Jump to a key chapter
Introduction to Creating Triggers in SQL
Creating triggers in SQL is an essential skill for any computer science student interested in database management and application development. This article provides a comprehensive overview of what triggers are, their purposes, and the different types you may encounter when working with SQL databases. By understanding the basics of triggers, you'll be well-prepared to create your own and optimise your database applications.
Definition and Purpose of Triggers in SQL
Triggers are automated actions that are executed in response to specific events occurring in a database. These events can include inserting, updating, or deleting data within a table. Triggers are used to maintain data integrity, enforce business rules, and automating specific processes that take place when data in the database is modified.
For example, consider a banking application where a user makes a money transfer. A trigger could automatically generate a new transaction in the 'Transactions' table whenever a new record is inserted into the 'Transfer' table. This ensures that all transactions are recorded, and helps maintain consistency and data integrity within the database.
Triggers can be thought of as stored procedures that are attached to a specific table and are invoked automatically when a specified event occurs. They are an integral part of database management because they allow developers to enforce specific conditions and automate various processes related to data modification.
Types of Triggers: After INSERT and After UPDATE
In SQL, triggers can be classified into various types depending on the event that they respond to. The main event types are:
- After INSERT
- After UPDATE
- After DELETE
- Instead of INSERT
- Instead of UPDATE
- Instead of DELETE
Each of these types corresponds to a specific action and event. We will focus on two of the most commonly used trigger types: After INSERT and After UPDATE.
After INSERT Trigger
An After INSERT trigger is executed when new data is inserted into a table. This type of trigger can help maintain data integrity and automate processes after adding a new row in the database. Some common applications of After INSERT triggers include:
- Validating the data being inserted
- Maintaining history or log of all changes made in the table
- Automatically updating values in other tables based on the new row
An example of an After INSERT trigger might involve an online store inventory. When a new product is added, the trigger could automatically increase the stock quantity in the inventory table, ensuring accurate stock levels are maintained.
After UPDATE Trigger
An After UPDATE trigger is executed when data in a table is updated. It can help maintain consistency and automate processes after modifying existing data in the database. Some typical implementations of After UPDATE triggers include:
- Validating the updated data
- Keeping a record of changes made to the data
- Updating related records in other tables based on the updated data
An example of an After UPDATE trigger might involve a hotel booking system. When a guest extends their stay, the trigger could automatically update the total cost of the booking, ensuring the guest is billed correctly.
Overall, understanding how to create triggers in SQL, and becoming familiar with their different types, allows you to better manage data by enforcing specific conditions and automating processes that take place when data in your database is modified.
Creating Triggers in SQL Explained
Creating triggers in SQL involves understanding their syntax, structure, and best practices. By mastering these elements, you will be able to build effective triggers that help manage and enforce data integrity, consistency, and automation within your database.
Syntax and Structure of SQL Triggers
To create a trigger in SQL, you must follow a specific syntax that communicates the event-type, timing, and conditions for the trigger to be executed. In general, the structure of an SQL trigger comprises:
- Trigger name
- Trigger event
- Trigger table
- Trigger timing
- Trigger action
The basic syntax for creating a trigger in SQL is as follows:
CREATE TRIGGER trigger_name ON table_name FOR|AFTER|INSTEAD OF event_name AS BEGIN -- Trigger action here END;
Here's a breakdown of the syntax components:
- CREATE TRIGGER: This is the SQL command used to create a new trigger.
- trigger_name: This is a unique identifier for the trigger.
- ON table_name: This specifies the table that the trigger will apply to.
- FOR|AFTER|INSTEAD OF: These keywords define the timing and type of the trigger. FOR and AFTER are used interchangeably, while INSTEAD OF is applied when the trigger should happen before the specified event.
- event_name: This can be INSERT, UPDATE, DELETE or a combination of these events, indicating when the trigger should be executed.
- AS, BEGIN, END: These keywords specify the start and end of the trigger action code block.
An example of a trigger that adds a new row to an audit table after updating a 'Customer' table might look like this:
CREATE TRIGGER UpdateCustomerTrigger ON Customer AFTER UPDATE AS BEGIN INSERT INTO Audit (Action, TableName, OldValue, NewValue) VALUES ('UPDATE', 'Customer', 'OldValue', 'NewValue') END;
Writing Trigger Code: Best Practices and Tips
When writing trigger code, it is essential to follow certain best practices and tips to ensure efficiency, maintainability, and performance in your database applications. Here are some useful guidelines:
- Keep triggers small and focused: Write concise trigger code, concentrating on specific tasks. Avoid complex logic to ensure easy maintenance and reduce performance issues.
- Use informative trigger names: Name your triggers descriptively, incorporating information about their purpose and the table on which they operate, making it easier to understand their function.
- Avoid using too many triggers: Relying heavily on triggers can reduce performance and complicate the database schema. Use triggers judiciously and consider alternative solutions such as stored procedures or application-level constraints.
- Test your triggers: Ensure your triggers function correctly and efficiently by testing them with various scenarios. Include edge cases to validate their performance under different conditions.
