Jump to a key chapter
SQL DELETE Explained: A Comprehensive Guide
SQL DELETE is a statement in Structured Query Language (SQL) that is used to remove one or more records (rows) from a table in a database. It is a part of Data Manipulation Language (DML) operations, which also include SELECT, INSERT, and UPDATE statements.
For example, imagine that you have a table called 'students' containing information about different students, and you want to delete all the records of students who have graduated. You can execute the DELETE statement with the appropriate WHERE clause to remove these rows.
Syntax and Basic Execution
To understand SQL DELETE better, let's start with its syntax. The basic syntax for the DELETE statement is as follows:DELETE FROM table_name WHERE condition;Here's a breakdown of the main components in the SQL DELETE statement:
- DELETE FROM: This is the keyword that initiates the DELETE operation.
- table_name: This refers to the name of the table from which you want to delete rows.
- WHERE: This is an optional keyword that specifies the condition that must be satisfied for a row to be deleted.
- condition: This expression defines the criteria that a row must meet to be deleted. If no condition is provided, all rows in the table will be removed.
Common Applications and Use Cases
SQL DELETE is often used in various scenarios to manage and maintain the data in a database effectively. Here are some common applications and use cases for using the DELETE statement: 1. Cleaning up outdated data: Over time, some data in the database may become outdated and irrelevant. The DELETE statement allows you to remove such data to free up storage space and keep the database optimized. 2. Removing duplicate records: Occasionally, a database might contain duplicate rows due to data entry errors or other issues. The DELETE statement can be used alongside SELECT DISTINCT to identify and remove these duplicates. 3. Data archiving: In some cases, you might need to archive data from a specific table by moving the rows to another table before deleting them. The DELETE statement is used to remove rows from the original table once they have been copied over. 4. Maintaining referential integrity:If your database has foreign key constraints, you might need to delete related rows in other tables before removing the primary record to ensure referential integrity. The DELETE statement can be utilized to achieve this. Remember that when using SQL DELETE, you must always exercise caution as the deletion operation is permanent, and any deleted data cannot be easily recovered. Make sure to properly use the WHERE clause to specify conditions and test your DELETE statement before executing it on production data.Different Types of SQL DELETE Statements
In this section, we will discuss the various types of SQL DELETE statements and delve into their specific applications and use cases. By understanding these various forms, you can execute SQL DELETE statements more efficiently and effectively in different scenarios.Simple Delete
The simplest form of the SQL DELETE statement targets a specific table and removes all rows contained within it. This type of query does not include any condition to filter rows and should be used cautiously, as it will remove everything from the table.
DELETE FROM table_name;Here, 'table_name' refers to the table you want to perform the delete operation on. The absence of a WHERE clause means that every row in the table will be deleted, effectively emptying the table.
Conditional Delete
In most real-world scenarios, you will need to delete specific rows from a table based on a condition. To accomplish this, you can use the WHERE clause in conjunction with the SQL DELETE statement. The WHERE clause allows you to define the condition that must be met for a row to be selected for deletion.DELETE FROM table_name WHERE condition;The 'condition' expression can include various logical operators, comparison operators, and columns in the table. Here are some examples of conditional delete statements: 1. Deleting rows where a specific column value equals a certain value:
DELETE FROM students WHERE grade = 'F';2. Deleting rows where a specific column value is within a range:
DELETE FROM orders WHERE order_date BETWEEN '2021-01-01' AND '2021-06-30';3. Deleting rows where a specific column has a value in a given list:
DELETE FROM employees WHERE department_id IN (1, 3, 5, 7);
Truncate: A Special Case
While not strictly an SQL DELETE statement, it's worth mentioning the TRUNCATE TABLE command, which serves a similar purpose but operates differently. By using TRUNCATE TABLE, you can quickly delete all rows in a table but retain the table structure and schema. The TRUNCATE TABLE statement is commonly used when you want to efficiently remove all data from a table without affecting its structure.TRUNCATE TABLE table_name;The main differences between the TRUNCATE TABLE and a simple DELETE statement are that TRUNCATE TABLE is faster, cannot be rolled back, and does not preserve any row-level triggers.
