Jump to a key chapter
Understanding SQL Invoked Functions
SQL Invoked Functions are a crucial part of contemporary database management, offering a dynamic approach to handling data. They allow for complex operations to be encapsulated within a callable routine.
What is a SQL Invoked Function? An In-Depth Look
SQL Invoked Function: A user-defined routine that accepts parameters, performs an operation, and returns a result. These functions are stored and run within the database server.
At its core, a SQL Invoked Function (SIF) is built to perform a specific task within a SQL database. Unlike built-in functions provided by SQL, these user-defined functions can be tailored to meet the unique requirements of your database applications. Functions may return a single value or a table and can be invoked in SQL queries to process data efficiently.
Example of a SQL Invoked Function:
CREATE FUNCTION getEmployeeAge (employeeID INT) RETURNS INT BEGIN DECLARE age INT; SELECT birthday INTO age FROM Employees WHERE ID = employeeID; RETURN (YEAR(CURRENT_DATE) - YEAR(age)); END;This function calculates the age of an employee based on their ID by retrieving their birthday from a table and comparing it to the current year.
SQL Invoked Functions can be either scalar, returning a single value, or table-valued, returning a set of rows.
Why SQL Invoked Functions are Important in Database Management
SQL Invoked Functions enhance database functionality, offering a flexible toolset for data operation. This adaptability is particularly useful in data manipulation and customized reporting.
Their importance lies in the ability to encapsulate complex operations, thus simplifying SQL queries. By defining operations that can be reused across multiple SQL statements, you avoid redundancy and ensure a more organised and maintainable database structure. Moreover, functions can improve performance by allowing operations to be executed closer to the data.
Business use case: Imagine a business analyst needs to generate monthly reports on employee productivity based on various metrics. Instead of writing complex queries every month, a SQL Invoked Function can be created to calculate and summarise this data. Whenever the report is required, simply calling this function with the appropriate parameters generates the necessary information, streamlining the reporting process.
Performance Advantages of SQL Invoked Functions:Although SQL Invoked Functions bring organisational benefits, their impact on database performance is nuanced. Under certain conditions, such as when the function’s logic is highly optimised and database caching mechanisms are effectively utilised, these functions can significantly reduce the amount of data transferred between the database server and client application, leading to better overall performance. Conversely, poorly designed functions may introduce performance penalties. Therefore, optimisation and testing are key when implementing SQL Invoked Functions.
How to Invoke Function in SQL Server
Invoking a function in SQL Server is a skill that elevates your database management and query formulation. These functions, tailored to specific tasks, can be powerful tools in processing and organizing data efficiently.
Step-by-Step Guide: Invoking Functions in SQL
Invoking a function in SQL Server involves understanding the type of function you are working with and knowing the correct syntax to execute it. Here's a comprehensive step-by-step guide:
1. Identify the Function Type: SQL Server supports several types of functions, including scalar functions, which return a single value, and table-valued functions (TVFs), which return a table data type.2. Understand the Syntax: The basic syntax for invoking a scalar function is
SELECT dbo.FunctionName(arguments);For table-valued functions, it might look like
SELECT * FROM dbo.FunctionName(arguments);3. Passing Arguments: If your function requires arguments, ensure they are passed in the correct order and data type.4. Executing the Function: Once you have your function and arguments ready, execute the query in your SQL Server management interface.
Example of Invoking a Scalar Function:
SELECT dbo.getEmployeeAge(12345);This query invokes a scalar function named
getEmployeeAge
, passing it the employee ID 12345
as an argument. When invoking table-valued functions, consider joining it with other tables to integrate its results into broader queries. This approach leverages the power of SQL to perform complex data analysis:
SELECT Employees.Name, AgeData.Age FROM Employees JOIN dbo.getEmployeeAgeData() AS AgeData ON Employees.ID = AgeData.EmployeeID;This joins the results of
getEmployeeAgeData
, a table-valued function, with an Employees table, allowing for enriched data retrieval.Common Mistakes to Avoid When Invoking SQL Functions
As you embark on invoking SQL functions, being aware of common pitfalls can save you from unnecessary frustration and errors. Here are key mistakes to avoid:
- Ignoring the Function's Return Type: Ensure you understand whether the function returns a scalar value or a table, as this affects how you incorporate it into your queries.
- Incorrect Syntax: A slight deviation in syntax, such as a missing parenthesis or incorrect argument order, can result in errors. Always double-check your syntax.
- Improper Use of Arguments: Passing arguments that do not match the function's required data types or omitting required arguments can lead to unexpected results.
- Not Testing in Development: Always test new functions in a development environment before deploying them in production. This practice helps identify and rectify potential issues early in the development cycle.
For complex functions requiring optimization, use the SQL Server Profiler or Execution Plan to analyse performance and fine-tune as necessary.
