Jump to a key chapter
Understanding HMAC
To gain a complete understanding of HMAC, you need to know its role and how it functions within cryptography. HMAC stands for Hash-based Message Authentication Code, and it is an essential method leveraged to ascertain the integrity and authenticity of a message.
Definition
HMAC is a specific construction for calculating a message authentication code (MAC) involving a cryptographic hash function and a secret cryptographic key. HMAC can verify the data integrity and authenticity of a message transmitted over the network by combining a specific hash function with a secret key.
To better understand HMAC, consider the following pseudocode example that uses the HMAC function:
function HMAC(key, message): blockSize = 64 //for SHA-256 if (length(key) > blockSize): key = hash(key) if (length(key) < blockSize): key = key + zeros(blockSize - length(key)) oKeyPad = key XOR [0x5c * blockSize] iKeyPad = key XOR [0x36 * blockSize] return hash(oKeyPad || hash(iKeyPad || message))
HMAC Technique in Cryptography
The HMAC Technique is a cornerstone in cryptography, designed to ensure both the integrity and authenticity of messages transmitted over insecure channels. This technique combines a cryptographic hash function with a secret key to create a message authentication code.
Mechanism of HMAC
Understanding the mechanism of HMAC requires a deep dive into its components and operations:
- Hash Functions: HMAC utilizes standard hash functions like SHA-256 or SHA-1 to ensure message integrity.
- Secret Key: A private key is shared between the communicating parties to authenticate the message.
- Padding: The secret key is extended to match the block size of the hash function.
- XOR Operations: Two layers of XOR operations are applied, using inner and outer padding constants.
Consider the example of using HMAC for message authentication in a network protocol:
def generate_hmac(key, message): block_size = 64 # for SHA-256 if len(key) > block_size: key = hash_function(key) if len(key) < block_size: key = key + b'\0' * (block_size - len(key)) o_key_pad = bytes((x ^ 0x5c) for x in key) i_key_pad = bytes((x ^ 0x36) for x in key) return hash_function(o_key_pad + hash_function(i_key_pad + message))This code applies the HMAC mechanism using XOR operations and a hash function. The message’s integrity is verified through this process, ensuring it originates from the known sender.
Let's explore deeper into how HMAC functions in real-world applications. The usage of HMAC extends beyond simple message verification. It is especially prevalent in:
- Secure Communications: HMAC is used in Transport Layer Security (TLS) and Secure Sockets Layer (SSL) protocols to ensure secure communication over the internet.
- API Authentication: Numerous web services use HMAC to authenticate API requests, ensuring that requests have not been altered in transit.
- Data Integrity Verification: In financial services, HMAC is an essential part of ensuring the integrity of transactions and data records.
HMAC can be combined with any cryptographic hash function, but its security depends on the strength of both the hash function and the secret key length.
Exploring HMAC Hash Functions
As you explore the domain of cryptography, you'll encounter the term HMAC, which stands for Hash-based Message Authentication Code. HMAC is crucial for verifying the integrity and authenticity of information shared over a network.
The Role of Hash Functions in HMAC
The foundation of HMAC lies in its use of hash functions. Hash functions are mathematical algorithms that transform input data into a fixed-size string of bytes. These functions play a pivotal role in HMAC by ensuring:
- Consistency: A given input will always produce the same hash output.
- Efficiency: The function must be able to process data swiftly.
- Determinism: The output is uniquely related to the input, providing security through unpredictability.
The following example uses the HMAC function in Python, highlighting how hash functions come into play:
import hashlibimport hmacdef generate_hmac(key, message): return hmac.new(key, message, hashlib.sha256).hexdigest()key = b'secret_key'message = b'my_message'hmac_result = generate_hmac(key, message)print(hmac_result)This code sample demonstrates how HMAC uses SHA-256 to generate a secure hash that verifies the message authenticity.
Mathematics Behind HMAC
The mathematical construction of HMAC is detailed and robust. At its core, the formula involves several steps:
- Key Padding: Adjusting the key by hashing or zero-padding to fit the block size of the hash function, commonly 64 bytes for SHA-256.
- Form the inner padding (i_key_pad): (key XOR 0x36 * 64).
- Form the outer padding (o_key_pad): (key XOR 0x5C * 64).
- Compute HMAC: \(HMAC = hash(o_key_pad \parallel hash(i_key_pad \parallel message)))\.
Did you know? While the key length in HMAC doesn't need to be secret, it should be sufficiently random and long to prevent brute force attacks.
To delve further into HMAC's practical applications, it's crucial to understand its adaptation in various security protocols.
Application | Description |
TLS and SSL | HMAC is employed to authenticate messages ensuring secure online transactions. |
IPsec | Used for verifying data packets in network security architectures. |
Amazon Web Services (AWS) | HMAC authenticates API requests to enhance security by preventing unauthorized access. |
HMAC Security Considerations
In understanding the security considerations of HMAC, it is vital to delve into how it upholds data integrity and authenticity. The method of using a combination of a hash function and a secret key makes HMAC robust against tampering.
Implementing HMAC-SHA1
Implementing HMAC-SHA1 involves using the SHA-1 hash function within the HMAC protocol. This method is commonly employed for verifying the integrity of information in cryptographic applications.
HMAC-SHA1 is a version of HMAC that uses the SHA-1 cryptographic hash function. It ensures that message data has not been altered by using both a key and a hashing process for verification.
