Jump to a key chapter
Delete Trigger SQL: Explained
In SQL, a trigger is a special type of stored procedure that is automatically executed in response to specific events such as data modification operations. These events include INSERT, UPDATE, and DELETE queries executed on a specified table or columns.
A Delete Trigger is a type of SQL trigger that is specifically invoked when a DELETE query is executed on a particular table or column. It can be used to enforce data integrity, maintain history records, and automate actions based on data deletion.
Typically, DELETE triggers are defined using the CREATE TRIGGER statement, which consists of several clauses to specify the trigger's timing, events, and actions. An example of a DELETE trigger creation is as follows:
CREATE TRIGGER trigger_name
AFTER DELETE
ON table_name FOR EACH ROW
BEGIN
-- Trigger actions
END;
Triggers help in keeping the data consistent and maintain the desired state of the database. They perform actions such as:
- Validating input data
- Maintaining referential integrity
- Recording audit information
- Cascading actions to related tables
Types of SQL Triggers: An Overview
SQL triggers can be classified based on their activation time and the specific event they are set to respond to. Here are the main categories of SQL triggers:
1. Based on Activation Time
Triggers can be classified into two types based on their activation time:
Before Trigger | These triggers are activated before the specified data modification event. They are useful for validating data, enforcing business rules, and performing data transformations before the data is inserted, updated, or deleted. |
After Trigger | These triggers are activated after the specified data modification event. They are typically used for maintaining the history records, updating other tables, or notifying other users or systems about the changes. |
2. Based on Event Type
Triggers can also be classified into three main types based on the specific event they are set to respond to:
Insert Trigger | These triggers are activated when an INSERT query is executed on the specified table or column. They can be used to enforce constraints, add default values, and maintain the auto-generated fields. |
Update Trigger | These triggers are activated when an UPDATE query is executed on the specified table or column. They can be used to validate the updated data, enforce business rules, maintain history records, and update dependent columns or tables. |
Delete Trigger | These triggers are activated when a DELETE query is executed on the specified table or column. They can be used to enforce referential integrity, maintain history records, and perform cascading actions on related tables. |
In conclusion, understanding DELETE triggers in SQL is crucial for maintaining data consistency and automating actions based on data deletion events. With proper usage, triggers can greatly enhance database management and application performance.
How to Create a Delete Trigger in SQL Server
Before creating a delete trigger in SQL Server, it is essential to familiarise yourself with the necessary prerequisites. Meeting these requirements ensures a smooth and error-free process while working with delete triggers.
Here are the key prerequisites for creating a delete trigger in SQL Server:
- SQL Server installation: Ensure that you have SQL Server installed on your system. You can use any edition of SQL Server, such as Express, Standard, or Enterprise, based on your requirements and resources.
- SQL Server Management Studio (SSMS): You need a reliable and effective tool to manage your SQL Server database, and SQL Server Management Studio is the most popular choice for this purpose. Install the latest version of SSMS for optimal functionality.
- Database: You should have an existing database on your SQL Server that you want to create the delete trigger on.
- Permission: The account you are using to create the delete trigger must have ALTER permissions on the table or columns on which the trigger will be defined. Acting with the proper permissions ensures that you can create and modify triggers without encountering any issues.
Step by Step Guide: Create Delete Trigger in SQL Server
In this comprehensive step-by-step guide, we will thoroughly explore how to create a delete trigger in SQL Server. The procedure is outlined below:
- Open SQL Server Management Studio: Launch SSMS and connect to your SQL Server instance using your credentials.
- Select the database: In the Object Explorer pane, expand the 'Databases' folder and locate the desired database you want to create the delete trigger on.
- Create a new query: Right-click on the target database, choose 'New Query' from the context menu, and a new query editor window will open.
- Write the CREATE TRIGGER statement: Begin the process of creating the trigger by writing the CREATE TRIGGER statement in the query editor. The basic syntax for creating a delete trigger is as follows:
CREATE TRIGGER trigger_name
ON table_name
AFTER DELETE
AS
BEGIN
-- Trigger actions
END;
Here, replace 'trigger_name' with a meaningful name for your delete trigger and 'table_name' with the name of the table on which you want to define the trigger. After the DELETE statement, write the trigger action(s) you want to perform in the BEGIN...END block.