A deep understanding of SQL Server's error messages can significantly help in troubleshooting function invocation errors. For example, an Incorrect syntax near the keyword 'FROM'
error might indicate a misplaced keyword or a typo in the function's name or arguments. Leveraging the detailed information these error messages provide can greatly expedite the debugging process.
SQL Invoked Function Example and Syntax
Diving into the world of SQL Invoked Functions offers a unique way of handling database operations efficiently and effectively. By customising queries, you become equipped to tackle complex data manipulations with ease.
Breaking Down SQL Invoked Functions Syntax
Understanding the syntax of SQL Invoked Functions is fundamental to leveraging their full potential. A basic grasp of this syntax facilitates the creation and utilisation of functions tailored to specific data processing needs.The syntax structure for creating a SQL Invoked Function can generally be broken down as follows:
CREATE FUNCTION FunctionName (Parameters) RETURNS ReturnType [WITH {SCHEMABINDING | ENCRYPTION}] AS BEGIN -- Function body RETURN [Value] END;This outlines the template for defining a new SQL Invoked Function, where:
- Function Name is the unique identifier of your function.
- Parameters are the inputs the function will work with. These are optional.
- ReturnType defines what your function will return. This could be a scalar value or a table, depending on the function type.
- The Function Body contains the SQL statements that define the operations to be performed.
- RETURN specifies the output the function will produce.
Remember, the 'RETURNS' keyword is crucial as it directly influences how the function can be called and used within queries.
Practical Examples of SQL Invoked Functions
To fully grasp how SQL Invoked Functions can be utilised in real database operations, exploring practical examples is invaluable. These instances demonstrate the flexibility and power of custom functions in manipulating data.Consider a scenario where you need to find the total number of orders placed by a customer in an e-commerce database:
CREATE FUNCTION TotalOrders (@CustomerID INT) RETURNS INT AS BEGIN DECLARE @Result INT; SELECT @Result = COUNT(*) FROM Orders WHERE CustomerID = @CustomerID; RETURN @Result; END;This function accepts a CustomerID as an input parameter and returns the total count of orders placed by that customer. It encapsulates a common query inside a reusable function, enhancing code readability and maintenance.
Taking a deeper dive into the mechanics of SQL Invoked Functions reveals their capability to not just simplify queries, but also to secure sensitive data processes and enhance database performance. By localising critical operations within the database, these functions minimise the need for multiple elaborate queries, reducing network traffic and server load.Moreover, through optional features like ENCRYPTION and SCHEMABINDING, these functions offer additional layers of security and integrity, safeguarding the function's definition and preventing unauthorised modifications.
Control Statements in SQL and Their Role in SQL Invoked Functions
In the realm of SQL (Structured Query Language), control statements play a pivotal role in managing the flow of execution within SQL Invoked Functions. These statements provide the necessary logic to perform dynamic data processing, making the functions more versatile and powerful.Understanding how to incorporate control statements into SQL Invoked Functions can significantly enhance the efficiency and capability of database applications, allowing for more complex and conditional data manipulation.
An Overview of Control Statements in SQL
Control statements in SQL are instructions that manage the execution flow of SQL scripts, giving you the ability to perform conditional processing, looping, and flow control. These include IF-ELSE statements, WHILE loops, and CASE statements, among others.Utilising these control statements allows for the creation of more dynamic and responsive SQL queries and functions, enabling decision-making processes within SQL code.
Example of an IF-ELSE Statement:
IF (condition) BEGIN -- Statements to execute if condition is true END ELSE BEGIN -- Statements to execute if condition is false END;This illustrates how an IF-ELSE statement can be used to execute different SQL code blocks based on a specific condition.
How Control Statements Enhance SQL Invoked Functions
Integrating control statements into SQL Invoked Functions elevates the functionality and adaptability of these functions. By embedding conditional logic, loops, and other control flows, functions can dynamically respond to different data and conditions, making them incredibly efficient for database management.This adaptability is essential for developing complex data processing routines within a database, supporting a wide range of applications, from data validation to dynamic report generation.
Control statements, particularly within SQL Invoked Functions, are fundamental in implementing complex logic directly on the database level. This has significant performance implications, as it reduces the need for application-level data processing, lessening the processing load on the client side and minimizing network traffic. Moreover, by encapsulating this logic within the database, we improve data integrity and security, as the operations are executed in a controlled environment.Furthermore, the use of control statements in SQL Invoked Functions facilitates the creation of a modular code structure. This modularity makes the code more maintainable and reusable, as functions can be easily modified or combined without affecting other parts of the database system.
Leveraging control statements in SQL Invoked Functions can also simplify error handling by implementing TRY-CATCH blocks within functions to manage exceptions and errors effectively.
Sql Invoked Functions - Key takeaways
- SQL Invoked Function Definition: A user-defined routine that executes within the database server, which can accept parameters, perform operations, and return a result. They are designed to handle specific tasks that can either return a single scalar value or a table.
