Jump to a key chapter
What is Cybersecurity in Computer Science
Cybersecurity is an essential area of computer science focused on safeguarding computers, networks, and data from malicious attacks, unauthorized access, and damage. With the growing reliance on technology, cybersecurity plays a crucial role in ensuring the safety of digital assets.
Definition and Importance
Cybersecurity refers to the practice of protecting systems, networks, and programs from digital attacks. These cyberattacks are usually aimed at accessing, changing, or destroying sensitive information, extorting money, or interrupting normal business processes.
The importance of cybersecurity cannot be understated in today's digital world. With increasing amounts of data being transferred over networks, there is a significant threat of these data being compromised. Effective cybersecurity measures can prevent such breaches and protect users' personal and financial information.
The global cybersecurity market is expected to reach a value of over $280 billion by 2025, highlighting its growing importance and demand.
Key Concepts in Cybersecurity
To understand cybersecurity, you should be familiar with several key concepts, including:
- Authentication: The process of verifying the identity of a user or system.
- Encryption: Transforming data into a secure format to prevent unauthorized access.
- Firewall: A network security device that monitors and controls incoming and outgoing network traffic based on predetermined security rules.
- Intrusion Detection System (IDS): A tool that monitors network traffic for suspicious activities and issues alerts when such activities are discovered.
- Malware: Malicious software designed to harm, exploit, or otherwise compromise computer systems.
- Penetration Testing: An authorized simulated cyberattack on a computer system to evaluate its security.
A real-world example of cybersecurity in action is the use of two-factor authentication (2FA). This method requires not only a password and username but also something that only the user has on them, i.e., a piece of information only they could know or have immediately to hand, such as a physical token.
Dive deeper into encryption, a cornerstone of modern cybersecurity. Encryption can be symmetric or asymmetric.
- Symmetric Encryption: Uses the same key for both encrypting and decrypting data. It's fast but requires secure key management.
- Asymmetric Encryption: Uses public and private keys for encryption and decryption, making it more secure but slower.
Cybersecurity vs. Information Security
While often used interchangeably, cybersecurity and information security have distinct differences. Cybersecurity specifically focuses on defending digital data and systems from cyber threats. Information security (InfoSec), meanwhile, is a broader term that encompasses all aspects of protecting data, whether it's in digital or physical form.
The scope of information security includes various measures, such as:
- Physical security to protect hardware and networks from physical threats
- Administrative controls such as security policies
- Logical controls like access management systems
Understanding the distinction between security terms is critical for professionals when designing security solutions tailored to specific needs.
Cybersecurity Coding Examples
When exploring cybersecurity in computer science, understanding coding examples is essential. These examples illustrate how various tools protect against cyber threats effectively.
Common Cybersecurity Algorithms
Algorithms form the backbone of cybersecurity strategies, helping to detect intrusions, encrypt data, and manage security protocols. Some common algorithms include:
- AES (Advanced Encryption Standard): A symmetric encryption technique widely used today due to its reliability and speed.
- RSA: A public-key cryptosystem used for secure data transmission and particularly important for safe exchanges over the internet.
- SHA-256: A cryptographic hash function used for verifying data integrity.
Consider a simple Python example of RSA encryption:
from Crypto.PublicKey import RSA key = RSA.generate(2048) private_key = key.export_key() public_key = key.publickey().export_key() print(private_key.decode('utf-8')) print(public_key.decode('utf-8'))This code generates a pair of RSA keys (public and private) used for encrypting and decrypting data securely.
Delve deeper into the AES algorithm, which operates on fixed block sizes of 128 bits with key sizes of 128, 192, or 256 bits. It's based on a symmetric key algorithm, meaning the same key is used for both encryption and decryption. The algorithm's robustness comes from multiple rounds of processing, each involving substitution, permutation, and mixing.
Programming Languages Used in Cybersecurity
Different programming languages are suited to various aspects of cybersecurity due to their unique characteristics. Some of the most commonly used languages include:
- Python: Known for its readability and simplicity, Python is widely used for building scripts and automation tools in cybersecurity.
- C/C++: These languages offer low-level access to memory and system resources, making them suitable for developing security software and system-level programming.
- Java: Due to its scalability, Java is often used in large enterprise environments and web applications requiring secure data transactions.
Python's extensive libraries, such as Scapy and Nmap, are popular choices for developing security applications and tools.
Best Practices in Cybersecurity Coding
Effective cybersecurity coding involves adhering to best practices that enhance the security and resilience of applications. Consider the following guidelines:
- Regular Code Reviews: Routine examination of code by multiple developers to spot vulnerabilities.
- Use of Strong Authentication and Authorization: Implement robust methods for confirming identities and controlling access to resources.
