Jump to a key chapter
Understanding Data Validation
Data validation is an essential concept in computer science, ensuring that data input is both correct and useful. With its application stretching across various domains, understanding data validation helps in maintaining the reliability and accuracy of your data-driven projects.
What is Data Validation?
Data validation refers to the process of verifying that a program operates on clean, correct, and useful data. This process often involves the use of validations rules, or check routines, that ensure data quality. By implementing data validation, you prevent invalid data from entering the system and causing errors or misinterpretations.
Data validation is the practice of checking, verifying, and ensuring the accuracy and quality of data before it is processed or stored.
An example of data validation is using a form in a web application to collect email addresses. By applying validation, you can ensure that inputs like 'user@domain' are correctly formatted before accepting them into the database.
Data validation can be applied in both manual and automated processes to maintain data integrity.
Importance of Data Validation in Computer Science
Data validation plays a critical role in computer science for several reasons:
- Data Integrity: Ensures that data remains accurate and consistent across the system.
- Security: Protects the system from malicious inputs and vulnerabilities.
- User Experience: Enhances the user interface by preventing input errors and guiding users in providing acceptable input.
- Reliability: Increases the dependability of the system by preventing faulty data processing.
The process of data validation is not only crucial for preventing immediate data entry errors but also plays a significant role in data engineering and analytics. In data engineering, cleaning and validating the data helps in building robust data pipelines that ensure smooth data flows. Similarly, in data analytics, validated data enables more accurate insights and decision-making based on reliable datasets. By implementing systematic data validation, data scientists and engineers can avoid pitfalls associated with data mismatch, anomalies, and inconsistencies that can skew their analysis outcomes.
Data Validation Process Overview
A comprehensive data validation process often involves several key steps:
- Input Validation: This step ensures that incoming data meets predefined criteria before processing. For instance, checking an integer field doesn't contain text.
- Data Type Check: Ensures data is of the correct data type. For example, numeric fields are numbers.
- Range Check: Validates that data falls within a specific range. An example is age fields accepting values only between 0 and 120.
- Format Check: Ensures data conforms to the required format, such as dates in YYYY-MM-DD.
- Cross-field Validation: Compares values across different fields, such as end dates always being after start dates.
Consider a simple data input form processing library in Python that checks whether a user-provided number input is within an expected range before saving it to a database:
def validate_number_in_range(number, min_value, max_value): if min_value <= number <= max_value: return True else: return Falseinput_number = 25if validate_number_in_range(input_number, 0, 100): print('Number is valid.')else: print('Number is invalid.')
Data Validation Techniques in Computer Science
In computer science, data validation is a crucial practice to ensure that data entered into a system is correct and useful. Various techniques are employed to perform this validation effectively and efficiently.
Common Data Validation Methods
Several common methods are used to validate data in computer systems. These methods can be applied individually or in combination to enhance data accuracy and reliability.
The process of verifying that input data meets a set of predefined criteria to ensure its quality and accuracy is known as data validation.
Consider a user registration form that validates input.
- Email Validation: Ensures the input follows standard email format (e.g., user@example.com).
- Password Length Check: Verifies the password is within an accepted length range (e.g., 8-16 characters).
Data validation can also involve more complex checks like referential integrity, which ensures that all references within the data are valid. For instance, in a database, vehicle registration entries must align with existing owner records. If an ID cited in one table does not exist in the referenced table, it signals an inconsistency. Addressing such issues typically involves joining tables and cross-referencing records, an operation often seen in relational databases. While straightforward mechanisms may only check data format, sophisticated systems employ integrity constraints and rely on foreign keys in databases to ensure proper and legitimate relationships between dataset entities.
Automated vs. Manual Data Validation Techniques
Data validation can be performed through automated techniques or manually by data workers. Each approach has its advantages and applications.
Automated data validation involves the use of software tools and scripts to check data against validation rules without manual intervention.
Manual data validation refers to human inspection and verification of data accuracy, often involving decision-making or complex data interpretations.
Automated data validation is ideal for large datasets, while manual validation can be more effective for intricate or nuanced data interpretations.
Criteria | Automated Validation | Manual Validation |
Speed | Fast and efficient | Time-consuming |
Consistency | High consistency | Potential human error |
Flexibility | Limited to predefined rules | Highly adaptable |
Data Validation Challenges Faced by Developers
Developers often encounter several challenges while implementing data validation procedures. Understanding these challenges is key for building reliable systems.
A frequent challenge is dealing with incomplete data. For example, a system may require a customer's phone number and email, but sometimes only one is provided. To overcome this, developers might implement conditional rules to permit operation under incomplete conditions while flagging records for follow-up.
Implementing timely feedback for user input errors can enhance user experience and data quality.
