Jump to a key chapter
Understanding SQL Join Tables
When working with databases, you will often encounter situations where it's necessary to retrieve data from multiple tables. SQL Join Tables facilitates this process by allowing you to combine two or more tables based on a related column between them. In this way, you can create a new, temporary result set that includes all the required information.
Types of SQL Join Operations
SQL provides a variety of join types to handle different scenarios when combining tables. Let's delve into the most common types: 1. INNER JOIN: Returns rows from both tables only if there's a match between the specified columns. 2. LEFT JOIN (or LEFT OUTER JOIN): Retrieves all records from the left table along with matching records from the right table. If no matching rows exist for the right table, NULL values are displayed. 3. RIGHT JOIN (or RIGHT OUTER JOIN): It's the opposite of a LEFT JOIN. It returns all records from the right table and matching rows from the left table. If no match is found, NULL values are displayed for left table columns. 4. FULL JOIN (or FULL OUTER JOIN):Combines all rows from both tables. If there's no match between the columns, the non-matching rows will display NULL values for respective columns.Consider two tables: 'orders' and 'customers'. To get a list of all orders along with customer information, we can use an INNER JOIN operation like this:
SELECT orders.order_id, orders.order_date, customers.customer_id, customers.customer_name
FROM orders
INNER JOIN customers ON orders.customer_id = customers.customer_id;
Applying SQL Join Multiple Tables
At times, you might need to retrieve data from more than two tables. SQL allows you to perform join operations on multiple tables, including any combination of INNER, LEFT, RIGHT, and FULL JOINs. Just ensure that there's a relationship between each involved table.An important aspect to remember when joining multiple tables is the order in which you apply the JOIN clauses. This is because JOIN operations are performed sequentially from left to right in the SQL query, and the order can impact your result.
Advanced techniques to join 3 tables
When joining three or more tables, it's essential to use a logical approach and strategically apply join clauses based on the desired output. Here's a breakdown of how to perform multi-table JOIN operations: 1. Identify related columns: Determine which columns link the tables together. 2. Choose JOIN types: Analyse the desired output and select appropriate JOIN types (INNER, LEFT, RIGHT, or FULL). 3. Write the SQL query:Add the JOIN clauses and the ON conditions in the correct order.Suppose you have three tables: 'orders', 'customers', and 'products'. To retrieve a list of all orders, customer information, and product details, you can structure a SQL query as follows:
SELECT orders.order_id, orders.order_date, customers.customer_id, customers.customer_name, products.product_id, products.product_name
FROM orders
INNER JOIN customers
ON orders.customer_id = customers.customer_id
INNER JOIN products
ON orders.product_id = products.product_id;
SQL Join Tables from Different Databases
Strategies for Effective SQL Join Tables Across Databases
Joining tables from different databases can be a bit complex, but with the right strategies, you can accomplish this task efficiently. Some of the common techniques include:
1. Federated Tables: Some database management systems like MySQL support the concept of federated tables, which allows you to create a virtual table in your local server that is linked to a table in a remote server. This way, you can perform complex JOIN operations across different databases as if they were local tables.
2. Database Linking: In SQL Server and Oracle, you can create a database link (also known as Linked Servers in SQL Server), which establishes a connection between two databases. With this approach, you can reference tables from the linked database using a fully qualified table name, and perform JOIN operations as usual.
3. ETL (Extract, Transform, Load) process: Another approach is extracting data from the tables in different databases, transforming it as needed, and loading the transformed data into a temporary table in one of the databases. This method allows you to work with the data as if it were from a single database, and perform join operations without needing direct access to the other database.
Performance considerations of joining tables from different databases
Joining tables across different databases can have performance implications. It's crucial to consider these factors when designing your SQL query: 1. Network Latency: When fetching data from a remote database, network latency can cause delays. To reduce latency, consider placing the databases closer in terms of network proximity, or using caching mechanisms. 2. Data Transfer Size: Large volumes of data transferred between databases can cause performance degradation. Consider filtering or aggregating the data before joining tables from different databases. 3. Query Optimisation: Execution plans for queries that involve cross-database join operations could be sub-optimal. Improve performance by providing database hints, re-writing the SQL query or materialising the intermediate results. 4. Type of Join Operation: Certain types of joins, such as nested-loop joins, can be less efficient when querying across different databases. Consider using more efficient join algorithms like hash joins or merge joins when it's supported in the databases. 5. Indexing Strategy: Proper indexing can significantly improve join performance. Carefully plan your indexing strategy for the tables involved in cross-database join operations, considering the join predicates being used. By deploying these strategies and keeping performance considerations in mind, you can effectively work with SQL Join Tables across different databases while maintaining acceptable performance levels. Remember to monitor your queries and analyse their performance to further optimise and improve them over time.Advantages and Disadvantages of Joining Tables without Key
There might be scenarios where two tables may not share a common key, but it's still necessary to join them. In such cases, joining tables without key can provide certain benefits as well as drawbacks. Advantages:- Flexibility: Allows you to combine tables based on conditions other than exact key matches.