- Input Validation: Ensure that all user inputs are checked and validated to prevent injection attacks.
- Secure Data Storage: Encrypt sensitive data both at rest and during transmission.
- Logging and Monitoring: Maintain logs and monitor systems continuously to detect unusual activities.
Here's a sample snippet highlighting safe user input handling in Python:
import re def is_valid_email(email): return re.match(r'^[\w\.-]+@[\w\.-]+\.[a-zA-Z]{2,6}$', email) is not None email_input = input('Enter your email: ') if is_valid_email(email_input): print('Email is valid.') else: print('Invalid email.')This code checks if a user's email input fits a valid pattern before accepting it, protecting against injecting potentially harmful data.
Cybersecurity Safeguarding Techniques
Cybersecurity safeguarding techniques are vital for protecting networks, data, and user identities from various cyber threats. These techniques encompass a range of measures designed to prevent unauthorized access and ensure the integrity, confidentiality, and availability of digital information.
Network Security Measures
Network security measures are essential components of a comprehensive cybersecurity strategy. They focus on protecting the data during transmission and include various tools and processes to secure the network infrastructure. Some key measures include:
- Firewalls: Firewalls act as barriers between your internal network and external networks, filtering incoming and outgoing traffic based on predetermined security rules.
- Intrusion Detection Systems (IDS): An IDS monitors network traffic for suspicious activities and generates alerts when potential threats are detected.
- Virtual Private Networks (VPNs): VPNs create a secure, encrypted connection over a less secure network, such as the internet, ensuring secure data transmission.
Consider configuring a simple firewall using iptables in a Linux system by running the following command:
iptables -A INPUT -p tcp --dport 22 -j ACCEPT # Allow SSH iptables -A INPUT -j DROP # Drop all other trafficThis configuration allows SSH traffic while blocking all other connections, maintaining a secure network environment.
Take a closer look at VPNs, which use tunneling protocols and encryption techniques to transport data securely between a client and a server. A popular protocol is OpenVPN, which supports a variety of encryption standards like AES, offering robust data protection.
Data Encryption Methods
Data encryption is a critical technique used to protect sensitive information by converting it into a secure format. This method ensures that only authorized parties can read the data. Some commonly used encryption methods include:
- Symmetric Encryption: Utilizes one key for both encryption and decryption. It's fast and suitable for encrypting large volumes of data but requires secure key distribution.
- Asymmetric Encryption: Involves a pair of keys—one public and one private—for encryption and decryption. Although slower, it enhances security and is often used in digital signatures.
- Hashing: Turns data into a fixed-length string of characters, which appears random. Used primarily for verifying data integrity.
A typical use case of encryption in Python by encrypting a message with the AES encryption standard:
from Crypto.Cipher import AES from Crypto.Random import get_random_bytes key = get_random_bytes(16) cipher = AES.new(key, AES.MODE_EAX) ciphertext, tag = cipher.encrypt_and_digest(b'Secret Message')This snippet encrypts a message, ensuring it remains unreadable without the correct key.
Always use encryption algorithms that are industry-approved to ensure data security, as they are rigorously tested and regularly updated to address new vulnerabilities.
Access Control and Authentication
Access control and authentication are critical components of cybersecurity, designed to restrict who can enter a system and what resources they can use. Effective implementation includes:
- Password Protection: Ensures that only authorized users can access the systems with strong password policies and regular updates.
- Two-Factor Authentication (2FA): Combines something you know (like a password) with something you have (like a phone) to provide additional security.
- Role-Based Access Control (RBAC): Assigns user access based on their role within the organization, limiting access to necessary resources only.
Implementing 2FA can be seen in platforms requiring an OTP (One-Time Password) sent to your mobile after entering your password. This second layer of verification bolsters account security.
Use authentication methods such as OAuth for third-party access, as it allows limited access without exposing user passwords.
Computer Information Science and its Role in Cybersecurity
Computer information science is key to understanding and advancing cybersecurity measures. It provides the theoretical foundation and practical skills needed to design, implement, and manage secure systems. By studying patterns in data and system behavior, professionals can anticipate threats and create robust defense mechanisms.
Analyzing Data for Threat Detection
In cybersecurity, threat detection relies heavily on analyzing data to identify and respond to potential risks. With vast amounts of data flowing through networks daily, identifying anomalies that may indicate threats is crucial. Several methods are employed to enhance threat detection:
- Pattern Recognition: Identifies regularities and irregularities within data.
- Machine Learning: Algorithms learn from patterns and improve their detection capabilities over time.
- Data Mining: Uses statistical techniques to discover patterns in large datasets.