- Execute the query: After writing the CREATE TRIGGER statement, click the 'Execute' button or use the shortcut key 'F5' to execute the query. If the query is successful, a message will appear in the 'Messages' tab confirming the trigger's creation.
- Verify the trigger: To ensure that the delete trigger has been created successfully, expand the 'Tables' folder in the database and locate the target table. Within the table, go to the 'Triggers' folder, and you should see your newly created delete trigger listed.
- Test the trigger: To test the delete trigger, perform a DELETE operation on the specified table or column. Verify that the trigger is executing the desired actions upon the deletion event. In case of any issues, make sure to debug and modify your trigger code accordingly.
By following this comprehensive guide, you can successfully create and implement a delete trigger in SQL Server. Ensure that you adhere to the prerequisites and follow each step closely to achieve the desired functionality and maintain data integrity within your database.
SQL Delete Trigger Examples and Scenarios
In this section, we will examine a basic example of a SQL DELETE trigger and delve into the various components of the trigger syntax. This example demonstrates how we can protect referential integrity when deleting data from a primary table by using a delete trigger to remove related entries in a foreign table.
Consider the following main tables:
Customers: | CustomerID (Primary Key), CustomerName, ContactDetails |
Orders: | OrderID (Primary Key), CustomerID (Foreign Key), ProductDetails, OrderDate |
If a customer's record is deleted from the Customers table, we need to ensure that their related orders are also removed from the Orders table. This can be achieved by implementing a delete trigger. Here's the basic syntax for our example:
CREATE TRIGGER delete_orders_trigger
ON Customers
AFTER DELETE
AS
BEGIN
DELETE FROM Orders
WHERE CustomerID IN (SELECT CustomerID FROM deleted);
END;
In the above example:
- 'delete_orders_trigger' is the name assigned to the delete trigger.
- 'Customers' is the primary table which we define the trigger on.
- 'AFTER DELETE' refers to the event during which the trigger will execute.
- 'AS' separates the trigger header from the trigger body.
- The query within the BEGIN...END block will be executed as the action that the trigger initiates when the specified DELETE event occurs.
- 'deleted' is a temporary table created internally within the delete trigger that holds the rows removed from the Customers table. It helps to retrieve the CustomerID values of the deleted rows to delete the corresponding orders from the Orders table.
Real-Life Scenarios: Implementing Delete Trigger SQL Query
Now let's discuss some real-life scenarios where DELETE triggers can be helpful in maintaining data integrity and implementing automated actions. In each case, we will provide a brief overview of the situation, followed by a detailed explanation of the delete trigger implementation.
Scenario 1: Archiving Deleted Records
In an online shopping platform, when products are removed from the inventory, a record of the deleted products needs to be maintained for future reference and auditing purposes. To achieve this, a delete trigger can be implemented to automatically copy the deleted records into an archive table before their removal from the main Products table.
Consider the following tables:
Products: | ProductID (Primary Key), ProductName, Category, Price, Stock |
Archived_Products: | ProductID (Primary Key), ProductName, Category, Price, Stock, DeletionDate |
Here is an example of a delete trigger that archives the deleted records:
CREATE TRIGGER archive_products_trigger
ON Products
AFTER DELETE
AS
BEGIN
INSERT INTO Archived_Products (ProductID, ProductName, Category, Price, Stock, DeletionDate)
SELECT ProductID, ProductName, Category, Price, Stock, GETDATE()
FROM deleted;
END;
Scenario 2: Enforcing Referential Integrity with Cascading Deletes
In an organisation's HR system, if an employee's record is deleted, all of the employee's related records such as address, performance reviews, and payroll records must also be deleted to maintain consistency in the database.
