Jump to a key chapter
What is a Hash?
In computer science, a hash is a function that converts input into a string of numbers and letters, known as the output or hash value. This concept is invaluable due to its efficiency in searching and data retrieval operations.
Definition and Concept
A hash function takes an input (or 'message') and returns a fixed-length string of characters, which is often a sequence of random-looking letters and numbers. The primary purpose of hashing is to process data quickly and efficiently, ensuring that data lookup, comparison, or indexing becomes more convenient.
Hash Function: A mathematical algorithm that maps data of arbitrary size to data of a fixed size.
Consider the hash function as a formula like:
function hash(x): return (x * 7) % 5Here, \
such a formula allows distribution of data across an available range in a consistent manner. Understanding the mechanics of hash functions allows you to optimize databases and improve system performance. When implementing hashing, key properties to keep in mind include:
- Deterministic: A given input must always produce the same hash value.
- Fast Computation: The hash value should be quick to compute, regardless of input size.
- Compression: Any input can be reduced to a fixed size hash, typically shorter than the original data.
- Uniform Distribution: Data should be evenly spread across the range of hash values.
- Pre-image Resistance: Given a hash value, it should be infeasible to determine the original input.
Hash functions are widely used in various fields, including data indexing, encryption, and load balancing.
History and Development of Hashing
The concept of hashing originated in the 1950s with the need to efficiently manage data storage and retrieval. In the early days of computing, hashing was developed as a solution to reduce data access time significantly.
Over the decades, numerous advancements have refined hashing algorithms, such as SHA (Secure Hash Algorithm) and MD5 (Message-Digest Algorithm 5), to balance speed and security.
Hashing can simplify database queries. For example, searching for a student ID within a college database becomes faster using hash tables, as opposed to scanning the actual list of IDs. This can be illustrated in pseudo code:
hash_table = {}student_list = ['Alice', 'Bob', 'Cathy']for student in student_list: hash_value = hash(student) hash_table[hash_value] = studentOnce organized, lookups based on the hash value are far more efficient.
Hashing technology has evolved to serve in areas beyond simple data management. Cryptographers began integrating hash functions into the encryptions of modern networks. Specialized versions of hash functions were needed to enhance security measures across internet-based transactions, thus spawning cryptographic hash functions.
Cryptographic hash functions are crucial because they not only preprocess data, but also provide assurance of data integrity through properties like collision resistance. Collision resistance ensures that it is computationally challenging to find two different inputs that produce the same hash output. The evolution has led us through versions designed to be increasingly resilient against attacks, contributing to internet security protocols today.
Hashing in Computer Science
Hashing is a fundamental concept in computer science that helps transform input data into a hash code. It plays a central role in many applications such as data storage, retrieval, and encryption, ensuring efficiency and security.
Role of Hashing in Data Structures
Hashing is crucial in data structures, especially for hash tables, which provide efficient data retrieval. A hash table uses a hash function to compute an index into an array of slots, from which the desired value can be found. It is vital to enable quick access to data, optimizing both the read and write processes. Hash tables are particularly effective in scenarios where there is a significant number of insertions and lookups.
class HashTable: def __init__(self): self.table = [None] * 10 def insert(self, key): index = hash(key) % len(self.table) self.table[index] = keyIn this Python example, a simple hash table class is implemented. Using modulo operator ensures the index is within range.
In hash tables, collisions occur when two keys map to the same index. Handling collisions efficiently is essential for maintaining performance.
Hash Table: A data structure that maps keys to values, optimizing the operation of simple operations like search, insert, and delete.
To further understand hash tables, consider its applications in various data structures like dictionaries in Python or maps in Java. These structures use hashing to quickly find associated values. Hashing ensures that dictionary lookups, even with a vast number of elements, remain time-efficient, average out to constant time O(1). The effectiveness of a hash table is heavily dependent on a good hash function and the strategy used for collision resolution.
Use of Hashing in Cryptography
Hashing in cryptography ensures data integrity and security. Unlike simple data structures, cryptographic hashes are designed to be a one-way function, meaning they should not be easily reversible. This property makes them suitable for verifying data, creating digital signatures, and ensuring data integrity.
Cryptographic Hash Function: A hash function that provides security properties such as pre-image resistance, collision resistance and is used in cryptographic applications.
