Jump to a key chapter
Introduction to SQL HAVING and Its Functions
In the world of Computer Science, SQL is a powerful programming language that enables you to manage and retrieve data from relational databases. One of the essential features in SQL is the HAVING clause, which filters the results of a GROUP BY query based on a specified condition. In this article, you'll gain a solid understanding of the SQL HAVING clause, its functions, and how to utilise it effectively in various real-life scenarios.
Understanding the difference between HAVING and WHERE in SQL
In SQL, both HAVING and WHERE clauses serve as filters for the data being queried. While they are similar in purpose, their functional difference lies in the fact that the WHERE clause filters data prior to aggregation while the HAVING clause filters data after aggregation.
Here's a brief comparison of the HAVING and WHERE clauses:
- WHERE is used to filter records before any aggregations are performed.
- HAVING is used to filter the aggregated data based on a specified condition.
- WHERE is not used with aggregate functions (e.g., COUNT(), SUM(), AVG()), whereas HAVING is exclusively used with such functions.
HAVING Clause | WHERE Clause |
Filters aggregated data | Filters records before aggregation |
Used with aggregate functions | Not used with aggregate functions |
It's crucial to understand the functional difference between the HAVING and WHERE clauses to ensure efficient data filtering and maintain query performance in your databases.
Utilising SQL Server HAVING in real-life examples
For a better understanding of the SQL HAVING clause, let's explore how it can be used to solve various real-life problems by analysing different aggregate functions. Using practical examples not only makes it easier to comprehend the concept but also reinforces your skills and knowledge in SQL.
SQL Server HAVING with COUNT() function
Imagine you have a table containing information about various products and their categories. You want to find the categories that contain more than a certain number of products. In this case, you can use the COUNT() function along with HAVING to achieve your goal.
The following SQL query returns the categories having more than 5 products:
SELECT category, COUNT(product_id) AS product_count
FROM products
GROUP BY category
HAVING product_count > 5;
SQL Server HAVING with SUM() and AVG() functions
Suppose you have a table containing sales data with each row representing an individual sale. You want to find the salespersons who have generated a total revenue above a certain amount or have an average sale amount above a particular threshold.
The following SQL query returns the salespersons who have generated a total revenue greater than 10,000, and have an average sale amount greater than 500:
SELECT salesperson_id, SUM(sales_amount) AS total_revenue, AVG(sales_amount) AS average_sale
FROM sales
GROUP BY salesperson_id
HAVING total_revenue > 10000 AND average_sale > 500;
SQL Server HAVING with MAX() and MIN() functions
Imagine you have a table containing information about different stock prices for various companies. You want to find the companies with a maximum stock price above a certain value and a minimum stock price below another specific value.
The following SQL query returns the companies satisfying both conditions with a maximum stock price above 100 and a minimum stock price below 50:
SELECT company_id, MAX(stock_price) AS max_price, MIN(stock_price) AS min_price
FROM stocks
GROUP BY company_id
HAVING max_price > 100 AND min_price < 50;
Remember, when using the SQL HAVING clause with multiple aggregate functions, it's important to ensure that each condition is well-defined and the query is properly structured for an accurate and efficient filtering process.
Practical Exercises for Mastering SQL HAVING
Engaging in practical exercises is a great way to enhance your understanding of the SQL HAVING clause and improve your skills in data filtering using different aggregate functions. The following exercises will enable you to apply your knowledge of SQL HAVING in real-life scenarios and develop complex queries involving multiple aggregate functions or grouping records by unique categories.
SQL Server HAVING clause tasks for data filtering
To master the HAVING clause in SQL Server, it's crucial to practice various tasks related to data filtering. By addressing these tasks, you'll work on real case scenarios that aid in fully grasping the potential of the HAVING clause. Below are some exercises that will challenge and deepen your understanding of the SQL HAVING clause:
Grouping records by unique categories
Consider a table called 'orders' representing customer orders with order_id, customer_id, order_date, and sales_amount columns. Your task is to find the number of orders placed by each customer and the total sales amount for customers with more than 5 orders and a total sales amount greater than 7,000. To accomplish this, follow the steps below:
- Use the GROUP BY clause to group the records by customer_id.
- Apply the COUNT() function to find the number of orders per customer.
- Utilise the SUM() function to compute the total sales amount for each customer.
- Include the HAVING clause to filter the result set based on the given conditions (number of orders > 5 and total sales amount > 7,000).
After completing these steps, evaluate and analyse the results obtained to better understand how the HAVING clause, when coupled with aggregate functions and GROUP BY, can be effectively employed to filter records.
