Jump to a key chapter
SQL Transaction Explained
A SQL transaction is a sequence of database operations that behave as a single unit of work. It ensures that multiple operations are executed in an atomic and consistent manner, which is crucial for maintaining database integrity. In the following sections, you will learn about the overview of SQL transactions, its principles, and various types and properties.
An Overview of SQL Transactions
In a database management system, particularly a relational one, it is essential to maintain the consistency and integrity of the data during various operations. SQL transactions are used to properly manage and execute these operations. A SQL transaction starts when the first executable SQL statement is encountered and ends with a commit or rollback. A transaction can include multiple operations, like insertion, deletion, and modification of data in a database.
Transactions have several benefits, such as:
- Controlling the concurrent execution of operations and preventing conflicts among them
- Ensuring data integrity even when an operation fails or a system crash occurs
- Allowing easy recovery from errors and maintaining a consistent state of the database
Transactions provide an essential isolation mechanism to prevent one user's operations from affecting another user's data. This helps maintain the database's consistency even when multiple users are working on the same data concurrently.
Principles of SQL Transactions: ACID
SQL transactions adhere to a set of principles known as ACID, which stands for Atomicity, Consistency, Isolation, and Durability. These principles ensure that transactions are executed correctly and maintain the database's integrity. The ACID properties are:
- Atomicity
- Atomicity ensures that either all operations within a transaction are executed completely, or none of them are. If any operation fails, the entire transaction is rolled back, undoing any changes made by other operations in the transaction. This helps prevent partial transactions from affecting the data integrity.
- Consistency
- Consistency guarantees that the database remains in a consistent state after the transaction is executed. This means that all integrity and business rules are strictly followed, and any erroneous data will not be stored in the database.
- Isolation
- Isolation ensures that the intermediate states of a transaction are invisible to other concurrent transactions. This prevents conflicts among multiple transactions operating on the same data and maintains data consistency.
Types and Properties of SQL Transactions
There are various types of SQL transactions based on their properties and usage. Some common types are:
- Read-only transaction: A transaction that only reads data but does not modify it.
- Write transaction: A transaction that modifies the data, i.e., inserts, updates, or deletes records from the database.
- Distributed transaction: A transaction that spans across multiple databases or systems.
Depending on the isolation level of a transaction, it can have different behaviour and properties to ensure consistency.
- Read uncommitted: This isolation level allows a transaction to read data that has not yet been committed by other transactions. This can lead to issues like dirty reads, non-repeatable reads, and phantom reads.
- Read committed: This level allows a transaction to read only committed data. While this avoids dirty reads, it can still lead to non-repeatable reads and phantom reads.
- Repeatable read: This level ensures that a transaction can read the same data multiple times and get the same result. However, it can still have phantom reads.
- Serializable: This level ensures complete isolation between transactions, preventing dirty reads, non-repeatable reads, and phantom reads.
For example, if a transaction is set to have a repeatable read isolation level, it ensures that the data read by the transaction is the same regardless of whether the transaction reads the data multiple times during its execution. However, it may still experience phantom reads if new rows are added or existing rows are deleted by other transactions.
Understanding SQL transactions, their types, and properties allow you to maintain database integrity and ensure the consistent execution of operations. Always consider the ACID principles when working with SQL transactions, as it helps maintain the overall health of the database.
SQL Transaction Example
In this section, you will discover the basic syntax of SQL transactions and explore some real-world scenarios for their usage. Additionally, you will learn about common issues with SQL transactions and techniques for troubleshooting them effectively.
Basic SQL Transaction Syntax
The SQL transaction syntax is quite straightforward, requiring a series of SQL statements enclosed with transaction control statements. The primary statements used for managing transactions are:
- BEGIN TRANSACTION (or START TRANSACTION)
- COMMIT
- ROLLBACK
BEGIN TRANSACTION marks the beginning of a transaction block, followed by one or more SQL statements that perform data manipulation operations. The TRANSACTION block is terminated by either a COMMIT statement, which saves the changes made within the transaction block to the database, or a ROLLBACK statement, which undoes the process if an error occurs or specific conditions are not met.
For example, consider a bank database with two tables: Customers (customer_id, name, account_balance) and Transactions (transaction_id, transaction_amount, customer_id). To transfer a specific amount from one customer to another securely, you would use a SQL transaction as follows:
BEGIN TRANSACTION; -- Reduce the balance of the sender UPDATE Customers SET account_balance = account_balance - 100 WHERE customer_id = 1; -- Increase the balance of the receiver UPDATE Customers SET account_balance = account_balance + 100 WHERE customer_id = 2; -- Insert a new entry into the Transactions table INSERT INTO Transactions (transaction_amount, customer_id) VALUES (-100, 1), (100, 2); -- Check if the sender's balance is sufficient IF (SELECT account_balance FROM Customers WHERE customer_id = 1) >= 0 COMMIT; ELSE ROLLBACK;
Real-World SQL Transaction Scenarios
SQL transactions are crucial in various real-world scenarios that require multiple database operations to occur atomically and consistently. Below are some common examples:
- E-commerce: When processing an order that includes billing, shipping, and updating the inventory, it is essential to execute these actions as a single transaction to ensure data consistency and avoid potential double bookings, incorrect inventory updates, or incomplete order processing.