Cryptographic hashes like SHA-256 generate fixed-size outputs from inputs of any size, making it extremely difficult to recreate the original input without extensive computational resources. These are primarily used in verifying data integrity. For example:
A simple hashing process for password verification might look like:Step 1: Input passwordStep 2: Hash password using a cryptographic hash functionStep 3: Compare hash result to stored hash value
def verify_password(stored_hash, user_input): return hash(user_input) == stored_hash
Cryptographic hash functions underpin modern encryption protocols like SSL/TLS, ensuring secure communications over the internet. Their robustness comes from properties such as collision resistance, which ensures distinct inputs nearly always produce unique hash outputs. For SSL/TLS, for instance, hashes ensure the integrity of transmitted data packets, securing them against tampering.
Hashing Algorithm
The hashing algorithm is a method of converting input data into a fixed-size string of characters, known as a hash value or hash code. These algorithms are essential in computing for tasks such as searching, data storage, and ensuring data integrity.
Popular Hashing Algorithms
Various hashing algorithms have developed over the years, each suitable for different applications. The most common ones include:
- MD5 (Message-Digest Algorithm 5): Once a widely-used hashing algorithm known for its fast computation, MD5 produces a 128-bit hash value. However, due to vulnerabilities and the ease of collision attacks, it is no longer considered secure for cryptographic purposes.
- SHA-1 (Secure Hash Algorithm 1): Producing a 160-bit hash value, SHA-1 was used extensively before vulnerabilities led to its deprecation in favor of more secure algorithms.
- SHA-256 (Secure Hash Algorithm 256): Part of the SHA-2 family, SHA-256 creates a 256-bit hash and is widely used in cryptographic functions, offering a good mix of security and performance.
- Bcrypt: Unlike traditional algorithms, Bcrypt is adaptive and includes a salt—a random data value—to protect against rainbow table attacks. It recalculates its hashes over time, an advantage in password hashing.
Let's see how SHA-256 is implemented using Python:
import hashlibdef compute_sha256(input_string): sha_signature = hashlib.sha256(input_string.encode()).hexdigest() return sha_signature
SHA-256's robustness comes from its structure of the Merkle-Damgård construction, which processes input data in fixed-size blocks, generating secure hash codes. Its resilience against pre-image and collision attacks makes it suitable for applications such as certificate signatures and integrity checking. Despite its security, future standards may demand even stronger algorithms like SHA-3, necessitated by ongoing advancements in computational power.
How Hashing Algorithms Work
Hashing algorithms operate by transforming data of any size into a predetermined size using a series of steps, typically involving bitwise operations, modular arithmetic, and other computational techniques. The resultant hash value serves as a fingerprint for the input data.
Understanding the operation of hashing algorithms relies on several key phases:
- Initialization: The algorithm initializes certain parameters like block size and initial hash values. For example, SHA-256 has specific initial hash values defined by its standard.
- Processing: Inputs are divided into fixed-size blocks. For methods like SHA-256, padding is added to ensure the input size matches the necessary requirements for the blocks.
- Compression: Each block undergoes a compression function, usually involving permutations and logical operations to mix data thoroughly, improving diffusion and avalanche effect, where a small input change results in a significant change in output.
- Finalization: The final hash value is concatenated from the output of the last block's operation, providing the ultimate digest or signature of the input.
Avalanche Effect: A characteristic of good hash functions where a small change in input results in a drastic change in the output.
Consider modeling a simplified hashing function with basic operations:
def simple_hash(input): hash_value = 0 for char in input: hash_value = (hash_value + ord(char) * 31) % 1000 return hash_valueHere, simple_hash iterates over each character, modifying hash_value with each iteration, demonstrating principles like using modular arithmetic and constants for mixing block values.
When evaluating hash functions, consider properties like collision resistance, pre-image resistance, and secondary pre-image resistance to ensure high security and low probability of two different inputs producing the same hash.
Hash Function Properties
Understanding the properties of hash functions is essential for comprehending their applications and effectiveness in various scenarios. These properties ensure that hash functions perform optimally in both typical and cryptographic contexts, providing both efficiency and security.
Key Properties of Hash Functions
A well-defined hash function should have several important properties to cater to diverse computing needs:
- Deterministic: Each input should consistently provide the same output, ensuring reliability in data operations.
- Fast Computation: Hash functions should compute the hash value swiftly, optimizing processing time regardless of input size.
- Compression: Inputs of arbitrary size are compressed into a fixed-size output, conducive for space constraints.
- Pre-image Resistance: Given a hash value, it should be computationally infeasible to determine the original input.
- Collision Resistance: It should be hard to find two different inputs with the same hash output, ensuring uniqueness.
- Secondary Pre-image Resistance: For given \text{input 1} and \text{output}, it should be infeasible to find \text{input 2} such that both produce the same output.
