Jump to a key chapter
Types of Integrity Constraints in SQL
Integrity Constraints are an essential part of SQL databases, as they ensure the accuracy and consistency of data stored. They define rules for maintaining data integrity and protect the database from data issues.
There are several types of Integrity Constraints in SQL, each one serving a particular purpose. By understanding these constraints, you can build reliable and efficient databases. Let's dive deeper into these constraint types.
Domain Integrity Constraints in SQL
Domain Integrity Constraints define the permissible values for a given column. By applying these constraints, you can restrict the data entered into a specific column, ensuring consistent data values across your database.
Some commonly used domain integrity constraints include:
- Data type – The column must contain values of a specific data type
- Data format – The format of the values in a column must follow a defined pattern
- Range – The values must fall within a specified range
- Enumeration – The values in the column can only be taken from a predefined set of values
For example, if you have a table containing information about employees' salaries, you might enforce a domain integrity constraint on the "salary" column to ensure that only numeric values within a specific range are entered.
Entity Integrity Constraints in SQL
Entity Integrity Constraints involve uniquely identifying the rows in a database table, such that there are no duplicate or null values in a primary key column. A primary key is a unique column in a table that uniquely identifies every row in the table. This constraint helps maintain the uniqueness and integrity of data by preventing the existence of duplicate rows.
For instance, in a table storing customer information, a unique identification number (‘customer_id’) can be assigned as the primary key to uniquely identify every customer.
Referential Integrity Constraint in SQL
Referential Integrity Constraint ensures that relationships between tables are maintained consistently. It is enforced by using foreign keys, which are columns in a table that refer to a primary key in another table. The foreign key helps to maintain the referential integrity between two related tables by making sure that changes in one table's primary key are reflected in the corresponding foreign key in another table.
There are two main rules to uphold when it comes to Referential Integrity Constraints:
- If a primary key value is updated or deleted, the corresponding foreign key values in the related table must be updated or deleted as well.
- Any new foreign key value added to the related table must have a corresponding primary key value in the other table.
For example, imagine a database with two tables: ‘Customers’ and ‘Orders’. The ‘customer_id’ field in the ‘Customers’ table is the primary key, while the same field in the ‘Orders’ table is a foreign key. If a customer is deleted from the ‘Customers’ table, the Referential Integrity Constraint would ensure that all the associated orders are deleted as well. Similarly, if an order is added to the ‘Orders’ table, the constraint would require that the associated customer exists in the ‘Customers’ table.
Implementing Complex Integrity Constraints in SQL
Complex Integrity Constraints in SQL involve implementing custom constraints and using triggers for specific scenarios. These constraints enable the database to handle more complex data requirements than the standard constraints allow. Implementing complex constraints allows you to maintain data integrity, consistency, and accuracy in the database effectively while addressing specific business needs.
Creating Custom Constraints
Creating Custom Constraints involves defining rules based on specific requirements that cannot be addressed using the standard predefined constraints. These constraints help to maintain the data validity and consistency according to the specific business logic. Custom constraints can be implemented using SQL CHECK constraints.
Here are a few essential aspects to consider when creating custom constraints:
- Custom constraints must be based on columns within the same table.
- The constraint must consist of a logical expression, not a SQL query.
- The constraint must be verified and maintained during all INSERT, UPDATE and DELETE operations on the table.
To create a custom constraint in SQL, you can use the CHECK keyword followed by the desired constraint condition.
For instance, if you have a table named ‘Employees’ with a ‘salary’ and ‘join_date’ column, you can create a custom constraint ensuring that employees hired after a certain date have a minimum salary requirement:
CREATE TABLE Employees (
employee_id INT PRIMARY KEY,
salary NUMERIC(10,2),
join_date DATE,
CHECK (join_date > '2000-01-01' AND salary >= 20000)
);
By creating custom constraints, you can maintain data integrity and enforce specific business rules that are not achievable using standard SQL constraints.
Using Triggers for Complex Constraints
Triggers are a powerful tool in SQL that facilitate the implementation of complex integrity constraints. SQL triggers are attached to specific events such as INSERT, UPDATE, DELETE or even multiple events on specific tables or views. Triggers are automatically invoked when the defined events occur, making it possible to verify constraints and apply alterations automatically.
When you need to apply complex constraints that involve multiple tables or complex calculations, triggers become a suitable method to maintain data integrity.
Triggers can be classified into two types:
- Before Triggers – These triggers are executed before the specified event. They are useful for maintaining complex constraints by validating and manipulating data before it is permanently stored in the database.
- After Triggers – These triggers are executed after the specified event. They can be used for maintaining data integrity by performing additional actions like updating other tables or generating log entries for maintaining an audit trail.