Additional challenges include:
- Handling exceptions: Identifying rare cases that aren't covered by existing rules.
- Performance concerns: Extensive validation can burden system resources, affecting application speed.
- Ensuring security: Protecting against malicious input through validation.
- Scalability: Adapting validation rules as datasets grow and become more complex.
Data Validation Best Practices
Adopting data validation best practices is crucial for ensuring data quality and reliability in any system. Properly validated data prevents erroneous inputs and enhances system performance and security.
Implementing Effective Data Validation
Implementing effective data validation involves several key elements that ensure input data is consistent, accurate, and secure. Consider the following practices:
- Define Validation Rules: Establish clear criteria for what constitutes valid data. This could include format checks, range checks, and mandatory field checks.
- Create Comprehensive Error Messages: Provide specific and actionable error messages that guide users towards correct input.
- Use Regular Expressions: Regular expressions are powerful for pattern matching, particularly for string validations such as URLs and emails.
For example, using a regular expression to validate an email input:
import redef is_valid_email(email): pattern = r'^[\w\._%+-]+@[\w\.-]+\.[a-zA-Z]{2,}$' return re.match(pattern, email) is not NoneThis code checks the email format and confirms its validity.
In more complex data environments, validation extends beyond the superficial checks. For instance, consider validation in hierarchical data structures, such as XML or JSON. It's crucial to ensure not only that individual data entries are valid but that relationships between data nodes comply with predefined schemas. In XML, the use of DTD (Document Type Definition) or XSD (XML Schema Definition) aids this process, while JSON schemas, defined in JSON Schema form, impose rules on JSON data to maintain data integrity across different applications.
Ensuring Data Integrity and Security
Data integrity and security are paramount in all data validation processes. Here are some ways you can strengthen these aspects:
- Implement Input Sanitization: Strip unwanted characters from user input to prevent SQL injections and other attacks.
- Use Secure Connection Protocols: Ensure data transmission is secured through HTTPS or other encrypted protocols.
- Perform Regular Audits: Routinely check data for consistency, accuracy, and compliance with validation rules.
Method | Purpose |
Input Sanitization | Protects against code injection |
Secure Protocols | Secures data in transit |
Regular Audits | Maintains ongoing data quality |
Consider an example of using parameterized queries in SQL to prevent injection attacks:
import sqlite3def fetch_user_data(user_id): conn = sqlite3.connect('example.db') cur = conn.cursor() cur.execute('SELECT * FROM users WHERE id = ?', (user_id,)) return cur.fetchall()This approach ensures user input is appropriately escaped before execution.
Best Practices for Streamlined Data Validation
Streamlining data validation can greatly enhance system performance and user experience. Consider implementing these best practices:
- Modularize Validation Logic: Keep your validation code separate from business logic to enhance maintainability and scalability.
- Leverage Built-in Functions: Utilize available library functions and frameworks for efficient validation processes.
- Automate Repetitive Tasks: Use tools to automate frequent and repetitive validation tasks to reduce manual effort.
Addressing Data Validation Challenges
Data validation is critical to ensuring data is accurate, secure, and consistent. However, several challenges can arise during its implementation that affects performance and reliability. Understanding and addressing these challenges helps maintain robust data systems.
Overcoming Common Data Validation Issues
Addressing common data validation issues involves identifying sources of error and implementing strategies to mitigate them. These issues can include:
- Incorrect Data Types: Occur when data does not match the expected type, like a string in a numeric field.
- Data Format Inconsistencies: Happen when data does not adhere to the required format, such as a malformed date.
- Missing or Incomplete Data: Occur when fields expected to hold data are empty or lack necessary information.
Consider a scenario where user input is consistently lacking the expected email format in a registration form. A validation rule can be applied to check for '@' and '.' symbols and ensure domain length criteria. This reduces format-related validation errors.
def validate_email_format(email): if '@' in email and '.' in email.split('@')[1]: return 'Valid email' else: return 'Invalid email format'
Another challenge is maintaining data integrity across distributed databases. As data is replicated across locations, ensuring consistency despite latency and updates is crucial. Techniques such as eventual consistency or using consensus algorithms (e.g., Paxos or Raft) can be employed. These approaches balance performance with consistency, allowing updates to converge over time, ensuring data reliability without severely impacting responsiveness.
Consider using a validation library or framework that offers built-in support for common validation rules, reducing custom code and errors.
Strategies to Handle Data Validation Errors
Handling data validation errors efficiently prevents adverse impacts on data integrity and system performance. Implementing the following strategies can mitigate errors:
- Error Logging: Capture validation errors in logs to analyze patterns and identify recurring issues efficiently.
- User Feedback: Offer clear error messages and instructions to guide users in correcting their input mistakes.