The Avalanche Effect is a fundamental attribute where a slight change in input dramatically alters the output, enhancing security.
For example, consider two similar inputs that are hashed, but just one character is different. The resultant hashes should be drastically different due to the avalanche effect. In mathematical terms: For inputs input_1 and input_2, if hash(input_1) ≠ hash(input_2)
strongly holds, the effect is achieved.
An example of a deterministic hashing using Python's hash function:
def demonstrate_hash(input_string): return hash(input_string) == hash(input_string)Here, demonstrating determinism means the input yields the same hash when processed multiple times.
One real-world application of hash functions showcasing these properties is in digital signatures. A message's hash code—a succinct representation of the content—is encrypted; the encrypted hash allows recipients to verify the message's integrity without exposing the original message. This works under the assumption that producing the same hash requires an identical original message, leveraging collision resistance effectively. On a deeper level, collision resistance has significant implications for blockchains, where each block references the hash of the previous block. Discovering two distinct data sets with identical hashes—creating a 'collision'—could potentially allow fraudulent activities in this distributed ledger technology. Hence, careful consideration and selection of robust hash functions are fundamental in these systems.
While designing systems, it's crucial to balance between the speed and security of hash functions based on the application's specific requirements.
Importance of Hash Function Properties
Hash function properties are pivotal in ensuring that data manipulation and storage remain efficient and secure. Emphasizing these properties allows developers to build scalable and reliable applications.
The importance of these properties is highlighted by their ability to:
- Maintain Data Integrity: Hash functions verify the integrity of data by comparing hash values before and after transmission.
- Enhance Performance: Fast computation and deterministic nature reduce querying time in data structures like hash tables, supporting large-scale applications.
- Secure Data: Properties like collision and pre-image resistance fortify cryptographic applications, safeguarding sensitive information.
- Enable Efficient Map Storage: Hash functions are crucial for effective storage and retrieval in associative arrays, or hash maps.
Consider using a hash function to store and quickly retrieve user passwords securely:
def hash_password(password): # Pseudo-salt application for added security salt = '12345' return hash(password + salt)The above example shows how hashing combined with salting strengthens password security during storage.
In situations where security is paramount, choosing robust hashing techniques can make the difference between a secure system and one vulnerable to compromise.
In complex systems such as distributed caches or cloud-based storage architectures, the choice of a hash function impacts load balancing and fault tolerance immensely. Hash functions help distribute data consistently across servers, ensuring no single server is overburdened. This introduces the concept of consistent hashing, which is instrumental in dynamically adding or removing storage nodes with minimal data redistribution. The efficiency offered by a well-chosen hash function is indispensable in maximizing resource utilization and performance of the entire system.
Importance of Hashing
Hashing stands as a cornerstone in the realm of computer science, pivotal in the efficient management, integrity, and security of data. From optimizing search algorithms to securing sensitive information in databases, the role of hashing spans a broad spectrum of applications. Its importance is reflected in its capability to transform data into a secure, manageable format, making it indispensable for both computational integrity and security protocols.
Why Hashing Matters in Cybersecurity
In cybersecurity, the need for hashing is paramount as it enhances the security frameworks that protect sensitive information from unauthorized access and alterations. Hash functions are part of the backbone in securing digital communications, as they ensure data integrity and authentication without exposing the data itself.
By generating unique hash values, even minor alterations in the input data can be detected instantly. Hence, hashes are integral to cybersecurity in aspects like:
- Data Integrity: Hashing verifies that data received is exactly as sent; unchanged and untampered.
- Password Security: Storing passwords in their raw form presents security risks. Hashing encrypts passwords, creating a barrier against direct attacks.
- Secure Transactions: Digital signatures often involve hashing, ensuring the authenticity and integrity of messages and documents.
Consider a financial institution that uses hashing to ensure transaction integrity:
def generate_transaction_hash(transaction_details): import hashlib return hashlib.sha256(transaction_details.encode()).hexdigest()This method ensures that any unauthorized changes to the transaction would result in a completely different hash, flagging potential data tampering.
Hash collisions are a vulnerability in cybersecurity; choosing robust hashing algorithms reduces the possibility of two inputs producing the same hash.
