntax errors, resulting in more efficient, maintainable, and error-free code. Continually refining these skills will not only enhance your programming abilities but also contribute to the overall success of your projects.
Syntax Errors - Key takeaways
Definition of syntax errors: mistakes in source code that prevent the program from compiling and executing properly
Common syntax errors: missing/extra parentheses or braces, mismatched quotes, missing/misplaced semicolons, incorrectly nested loops/blocks, invalid variable/function names, incorrect use of operators
Syntax error prevention techniques: learning language syntax, using a code editor with syntax highlighting, following a consistent coding style, dividing complex tasks, testing code frequently, peer review and pair programming
Debugging tips for syntax errors: examining compiler/interpreter error messages, using descriptive variable/function names, indenting/commenting code, breaking complex expressions into smaller parts, using a debugger tool, and testing code frequently
Benefits of proper syntax: code readability, code efficiency, easier debugging, and better collaboration
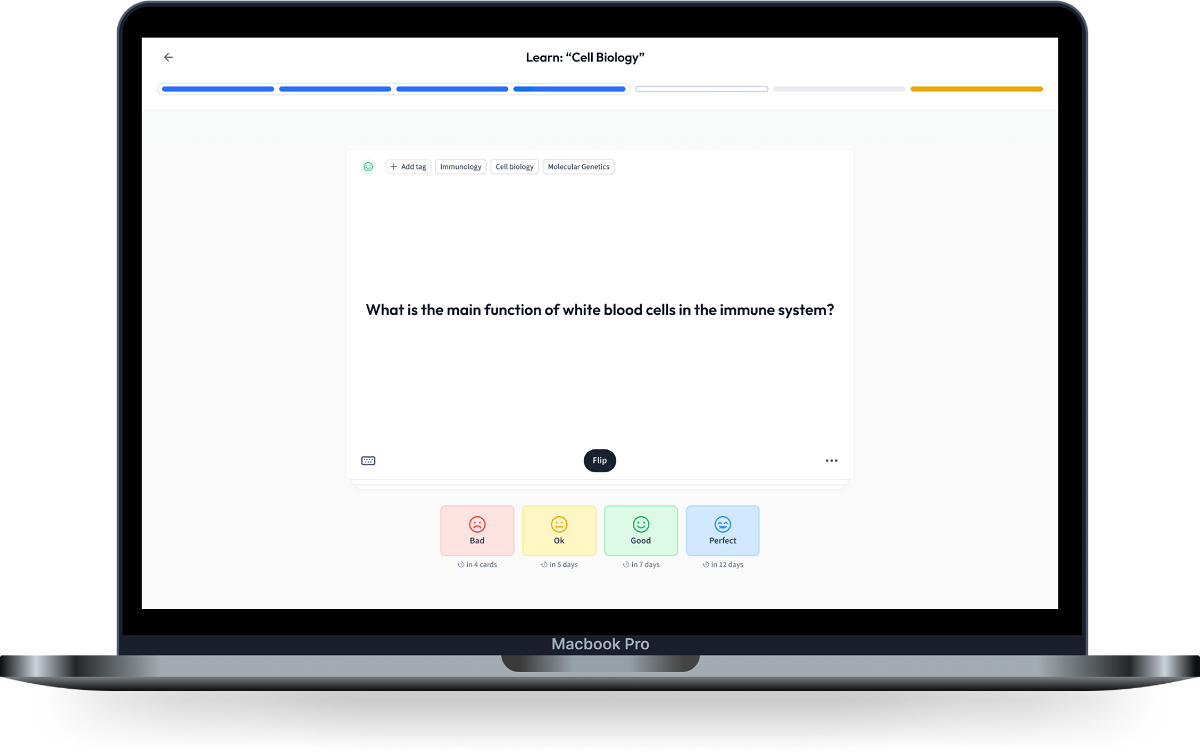
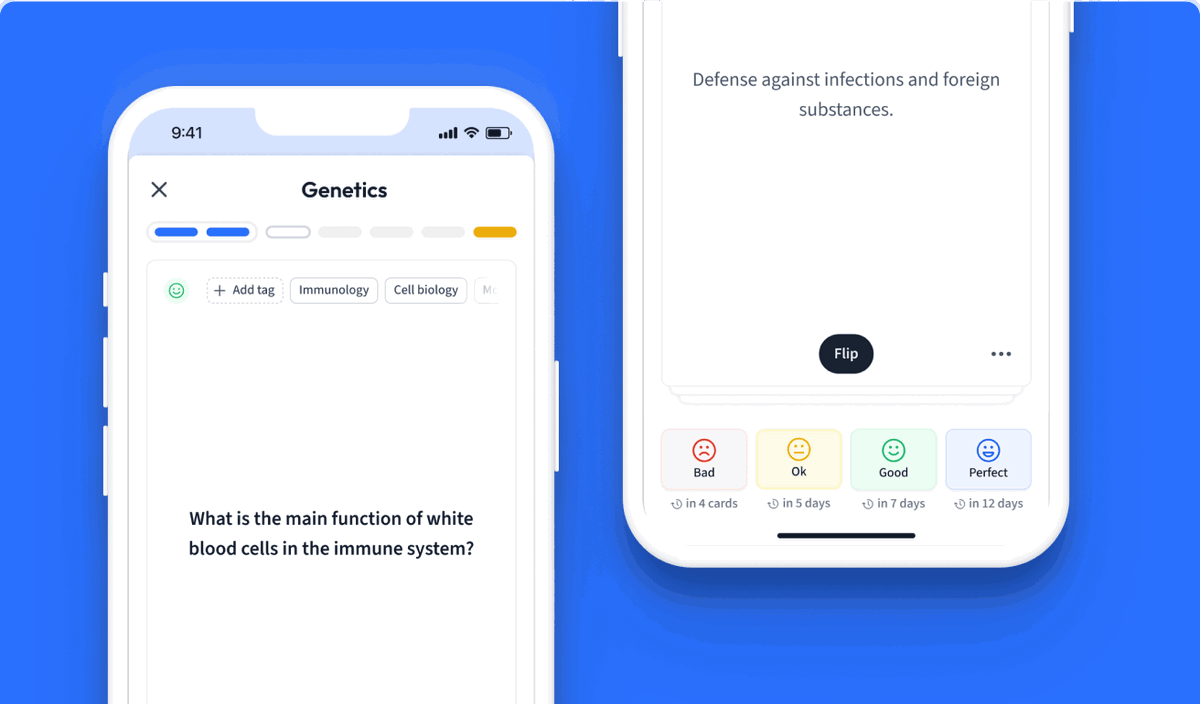
Learn with 15 Syntax Errors flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Syntax Errors
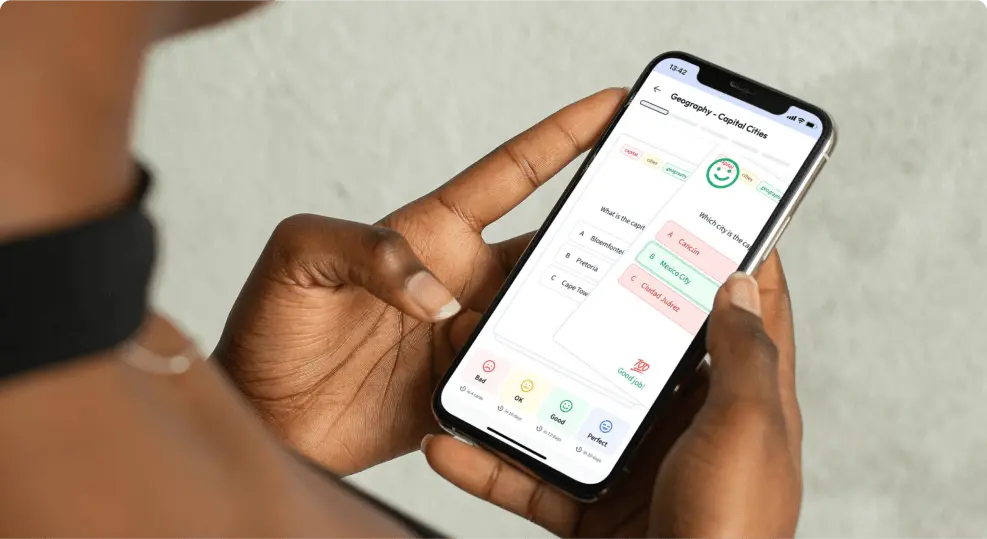
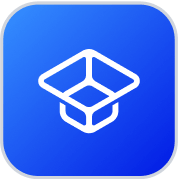
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more