Jump to a key chapter
Definitions of Advanced Data Structures
Advanced data structures are vital in optimizing the performance of algorithms, ensuring efficient handling of complex data sets. They provide solutions to specific data problems that primitive structures like arrays and linked lists may not handle effectively.
Basic Concepts of Advanced Data Structures
To understand the basic concepts of advanced data structures, you need a grasp of how these data structures optimize operations like insertion, deletion, and search. Key concepts you may encounter include:
- Trees: Hierarchical structures for sorted data representation, such as binary search trees and AVL trees.
- Heaps: Specialized tree-based structures that satisfy the heap property, aiding in efficient priority queue operations.
- Graphs: Structures consisting of nodes and edges, which are crucial in network modeling and pathfinding algorithms.
- Hash Tables: Structures that utilize hash functions to map keys to values, allowing for average O(1) time complexity for accesses.
- Tries: Tree-like structures commonly used for efficient retrieval of strings, crucial in lexical analysis and predictive text applications.
Advanced Data Structures: These are specialized data systems created to optimize certain operations and handle more complex data patterns effectively, beyond the capabilities of basic structures like arrays or lists.
Consider a binary search tree (BST), an advanced data structure that maintains sorted data. In a BST, each node follows the rule: all left descendants have smaller values, and all right descendants have larger values, ensuring efficient O(log n) search, insertion, and deletion times under average conditions.
One fascinating aspect of advanced data structures is how they enhance algorithm efficiency. For instance, in networking applications, the use of a graph to model relationships can dramatically improve the performance of route-finding algorithms. The famous Dijkstra's algorithm, which calculates the shortest path in weighted graphs, relies heavily on these intricate data structures. By representing roads as edges and intersections as vertices, it can efficiently find the shortest path, reducing complexity from exponential to polynomial time. Advanced data structures like these enable significant leaps in computational capability, broadening the horizons for what can be accomplished with data manipulation.
Advanced Data Structures Techniques Overview
Techniques in advanced data structures seek to refine and enhance standard operations to meet particular application needs. Understanding these techniques can significantly improve problem-solving efficiency in diverse computing scenarios. Here are some techniques:
Augmentation | Enhancing standard structures with additional data; for example, augmenting a BST to become an interval tree for range queries. |
Balancing | Maintaining tree balance to ensure logarithmic operations, as seen in AVL or Red-Black trees. |
Caching | Utilizing hash maps within other structures to store and quickly retrieve computed data bits. |
Partitioning | Dividing data into segments, improving the efficiency of search operations. |
Dynamic Programming | Storing previously calculated results to avoid redundant calculations, heavily useful in optimization problems. |
Always choose your data structure based on the operations' frequency you expect to perform and the data type you handle.
Advanced Data Structures and Algorithms
Advanced data structures and algorithms form the backbone of efficient computational solutions. These specialized structures harness unique designs to tackle problems that require optimized handling beyond the capacity of basic structures.
Relationship Between Data Structures and Algorithms
The relationship between data structures and algorithms is intrinsic to computer science. While data structures outline the method of storing and organizing data, algorithms define the process or set of rules to address data manipulation tasks. Choosing the right combination significantly impacts an application's performance.Consider sorting algorithms; the choice between using a heap (in Heap Sort) and an array directly modifies the algorithm's efficiency and resource usage. Efficiency, in this context, pertains to space and time complexity — critical factors represented using Big O notation.For effective performance optimization, use advanced data structures such as:
- Trees: These improve searching times, with certain types like Red-Black Trees keeping data balanced.
- Graphs: Essential for pathfinding and network flow algorithms.
- Hash Tables: Offer constant time complexity for searches, making them reliable for quick data retrieval.
- Tries: Perfect for managing string-data efficiently, utilized in auto-completion features.
When sorting data, employing a priority queue constructed using a heap optimizes the sorting process as follows in Python:
import heapqnums = [4, 1, 7, 3, 8, 5]heapq.heapify(nums) # Transform list into a heapsorted_nums = [heapq.heappop(nums) for _ in range(len(nums))]This transforms the list into a min-heap, ensuring retrieval of the smallest element first, leading to a sorted array in ascending order.