- Document your triggers: Provide clear descriptions and comments within the trigger code to facilitate maintenance and understanding for other developers.
- Avoid using non-deterministic functions: Using non-deterministic functions, such as GETDATE(), in triggers can lead to unexpected results and potential data corruption. Stick to deterministic functions when possible.
- Manage errors efficiently: Implement proper error handling and logging mechanisms within your triggers to ensure smooth operation and easy debugging.
Following these best practices and tips will enable you to create well-structured, efficient, and maintainable triggers in SQL. By mastering the syntax, structure, and principles of effective trigger code, you can leverage the power of triggers to enforce data integrity, automate processes, and optimise your database applications.
Creating Triggers in SQL Examples
In this section, we will delve into detailed examples covering the creation of triggers in SQL. Examining scenarios for After INSERT and After UPDATE triggers, you gain a thorough understanding of their practical implementations. Additionally, we will look into creating triggers in Oracle Database, showcasing sample code and use cases for a more comprehensive understanding of triggers across platforms.
Creating Triggers in SQL After INSERT Example
Let's consider a scenario where we want to create an After INSERT trigger to track added data in a 'Sales' table. Each time a new sale is registered, we want the trigger to automatically record the event in the 'SalesHistory' table for audit purposes.
First, we will create the 'Sales' and 'SalesHistory' tables:
CREATE TABLE Sales ( SalesID INT PRIMARY KEY, Product VARCHAR(100), Quantity INT, Price DECIMAL(10, 2), SalesDate DATE ); CREATE TABLE SalesHistory ( HistoryID INT PRIMARY KEY IDENTITY(1,1), SalesID INT, Product VARCHAR(100), Quantity INT, Price DECIMAL(10, 2), SalesDate DATE, AuditDate DATETIME );
Next, we will create the After INSERT trigger 'LogSalesHistory' that tracks new data added to the 'Sales':
CREATE TRIGGER LogSalesHistory ON Sales AFTER INSERT AS BEGIN INSERT INTO SalesHistory (SalesID, Product, Quantity, Price, SalesDate, AuditDate) SELECT SalesID, Product, Quantity, Price, SalesDate, GETDATE() FROM inserted END;
In this example, the LOGSalesHistory trigger fires after each new row added to the 'Sales'. It takes corresponding information from the 'inserted' table and inserts it into 'SalesHistory', adding the current date and time for the 'AuditDate' column.
Creating Triggers in SQL After UPDATE Example
In this example, we handle an After UPDATE trigger to track changes made to the 'Employee' table. Whenever an employee's salary is updated, the trigger should record the event in the 'SalaryHistory' table, storing old and new salary details for the affected employee.
First, let's create the 'Employee' and 'SalaryHistory' tables:
CREATE TABLE Employee ( EmployeeID INT PRIMARY KEY, FirstName VARCHAR(100), LastName VARCHAR(100), Salary DECIMAL(10, 2) ); CREATE TABLE SalaryHistory ( HistoryID INT PRIMARY KEY IDENTITY(1,1), EmployeeID INT, OldSalary DECIMAL(10, 2), NewSalary DECIMAL(10, 2), UpdateDate DATETIME );
Next, we will create the After UPDATE trigger 'LogSalaryHistory' which records updates in the 'Employee' table:
CREATE TRIGGER LogSalaryHistory ON Employee AFTER UPDATE AS BEGIN IF UPDATE(Salary) BEGIN INSERT INTO SalaryHistory (EmployeeID, OldSalary, NewSalary, UpdateDate) SELECT i.EmployeeID, d.Salary, i.Salary, GETDATE() FROM inserted i INNER JOIN deleted d ON i.EmployeeID = d.EmployeeID END END;
The 'LogSalaryHistory' trigger only fires when the 'Salary' column is updated. It captures information about the updated employee from both the 'inserted' and 'deleted' tables, retrieving the new and old salary values. Finally, it inserts the data into 'SalaryHistory', recording the update date and time.
Create Trigger in SQL Oracle: Sample Code and Use Case
Creating triggers in Oracle Database involves similar principles as other SQL databases. However, Oracle syntax has slight differences, and variables specific to Oracle must be considered. To demonstrate, let's create an After UPDATE trigger in Oracle that logs changes made to the 'Order' table, recording them in an 'OrderHistory' table.
First, we create the 'Order' and 'OrderHistory' tables:
CREATE TABLE "Order" ( OrderID NUMBER PRIMARY KEY, CustomerID NUMBER, OrderDate DATE ); CREATE TABLE OrderHistory ( HistoryID NUMBER PRIMARY KEY, OrderID NUMBER, OldOrderDate DATE, NewOrderDate DATE, UpdateDate DATE );
Next, we create a sequence for the 'HistoryID', since Oracle doesn't support IDENTITY columns:
CREATE SEQUENCE OrderHistorySeq START WITH 1 INCREMENT BY 1;
Finally, we create the After UPDATE trigger 'LogOrderHistory' for the 'Order' table:
CREATE OR REPLACE TRIGGER LogOrderHistory AFTER UPDATE ON "Order" FOR EACH ROW WHEN (NEW.OrderDate != OLD.OrderDate) DECLARE v_historyId NUMBER; BEGIN SELECT OrderHistorySeq.NEXTVAL INTO v_historyId FROM dual; INSERT INTO OrderHistory (HistoryID, OrderID, OldOrderDate, NewOrderDate, UpdateDate) VALUES (v_historyId, :NEW.OrderID, :OLD.OrderDate, :NEW.OrderDate, SYSDATE); END; /
The 'LogOrderHistory' trigger fires when the 'OrderDate' column is updated. It checks for differences between the new and old values using the 'NEW' and 'OLD' references. A new 'HistoryID' is generated using the sequence, and the updated information is inserted into 'OrderHistory' as a new row, along with the current date.
