Jump to a key chapter
Introduction to SQL Functions
SQL functions are essential building blocks in a Structured Query Language (SQL) database system. They are critical in managing and manipulating data stored in relational databases. SQL functions can be divided into two broad categories: aggregate and scalar functions. In this article, you will learn about various types of SQL functions and common examples to help you better understand their use in real-world applications.
Types of SQL Functions
As previously mentioned, SQL functions can be categorised into two types:
- Aggregate Functions: These functions are used to perform calculations on multiple rows of data, returning a single output value. They are commonly used for statistical analysis or to summarise data. Examples of aggregate functions in SQL include COUNT, SUM, AVG, MIN, and MAX.
- Scalar Functions: These functions operate on a single value and return a single value. Scalar functions can be further divided into categories based on their purpose, such as string functions, numeric functions, and date and time functions. Examples of scalar functions include UPPER, LOWER, ROUND, and NOW.
Common SQL Functions List
Here is a list of commonly used SQL functions, grouped by category:
Aggregate Functions:
- COUNT(): Returns the count of rows in a table or group.
- SUM(): Calculates the sum of values in a column.
- AVG(): Computes the average value for a specified column.
- MAX(): Retrieves the maximum value in a column.
- MIN(): Finds the minimum value in a column.
String Functions:
- UPPER(): Converts a string to uppercase.
- LOWER(): Transforms a string to lowercase.
- LEFT(): Extracts characters from the left of a string.
- RIGHT(): Extracts characters from the right of a string.
- LENGTH(): Determines the length of a string.
Numeric Functions:
- ABS(): Returns the absolute value of a number.
- ROUND(): Rounds a number to the nearest integer or specified number of decimal places.
- CEILING(): Returns the smallest integer greater than or equal to a number.
- FLOOR(): Finds the largest integer less than or equal to a number.
Date and Time Functions:
- NOW(): Displays the current date and time.
- DATE(): Extracts the date portion of a date or datetime value.
- TIME(): Retrieves the time portion of a date or datetime value.
- DATEDIFF(): Calculates the difference between two date values.
SQL Functions with Examples
Now that we have a list of common SQL functions, let's take a closer look at some examples to see how they can be used in real-world scenarios.
Example 1: Using COUNT() to determine the number of items in a table.
SELECT COUNT(*) FROM items;
This query will return the total number of rows in the "items" table.
Example 2: Using UPPER() and LOWER() to change the case of strings.
SELECT UPPER(name), LOWER(name) FROM items;
This query will return the "name" column in uppercase and lowercase for each row in the "items" table.
Remember that the table and column names used in the examples presented here may vary depending on your specific database schema. Always adjust the queries to match your table and column names.
As a computer science student, understanding SQL functions is fundamental to working with relational databases. By mastering the functions outlined in this article, you can significantly improve your ability to manipulate and analyse data efficiently in your database systems.
SQL Functions Explained
As a thorough examination of SQL functions, it is now necessary to have an in-depth exploration of their significance by focusing on aggregate and scalar functions. Details are provided regarding the purpose and usage of these functions, along with beneficial examples.
Aggregate Functions in SQL
Aggregate functions in SQL are vital for processing and analysing data on multiple rows, often used for tasks such as summarising or statistical analysis. These functions typically perform calculations on a specific column, taking into account multiple rows within that column, and produce a single resulting value as the output. Here is a closer examination of the most common aggregate functions:
- COUNT: The COUNT function is designed to return the count of rows in the specified column, or simply the total number of rows in a group. It is frequently used to determine the size of a dataset or the number of records that match specific criteria.
- SUM: The SUM function calculates the sum of values in the specified column, offering a quick and straightforward way to compute the total value of numeric data. It can be particularly useful for tasks such as aggregating sales data or calculating total costs.
- AVG: The AVG function computes the average value of a specified column, allowing users to determine the mean value of a dataset. This is especially practical when dealing with outcomes or averages, like exam scores, ratings, or any quantitative data.
- MAX: The MAX function identifies the maximum value in a selected column, delivering the highest value stored within the dataset. It is predominantly used to single out records or groups with the highest or most significant values.
- MIN: The MIN function, in contrast to MAX, retrieves the minimum value in the given column, and presents the lowest value contained within the dataset. It is useful for identifying records or groups with the lowest values or the smallest numbers.