Data structures like graphs readily reinforce algorithms in complex domains such as cryptography or network analysis. For instance, employing an adjacency list versus an adjacency matrix for graph representation can influence the overall algorithm performance significantly. While adjacency lists efficiently cater to sparse graphs, with operations like edge addition taking constant time, adjacency matrices ease edge elimination and achieve faster access to cells but require more space due to their dense nature.The implications of selecting one data structure over another ripple through associated algorithms, as evidenced by pathfinding algorithms (e.g., Dijkstra's algorithm relies heavily on graphs). By representing nodes and connections appropriately, the algorithm can function optimally, respecting both time and space constraints.
Remember, the aptness of a data structure is directly proportional to the requirements and constraints of the algorithm you intend to implement.
With increasing data complexities, relying on advanced data structures to optimize algorithm operations is indispensable. This symbiotic relationship enhances the efficiency of computational tasks, ensuring swift and reliable data manipulation.
Examples of Advanced Data Structures Used in Algorithms
Various sophisticated data structures are deliberately crafted for specific use cases within algorithm applications. Leveraging these structures enhances an algorithm's capability to process larger datasets efficiently.Examples include:
- Segment Trees: Useful for answering range queries over static arrays, particularly in scenarios where modifications to data are sparse.
- Fenwick Trees or Binary Indexed Trees: Tailored for querying prefix sums and updates, these trees are swift solutions when dealing with arithmetic sequences.
- B-Trees: Utilized in databases and file systems for efficiently managing large blocks of sorted data, B-Trees facilitate quick lookup, insertion, and deletion operations.
- Skip Lists: These are layered linked lists that allow for balanced skip and search operations, improving on regular linked list limitations.
- Splay Trees: Self-adjusting trees with the splay operation that brings frequently accessed elements closer to the root, optimizing successive access.
In competitive programming, a Fenwick Tree or Binary Indexed Tree is often applied for quick cumulative frequency calculations. The operations in a Fenwick Tree, like prefix sum or update, run in logarithmic time, enhancing efficiency in scenarios with frequent prefix queries.
Applications of Advanced Data Structures
Advanced data structures are integral to the development of efficient and effective solutions in various domains. They empower algorithms to process complex data with ease, optimize performance, and ensure that systems remain responsive even under heavy tasks.
Real-World Applications of Advanced Data Structures
In the real world, advanced data structures are used extensively across numerous industries to solve authentic problems. Their ability to represent, manage, and manipulate data efficiently makes them indispensable in scenarios where quick access and high-speed data processing are critical.Here are some notable applications:
- Search Engines: Employ tries and inverted indexes for fast text search and auto-completion features.
- Networking: Use graphs for routing protocols to determine the shortest paths between devices.
- Database Management: Rely on B-Trees for indexing large data sets and ensuring quick data retrieval.
- File Systems: Utilize Red-Black Trees for managing and organizing files efficiently.
- Artificial Intelligence: Implement decision trees and neural networks to simulate human decision-making processes.
In an e-commerce platform, a hash table could be used to manage inventory. The hash table allows the platform to quickly check stock levels or update quantities based on sales and returns with average constant time complexity.
When designing complex systems, choosing the appropriate data structure can drastically reduce operational bottlenecks.
The financial industry heavily leverages red-black trees for order matching algorithms on stock exchanges. These data structures facilitate efficient transaction processing by maintaining a balanced state, ensuring that trades are executed in order and system lags are minimized. The self-balancing nature of red-black trees is vital in such time-critical environments, reducing the risk of order processing delays and maintaining trading integrity.In transportation technology, routing services use advanced graph algorithms coupled with data structures like adjacency lists to provide real-time directions. These systems must account for changing conditions, like traffic patterns or construction zones, requiring highly adaptable structures to shorter and most efficient routes.
