Jump to a key chapter
What is a Variable in Programming
Variables are a fundamental concept in programming and form the building blocks for creating any software application. They act as containers that store data or information that can be manipulated throughout a program. Understanding how variables work will help you manage data efficiently as you write code.
Definition of a Variable
In programming, a variable is a symbolic name associated with a value and whose associated value may be changed. It is like a label for data you want to use in your program. Each variable can hold different data types such as integers, floats, strings, and more.
Declaring a Variable
Declaring a variable involves specifying its name and sometimes its data type. The syntax can vary slightly between different programming languages. Here is what declaring variables might look like in different programming languages:Python:
x = 10Java:
int x = 10;JavaScript:
let x = 10;In these examples, a variable named 'x' is declared and initialized with the value '10'.
Using Variables in Programming
Once a variable is declared, you can use it in expressions, perform arithmetic operations, or update its value. Here are some examples of different operations using variables:
- Arithmetic Operation: Adding two variables in Python:
a = 5b = 3sum = a + b
The variable sum will store the result of adding 'a' and 'b'. - Updating a Variable: You can change the value of a variable at any point in the program.
a = 5a = a + 1
After execution, 'a' will hold the value '6'.
Variable Naming Conventions
Variables have names that you define while programming. It's essential to follow certain conventions while naming variables to ensure your code is readable. Here are some general guidelines:
- A variable name must start with a letter or underscore (_).
- Variable names are case-sensitive (e.g., myVar, Myvar, and MYVAR are different).
- Avoid using reserved keywords (like int, float) as variable names.
- Use descriptive names to indicate the variable's purpose (e.g., totalCost, userName).
Consider a JavaScript function that calculates the area of a rectangle:
function calculateArea(width, height) { let area = width * height; return area;}let areaOfRectangle = calculateArea(5, 10);In this function, width and height are parameters that represent the dimensions of the rectangle. The function calculates the area using these variables.
Always initialize your variables to avoid unexpected results in your program.
Definition of Variables in Computer Science
In computer science, understanding variables is crucial as they form the cornerstone of seamless and effective programming. By encapsulating values that may vary throughout a program's lifecycle, variables help in managing and processing dynamic data efficiently.
A variable in computer science refers to a symbolic name that is associated with a value. The primary purpose of a variable is to label and store data values that can be manipulated or retrieved within a program.
Declaring and Initializing Variables
To make use of a variable in your program, you must first declare and often initialize it. Declaration specifies the variable's name and type (though this is optional in some languages), while initialization assigns it a starting value.The syntax for declaring and initializing variables may differ across programming languages. Below are examples in several popular languages:
Language | Syntax |
Python | x = 20 |
Java | int x = 20; |
JavaScript | let x = 20; |
Working with Variables
Variables can be manipulated and utilized in various operations. You can perform calculations, update their values, or use them to influence program logic. Consider the following use cases:
- Mathematical Computation: Compute the sum of two numbers:
num1 = 15num2 = 25total = num1 + num2
Here, total would store the value '40'. - Conditional Logic: Use variables in an if-statement:
let temperature = 35;if (temperature > 30) { console.log('It's hot outside!');}
This statement evaluates the condition using the temperature variable.
Importance of Naming Variables
Choosing appropriate variable names is a key aspect of writing clean code. Variable names serve as cues to the reader about their purpose and use. Consider these best practices for naming:
- Begin with a letter or underscore.
- Be descriptive but not overly verbose.
- Avoid using special characters or digits at the start.
- Keep them consistent and follow naming conventions such as camelCase or snake_case.
Here's a Python function that calculates the price after tax. It demonstrates good variable naming and usage:
def calculate_price_with_tax(price, tax_rate): tax = price * (tax_rate / 100) final_price = price + tax return final_pricetotal_price = calculate_price_with_tax(100, 5)In this example, price, tax_rate, and final_price clearly represent their respective values.
In-depth understanding of variables involves recognizing scope, lifetime, and visibility. Variables can have different lifetimes and visibility based on where they are declared within the code. For instance, in many programming languages, a local variable declared within a function exists only during the function execution and is not accessible outside. Conversely, global variables are accessible throughout the program. Understanding these concepts is crucial for maintaining memory efficiency and avoiding errors in complex programs.