Creating Triggers in SQL - Key takeaways
Creating Triggers in SQL: Automated actions in response to events like inserting, updating, or deleting data.
Trigger Types: After INSERT and After UPDATE help maintain data integrity and automate processes.
Trigger Syntax: CREATE TRIGGER trigger_name ON table_name FOR|AFTER|INSTEAD OF event_name AS BEGIN [action] END.
Best Practices: Keep triggers small and focused, use informative names, test and document your triggers, and manage errors efficiently.
Create Trigger in SQL Oracle: Similar to other SQL databases with slight syntax differences and specific Oracle variables.
Learn faster with the 15 flashcards about Creating Triggers in SQL
Sign up for free to gain access to all our flashcards.
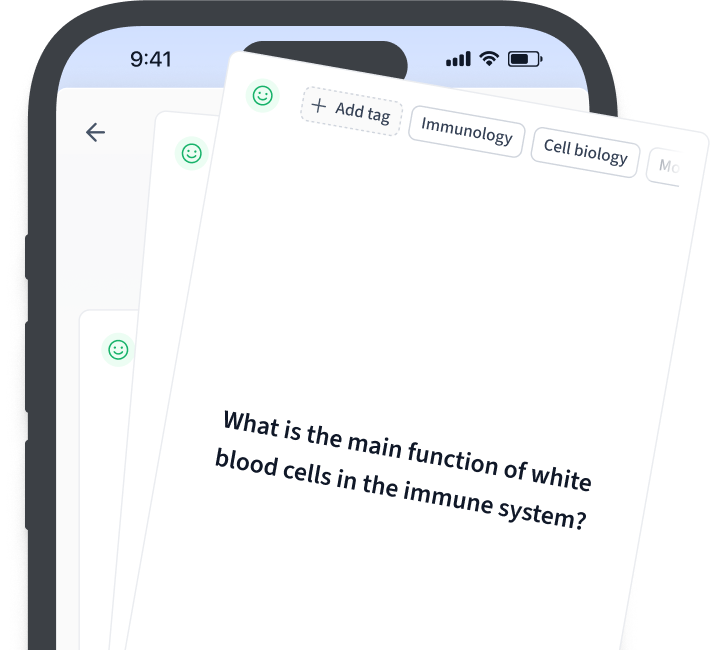
Frequently Asked Questions about Creating Triggers in SQL
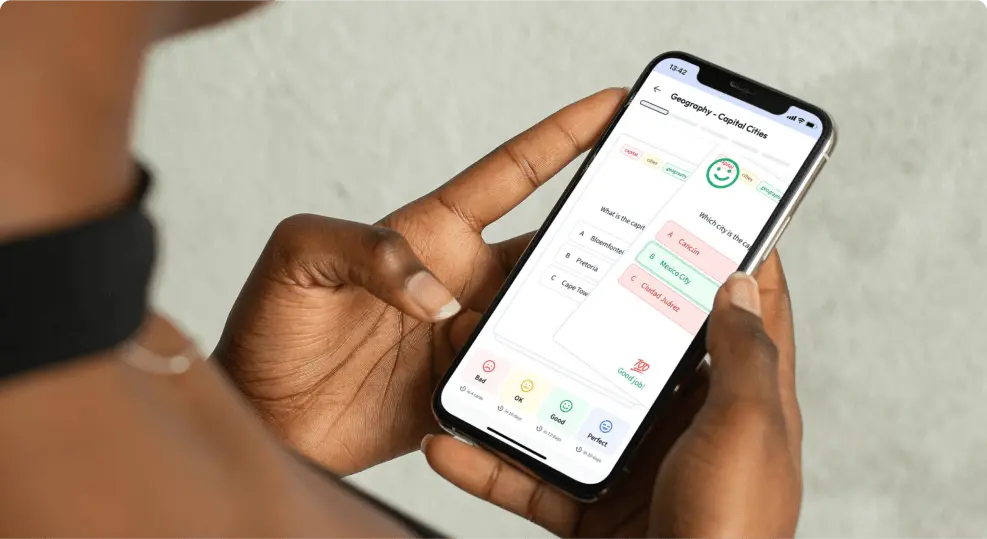
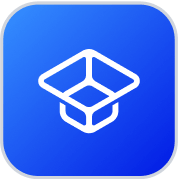
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more