Jump to a key chapter
AVL Tree Definition
AVL Trees are a type of self-balancing binary search tree where the difference in heights between the left and right subtrees cannot be more than one for any node. This ensures that the tree remains approximately balanced, allowing operations such as insertion, deletion, and lookup to be performed in logarithmic time, making AVL Trees highly efficient and ideal for dynamically changing data sets.
What is an AVL Tree?
An AVL Tree is a special type of Binary Search Tree (BST). In an AVL Tree, each node is assigned a balance factor, which is computed as the difference between the height of the left subtree and the height of the right subtree. An AVL Tree is always maintained so that the balance factor of every node is either -1, 0, or 1. This condition ensures the tree is balanced, which helps in maintaining the time complexity for various operations such as insertion, deletion, and retrieval at \(O(\log n)\).
Property | Value |
Balance Factor | -1, 0, or 1 |
Time Complexity | \(O(\log n)\) |
- Faster lookups compared to unbalanced trees due to the balanced height.
- Efficient for all basic operations, maintaining an \(O(\log n)\) time complexity.
Balance Factor is a property of an AVL Tree node calculated as the difference between the heights of its left and right subtrees: \(\text{Balance Factor} = \text{height(left subtree)} - \text{height(right subtree)}\)
Suppose you have an AVL Tree and you perform the following sequence of insertions: 20, 30, 10. The sequence will first create a tree with 20 as the root node. Adding 30 will leave the tree balanced, but inserting 10 as the left child of 20 would make the root unbalanced (left-heavy): Before balancing:
20 / \ 10 30The balance factor of node 20 becomes 2, which violates the AVL property. To rectify this, a 'right rotation' of the root node is performed, resulting in:After balancing:
10 / \ / 20 / \ 30Now, each node in the tree meets the balance factor condition, and the tree is once again balanced.
The balance factor helps determine the type of rotation required to maintain the AVL Tree's properties when nodes are added or removed.
Origin of AVL Trees
AVL Trees were named after the initials of their inventors, Adelson-Velsky and Landis, who introduced the concept in 1962. As one of the first self-balancing binary search trees, AVL Trees represented a significant advancement in data structure design by addressing the limitations of BSTs behaving more like linked lists in the worst case. The need for balanced trees arose from the challenge of maintaining the efficiency of search, insertion, and deletion operations in growing data collections. AVL Trees implement rotations to self-balance and maintain a height close to \(\log n\), which helps keep these operations efficient throughout the tree's lifespan. This made them particularly valuable in applications where performance and efficiency are of utmost importance, such as database systems and memory management in operating systems. This breakthrough in computer science has paved the way for modern balanced trees, including Red-Black Trees, which further optimized specific operations and usage scenarios.
AVL Tree Algorithm
The AVL Tree Algorithm is essential for maintaining balance in a tree structure, ensuring efficient operations by adjusting the placement of nodes. This section will explore the key operations: insertion, deletion, and search in AVL trees, which are designed to preserve the tree's height close to \(\log n\).
Insertion in AVL Tree
When inserting a new node in an AVL Tree, the following steps must be conducted to maintain balance:1. **Insert the node**: First, perform a standard BST insertion operation.2. **Check balance factors**: Traverse back up from the inserted node to the root to update and check the balance factors.3. **Perform rotations if needed**: If any node's balance factor becomes -2 or +2, rotations will be necessary to re-balance the tree. Here are the possible cases:
- Left-Left (LL) Case: A single right rotation is required.
- Right-Right (RR) Case: A single left rotation is applied.
- Left-Right (LR) Case: A left rotation followed by a right rotation.
- Right-Left (RL) Case: A right rotation followed by a left rotation.
Consider adding a node with value 13 to the following AVL Tree:
10 / \ 5 20 / \ 3 6Adding 13 to the right subtree:
10 / \ 5 20 / \ 13 6This insertion causes imbalance, remedied with a right-left (RL) case: Rotate right around 20, then left around 10, resulting in:
6 / \ 5 10 / \ 13 20
Always update the heights and balance factors of nodes traversed back during AVL Tree adjustments.