Here's a simplified Python example using machine learning to detect anomalies:
from sklearn.ensemble import IsolationForest import numpy as np X = np.array([[10], [15], [14], [10], [13], [110]]) clf = IsolationForest(random_state=0).fit(X) print(clf.predict([[12], [110]]))This code trains a model to identify outliers, useful in detecting abnormal network behavior.
Utilize tools like Wireshark to capture and analyze network traffic for better understanding of anomalies and threats.
Dive deeper into machine learning for threat detection, where techniques such as supervised and unsupervised learning can be applied.
- Supervised learning: Involves training algorithms on a labeled dataset, where the outcome is known, making it effective for classifying known threats.
- Unsupervised learning: Detects unknown threats by identifying patterns without pre-labeled data. Clustering algorithms are commonly used here.
Designing Secure Systems
Designing secure systems is a fundamental aspect of cybersecurity to ensure that they are resistant to attacks. Key principles include:
- Least Privilege: Granting the minimum level of access necessary to perform a function.
- Defense in Depth: Employing multiple layered security measures to protect systems.
- Security by Design: Building security into the architecture from the beginning rather than adding it later.
Regularly update systems and software to patch known vulnerabilities and reduce exploit risk.
When designing a web application, use the defense in depth approach:
- Implement input validation and sanitization techniques to mitigate injection attacks.
- Use HTTPS to secure communications.
- Enable secure password storage practices, such as salting and hashing.
Integrating Cybersecurity in Software Development
Cybersecurity integration into software development is essential to produce secure and resilient applications. This process involves embedding security throughout the software development lifecycle (SDLC). Important practices include:
- Secure Coding: Following best practices to write code that resists vulnerabilities.
- Security Testing: Including static and dynamic analysis tools to identify and fix vulnerabilities.
- Continuous Monitoring: Implementing systems to constantly check for new vulnerabilities post-deployment.
Use static analysis tools like SonarQube during development to detect potential issues in the code before it reaches production.
Explore the concept of DevSecOps, which integrates security practices into DevOps processes, ensuring continuous delivery with security as a central concern. It emphasizes collaboration across development, operations, and security teams to boost efficiency and security.
Current Cybersecurity Issues in Computer Science
Cybersecurity continues to pose significant challenges due to rapid technological advancements and evolving cyber threats. Understanding current issues is crucial to developing robust defenses and securing digital information.
Emerging Threats and Vulnerabilities
The landscape of cyber threats is constantly changing, with new vulnerabilities emerging regularly. These threats target various aspects of technology, from personal devices to large corporate networks. Key emerging threats include:
- Ransomware Attacks: Malicious software that encrypts your data, demanding payment to restore access.
- Phishing Scams: Fraudulent attempts to obtain sensitive information through deceptive emails or websites.
- Internet of Things (IoT) Vulnerabilities: Security gaps in interconnected devices, leaving networks exposed.
A recent example of ransomware is the attack on Colonial Pipeline in 2021, which disrupted fuel supplies across the Eastern USA by encrypting critical data and systems.
Regularly updating software and systems helps close vulnerabilities that could be exploited by ransomware and other attacks.
Delve into the IoT vulnerabilities: As more devices connect to the internet, the potential attack surface increases. Many IoT devices have limited security capabilities, making them prime targets for attackers. Thus, securing the IoT ecosystem involves rigorous testing and innovative security solutions such as blockchain technology to enhance trust and authentication.
Challenges in Protecting Personal Data
The protection of personal data is a critical concern, as data breaches can result in severe consequences for both individuals and organizations. Several challenges complicate this task:
- Data Proliferation: The rapid increase in data creation and sharing across various platforms.
- Inadequate Privacy Controls: Many systems lack robust measures to protect user privacy effectively.
- Complex Regulations: Navigating different data protection laws across regions is challenging for international companies.
The Facebook-Cambridge Analytica scandal highlighted how inadequate privacy controls could lead to unauthorized data harvesting and misuse.
End-to-end encryption is an effective layer of protection for ensuring data integrity during transmission.
Evolution of Cybersecurity Technologies
As cyber threats evolve, so do cybersecurity technologies. Innovations are continually developed to better protect against new and existing threats. Key advancements include:
- AI and Machine Learning: These technologies help in predicting and identifying threats faster than traditional methods.
- Zero Trust Security Model: A security framework that assumes breaches will occur and verifies every request as though it originates from an open network.
- Blockchain for Cybersecurity: Leveraging decentralized ledgers to enhance data security and integrity.
Explore the Zero Trust Security Model: This model shifts the security focus from perimeter-based defenses to protecting the actual identity and data. It includes micro-segmentation, encrypting data, and strictly enforcing access controls, thereby reducing risks even when the network perimeter is breached.