A deeper look into hashing and cybersecurity reveals the critical role played by algorithms like SHA-256 and SHA-3. These algorithms ensure not just data integrity but also reinforce cryptographic protocols such as digital certificates and blockchain technology. The strength of these hash functions lies in their ability to provide pre-image, collision, and secondary pre-image resistance, forming a formidable defense against hash breaches. Moreover, in applications like blockchain and secure socket layers (SSL/TLS), hashes reference previous blocks or certificates. This linkage ensures that any alteration is immediately apparent, thus preventing unauthorized interventions. The cryptographic security provided by hashing is fundamental for distinguishing credible data from fabricated or tampered data.
Advantages of Using Hashing
Hashing offers numerous advantages that extend beyond just cybersecurity. Its utility in varied computing contexts enhances both performance and data management.
Here are some of the key advantages provided by hashing:
- Efficiency: Hashing optimizes the process of data searching and retrieval, central to operations such as database indexing and cache storage.
- Data Integrity: Ensures the authenticity of data by detecting unauthorized modifications through checksum verification.
- Memory Optimization: By transforming large data inputs into concise hash codes, hashing reduces memory usage.
- Non-reversible: It's inherently difficult to reverse-engineer the original data from its hash, which enhances privacy.
Hash tables, for instance, illustrate hashing's efficiency in data storage:
class SimpleHashTable: def __init__(self, size): self.size = size self.table = [None] * size def add(self, key, value): index = hash(key) % self.size self.table[index] = valueThis Python class demonstrates a simplistic hash table that assigns values efficiently based on hash-generated indices.
Beyond mere security and efficiency, hashing serves advanced computing needs in distributed systems. Techniques like consistent hashing are essential in load balancing across multiple servers. By evenly distributing records, consistent hashing enables horizontal scaling, preventing server overload and ensuring seamless data management. This capability is crucial in large-scale cloud computing services like Amazon Web Services (AWS) or Google Cloud Platform (GCP), where services are dynamically allocated based on demand. In these scenarios, hashing algorithms contribute significantly to overall system resilience and performance, support redundancy, and enhance data availability.
When it comes to blockchain technology, hashes ensure that every block is connected securely, maintaining an immutable and cryptographically secure transaction ledger.
Hash Function Applications
Hash functions are foundational components in numerous computer science applications. Their ability to efficiently map and manage data makes them indispensable in modern technology.
Applications in Cybersecurity
Hash functions contribute significantly to enhancing cybersecurity measures. They serve critical roles, ensuring data integrity, confidentiality, and authentication. Cybersecurity implementations include:
- Data Integrity: Hash functions verify the integrity of files and messages. A hash of the original data, when compared with the received hash, confirms unchanged data integrity.
- Password Storage: Passwords are stored as hash values instead of plaintext. This prevents unauthorized access, as revealing the original password from the hash is nontrivial.
- Digital Signatures: Digital signatures utilize hashing to authenticate sender identity and verify that content has not been tampered with.
For instance, in ensuring data integrity:
import hashlib def verify_integrity(original_data, received_hash): computed_hash = hashlib.sha256(original_data.encode()).hexdigest() return computed_hash == received_hashThis Python code verifies whether the original data matches its expected hash, ensuring integrity.
SHA-256 is commonly used in cryptography due to its strong security properties against collision attacks.
Hashes secure blockchain networks where they form the backbone of block linking and verification. Each block's hash in the chain includes the preceding block's hash, creating a secure, tamper-proof ledger. Altering a single block would require regenerating hashes for all subsequent blocks, an infeasible task given current computational limits. This structure, combined with consensus algorithms, ensures blockchain integrity and credibility.
Other Uses of Hash Functions in Computer Science
Outside of cybersecurity, hash functions are employed extensively across various domains in computer science. They efficiently manage and organize data, crucial for performance optimization.
- Database Indexing: Hashing enhances the rapid retrieval and modification of data in databases by transforming search keys into fixed locations.
- Load Balancing: In distributed systems, hashes distribute requests evenly across servers, optimizing resource utilization and avoiding overload.
- Cache Operations: Hashes swiftly map requests to cached data, improving application response times in memory management. Hash-based caches allow efficient data retrieval and storage.
An application of hashing in databases might look like this for caching:
class Cache: def __init__(self): self.cache = {} def update_cache(self, key, data): hash_key = hash(key) self.cache[hash_key] = dataThis basic cache system uses hashed keys, enabling swift data access and storage.
In load balancing, consistent hashing is used to minimize the reorganization of cache or database entries when nodes join or leave the network.
