Jump to a key chapter
Input Validation Definition
Input validation is a crucial aspect of computing that ensures the correct, intended functionality of an application. By verifying and sanitizing user inputs, you protect systems from unexpected errors or malicious activities.
What is Input Validation?
Input validation is the process of checking that input data meets certain criteria before processing it. This is a fundamental aspect of software development, aimed at ensuring data integrity and security. Input validation helps in protecting applications from various vulnerabilities and bugs that could be exploited by attackers.
Common criteria used in input validation include:
- Data Type: Ensures the data received is of the type expected (e.g., string, integer).
- Range Checking: Verifies that numerical inputs fall within acceptable limits.
- Format and Length: Specifies how data should be structured (e.g., email format).
For example, a field requesting an age input should only accept numerical values and restrict entries to a realistic range, such as numbers between 0 and 120.
Here's a Python example of a simple input validation for an integer:
age = input('Enter your age: ')if age.isdigit() and 0 < int(age) < 120: print('Valid age entered')else: print('Invalid age!')
Sanitization Process: Refers to cleaning input data to ensure no harmful data is processed.
Input Validation vs. Output Validation
While input validation focuses on ensuring that data entering a system is correct and safe, output validation is about ensuring data leaving the system is correctly formatted and secure. These are complementary techniques in secure coding practices.
The main differences include:
- Objective:Input Validation: Protects the system from invalid or malicious input.Output Validation: Ensures that output is safe for the end user and their environment.
- Location:Input Validation: Conducted at entry points such as forms or APIs.Output Validation: Applied when displaying data, such as when rendering HTML pages.
Both input and output validation play a vital role in maintaining the integrity and security of an application, but they serve different purposes in the development cycle.
Diving deeper, understanding the role of validation in preventing security vulnerabilities such as SQL injection or cross-site scripting (XSS) is critical. For instance, inadequate input validation can lead to attackers inserting malicious SQL statements or scripts. By performing thorough input validation, you can filter out these potentially dangerous elements. Various strategies, like whitelisting and blacklisting, help developers decide which data passes the validation checks. Whitelisting only accepts data that meet specified safe conditions, whereas blacklisting attempts to block items known to be harmful. A combination of both techniques often yields the best security outcomes.
Importance of Input Validation
Input validation is a key component in software development. It enhances both the security and the reliability of applications by ensuring that only correct data is processed.
Security Benefits of Input Validation
Ensuring security through input validation is pivotal in modern app development. Malicious users frequently exploit vulnerabilities, such as SQL injection or cross-site scripting (XSS), when input validation is overlooked.
Effective input validation mitigates such risks by:
- Filtering out malicious data inputs
- Preventing unauthorized system access
- Sustaining data integrity
Consider an example in Python for validating an email address format:
import reemail = input('Enter your email: ')pattern = r'^[\w\.-]+@[\w\.-]+\.\w+$'if re.match(pattern, email): print('Valid email address')else: print('Invalid email address!')
SQL Injection: A code injection technique that could destroy your database.
Tip: Always validate data on both the client and server sides for enhanced security.
Data Integrity and Input Validation
Data integrity concerns the accuracy and consistency of data over its lifecycle. Input validation is integral in preserving data integrity.
Here's how input validation ensures data integrity:
- Type Checking: Allows only the expected data type, such as integers for age fields.
- Range Validation: Ensures a value falls within a specified range, for example, a score between 0 and 100.
- Constraints: Like enforcing the presence of values in mandatory fields.
In depth understanding of input validation showcases its utility beyond security. The process also fosters consistency across different application modules by establishing validation rules that every input must adhere to. This uniformity is crucial in scenarios where multiple systems interact or when input data drives substantial business logic. For example, automated data processing pipelines benefit greatly from consistent input formats, minimizing preprocessing steps and reducing potential errors down the line.
Input Validation Examples
Exploring examples of input validation helps solidify your understanding of this critical practice. Whether applied in real-world scenarios or web forms, input validation supports both security and functionality.