Example: Applying the COUNT, SUM, and AVG functions to extract information regarding sales data.
SELECT COUNT(sale_id) as total_sales, SUM(revenue) as total_revenue, AVG(revenue) as average_revenue FROM sales_data;
This SQL query will produce the total number of sales, the total revenue generated, and the average revenue per sale from the sales_data table.
Scalar Functions in SQL
Scalar functions in SQL, unlike aggregate functions, operate on a single value and return a single output value. These functions can be further categorised based on functionality, such as string functions, numeric functions, and date and time functions. The following are detailed descriptions of these categories and their most common scalar functions:
String Functions: Designed for manipulating or managing text-based data, string functions can perform operations like converting text case, extracting specific characters, or measuring the length of a string value.
- UPPER: The UPPER function converts a given string to uppercase, allowing for uniformity of data or easier comparison of text values.
- LOWER: The LOWER function, conversely, changes a string to lowercase to position data in a common format or facilitate simpler text value comparisons.
- LEFT: The LEFT function extracts a specified number of characters from the left side of a string, making it possible to retrieve certain parts of a text value for analysis or manipulation purposes.
- RIGHT: Similar to LEFT, the RIGHT function derives a defined number of characters from the right side of a string for similar data processing requirements.
- LENGTH: The LENGTH function calculates the length of a string in terms of the number of characters it contains. This can be crucial for validating the consistency of data or analysing the distribution of character lengths in a dataset.
Numeric Functions: Numeric functions focus on performing mathematical operations or calculations on numeric data, such as rounding values, determining absolute values, or identifying the largest or smallest integers relative to a given number.
- ABS: The ABS function returns the absolute value of a given number, eliminating the negative sign, if present. It is handy for calculating distances or determining the magnitude of a value regardless of its direction.
- ROUND: The ROUND function rounds a number to the nearest specified integer or decimal place value, ensuring precision or simplifying numeric data for easier comprehension or comparison.
- CEILING: The CEILING function calculates the smallest integer greater than or equal to a specified number, often required for rounding up, estimating, or approximating values.
- FLOOR: The FLOOR function determines the largest integer less than or equal to a given number, a technique used for rounding down or generating conservative approximations of numerical data.
Date and Time Functions: Date and time functions are designed to manipulate and manage date and time values, making it possible to extract parts of a datetime value, calculate differences between two dates, or return the current date and time.
- NOW: The NOW function displays the current date and time, enabling users to timestamp records or events in the database system.
- DATE: The DATE function extracts only the date portion of a date or datetime value, allowing for the separation and individual analysis of date-related information from datetime data.
- TIME: The TIME function retrieves only the time portion of a date or datetime value, facilitating the isolation and examination of time-specific information within datetime data.
- DATEDIFF: The DATEDIFF function calculates the difference between two given date values, useful for determining the elapsed time between two events or the age of a record within the database.
SQL Functions vs Procedures
SQL functions and procedures are both essential components in designing and managing SQL database systems. Though they may appear similar at first glance, they serve different purposes and have distinctive characteristics. Before diving into the key differences between SQL functions and procedures, let's briefly define them:
- SQL Function: A function is a pre-defined, reusable piece of SQL code that accepts input parameters, performs an operation or calculation, and returns a single output value. Functions are typically used for manipulating and retrieving data in the database.
- SQL Procedure: A procedure, also known as a stored procedure, is a pre-defined, reusable SQL script that can accept input parameters, execute multiple statements, and return multiple output values in the form of a result set or table. Procedures are often used to encapsulate complex, multi-step SQL tasks.
Now that we have established the basic definitions, we can explore the key differences between SQL functions and procedures as well as when to use these constructs to optimise your database operations.
Key Differences Between Functions and Procedures
Understanding the fundamental differences between SQL functions and procedures can help you determine which option is best for your specific use case. The following key differences between functions and procedures should guide you towards an informed decision:
- Return Value: Functions must return a single value, whereas procedures can return multiple result sets or no value at all. Since procedures can perform several SQL statements sequentially, their output can be more complex and versatile than that of functions.
- Data Manipulation: While functions can perform data retrieval and calculation operations, they generally cannot perform data manipulation tasks (INSERT, UPDATE, or DELETE) directly. On the other hand, procedures can execute a wider range of SQL statements, including data manipulation and transaction control commands.