Consider the following tables:
Employees: | EmployeeID (Primary Key), FirstName, LastName, Position, Department |
Addresses: | AddressID (Primary Key), EmployeeID (Foreign Key), Street, City, PostalCode |
Performance_Reviews: | ReviewID (Primary Key), EmployeeID (Foreign Key), ReviewDate, Rating, Comments |
The delete trigger for cascading deletes can be implemented as follows:
CREATE TRIGGER cascade_employees_deletion_trigger
ON Employees
AFTER DELETE
AS
BEGIN
DELETE FROM Addresses
WHERE EmployeeID IN (SELECT EmployeeID FROM deleted);
DELETE FROM Performance_Reviews
WHERE EmployeeID IN (SELECT EmployeeID FROM deleted);
END;
By applying DELETE triggers in real-life scenarios like these, database management can be streamlined, ensuring data consistency and integrity, as well as automating essential tasks upon data deletion events.
Managing Delete Triggers in SQL Server
Managing delete triggers in SQL Server involves various actions, such as modification, updates, and removal of triggers based on the requirements and objectives of your database management strategy. This section provides a comprehensive insight into these aspects, along with best practices for database administrators to follow.
Modifying and Updating Delete Trigger SQL Server
Over time, it may be necessary to modify or update delete triggers in order to accommodate changes in the database or business rules. In SQL Server, there are multiple ways to modify and update delete triggers, with ALTER TRIGGER being the primary method. Here's a detailed look at modifying and updating delete triggers in SQL Server:
- Using ALTER TRIGGER: The ALTER TRIGGER statement can be used to modify the code inside an existing trigger. The syntax for modifying a delete trigger is similar to the CREATE TRIGGER statement, but with ALTER TRIGGER replacing CREATE TRIGGER. You can make required changes to the trigger actions or conditions within the BEGIN...END block. The basic syntax for the ALTER TRIGGER statement is as follows:
ALTER TRIGGER trigger_name
ON table_name
AFTER DELETE
AS
BEGIN
-- Updated Trigger actions
END;
In addition to using the ALTER TRIGGER statement, other methods and tools can also be employed to modify and update delete triggers in SQL Server:
- Using SQL Server Management Studio (SSMS): SSMS provides a graphical user interface to view and edit triggers. Locate the desired trigger within the Triggers folder of the respective table, right-click it and select 'Modify'. Make the necessary changes within the editor and click 'Execute', or press F5, to save the changes.
- Using Visual Studio: In Visual Studio, you can modify and update triggers using the SQL Server Data Tools (SSDT). Open your database project, locate the desired trigger, and modify it using the code editor. Finally, save and deploy the changes to apply them.
Removing Delete Triggers: Best Practices
There may come a time when a delete trigger is no longer needed or needs to be replaced with a more efficient solution. In such cases, the removal of the trigger becomes necessary. However, it is crucial to follow some best practices when dealing with the removal of delete triggers in SQL Server:
- Using DROP TRIGGER statement: To safely remove a delete trigger in SQL Server, the DROP TRIGGER statement should be employed. The syntax is simple and straightforward:
DROP TRIGGER trigger_name;
Make sure to replace 'trigger_name' with the actual name of the trigger you want to remove.
- Backup before removal: Before removing a delete trigger, ensure that you have a backup of the trigger code. This is essential in case you need to restore the trigger later or use the code as a reference for future triggers. Back up the trigger code using any of the following methods:
- Copy and paste the trigger code into a text file or a documentation tool of your choice.
- Export the trigger definition using SSMS or SQL Server Data Tools (SSDT) in Visual Studio.
- Test the impact of trigger removal: Before permanently removing a delete trigger, it is crucial to test and verify that its removal will not negatively impact the database or violate data integrity constraints. You can perform the following checks for this purpose:
- Ensure that no other tables or stored procedures are dependent on the trigger.
- Test the affected DELETE operations on the table and confirm that the trigger's absence does not lead to inconsistent or incorrect data.
By following these best practices, you can manage delete triggers in SQL Server efficiently, ensuring the integrity, performance, and maintainability of your database system. Remember to always exercise caution while modifying, updating or removing delete triggers, and consider the impact on your database before making any changes.
Delete Trigger SQL - Key takeaways
Delete Trigger SQL: A type of SQL trigger that activates when a DELETE query is executed on a table or column, used for enforcing data integrity and automating actions.
Types of SQL Triggers: Divided into categories based on activation time (Before Trigger and After Trigger) and event type (Insert, Update, and Delete Triggers).