Real-World Scenarios of Input Validation
Input validation in real-world applications safeguards various systems and enhances user experiences. Here are several scenarios where input validation is strategically applied:
- Online Banking Systems: Ensures user inputs during transactions like fund transfers are valid and prevents fraudulent transactions.
- eCommerce Sites: Validates product quantities and payment method information to maintain order accuracy.
- Healthcare Applications: Confirms patient data input is thorough and within accepted parameters to ensure reliable healthcare delivery.
An illustration of email validation using HTML and JavaScript:
function validateEmail(email) { const re = /^[\w\.-]+@[\w\.-]+\.\w+$/; return re.test(email);}const email = 'example@domain.com';if(validateEmail(email)) { console.log('Valid email');} else { console.log('Invalid email');}
Tip: Use client-side validation to enhance user responsiveness and server-side validation for a second security layer.
Input Validation in Web Forms
Web forms are integral to online interactions, and input validation is essential in these interfaces. Proper validation in web forms ensures that users provide accurate, safe, and valuable data.
Key aspects of input validation in web forms include:
- Required Fields: Ensures all necessary information is filled out before submission.
- Pattern Checks: Uses regular expressions to validate formats, like zip codes or phone numbers.
- Length Validation: Confirms that the input does not exceed the specified character limit.
Diving deeper, input validation in web forms can also involve complex logic to handle scenarios such as conditional fields. Conditional fields appear based on previous inputs (e.g., asking for delivery information only if the delivery option is chosen). Advanced web forms may use JavaScript libraries like jQuery or frameworks such as Angular for enhanced validation capabilities. These tools allow developers to create dynamic validations that provide real-time feedback to users, improving the user experience by indicating errors before submission.
Input Validation Techniques Explained
Understanding and implementing input validation techniques is essential for creating robust software systems. These techniques ensure that the data received by the application is both correct and safe for processing.
Common Input Validation Techniques
To secure data and maintain application integrity, several input validation techniques are widely used:
- Whitelist Validation: Accept only inputs that match predefined criteria.
- Blacklist Validation: Reject inputs that match known malicious values.
- Data Type Checks: Ensure data is of the expected type, such as integer or string.
- Bound Checking: Verify that inputs fall within established limits.
Consider a Python script that performs whitelist validation for usernames:
allowed_users = ['Alice', 'Bob', 'Charlie']username = input('Enter your username: ')if username in allowed_users: print('Access granted')else: print('Access denied')
In-depth knowledge of validation highlights its role in layered security strategies. Advanced techniques such as context-aware validation use the context of the input to make decisions. For instance, input expected at night might differ in validation steps compared to daytime data entries. Context-aware systems adapt validations based on environmental conditions, striving for even tighter security and personalization.
Form Input Validation Strategies
Form input validation is essential for collecting accurate and secure information through user interfaces. Effective strategies include:
- Client-side Validation: Enhances user experience by providing immediate feedback.
- Server-side Validation: Acts as the primary guard against harmful inputs, ensuring data integrity.
- Regular Expressions: Used extensively to validate formats like emails or phone numbers.
By incorporating multiple levels of validation, applications can enhance security and maintain data quality.
Validation Level | Purpose |
Client-side | Immediate user feedback |
Server-side | Prevent malicious data |
Developers should always implement basic validation logic independently on both the client and server sides to ensure application security.
Input Validation Python Methods
Python offers various methods to perform input validation efficiently and effectively. Here are some common Python techniques:
- isnumeric(): Checks if a string only contains numbers.
- Try-Except Blocks: Safely handles exceptions for incorrect data.
- Regular Expressions: Validates complex string patterns.
Here's a Python form validation example using try-except:
try: number = int(input('Enter a number: ')) print('You entered:', number)except ValueError: print('Invalid input! Please enter a number.')
Advanced validation with Python could employ frameworks like PyInputPlus, a third-party module designed specifically for input-checking in terminal applications. PyInputPlus includes features such as retry limits, timeout specifications, and customizable validators, streamlining the input validation process for developers.
input validation - Key takeaways
- Input Validation Definition: Process of checking input data against certain criteria to ensure data integrity and security in applications.
- Importance of Input Validation: Protects systems from errors and malicious activities, ensuring reliability and security of data.