- Invocation: Functions can be called from within a SELECT statement and are capable of being used in conjunction with other SQL expressions. In contrast, procedures are typically invoked using the CALL or EXECUTE statements, and cannot be used directly in a SELECT statement or expression.
- Error Handling: Procedures typically have built-in error handling features, allowing them to provide detailed feedback in case of failures or errors. Functions, due to their limited scope, generally do not include such extensive error handling capabilities.
- Transaction Control: Functions cannot utilise transaction control statements (COMMIT, ROLLBACK, or SAVEPOINT), whereas procedures can, providing greater control over the management and execution of database transactions.
When to Use SQL Functions and Procedures
Choosing between SQL functions and procedures depends on the specific requirements and goals of your project. Here are some guidelines to help determine when to use functions and when to use procedures:
- Use Functions When:
- You need to return a single value or perform a simple calculation based on input parameters.
- The main purpose of your code is data retrieval and manipulation rather than complex tasks or data manipulation.
- You want to embed the code as a part of a SQL statement or expression.
- There are limited error handling and transaction control requirements.
- Use Procedures When:
- The purpose of your code involves multiple complex statements or data manipulation tasks.
- You need to return multiple result sets or tables rather than a single value.
- Error handling and transaction control are essential to your SQL operations.
- Your code is not strictly concerned with data retrieval but also focuses on conditional logic, loops, or other programming constructs.
In conclusion, SQL functions and procedures have their respective advantages and limitations, so your choice depends on the specific requirements of your database operations. Remember to consider the essential differences and use case guidance in this article as you choose the best approach for your SQL operations.
Advanced SQL Functions
Beyond the basic SQL functions we have explored so far, there are some more advanced functions that can significantly enhance SQL querying capabilities and add efficiency to data handling tasks. In the following sections, we will discuss window functions and user-defined functions in SQL that provide essential tools in manipulating and analysing data in complex environments.
Window Functions in SQL
Window functions in SQL are a powerful set of features that allow you to perform calculations over a set of rows related to the current row within a query result. These calculations are made while retaining the original row details. Window functions work in tandem with the OVER() clause, which defines the window or range of rows for the respective calculations. The primary use of window functions is in solving complex data-related problems that involve aggregations, rankings, or cumulative calculations.
There are three main categories of window functions in SQL:
- Aggregation Window Functions: These functions are similar to the traditional SQL aggregate functions like COUNT, SUM, AVG, MIN, MAX except that they operate on the window of rows defined by the OVER() clause.
- Ranking Window Functions: These functions help to rank rows within the result set based on specific criteria, including ROW_NUMBER, RANK, DENSE_RANK, and NTILE.
- Offset Window Functions: Applied to retrieve the value of a column from a row that is positioned at a relative offset within the window, these functions include LAG and LEAD.
Example: Applying the ROW_NUMBER() window function to rank sales employees based on their revenue.
SELECT sales_employee, revenue, ROW_NUMBER() OVER (ORDER BY revenue DESC) AS revenue_rank FROM sales_data;
This query will assign each sales employee a rank based on their revenue, with the highest revenue earning a rank of 1.
When using window functions, keep in mind the important clauses:
- ORDER BY: Determines the sorting order of the rows within the window. It is an essential clause in most window functions, as it helps sequence and rank data according to specified criteria.
- PARTITION BY: Defines groups or partitions of rows that have the same values in specified columns. It leads to the application of the window function separately to each partition, generating separate rankings or calculations for each group.
- ROWS BETWEEN: Specifies the range of rows within the window, giving you finer control over the rows included in the calculations. It is useful when working with sliding or expanding windows that require variable row ranges for different calculations.
User-Defined Functions in SQL
User-Defined Functions (UDFs) in SQL are custom functions written by users that can be stored and reused within the SQL database system. UDFs enable you to create custom calculations, data manipulations, and operations tailored to your specific needs. These functions can be built using SQL or other programming languages, depending on the database management system (DBMS) and its support for external languages.
UDFs are generally classified into two types:
- Scalar User-Defined Functions: These UDFs accept one or more input parameters, perform an operation or calculation, and return a single value. Scalar UDFs can be used in SQL expressions, queries, views, and stored procedures just like built-in scalar functions.