Create Delete Trigger in SQL Server: A step-by-step guide to creating a delete trigger using the CREATE TRIGGER statement, followed by executing and verifying the trigger.
SQL Delete Trigger Example: An example demonstrating the basic syntax and usage of a delete trigger, focusing on maintaining referential integrity and archiving deleted records.
Managing Delete Triggers: Best practices for modifying, updating, and removing delete triggers in SQL Server, including using ALTER TRIGGER, DROP TRIGGER, and testing the impact of trigger removal.
Learn faster with the 16 flashcards about Delete Trigger SQL
Sign up for free to gain access to all our flashcards.
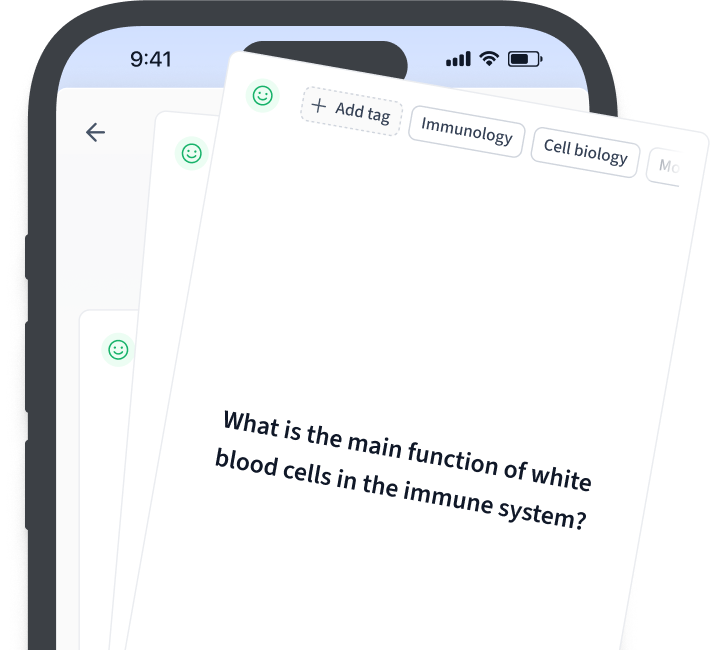
Frequently Asked Questions about Delete Trigger SQL
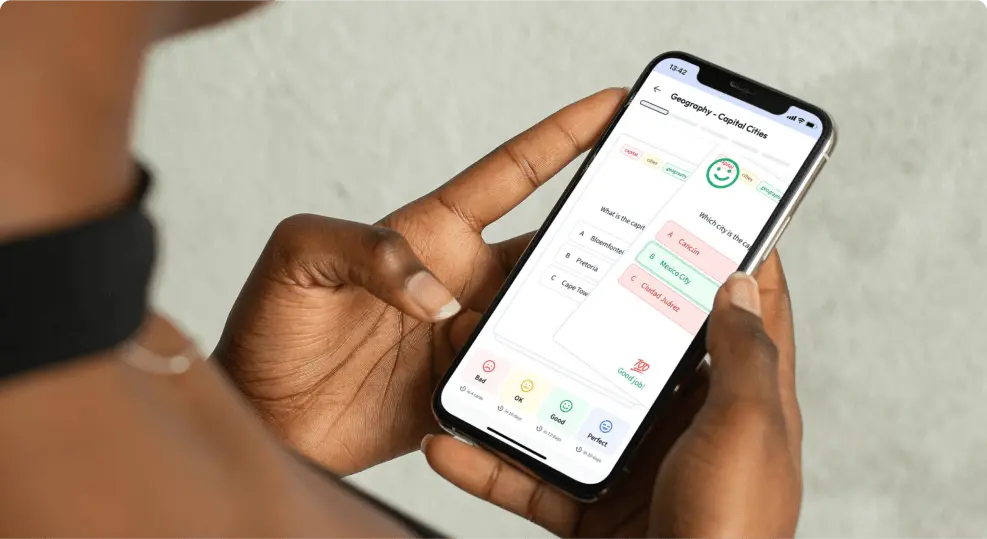
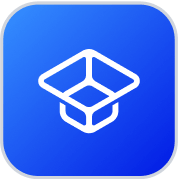
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more