Developing complex queries with multiple aggregate functions
In this exercise, consider a table named 'employees' containing employee_id, department_id, salary, and hire_date columns. Your goal is to find the departments with both the highest and lowest average salaries and an overall average salary above a specified threshold. To achieve this, undertake the following steps:
- Use the GROUP BY clause to group the records by department_id.
- Apply the AVG() function to find the average salary per department.
- Utilise the MAX() and MIN() functions to determine the highest and lowest average salaries among all departments.
- Incorporate the HAVING clause to filter the result set based on the given conditions (overall average salary above a particular threshold).
Upon successful completion of this exercise, you'll have a grasp of the flexibility and efficiency of the HAVING clause when used in conjunction with different aggregate functions and complex query requirements.
Repeatedly engaging in such exercises broadens your understanding of SQL HAVING and its functions, empowers you to develop intricate queries, and consolidates your foundation in SQL Server data filtering techniques.
Advanced Techniques for SQL HAVING Clause
As you become more proficient with the SQL HAVING clause, it's essential to explore advanced techniques that can enhance your query-writing capabilities and overall efficiency when working with relational databases. In this section, we'll delve into more in-depth aspects of the HAVING clause and cover techniques, including nested queries, use with other SELECT statement clauses, and using aliases for better query readability and maintenance.
Utilising Nested Queries with SQL HAVING
Nested queries, also known as subqueries, are a highly effective method for solving complex problems involving multiple levels of data filtering and analysis. By incorporating subqueries within the HAVING clause, you can achieve more refined and sophisticated result sets that meet unique business requirements. In this section, we'll examine the applications of nested queries with the SQL HAVING clause and provide examples for better comprehension.
Imagine you have a table called 'sales', which contains sales data with columns such as product_id, sales_date, and revenue. Your goal is to find products whose daily average revenue exceeds the overall average daily revenue across all products. To solve this problem using nested queries with the HAVING clause, you can follow these steps:
- Calculate the overall average daily revenue across all products using a subquery.
- Compute the average daily revenue for each product using a GROUP BY clause and the AVG() aggregate function.
- Employ the HAVING clause with the subquery to filter out products whose average daily revenue is above the overall average daily revenue.
The following SQL query can fulfil the desired task:
SELECT product_id, AVG(revenue) AS average_daily_revenue
FROM sales
GROUP BY product_id
HAVING average_daily_revenue > (SELECT AVG(revenue) FROM sales);
By utilising nested queries with the HAVING clause, you can significantly enhance your data analysis capabilities and tackle complex problems with ease.
Using SQL HAVING with Other SELECT Statement Clauses
The power of the HAVING clause can be further amplified when used alongside other SELECT statement clauses, such as DISTINCT, JOIN, and UNION. In this section, we'll cover examples of HAVING combined with these clauses to demonstrate its versatility and applicability in various scenarios.
SQL HAVING with DISTINCT
In some situations, you may want to filter aggregated records and return only unique values. You can achieve this by using the DISTINCT keyword along with the HAVING clause. For example, consider a table named 'employees' with columns employee_id, department_id, and salary. Your task is to find unique departments with an average salary above a defined threshold:
The following SQL query demonstrates how to use DISTINCT with the HAVING clause:
SELECT DISTINCT department_id
FROM employees
GROUP BY department_id
HAVING AVG(salary) > 5000;
SQL HAVING with JOIN
The HAVING clause can also play a crucial role in data retrieval when combining records from multiple tables using JOIN operations. Suppose you have two tables - 'employees' and 'departments', with columns employee_id, salary, department_id, department_name, respectively. Your goal is to find the department names with an average salary above a certain threshold.
The following SQL query demonstrates combining HAVING with a JOIN clause:
SELECT d.department_name, AVG(e.salary) AS avg_salary
FROM employees e
JOIN departments d ON e.department_id = d.department_id
GROUP BY d.department_name
HAVING avg_salary > 5000;
SQL HAVING with UNION
In cases where you need to filter aggregated results obtained by combining datasets from different tables, you can use the UNION operator in conjunction with the HAVING clause. For example, let's assume you have two tables - 'sales2019' and 'sales2020' containing product_id, sales_date, and revenue columns. Your objective is to find the products whose total revenue in both 2019 and 2020 exceeded a given amount:
Use the HAVING clause in combination with UNION for this purpose:
SELECT product_id, SUM(revenue) AS total_revenue
FROM (SELECT product_id, sales_date, revenue FROM sales2019
UNION ALL
SELECT product_id, sales_date, revenue FROM sales2020) AS combined_sales
GROUP BY product_id
HAVING total_revenue > 10000;
These examples illustrate the immense flexibility and applicability of SQL HAVING in combination with other SELECT statement clauses, enabling you to solve complex problems efficiently.