- Table-Valued User-Defined Functions: These UDFs accept input parameters and produce a table or tabular result set as their output. Table-valued UDFs can be used in a SELECT statement's FROM clause or as a part of a JOIN, allowing complex operations with tabular data that go beyond scalar values.
Example: Creating a simple scalar UDF to calculate sales commissions.
CREATE FUNCTION dbo.CalculateCommission(@revenue DECIMAL(18, 2)) RETURNS DECIMAL(18, 2) AS BEGIN DECLARE @commission_rate DECIMAL(4, 3); IF @revenue < 10000 SET @commission_rate = 0.10; ELSE SET @commission_rate = 0.15; RETURN @revenue * @commission_rate; END
This user-defined function takes the revenue as an input parameter and calculates the sales commission based on the appropriate commission rate.
When implementing UDFs in your SQL database system, consider the following best practices:
- Define the function with a clear, descriptive name to ensure easy identification and understanding of its purpose.
- Include comments and documentation explaining the function's functionality, input parameters, and outputs.
- Optimise the function code for performance and avoid resource-intensive operations, as UDFs may be executed repeatedly in large or complex queries.
- Test the function thoroughly to ensure its correctness, stability, and performance under various input conditions and scenarios.
By incorporating advanced SQL functions such as window functions and user-defined functions in your database, you can significantly expand your data processing capabilities, improve complex query performance, and create customised solutions tailored to your unique requirements.
SQL Functions Best Practices
Adhering to best practices while working with SQL functions plays a crucial role in ensuring the efficiency, readability, and maintainability of your database queries and systems. Following these best practices helps prevent common pitfalls and enables you to deliver high-quality solutions in a timely manner.
Efficient Use of SQL Functions
Using SQL functions efficiently and effectively is vital for optimising database operations and enhancing performance. To maximise the benefits and avoid potential issues, consider implementing the following best practices:
- Opt for appropriate functions: Be mindful of selecting the most suitable function for your task, considering both the type (aggregate, scalar, or window) and the function’s properties, such as data manipulation or calculation capabilities.
- Minimise nesting functions: Limit the depth of nested functions to reduce complexity and improve readability. Overuse of nested functions can lead to challenging queries, impacting performance and maintainability.
- Use built-in functions: Whenever possible, use built-in SQL functions, as they tend to be more optimised and provide better performance than user-defined functions, given the database management system's support and enhancements.
- Reduce function calls: To enhance query performance, minimise the number of function calls within a single query. Repeatedly calling the same function can lead to performance degradation, especially with resource-intensive functions.
- Test and optimise function performance: Thoroughly test the performance of functions in various scenarios and adjust the code, if needed. Monitor the database system's performance indicators and use profiling tools, such as execution plans, to identify potential bottlenecks and optimisation opportunities.
- Reusability and modularisation: Design functions to be reusable and modular, aiming to enhance maintainability and versatility within the database system. This takes advantage of the benefits of code reuse and allows easier debugging and performance tuning.
Common Pitfalls and How to Avoid Them
Understanding and avoiding common pitfalls when working with SQL functions is essential to reduce errors, enhance efficiency, and develop reliable database systems. The following are some prevalent pitfalls and recommended approaches to prevent them:
- Using functions for data manipulation: SQL functions, especially scalar ones, are not suited for direct data manipulation tasks, such as INSERT, UPDATE, or DELETE. Instead, use stored procedures or dedicated SQL statements for such operations.
- Misusing aggregate functions: Avoid applying aggregate functions to individual rows, as they are specifically designed for working with groups of data. Utilise scalar functions when focusing on a single row or value.
- Confusing functions and procedures: Ensure clear distinctions between SQL functions and procedures in your database operations, considering factors such as return values, invocation methods, and data manipulation capabilities. Focus on the specific requirements of your tasks when choosing between functions and procedures.
- Ignoring error handling: Be attentive to proper error handling, especially when using user-defined functions. Implement error handling mechanisms, such as input validation, exception handling, and informative error messages, to ensure smooth database functioning and accurate outputs.
- Overloading the database system: Prevent resource-intensive function calls and operations from negatively impacting the database system's performance. Monitor system resources, employ optimisation techniques, and consider scaling out your infrastructure to handle increased workloads when necessary.
- Formatting issues: Ensure correct formatting within your SQL statements, particularly when using functions involving parameters, dates, and string manipulation. Formatting errors can lead to incorrect results or database errors, affecting the overall integrity of your data.