Advanced Data Structures in Software Engineering
In the realm of software engineering, advanced data structures are pivotal in both system design and application development. These structures underscore an array of tasks from memory management to enhancing computational efficiency.Common implementations include:
- Cache Systems: Deploy hybrid structures such as hashmaps within arrays to provide both quick access and large storage faculties.
- Graphics Rendering: Utilize quad trees or octrees for spatial partitioning, helping to accelerate rendering in 3D applications.
- Compiler Design: Leverage symbol tables and graphs for representing intermediate code and syntax trees.
- Dynamic Memory Allocation: Implement splay trees to manage free memory blocks effectively in automated memory management practices.
- Concurrent Systems: Use lock-free structures to enable systems to handle multiple threads without downtime or corrupt data.
Quad Trees: A tree structure dividing a two-dimensional space by recursively subdividing it into four quadrants or regions.
An interactive game might use quad trees to manage and track object positions within the game's world. This data structure allows for rapid updates and efficient collision detection, ensuring smooth gameplay.
In concurrent programming, utilize fine-grained locking for critical sections to improve data access control and system responsiveness.
Examples of Advanced Data Structures
Advanced data structures are crafted to solve specific problems more efficiently than basic data structures. They often provide improved space and time complexities, accommodating the growing demands placed by modern applications and large datasets.
Characteristics of Advanced Data Structures
When examining advanced data structures, several characteristics become evident, reflecting their efficiency and adaptability. These include:
- Efficiency: Optimized for various operations like insertion, deletion, and searching.
- Scalability: Capable of handling growing datasets without significant performance degradation.
- Flexibility: Adaptable to support a range of operations and data types.
- Dynamicity: Ability to adjust automatically in response to data changes, such as balancing in self-balancing trees.
Dynamicity: A feature of some data structures that allows them to adapt as data changes over time, ensuring efficiency and performance are maintained.
For instance, consider a Red-Black Tree, which is a self-balancing binary search tree. By maintaining a balance despite a series of insertions and deletions, it ensures that operations such as search, insert, and delete always execute in logarithmic time in the worst case.
Exploring deeper, structures like AVL Trees offer assured logarithmic depth by enforcing strict balancing through rotations. This characteristic ensures they are slightly more balanced than Red-Black Trees, although rotations might be more frequent. The trade-off between balance and rotational frequency is a typical theme in the choice of advanced data structures. Thus, AVL Trees are particularly useful in scenarios where read-heavy applications require uniformly fast access times.Another significant example is the Fibonacci Heap, engineered for amortized analysis. In Fibonacci Heaps, certain operations are performed lazily, which connects with larger data structures found in applications like network routing and optimization tasks.
Common Examples of Advanced Data Structures
Several advanced data structures have become standard tools in computer science due to their specialized capabilities.
- Graphs: Utilize nodes and edges to model relationships, pivotal in network analysis and understanding complex systems.
- Tries: A form of search tree used to store a dynamic set, typically strings. Critical in text processing and auto-complete features.
- B-Trees: A generalization of a binary search tree that can have more than two children, essential in database indexing.
- Segment Trees: Used in scenarios requiring frequent range queries and updates, such as interval problems.
- Splay Trees: Self-adjusting trees that bring accessed elements to the root, optimizing frequent access times.
In database systems, a B-Tree is crucial for indexing, allowing the database engine to quickly locate, insert, or delete entries with minimal disk reads. This is particularly efficient for disk-based storage, where minimizing seeks is crucial.
Consider the computational cost and typical operations you will perform when choosing a data structure for your application.
Advanced Data Structures - Key takeaways
- Advanced Data Structures: Specialized data systems that optimize specific operations and handle complex data patterns beyond the capabilities of basic structures like arrays or lists.
- Key Concepts: Includes hierarchical tree structures, heaps, graphs, hash tables, and tries, each offering specific advantages in data manipulation and retrieval.
- Advanced Techniques: Includes augmentation, balancing, caching, and dynamic programming, which enhance efficiency and problem-solving in advanced data structures.