- Common Input Validation Techniques: Whitelist and blacklist validation, data type checks, and bound checking to verify input safety.
- Input Validation in Python: Methods like
isnumeric()
, try-except blocks, and regular expressions for efficient validation. - Form Input Validation: Ensures around client-side validation for user feedback and server-side validation for data integrity and security.
- Security Benefits: Mitigates risks like SQL injection or cross-site scripting by filtering malicious inputs and ensuring data consistency.
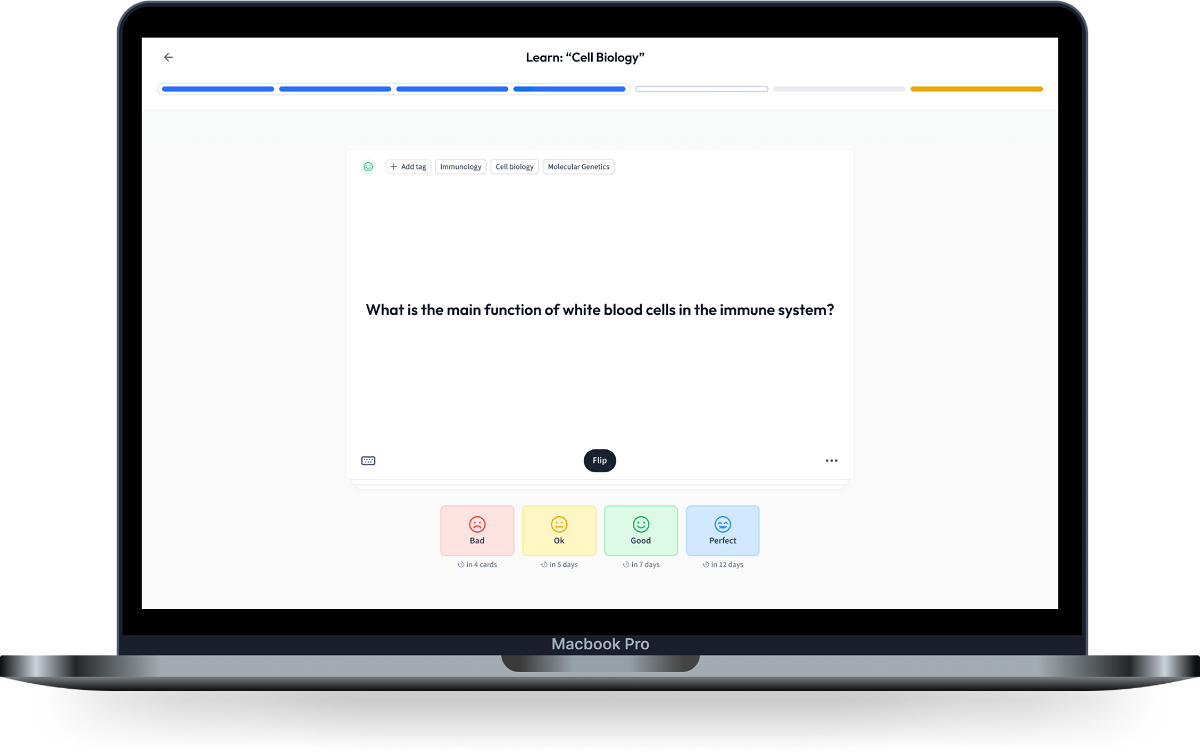
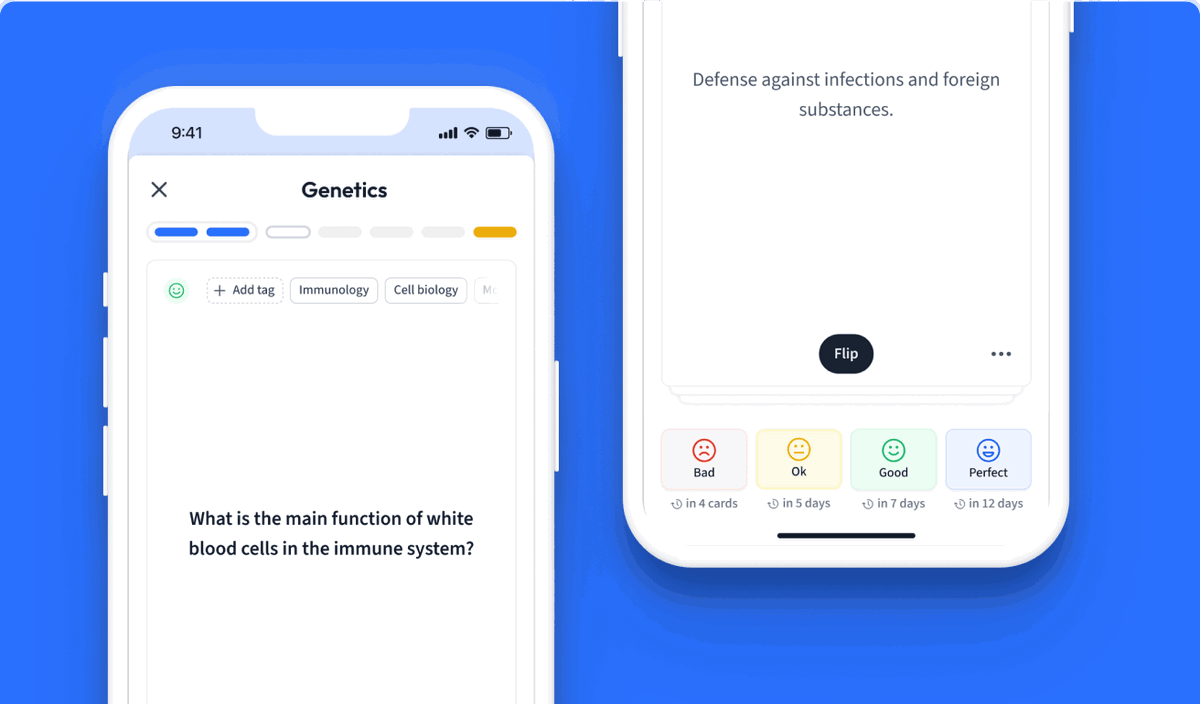
Learn with 12 input validation flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about input validation
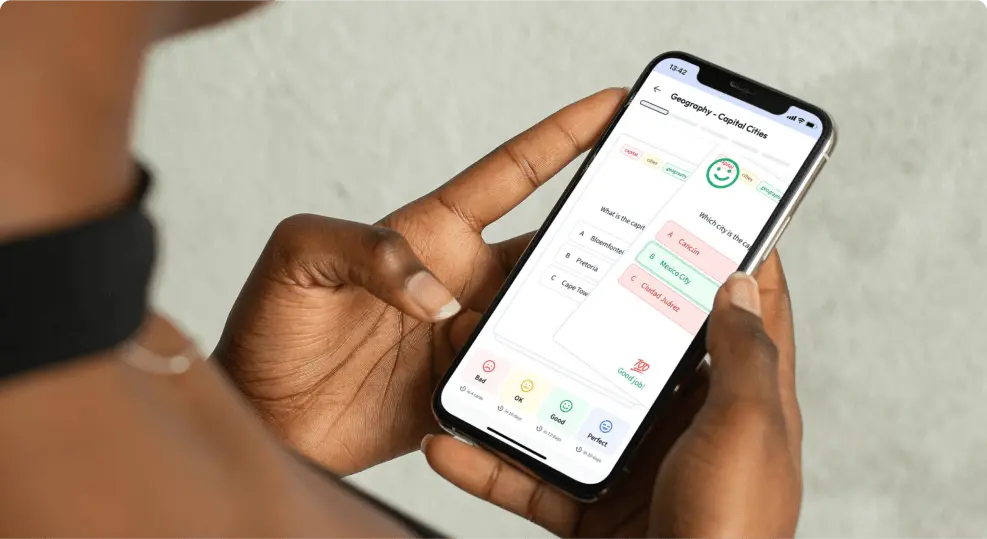
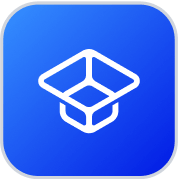
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more