Here is an example of a trigger that enforces a complex constraint:
Suppose you have two tables, 'Projects' and 'Tasks', and you want to ensure that a project's budget is not exceeded when adding new tasks. You can create an AFTER INSERT trigger on the 'Tasks' table that would check if the total estimated cost of all tasks related to a project exceeds the project's budget and rollback the transaction if the constraint is violated:
CREATE TRIGGER check_project_budget
AFTER INSERT ON tasks
FOR EACH ROW
BEGIN
DECLARE project_total_cost NUMERIC(10,2);
DECLARE project_budget NUMERIC(10,2);
SELECT SUM(estimated_cost)
INTO project_total_cost
FROM tasks
WHERE project_id = NEW.project_id;
SELECT budget
INTO project_budget
FROM projects
WHERE project_id = NEW.project_id;
IF project_total_cost > project_budget THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Project budget exceeded!';
END IF;
END;
In summary, using triggers allows you to maintain complex constraints involving multiple tables and calculations, ensuring data integrity, consistency, and adherence to business rules. By understanding when and how to use custom constraints and triggers, you can build a robust and optimized database for your specific needs.
Ensuring Data Consistency with Integrity Constraints in SQL
Integrity constraints play a vital role in ensuring data consistency within SQL databases by enforcing rules to maintain data integrity, uniqueness, and referential relationships across tables. This section will delve deep into the aspects of altering and dropping constraints and maintaining referential integrity during updates and deletes.
Altering and Dropping Constraints
When dealing with an evolving database, you might need to modify or remove constraints to accommodate changes in the data structure or business requirements. In SQL, you can easily do this by using the ALTER TABLE statement to alter or drop constraints.
However, when altering or dropping constraints, it's essential to follow certain methods and best practices to avoid compromising your data's integrity and consistency. Let us explore how to perform these actions safely and correctly with the following steps:
- Identify the constraint to be altered or dropped - You can usually find this information in the table schema or by querying the INFORMATION_SCHEMA.
- Ensure data integrity - Before altering or removing a constraint, make sure the existing data adheres to the new constraint requirements, or address any potential conflicts if the constraint is dropped.
- Perform the alteration or dropping using the ALTER TABLE statement - Issue the appropriate SQL commands to apply the changes to the constraints as needed.
- Verify the changes - Confirm that the constraints have been altered or dropped successfully by examining the updated table schema or running test queries.
Consider the following example: You have an 'Employees' table with a constraint on the 'salary' column to ensure that the salary value is greater than or equal to 15,000. If you need to update this constraint to set the minimum salary value to 18,000, you can follow these steps:
- Identify the constraint:
SELECT * FROM INFORMATION_SCHEMA.TABLE_CONSTRAINTS WHERE TABLE_NAME = 'Employees';
- Ensure that all existing salary values are equal to or greater than 18,000.
- Perform the alteration using the ALTER TABLE statement:
ALTER TABLE Employees DROP CONSTRAINT salary_constraint; ALTER TABLE Employees ADD CONSTRAINT salary_constraint CHECK (salary >= 18000);
- Verify the changes by checking the table schema and running test queries.
Maintaining Referential Integrity during Updates and Deletes
Referential integrity, as mentioned previously, is essential for preserving the consistency and reliability of the relationships between tables in your database. Updates and deletes on the primary key or foreign key columns can potentially break referential integrity if not handled carefully. Therefore, it's crucial to address the impact of these operations on related tables.
In SQL, you can use referential actions to maintain referential integrity during updates and deletes. These actions define how the changes in one table are propagated to related tables. The main referential actions are:
- CASCADE - This action automatically updates or deletes the corresponding foreign key rows in the related table when the primary key in the parent table is updated or deleted.
- SET NULL - If the primary key is updated or deleted, this action sets the corresponding foreign key values in the related table to NULL.
- SET DEFAULT - This action sets the foreign key values in the related table to their default values when the primary key in the parent table is updated or deleted.
- NO ACTION - No action is taken on the related table when the primary key is updated or deleted in the parent table. However, the SQL standard requires that the primary key update or deletion must be rejected if it violates the referential integrity constraint.
To maintain referential integrity, you can choose one of the above actions that best suits your specific data requirements. Define these actions using the FOREIGN KEY constraint definition in the CREATE TABLE or ALTER TABLE statement.