- Applications: Used in search engines, networking, database management, and AI for efficient data handling and quick retrieval.
- Examples: Includes segment trees, Fenwick trees, B-trees, skip lists, and splay trees, each designed for solving specific computational problems.
- Algorithmic Synergy: The relationship between data structures and algorithms enhances computational efficiency, crucial for performance optimization.
Learn faster with the 27 flashcards about Advanced Data Structures
Sign up for free to gain access to all our flashcards.
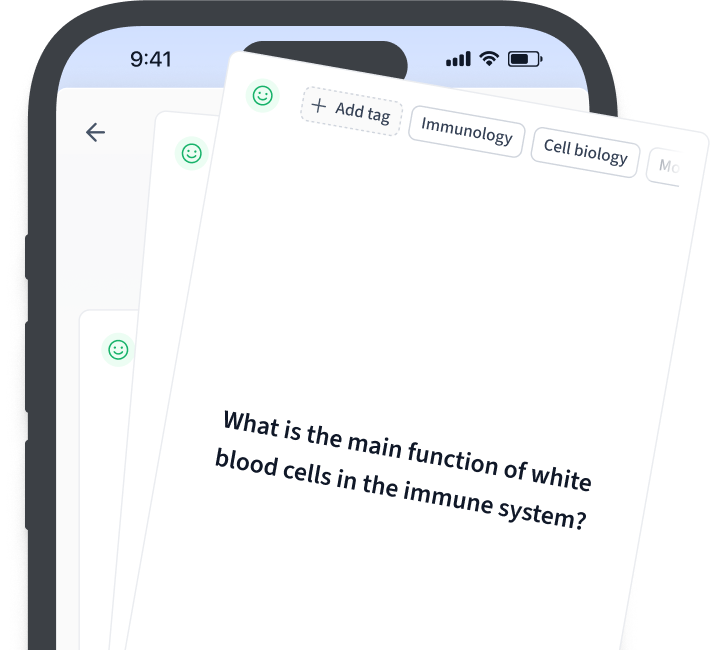
Frequently Asked Questions about Advanced Data Structures
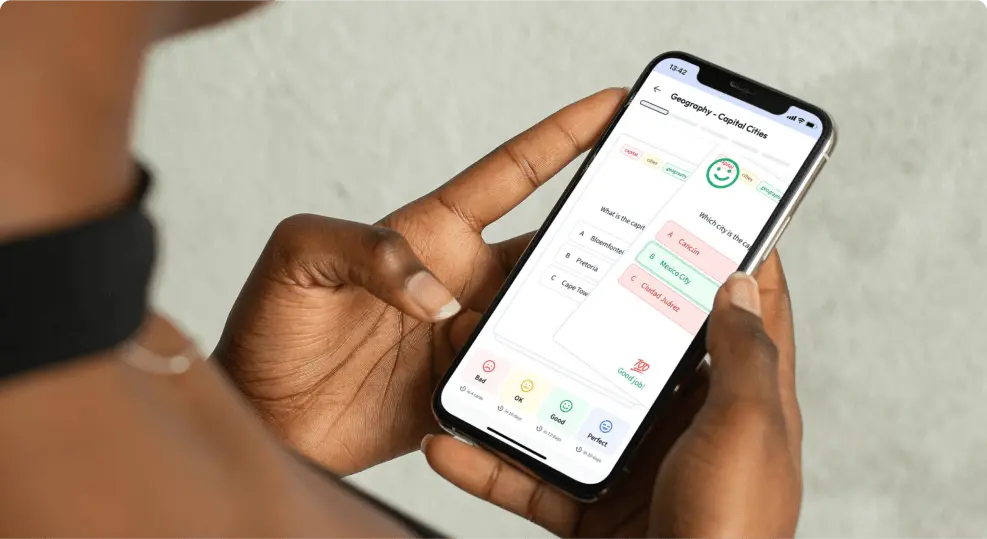
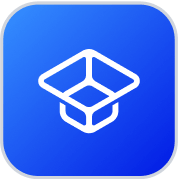
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more