Deletion in AVL Tree
Deletion in an AVL Tree follows these key steps:1. **Search and remove**: Locate and remove the node using the standard BST deletion technique.2. **Update heights**: From the point of deletion, update the height of nodes back to the root.3. **Balance the tree**: Where the balance factor is disrupted (i.e., |balance factor| > 1), perform the necessary rotations to re-balance:
- Cater for LL and RR imbalances with single rotations.
- For LR and RL imbalances, apply double rotations.
Deleting node 6 from the previous balanced AVL Tree:
6 / \ 5 10 / \ 13 20After removal:
6 / \5 10 / \ 13 20Now, re-balance by performing a LL rotation on root node 6:
10 / \ 5 20 \ 13
AVL Tree Search Operation
The search operation in an AVL Tree is very similar to searching in a standard BST owing to the structural similarity:1. **Start at the root**: Use the conventional binary search approach, where if the key to search is lesser than or greater than the current node, recurse on the respective left or right subtree.2. **Balanced heights**: With the AVL Tree's guaranteed balanced height, the search operation stays efficient, with worst-case time complexity remaining at \(O(\log n)\).Consider the logic for searching a node with value 13:
10 / \ 5 20 \ 13Start from the root, and since 13 > 10, move to the right. Then, as 13 < 20, check its left child to find 13.This demonstrates the efficiency and predictability of search operations in an AVL Tree due to its smart balancing mechanism.
AVL Tree Balancing Concepts
Balancing is a core concept in AVL Trees, ensuring they remain efficient and effective for various operations. By understanding how rotations contribute to this balance, you can appreciate their impact on the tree's structure and operation times.
Understanding Rotations in AVL Trees
Rotations are operations that adjust the structure of an AVL Tree to maintain its balance after insertions or deletions. When nodes disrupt the balance factor, rotations restore it, allowing the tree to keep its properties.Key rotations are:
- Right Rotation - applied to a subtree that is left-heavy, bringing the left child up and rotating the previous root to the right.
- Left Rotation - applied to a right-heavy subtree, bringing the right child up and rotating the previous root to the left.
- Double Rotations - such as left-right or right-left rotations, applied when a direct rotation cannot balance the tree. These involve two steps, first rotating the child and then the parent.
Imagine you insert elements in an AVL Tree, leading to structure:
3 / 2 / 1This creates a left-left case, corrected by performing a right rotation:
2 / \ 1 3Now, the tree is balanced again with a height difference within allowable limits.
Remember that single rotations adjust only one side of the tree, while double rotations target more intricate imbalances.
Types of AVL Tree Rotations
AVL Tree rotations fall into several types, each specifically designed to handle a particular imbalance scenario:
- Single Right Rotation (LL): Corrects left-heavy imbalances by rotating nodes in a rightward direction.
- Single Left Rotation (RR): Address right-heavy nodes by moving nodes to the left.
- Double Left-Right Rotation (LR): Involves a single left rotation followed by a right rotation to address initial left-heavy trees where the subtree of concern is right-heavy.
- Double Right-Left Rotation (RL): A right rotation followed by a left rotation to manage right-heavy trees with a left-heavy immediate subtree.
Consider a scenario requiring a double rotation:Initial state:
5 / 2 \ \ 4Performing an LR rotation: First, rotate left around 2:
5 / 4 \ / 2Then, a right rotation around 5 results in:
4 / \ 2 5The tree is now balanced and follows AVL Tree rules.
Importance of Balancing in AVL Trees
The importance of balancing in AVL Trees cannot be overstated, as it directly influences their performance and efficiency. A balanced tree ensures that operations such as search, insertion, and deletion maintain logarithmic time complexity, which is vital for applications requiring swift data access and updates.A well-balanced AVL Tree:
- Reduces the maximum height, thereby minimizing worst-case scenarios often seen in basic binary search trees.
- Facilitates faster search and retrieval operations, crucial in large data sets and databases.
- Ensures consistent performance even with frequent and complex modifications to the tree's structure.