- How to Invoke Function in SQL Server: Determine the function type (scalar or table-valued), use the appropriate syntax (e.g.,
SELECT dbo.FunctionName(arguments);
for scalar functions), pass arguments correctly, and execute the query. - SQL Invoked Function Example:
CREATE FUNCTION getEmployeeAge (employeeID INT) RETURNS INT BEGIN DECLARE age INT; SELECT birthday INTO age FROM Employees WHERE ID = employeeID; RETURN (YEAR(CURRENT_DATE) - YEAR(age)); END;
This illustrates a function calculating an employee's age. - SQL Invoked Functions Syntax: The basic structure for defining these functions includes a function name, optional parameters, return type, function body, and the return value, following the format:
CREATE FUNCTION FunctionName (Parameters) RETURNS ReturnType AS BEGIN -- Function body RETURN [Value] END;
- Control Statements in SQL: Key in managing execution flow within SQL Invoked Functions, such as IF-ELSE statements, WHILE loops, and CASE statements, which allow for conditional processing and dynamic data handling.
Learn faster with the 28 flashcards about Sql Invoked Functions
Sign up for free to gain access to all our flashcards.
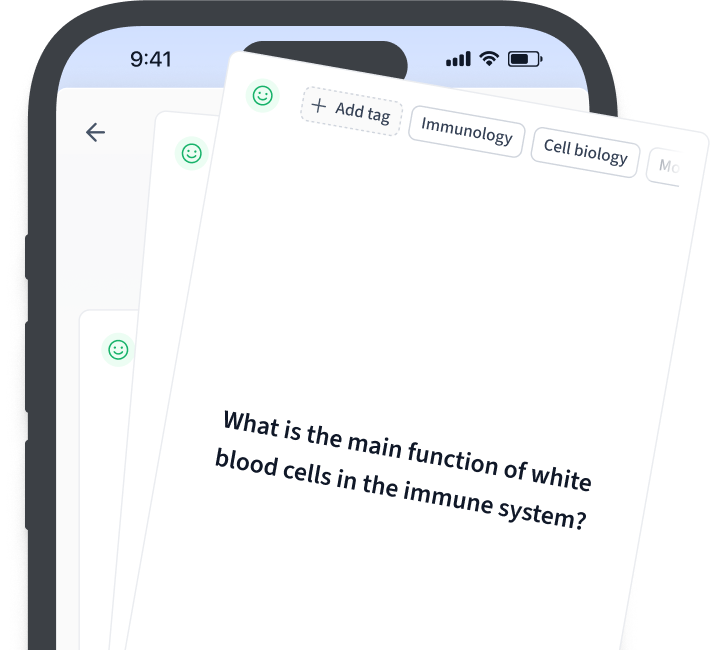
Frequently Asked Questions about Sql Invoked Functions
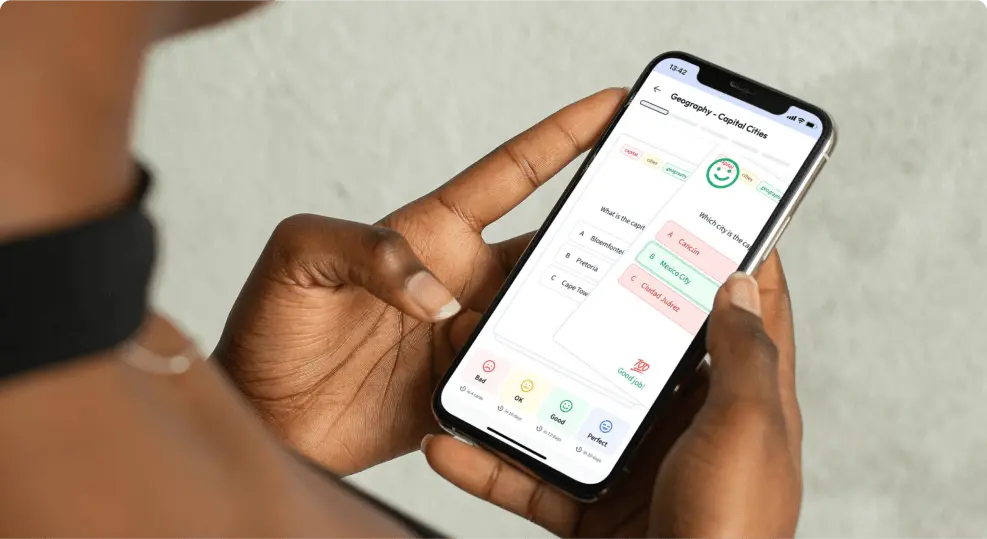
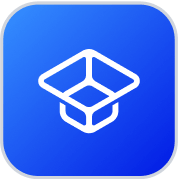
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more