SQL DELETE with Join: Combining Delete Operations
In some situations, you might need to delete rows from one table based on data in another table. To accomplish this, you can use SQL DELETE with a JOIN operation, effectively combining multiple tables to determine which rows should be deleted.Deleting Records Based on Relationships
By joining tables, you can reference data from other tables to determine whether a row in the main table should be deleted. Depending on the database management system (DBMS), the syntax for an SQL DELETE with JOIN statement may vary. However, the general concept remains the same. Here's an example of how to delete records based on a relationship between two tables:DELETE orders FROM orders JOIN customers ON orders.customer_id = customers.customer_id WHERE customers.country = 'United States';This statement deletes all rows in the 'orders' table where the related customer's country is 'United States'. Note that only the rows in the 'orders' table will be deleted, not the rows in the 'customers' table.
Best Practices and Tips
When using SQL DELETE with JOIN, it's essential to follow best practices to ensure efficiency and prevent data loss. Here are some tips to help you: 1. Always test your DELETE statement in a development or testing environment before executing it on production data. 2. Use the SELECT statement combined with your JOIN condition first to identify the rows to be deleted, allowing you to double-check the results before performing the actual deletion. 3. Be sure to consider any foreign key constraints and relations between tables before executing a DELETE with JOIN operation, ensuring referential integrity is maintained and preventing potential errors. 4. When working with large tables, consider breaking the delete operation into smaller, manageable chunks to reduce the impact on database performance and make it easier to monitor the execution progress.SQL DELETE Examples and Practice Exercises
In this section, we will go through multiple SQL DELETE examples and explore different scenarios in which you might need to use the DELETE statement. These examples will help you better understand how DELETE works and provide insight into various real-world situations.Removing Specific Records
One common scenario where SQL DELETE is used is to remove specific records from a table based on certain criteria. Let's say you have a 'product_reviews' table that contains user-submitted product reviews, and you want to delete a review with a particular 'review_id'. Here's how you could achieve this:
DELETE FROM product_reviews WHERE review_id = 42;In this example, the DELETE statement targets the 'product_reviews' table and removes the row that has a 'review_id' value of 42.
Deleting Based on Multiple Conditions
In some cases, you might need to delete multiple rows based on a combination of conditions. Consider that you have a 'sales_data' table containing information about sales transactions. You want to delete rows where the 'sales_amount' is less than £1000 and the 'transaction_date' is earlier than 1st January 2020:DELETE FROM sales_data WHERE sales_amount < 1000 AND transaction_date < '2020-01-01';Here, the DELETE statement uses two conditions in the WHERE clause: 'sales_amount' and 'transaction_date'. Both conditions must be satisfied for a row to be deleted.
SQL DELETE INTO: Cloning and Deleting Data
The SQL DELETE INTO operation is not a standard SQL operation; however, it is an essential concept to be aware of. The idea behind this operation is to clone data into a new table or an existing table and then delete the original data. It is useful in situations where you need to archive or backup data before deleting it from the main table. It involves using two separate statements: INSERT INTO and DELETE.Use Cases for Delete Into
There are several scenarios where you might use the DELETE INTO concept, such as: 1. Data archiving: You want to move older data from a primary table, such as 'orders', into an archive table like 'orders_archive'. After moving the data, you can safely delete it from the 'orders' table. 2. Data partitioning: You want to separate data into different tables based on specific criteria, such as dividing customers based on their countries into different regional tables. 3. Backup and purge:Performing a regular cleanup of large tables by copying data to a backup table and removing it from the main table to optimize performance and maintain compliance.Syntax and Execution Tips
To implement SQL DELETE INTO, you need to follow these steps: 1. Clone data into a new or existing table using the INSERT INTO statement. 2. Delete the original data from the main table using the DELETE statement. Here is an example of how to clone and delete data using DELETE INTO:-- Clone data into a new table (orders_archive): INSERT INTO orders_archive SELECT * FROM orders WHERE order_date < '2020-01-01'; -- Delete the original data from the main table (orders): DELETE FROM orders WHERE order_date < '2020-01-01';In this example, the orders older than 1st January 2020 are first copied into the 'orders_archive' table and then deleted from the 'orders' table. To ensure accuracy, it is important to use the same WHERE condition for both INSERT INTO and DELETE statements. When using DELETE INTO, follow these best practices: 1. Verify the cloned data in the new or existing table before deleting it from the main table. 2. Be cautious with large tables as deleting a significant amount of data may affect performance. Consider performing the operation in smaller batches. 3. Always test your DELETE INTO operation in a safe, development environment before executing it on production data.