Balancing not only influences individual tree operations but also impacts overall system efficiency. In environments where AVL Trees back database indexes, consistent operation speeds can lead to significant improvements in data handling and retrieval times. Software that includes AVL Trees for dynamic datasets often sees better memory usage patterns and faster adaptive responses to data changes.Further, in computational applications where predictability of performance is crucial, AVL Trees provide a stable structure that can accommodate various complexities without sacrificing response times. This makes them a preferred choice in scenarios where data integrity and rapid access are essential, such as simulation environments and machine learning datasets that require real-time updates and evaluations.
AVL Trees in DSA
AVL Trees are a powerful tool in the realm of Data Structures and Algorithms (DSA). They maintain balance within binary search trees by dynamically adjusting nodes, ensuring swift data retrieval and manipulation.
Role of AVL Trees in Data Structures and Algorithms
In the context of data structures, AVL Trees serve several crucial roles:
- They provide logarithmic height for balanced operations.
- They enable efficient search, insertion, and deletion operations, all conducted in \(O(\log n)\) time.
- They help avoid degeneration into linked lists, maintaining optimal performance.
- Priority Queues, which utilize balanced trees for rapid priority-based retrieval.
- Databases, where AVL Trees manage index operations efficiently.
An AVL Tree is a self-balancing binary search tree where the balance factor, defined as the difference in heights between left and right subtrees, remains at -1, 0, or +1.
Let's walk through a simple example with an AVL Tree:Initial insertion of nodes creates this structure:
20 / \ 10 30Adding node 25 results in:
20 / \ 10 30 / 25This insertion makes node 30 unbalanced. A right-left (RL) rotation on node 30 resolves it.Final structure:
20 / \ 10 25 \ 30The rotations keep the tree balanced, preserving AVL properties.
Remember, rotations involve rearranging nodes while preserving the in-order sequence.
In algorithmic processes, the use of AVL Trees offers notable advantages over simpler structures. Consider real-time applications like memory-managed operating systems. An AVL Tree can dynamically manage memory allocation efficiently, thus enhancing processes that necessitate speed, such as dynamic loading of programs or resources.Further complexity arises in network databases where AVL Trees contribute to dynamic indexing. This is prominent in non-relational databases, supporting real-time data retrieval critical to social network functionalities, ensuring response under varying data loads.
Real-world AVL Tree Examples
AVL Trees find wide applications in real-world scenarios due to their balanced nature. You encounter these trees in:
- File Systems - Modern operating systems utilize AVL Trees for managing file indices to offer faster searches.
- Network Protocols - AVL Trees are integrated within network routing protocols to enhance the lookup speed for routing tables.
- Gaming Algorithms - They are used in vast multiplayer online games for rapidly accessing player data and states.
Inserting game data into AVL Trees ensures rapid access despite high-volume interactions.
Advantages of Using AVL Trees
The advantages of employing AVL Trees are numerous, particularly in environments demanding efficiency and reliability. Some highlighted benefits include:
- Predictability - AVL Trees maintain predictable operation times, crucial for real-time systems.
- Efficiency - Due to their balanced structure, AVL Trees consistently perform operations in \(O(\log n)\), optimizing processes linked to dynamic and large datasets.
- Robustness - They reduce the need for frequent restructuring compared to other tree types.
The robustness of AVL Trees extends into enhancing data systems like blockchain technology. Within blockchains, AVL Trees ensure fast cryptographic search throughout the distributed ledgers. This application is pivotal for maintaining transaction integrity while allowing swift access. The balance of AVL Trees ensures that even substantial chains do not significantly lag, preserving the efficiency required for real-time ledger updates and verifications critical to blockchain's security framework.
AVL Tree - Key takeaways
- AVL Tree Definition: An AVL Tree is a self-balancing binary search tree, where the height difference between left and right subtrees for any node is at most one, ensuring balanced tree operations.
- Balance Factor: In AVL Trees, the balance factor must be -1, 0, or +1, calculated as the height difference between left and right subtrees.
- AVL Tree Algorithm: The algorithm includes operations like insertion, deletion, and search, maintaining a balanced structure via rotations to ensure logarithmic time complexity.