By implementing these best practices and avoiding common pitfalls, you can make the most of SQL functions, enhance the performance and maintainability of your database systems, and deliver more efficient solutions.
SQL Functions - Key takeaways
SQL Functions: building blocks for data management and manipulation in relational databases; divided into aggregate and scalar functions
Aggregate functions: calculations on multiple rows of data, returning a single value; examples include COUNT, SUM, AVG, MIN, MAX
Scalar functions: operate on a single value, returning a single value; examples include UPPER, LOWER, ROUND, NOW
SQL Functions vs Procedures: functions return a single value and are used in SELECT statements, while procedures can return multiple values and perform data manipulation tasks
Advanced SQL Functions: window functions and user-defined functions (UDFs) offer extended capabilities for complex data manipulations and custom calculations
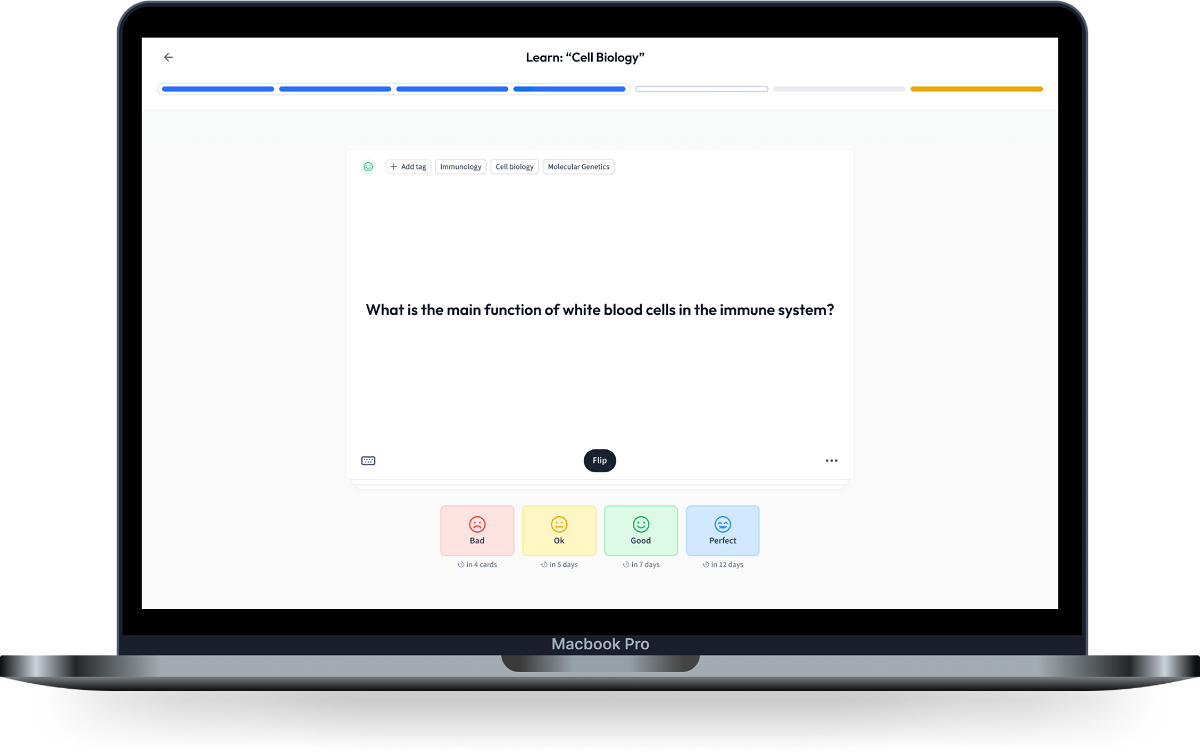
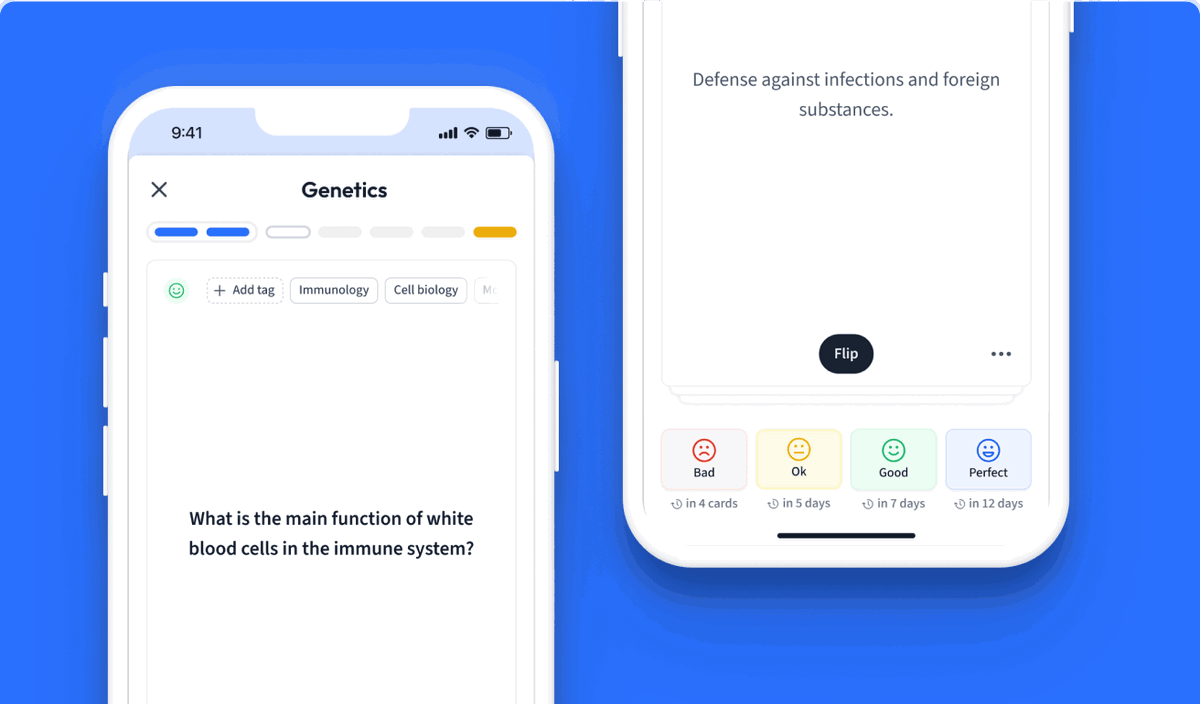
Learn with 15 SQL Functions flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about SQL Functions
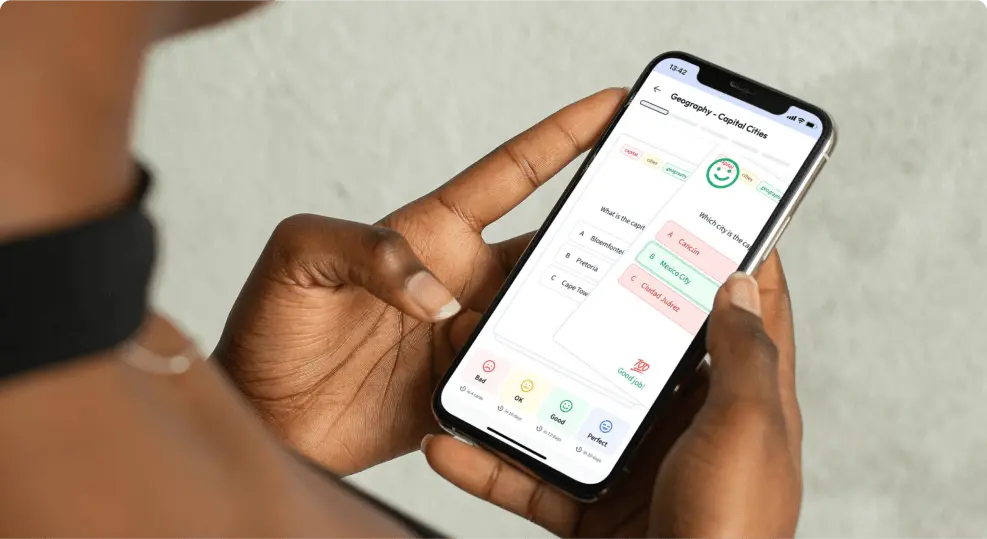
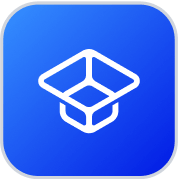
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more