Use comments in your code to annotate complex logic involving variables, enhancing clarity for other readers and your future self.
What are Programming Variables
Programming variables act as containers for storing data values. They are fundamental to any software development project as they enable you to manipulate data dynamically. By learning about variables, you can understand how data is stored and retrieved during a program's execution.
Essential Features of Variables
Variables have certain characteristics that define how they behave in a programming language. These features include:
- Data Type: Determines the kind of data a variable can hold, such as integers, strings, or booleans.
- Scope: Refers to the visibility of the variable within different parts of a program.
- Lifetime: Indicates how long the variable persists during the execution of a program.
- Mutability: Suggests whether the value of a variable can be changed after its initial declaration.
Types of Variables
Variables can be categorized based on their scope and usage within a program:
Type | Description |
Local Variable | Accessible within a specific block or function; its scope is limited to that block. |
Global Variable | Declared outside of functions and accessible from any part of the program. |
Instance Variable | A non-static variable that holds data related to an instance of a class in object-oriented programming. |
Static Variable | Belongs to the class rather than any single object; shared by all instances of the class. |
Declaring and Initializing Variables
The process of creating a variable involves declaring its name and assigning it an initial value. While syntax may differ among programming languages, the concept remains the same. Below are examples in different languages:Python:
x = 10Java:
int x = 10;C:
int x = 10;The syntax clearly identifies the variable's name ('x') and assigns it a value ('10').
Here is a simple JavaScript snippet that illustrates variable declaration and usage:
let length = 10;let breadth = 5;let area = length * breadth;console.log(area); // Outputs 50The variables length and breadth store measurements which are then used to calculate the area.
Understanding constant variables is also essential. A constant is a type of variable whose value cannot change once it's assigned. Constants are particularly useful when you want to define values that should not be altered during program execution, ensuring consistency and protecting crucial data. In JavaScript, you can declare a constant like this:
const PI = 3.14159;This guarantees that the value of PI remains constant throughout the program.
Remember to use meaningful variable names to make your code easier to read and maintain. This practice will benefit not only you but also others who may read your code in the future.
Examples of Programming Variables
In order to effectively implement solutions in programming, it's essential to understand the function of variables as they are the foundation of data storage and manipulation. Here, we will explore how variables are used in various programming scenarios.
Variable Program Concept Explained
The concept of variables in programming is akin to using placeholders or labels for data that can be manipulated throughout a program. Think of a variable as a container or a storage unit, where you can keep information that might change or be used elsewhere in your code.In a typical program, you will declare, initialize, and manipulate variables based on the requirements of your code logic. Below are several key operations you can perform with variables:
- Declaration
- Initialization
- Modification
- Utilization in Expressions
Consider a simple Python program to compute the sum of two numbers:
# Declare and initialize variablesa = 10b = 20# Calculate sumsum = a + bprint('The sum is:', sum)In this example, a and b are variables that store numeric data. The variable sum holds the result of their addition, showcasing the use of variables in expression evaluation.
To further deepen your grasp of the variable concept, it's beneficial to delve into scope and lifetime of variables. Scope determines where the variable can be accessed in the code. For instance, a variable declared inside a function is typically scoped to that function alone, making it invisible outside.
# Example in JavaScriptfunction sampleFunction() { let scopedVar = 'Hello'; // local to this function}console.log(scopedVar); // Error: scopedVar is not definedUnderstanding these concepts not only aids in organizing your code but also prevents errors such as inadvertently modifying data from an unintended scope or causing memory leaks with long-lived variables.
Utilize comments in your code to explain the purpose of each variable, enhancing clarity and maintainability.
Variable Program - Key takeaways
- Variable Program: A core concept in programming that refers to a mechanism for storing data through symbolic names, usable throughout a program.
- What is a Variable in Programming: A symbolic name for a value that can change, acting as a storage label for data manipulation in computer programs.
- Definition of Variables in Computer Science: Variables are symbolic names associated with values, used to label and manage data that can be manipulated or accessed in a program.