- AVL Tree Rotations: Key rotations include right (LL), left (RR), double left-right (LR), and double right-left (RL) rotations, used to balance the tree after modifications.
- AVL Trees in DSA: Widely used in data structures and algorithms for maintaining balanced trees, enabling efficient search, insertion, and deletion in O(log n) time.
- Real-world Applications: AVL Trees are utilized in file systems, network protocols, and gaming algorithms for efficient data retrieval and management.
Learn faster with the 17 flashcards about AVL Tree
Sign up for free to gain access to all our flashcards.
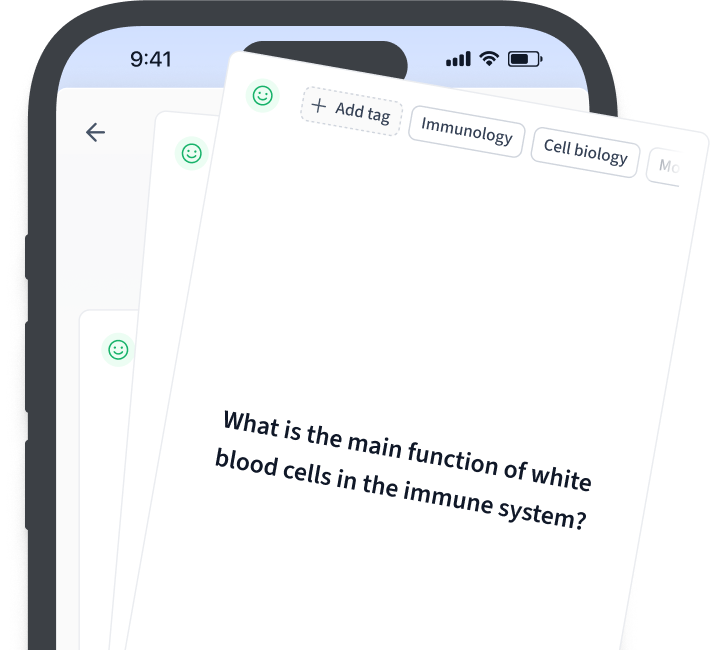
Frequently Asked Questions about AVL Tree
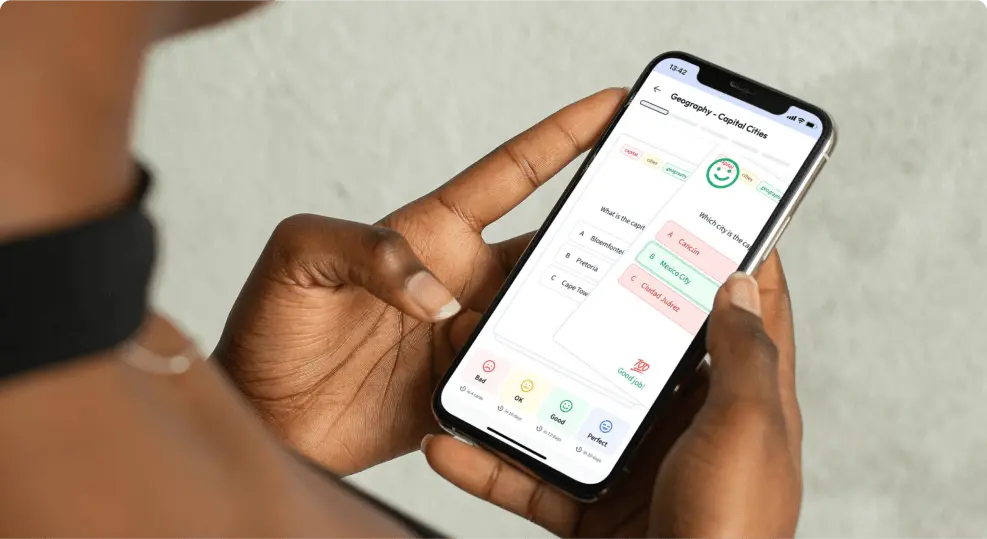
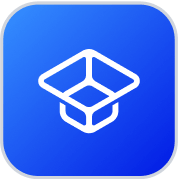
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more