In-memory databases like Redis and key-value stores rely heavily on hash functions. These sequences of bits allow databases to precisely index and evaluate lookup speed versus collision resistance for scalable, reliable storage solutions. The adaptability of hashing is further observed in data processing tasks like deduplication, anomaly detection, and large-scale data analysis, where rapid equality checks of data elements are essential.
hashing - Key takeaways
- Definition of a Hash: A hash is a function that converts input data into a fixed-length string, known as a hash value, used for efficient data retrieval.
- Hashing in Computer Science: Hashing is used to transform input data into hash codes, essential in systems for data storage, retrieval, and encryption.
- Hashing Algorithm: A method to convert input data into a hash value, critical for tasks like searching and data security.
- Hash Function Properties: Key properties include determinism, fast computation, compression, pre-image resistance, and collision resistance.
- Importance of Hashing: Hashing enhances data integrity, performance, and security in computing, particularly in search optimization and cybersecurity.
- Hash Function Applications: Used in cybersecurity for data integrity and authentication, and in computer science for tasks like database indexing and load balancing.
Learn faster with the 12 flashcards about hashing
Sign up for free to gain access to all our flashcards.
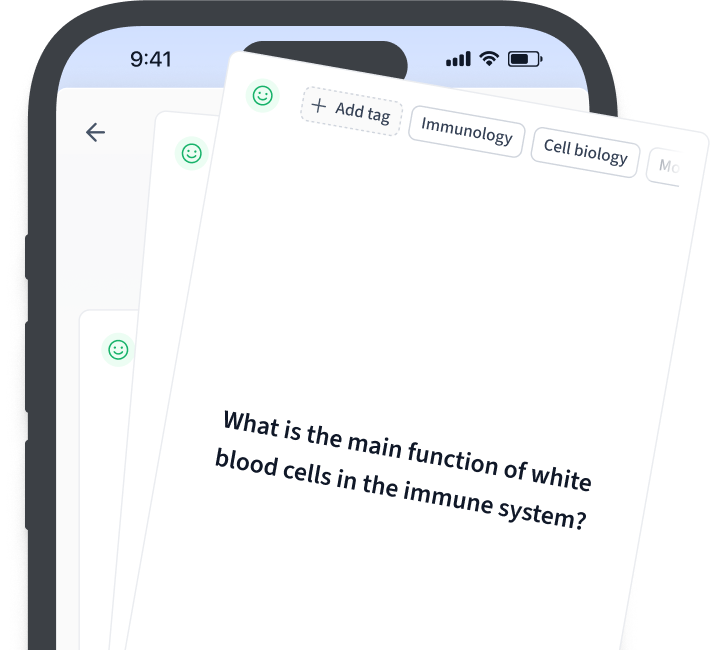
Frequently Asked Questions about hashing
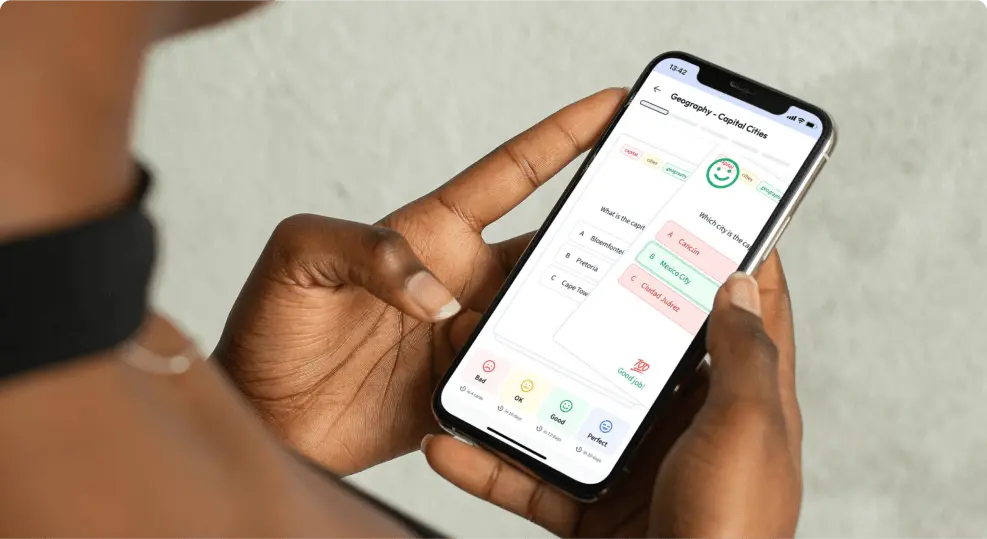
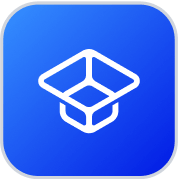
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more