- Declaring Variables: The process of specifying a variable's name and sometimes its type; varies across programming languages, like Python, Java, and JavaScript.
- Examples of Programming Variables: Arithmetic operations, updating values, and use in conditional logic show diverse applications of variables in code.
- Variable Program Concept Explained: Variables serve as containers or labels for data, aiding in code flexibility and interactions.
Learn faster with the 55 flashcards about Variable Program
Sign up for free to gain access to all our flashcards.
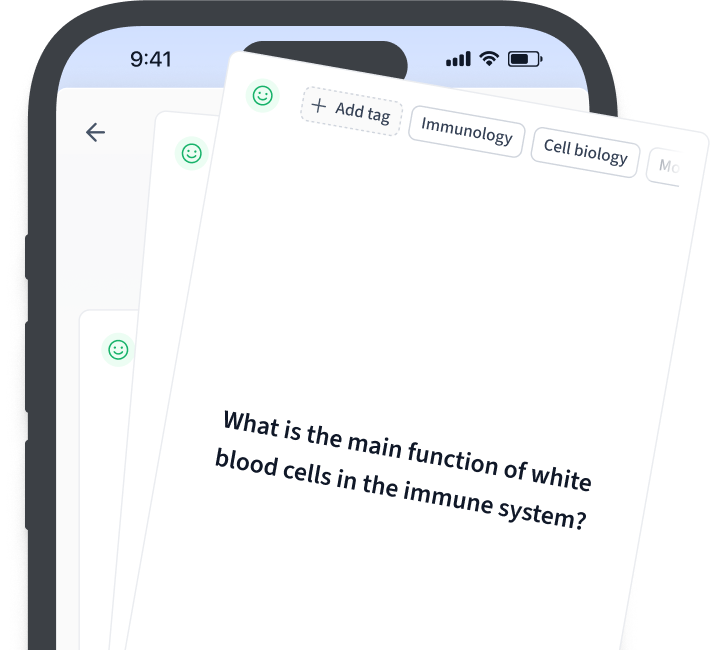
Frequently Asked Questions about Variable Program
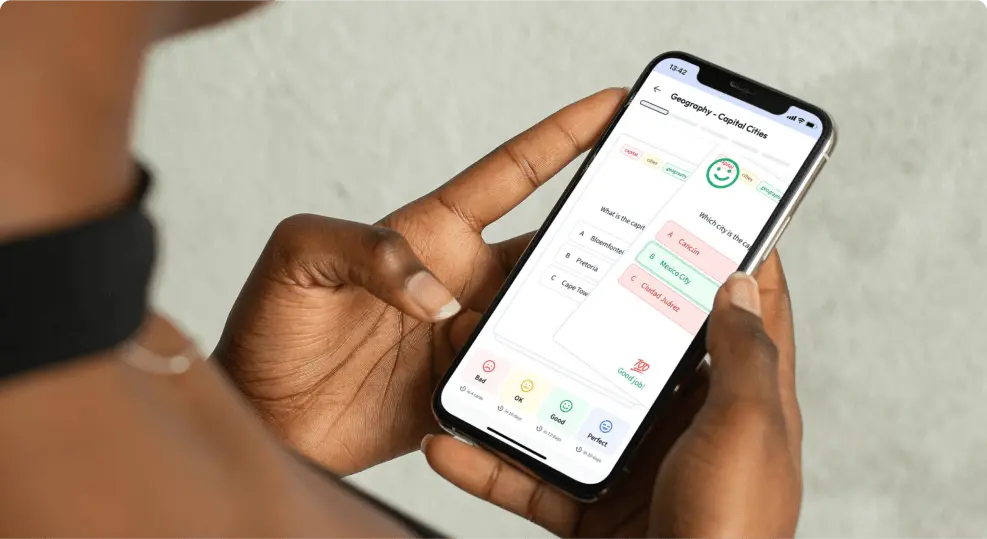
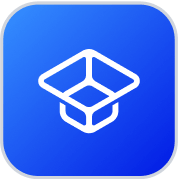
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more