- Graceful Degradation: Design the system to handle invalid data inputs without crashing by isolating faulty inputs for manual review.
A practical example is a web application form that flags invalid phone numbers immediately upon entry, providing guidance on the acceptable format, such as including country code. This approach prevents incomplete submissions and guides users towards successful data entry.
Automated testing can be integrated into the development process to ensure validation rules are effective against possible input variances.
Future Trends in Data Validation
The future of data validation is shaped by emerging technologies and methodologies that promise more intelligent and efficient processes.
- AI and Machine Learning: Integration of AI can enhance validation by intelligently predicting patterns and anomalies in data, allowing for more adaptive validation rules.
- Blockchain Technology: Offers decentralized validation, ensuring data's immutability and authenticity, particularly in transactions.
- Real-Time Validation: Advances in processing capabilities allow for instant validation, supporting applications that require rapid data verification.
data validation - Key takeaways
- Data validation: The process of verifying that data input is clean, correct, and useful before processing or storing.
- Importance in Computer Science: Ensures data integrity, security, reliability, and enhances user experience by preventing faulty data processing.
- Data Validation Process: Involves steps like input validation, data type checks, range checks, format checks, and cross-field validation to ensure data accuracy.
- Data Validation Techniques: Includes automated and manual techniques, leveraging technologies like regular expressions, referential integrity checks, and validation libraries/frameworks.
- Challenges: Developers face issues like incomplete data, handling exceptions, performance impacts, and ensuring security and scalability.
- Best Practices: Define clear validation rules, use error messages, regular audits, input sanitization, and leverage automated and manual validation for robust data integrity.
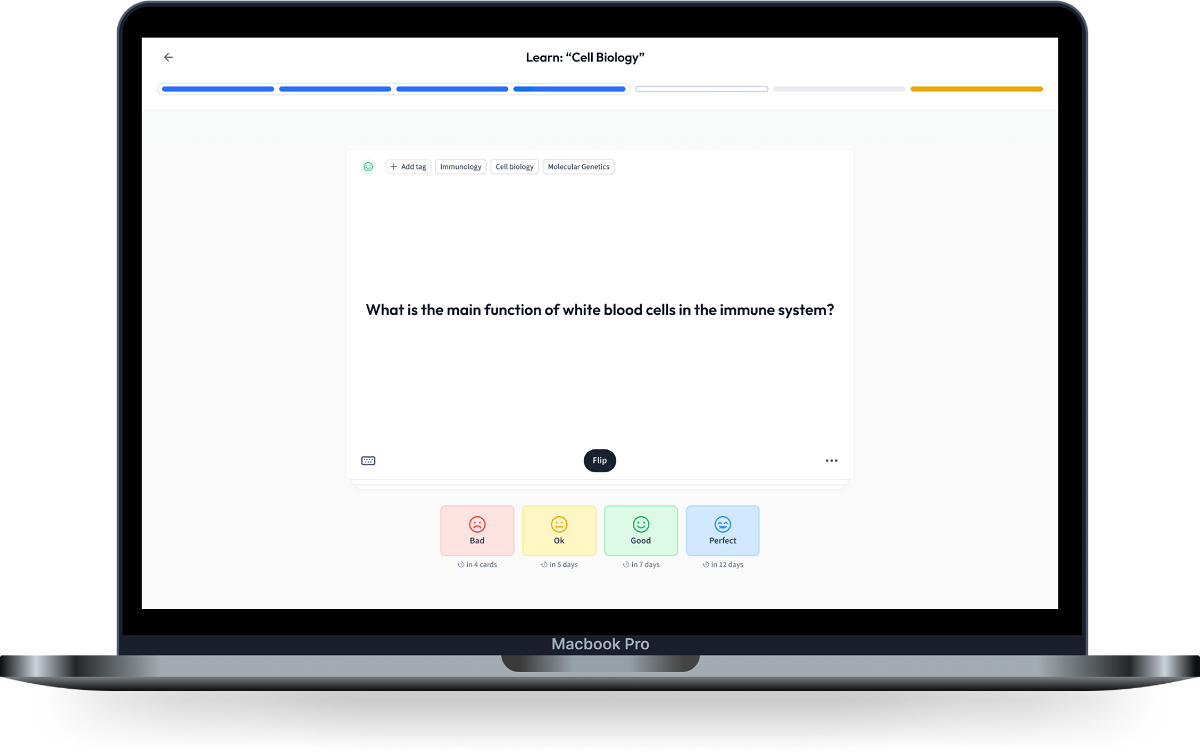
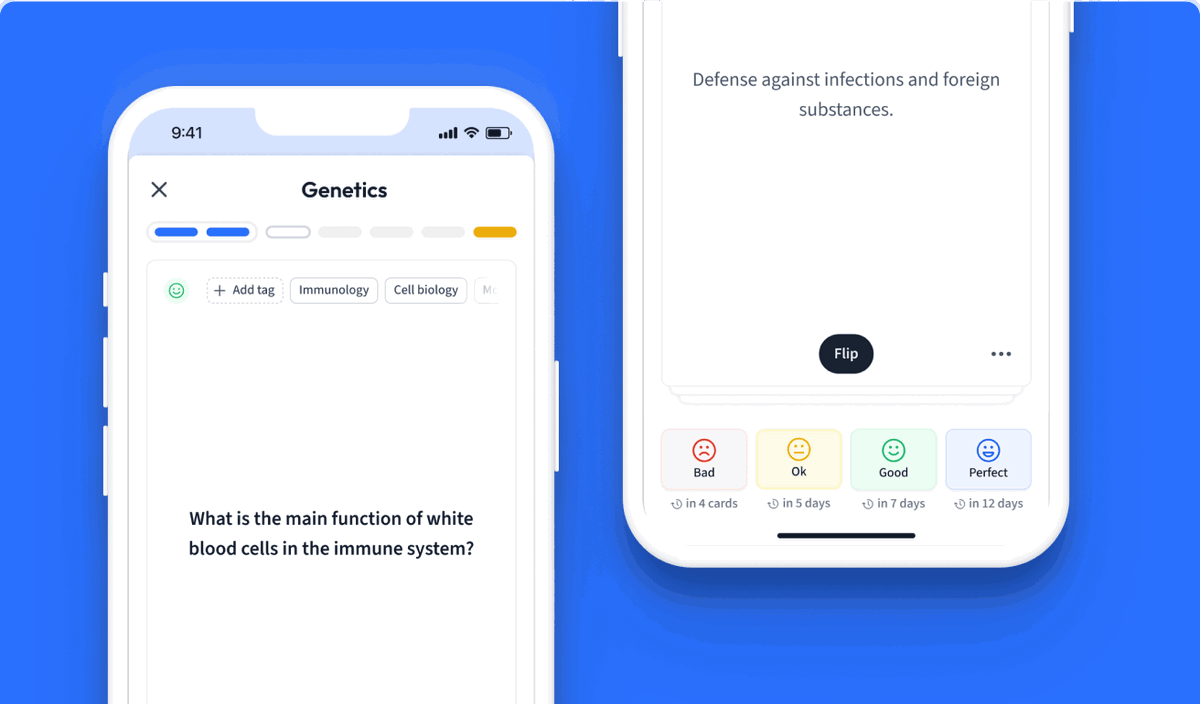
Learn with 12 data validation flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about data validation
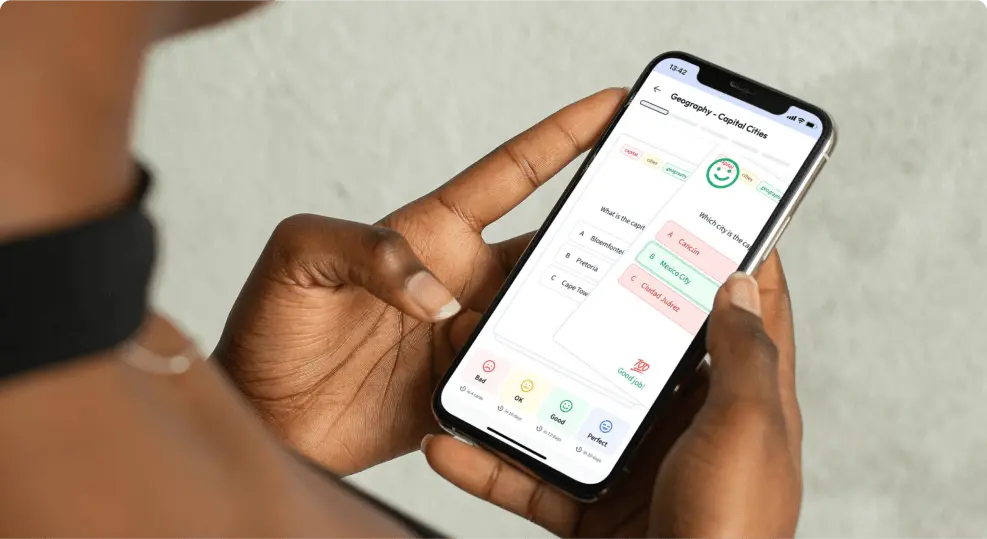
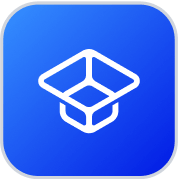
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more