Cybersecurity in Computer Science - Key takeaways
- Cybersecurity in Computer Science: Focuses on protecting computers, networks, and data from unauthorized access or attacks, ensuring digital asset safety.
- Key Concepts: Include authentication, encryption, firewalls, intrusion detection systems (IDS), malware, and penetration testing.
- Cybersecurity Algorithms: Examples include AES for encryption, RSA for secure data transmission, and SHA-256 for data integrity verification.
- Cybersecurity Safeguarding Techniques: Involves network security measures like firewalls and VPNs, data encryption methods, and access control and authentication strategies like two-factor authentication.
- Cybersecurity vs. Information Security: Cybersecurity focuses on digital data and cyber threats, whereas information security covers both digital and physical protection of data.
- Current Cybersecurity Issues: Include emerging threats like ransomware and IoT vulnerabilities, challenges in protecting personal data, and advancements in technologies such as AI and zero trust security models.
Learn faster with the 2740 flashcards about Cybersecurity in Computer Science
Sign up for free to gain access to all our flashcards.
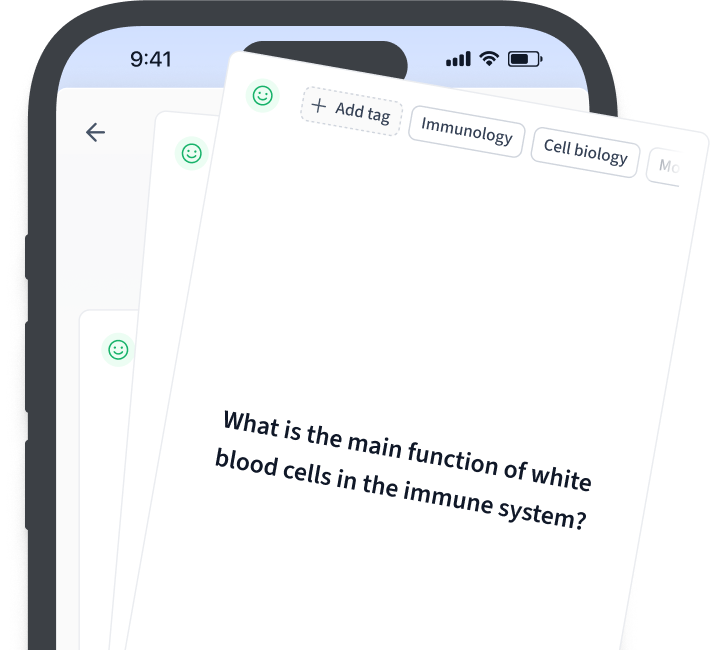
Frequently Asked Questions about Cybersecurity in Computer Science
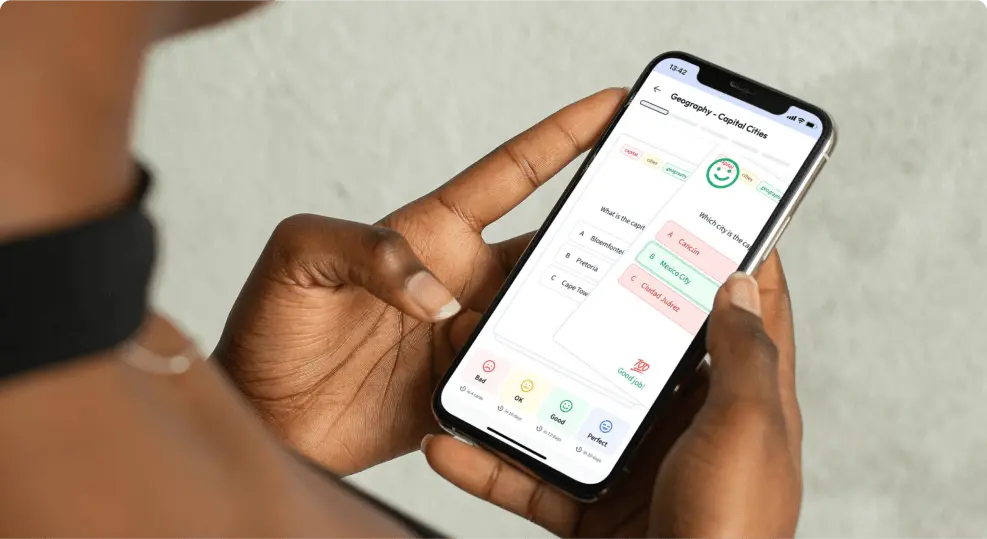
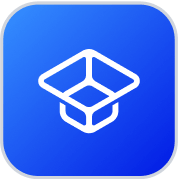
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more