- Increased data retrieval: Facilitates retrieving information that would otherwise be unattainable through regular join operations.
- Access to non-relational data: Enables performing join operations for non-relational tables that lack primary or foreign keys.
- Performance: Joining tables without a key can result in slower query execution, as it often requires a Cartesian product, which can result in large intermediate result sets.
- Complexity: Handling the conditions required for joining tables without key can be more complicated than using standard join operations.
- Low data integrity: Lack of foreign keys increases the chances of data anomalies, leading to less reliable results.
Techniques for performing SQL joins on tables with no common keys
Although not recommended as a standard practice, it's possible to perform SQL join operations on tables without common keys. Here are some techniques that can be employed in such situations: 1. CROSS JOIN: A CROSS JOIN, also known as a Cartesian product, is used to combine every row from one table with every row from another table. This often results in a large result set, thus it's important to filter the data using WHERE or HAVING clauses. When using the CROSS JOIN, it's essential to be cautious about the performance implications.Suppose you have two tables 'students' and 'courses'. To get a list of all possible student-course combinations, you can use a CROSS JOIN operation like this:
SELECT students.student_id, students.student_name, courses.course_id, courses.course_name FROM students CROSS JOIN courses;
In case two tables share a common value, but it needs transformation on one table before joining, then you can do the following:
SELECT table1.value1, table2.value2 FROM table1 INNER JOIN table2 ON ABS(table1.value1) = table2.value2;
In a scenario where one table contains an 'ID' column, and the other only contains values related to a range of 'ID's, you can leverage subqueries like this:
SELECT a.id, a.name, b.region
FROM ( SELECT id, name, FLOOR(id / 10) AS range FROM table1 )
AS a INNER JOIN table2
AS b
ON a.range = b.range;
SQL Join Tables - Key takeaways
SQL Join Tables: Combine data from two or more tables in a database based on a related column
Common join types: INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN
Join multiple tables: Use a combination of join types and ensure a relationship between involved tables
Join tables from different databases: Use techniques like Federated Tables, Database Linking and ETL process
Join tables without common keys: Employ techniques like CROSS JOIN, joining on calculated expressions, and using subqueries or CTEs
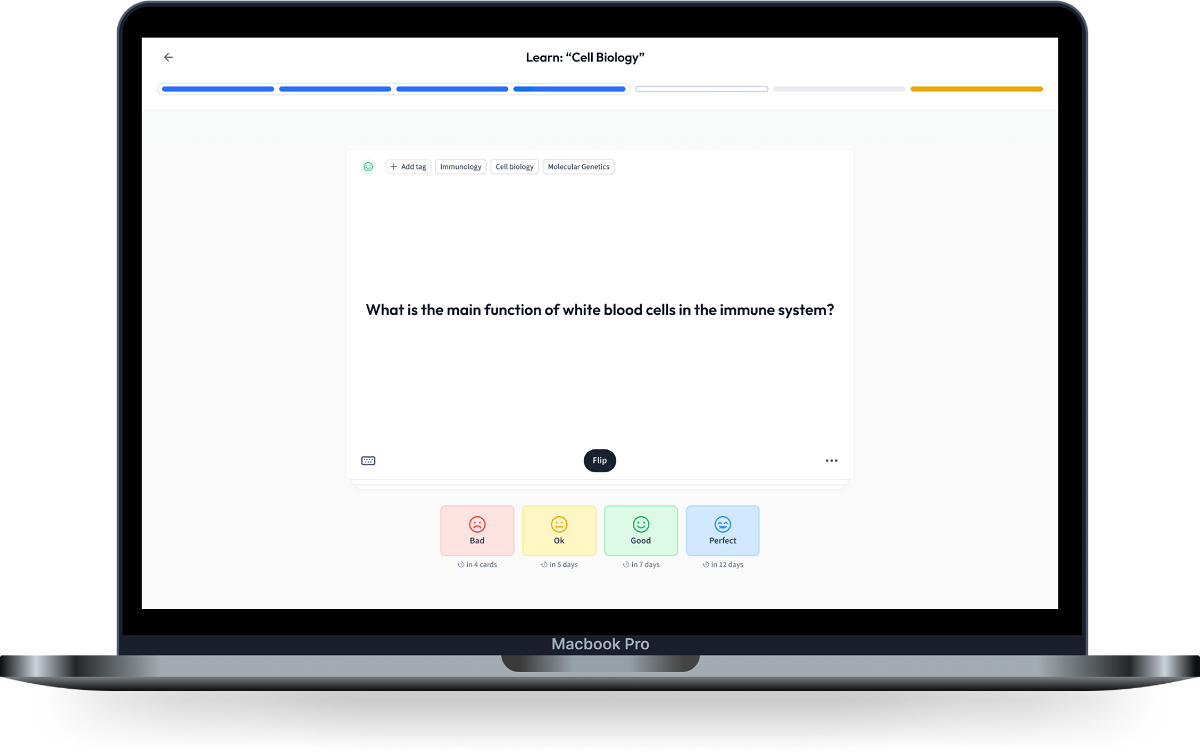
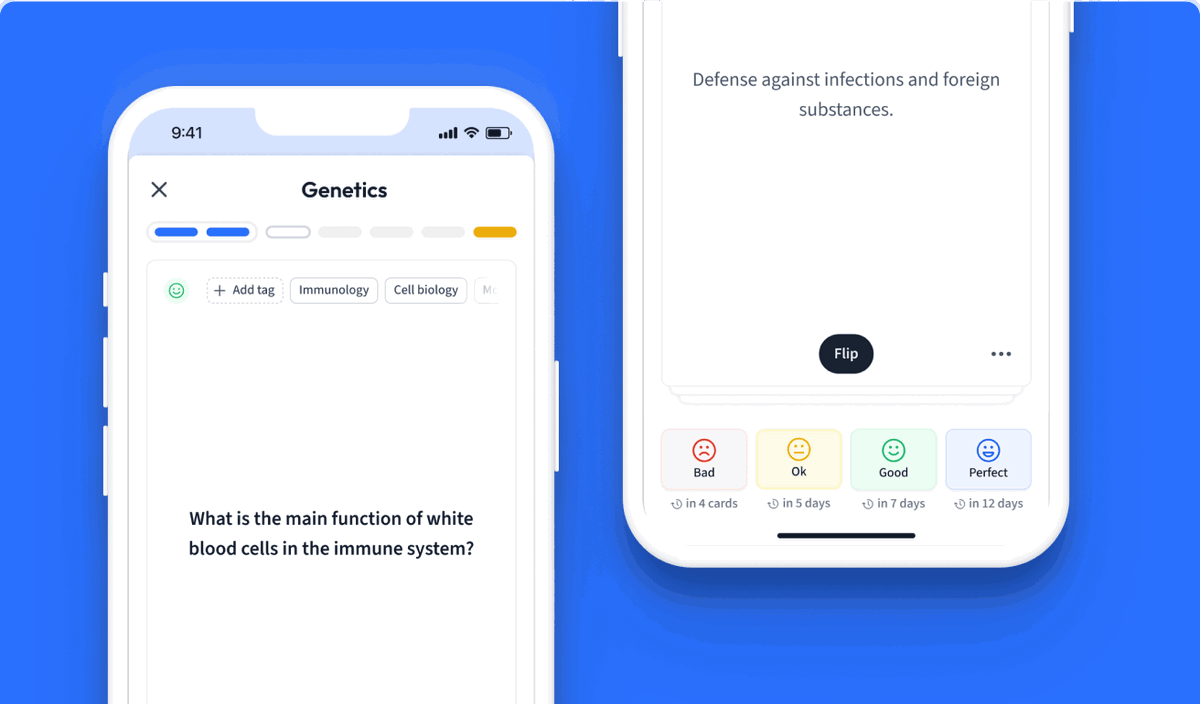
Learn with 11 SQL Join Tables flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about SQL Join Tables
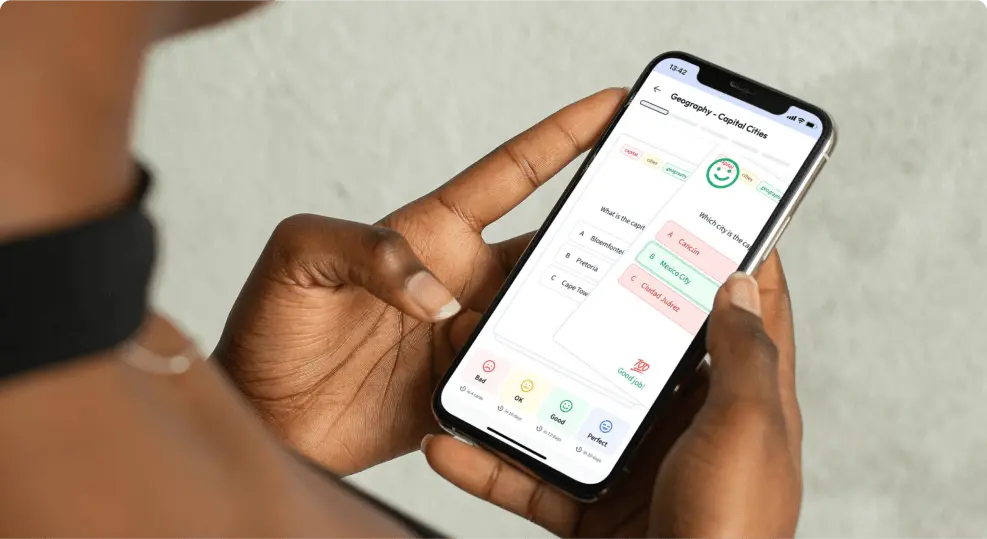
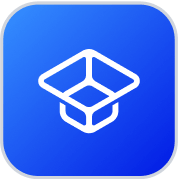
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more