SQL HAVING - Key takeaways
SQL HAVING: Filters results of a GROUP BY query based on a specified condition.
Difference having and where in SQL: WHERE filters data before aggregation, while HAVING filters data after aggregation.
SQL Server HAVING examples: COUNT(), SUM(), AVG(), MAX(), and MIN() functions for various data filtering tasks.
SQL HAVING clause with nested queries: Enhances data analysis capabilities by incorporating subqueries within the HAVING clause.
Using SQL HAVING with other SELECT statement clauses: DISTINCT, JOIN, and UNION for versatile and efficient data retrieval.
Learn faster with the 14 flashcards about SQL HAVING
Sign up for free to gain access to all our flashcards.
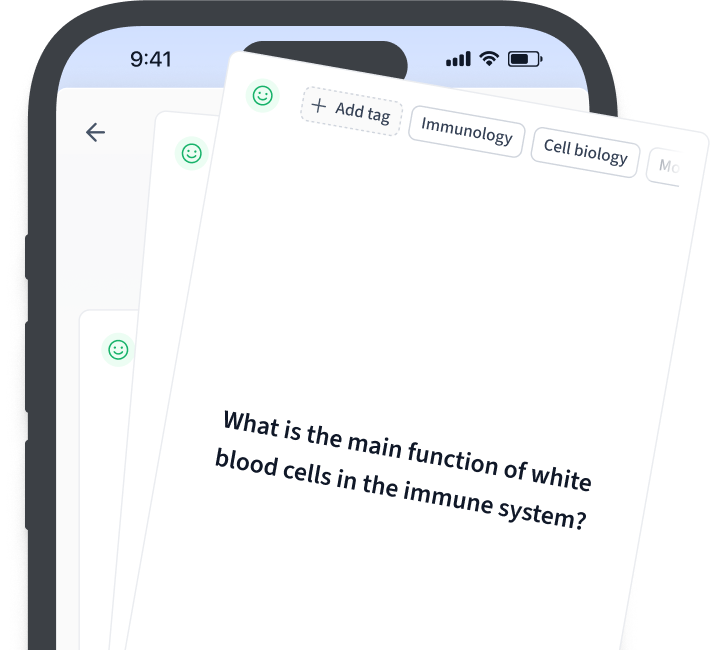
Frequently Asked Questions about SQL HAVING
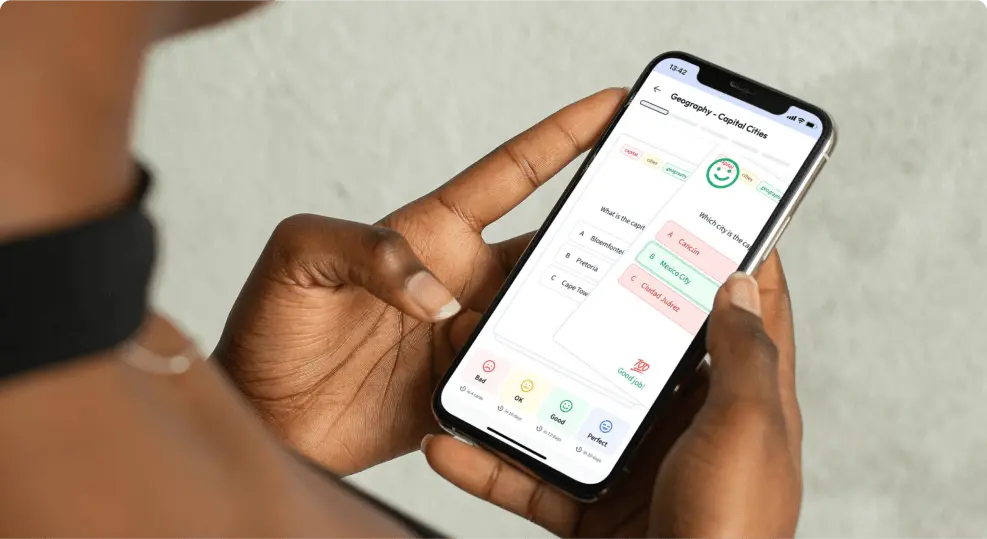
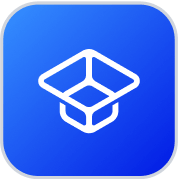
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more