For example, imagine a database with a 'Customers' table and an 'Orders' table, where the 'customer_id' column in the 'Orders' table is a foreign key referring to the 'customer_id' primary key in the 'Customers' table. You can use the referential action CASCADE to ensure that any update or deletion in the 'Customers' primary key is automatically reflected in the 'Orders' foreign key. This can be done as follows:
CREATE TABLE Orders (
order_id INT PRIMARY KEY,
customer_id INT,
product_id INT,
quantity INT,
FOREIGN KEY (customer_id) REFERENCES Customers(customer_id) ON UPDATE CASCADE ON DELETE CASCADE
);
By following these guidelines, you can effectively maintain referential integrity during updates and deletes and ensure data quality and consistency in your SQL database.
Integrity Constraints in SQL - Key takeaways
Integrity Constraints in SQL: Ensure consistency, accuracy, and reliability of data in databases.
Domain Integrity Constraints: Restrict permissible values in a column to maintain data consistency.
Entity Integrity Constraints: Enforce uniqueness of primary key column in a table to avoid duplicate or null values.
Referential Integrity Constraint: Maintain consistency in relationships between tables using primary and foreign keys.
Complex Integrity Constraints in SQL: Creating custom constraints and using triggers for specific scenarios to handle complex data requirements.
Learn faster with the 15 flashcards about Integrity Constraints in SQL
Sign up for free to gain access to all our flashcards.
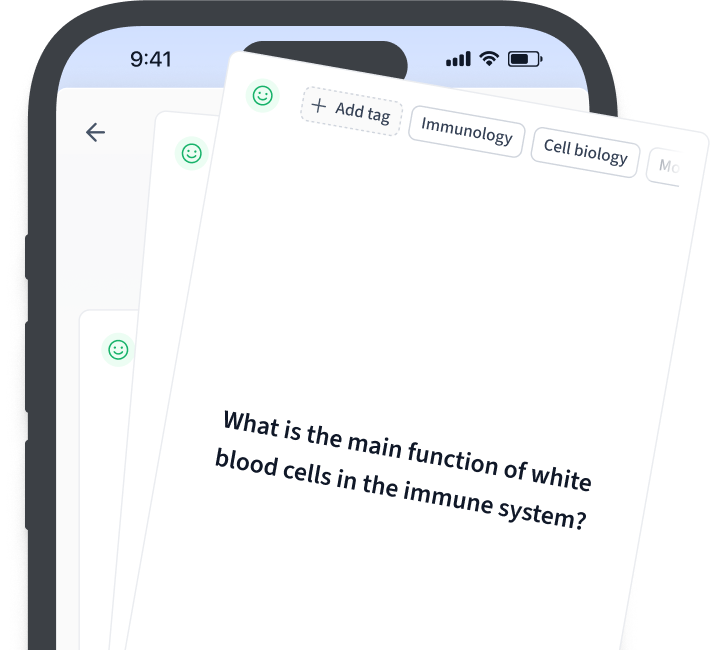
Frequently Asked Questions about Integrity Constraints in SQL
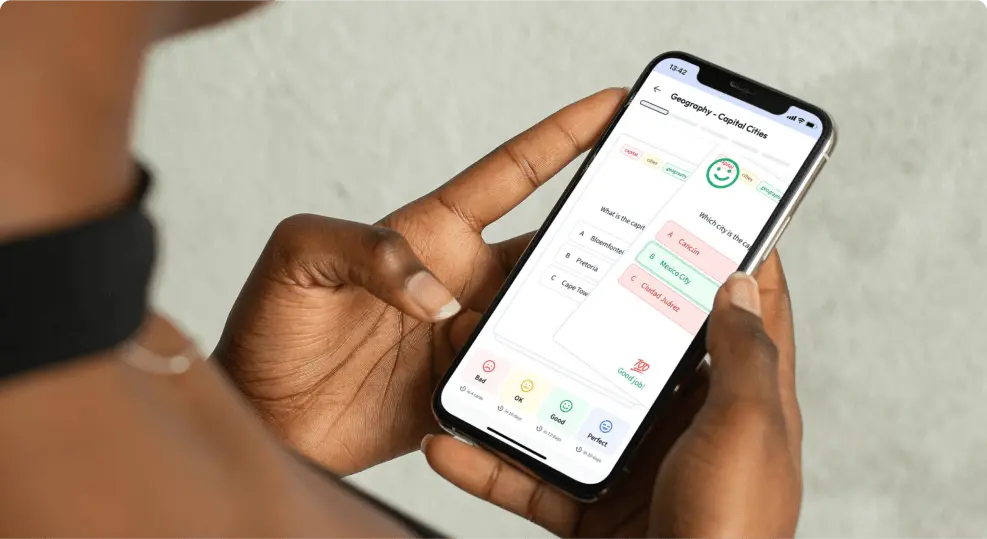
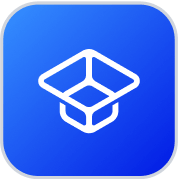
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more