Consider the implementation of HMAC-SHA1 in Python code:
import hmacimport hashlibdef generate_hmac_sha1(key, message): hmac_result = hmac.new(key, message, hashlib.sha1) return hmac_result.hexdigest()key = b'secret_key'message = b'message_to_authenticate'hmac_sha1_result = generate_hmac_sha1(key, message)print(hmac_sha1_result)This example demonstrates the generation of an HMAC using SHA-1 to authenticate a message.
A deep dive into the mathematics of HMAC-SHA1 reveals a few key steps:
- Key Padding: The secret key is adjusted, padded with zeros or hashed if longer than SHA-1's block size (64 bytes).
- XOR Operations: Two constants known as ipad and opad are used to XOR with the padded key.
- Inner and Outer Hashes: The inner hash is calculated using hash(ipad || message), and the outer hash is formed via hash(opad || inner hash).
While implementing HMAC, ensure that the secret key is of adequate length and complexity to prevent attacks that exploit weak keys.
HMAC - Key takeaways
- HMAC Definition: Hash-based Message Authentication Code, a method combining a cryptographic hash function with a secret key to ensure message integrity and authenticity.
- HMAC Hash Function: Utilizes standard hash functions like SHA-256 or SHA-1, essential for verifying data authenticity over a network.
- HMAC Technique: A cryptography method that protects message integrity through XOR operations, padding, and secure keys.
- HMAC Security: Provides robust data protection by combining a secret key with a hash function, making it resistant to tampering.
- HMAC-SHA1: A variant using the SHA-1 hash function for verifying message integrity in cryptographic applications.
- Applications: Used in secure protocols (TLS, SSL), API authentication, and data integrity verification in industries such as financial services.
Learn faster with the 12 flashcards about HMAC
Sign up for free to gain access to all our flashcards.
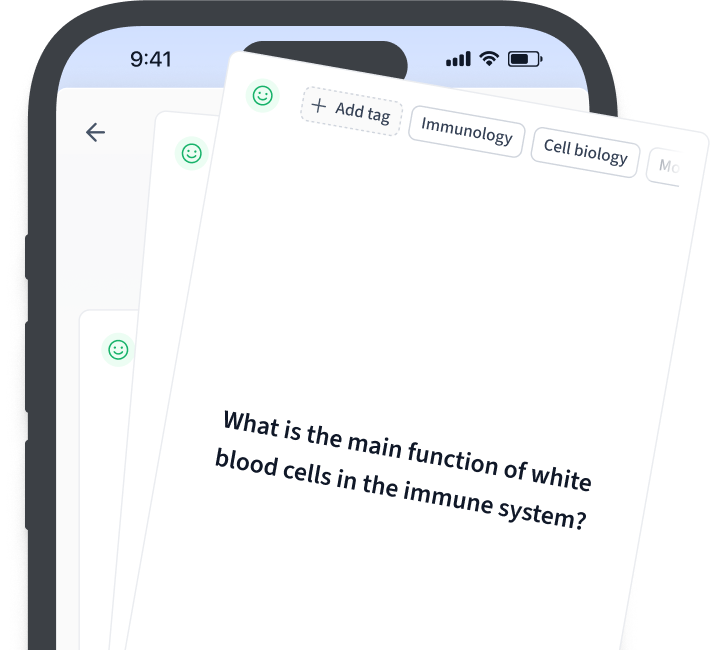
Frequently Asked Questions about HMAC
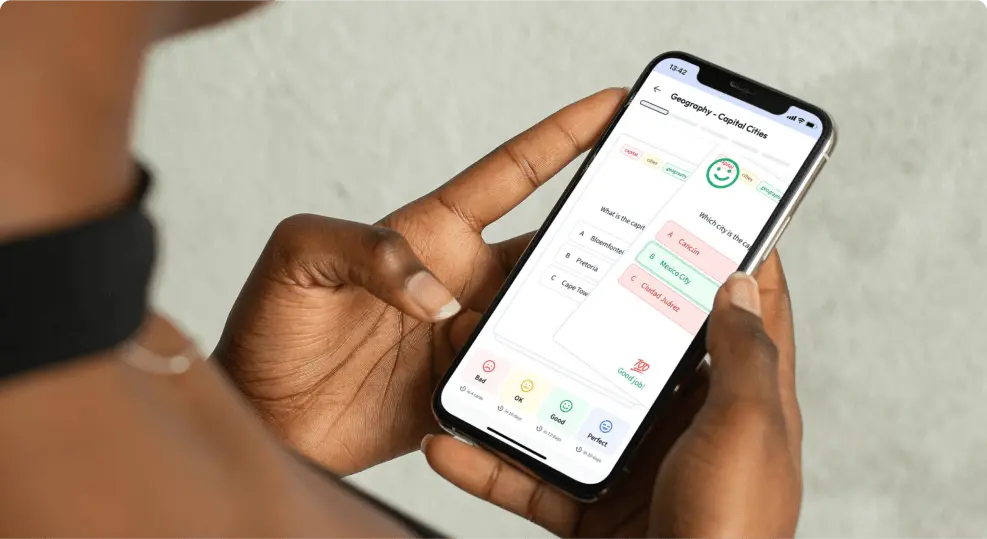
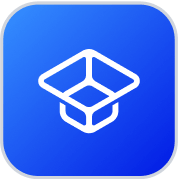
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more