SQL DELETE - Key takeaways
SQL DELETE: A statement in SQL used to remove one or more records from a table in a database.
SQL DELETE types: Simple Delete (no condition), Conditional Delete (with WHERE clause), and SQL DELETE with JOIN (based on data in another table).
SQL delete example:
DELETE FROM students WHERE grade = 'F';
SQL delete with join: Combine multiple tables using JOIN to determine which rows to delete from the main table.
SQL DELETE INTO: Clone data into a new or existing table and delete the original data, often used for data archiving or partitioning.
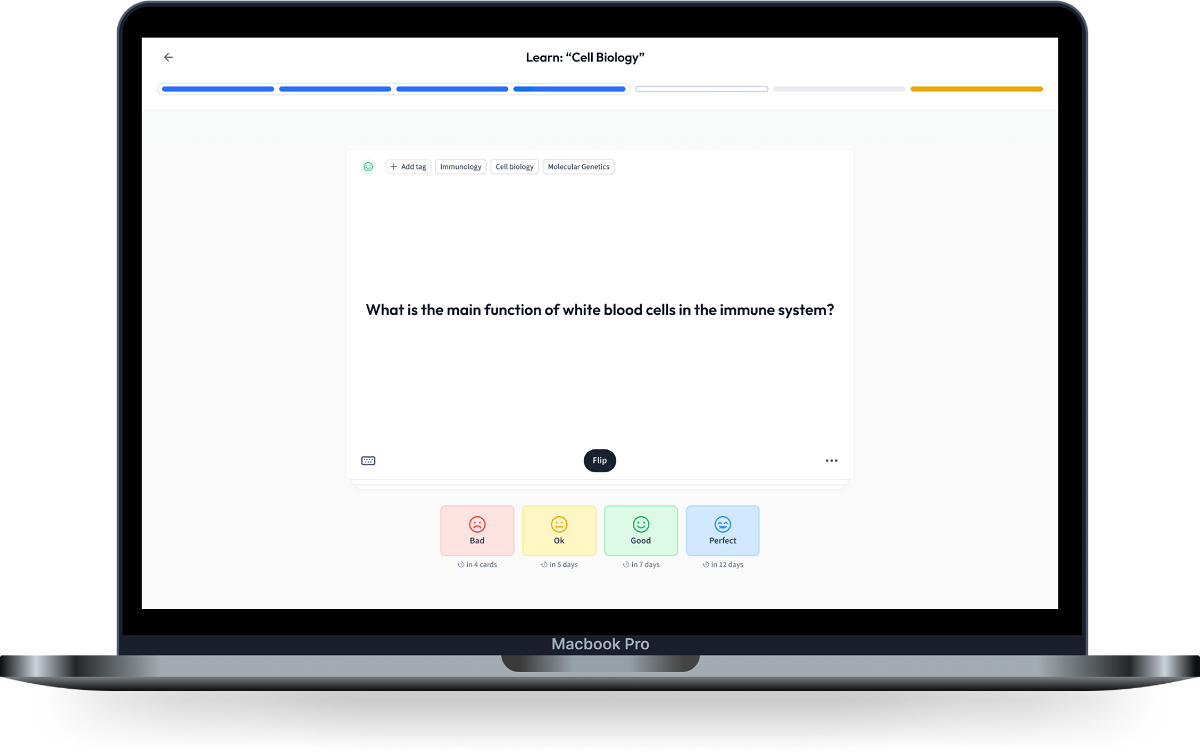
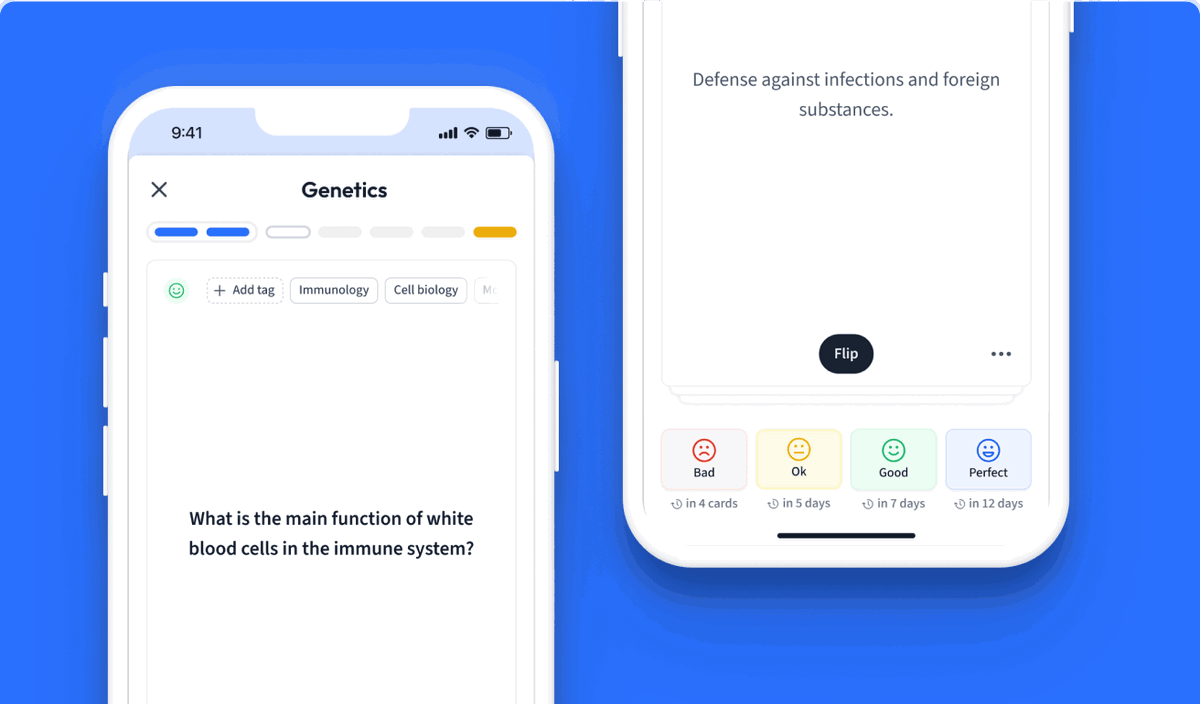
Learn with 19 SQL DELETE flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about SQL DELETE
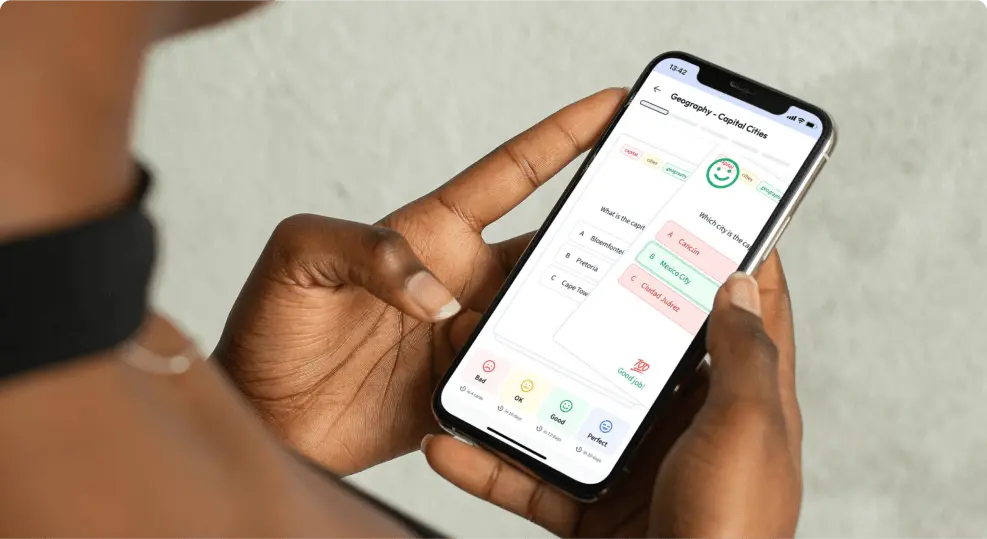
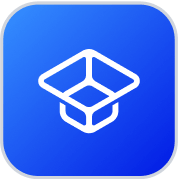
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more