- Banking and financial systems: Managing accounts, deposits, withdrawals, and transfers require transactions for ensuring data integrity and consistency while updating account balances and maintaining audit trails of all transactions.
- Reservation systems: For booking tickets or accommodations, the availability of the seats or rooms must be checked, confirmed, and updated in the system. Transactions are necessary for this process to prevent overbooking or incorrect reservations.
- User registration and authentication: While creating user accounts, it is vital to ensure that the account information is saved securely to the correct tables and without duplicates. Transactions can ensure atomicity and isolation of account data operations.
Troubleshooting SQL Transaction Issues
When working with SQL transactions, you may encounter various issues that can stem from improper usage, resource contention, or violation of isolation levels. Here are some common issues and their possible solutions:
1. Deadlocks:Deadlock occurs when two or more transactions are waiting for each other to release the locked resources. To resolve this, you can:- Reorganise the transaction logic and lock sequences consistently across all transactions
- Use timeouts or retries for transactions that cannot acquire the needed locks
- Employ deadlock detection algorithms or tools provided by your database management system (DBMS)
- Select the appropriate isolation level for your transactions
- Use lock hints or strategies as provided by your DBMS
- Choose a more strict isolation level for your transactions, such as Serializable or Repeatable Read
- Use row-level locking, optimisation hints, or snapshot isolation, depending on your DBMS capabilities, to handle concurrency effectively
- Optimise the operations within the transaction to improve the execution time
- Divide the transaction into smaller units if possible
- Monitor and fine-tune database resources and configurations to optimise transaction performance
Understanding and addressing these issues effectively will help you work with SQL transactions more efficiently and ensure that your database operations remain consistent and secure.
Begin SQL Transaction
Starting a SQL transaction is an essential step to ensure atomic and consistent execution of multiple interconnected database operations. Enclosing SQL statements within a transaction allows you to manage these operations as a single unit, enabling data integrity and concurrency control. In this section, you will learn how to initiate a SQL transaction, along with the steps and guidelines for doing so effectively. Additionally, you will explore useful commands like COMMIT, ROLLBACK, and SAVEPOINT that contribute to transaction control and management.
Initiate a SQL Transaction: Steps and Guidelines
To initiate a SQL transaction, follow the steps below:
- Begin the transaction: The process starts by using the BEGIN TRANSACTION (or START TRANSACTION) statement. This marks the beginning of the transaction and signifies that the following SQL statements will be a part of the transaction unit.
- Execute SQL statements: Perform your required data manipulation operations like SELECT, INSERT, UPDATE, or DELETE within the transaction. Ensure that the operations follow proper business logic and do not violate any constraints or consistency rules.
- Commit or roll back the transaction: Based on the success of the database operations and any conditions specified, either COMMIT the transaction to store the changes permanently or ROLLBACK the transaction to undo any changes made during its execution.
While initiating and working with transactions, consider the following guidelines:
- Keep the transactions as short as possible to minimise the risk of deadlocks or other concurrency issues.
- Select an appropriate isolation level based on your application's requirements to prevent unwanted read phenomena and maintain data consistency.
- Ensure proper error handling and recovery mechanisms are in place so that the system can respond to failure scenarios effectively.
Useful Commands: COMMIT, ROLLBACK, and SAVEPOINT
In transactions, the three most important commands used for controlling and managing the execution are COMMIT, ROLLBACK, and SAVEPOINT. Each command serves specific purposes and contributes to the transaction's overall success and consistency. The following sections explain these commands in detail:
- COMMIT
- The COMMIT command is used to permanently save the changes made during the transaction to the database. Once the COMMIT statement is executed, all successful operations within the transaction have been effectively applied, and the transaction is considered complete. It is essential to use the COMMIT statement diligently as committing a transaction too early or too late can lead to inconsistencies and errors in the database.
- ROLLBACK
- The ROLLBACK command is used to cancel or undo the changes made during the transaction. It restores the database to the state it was in before the transaction started, effectively negating all the operations within the transaction. ROLLBACK is used when an error occurs, or when the transaction conditions are not met, allowing the system to revert the changes and maintain the integrity and consistency of the data.
- SAVEPOINT
- A SAVEPOINT is a marker set within a transaction to which you can later roll back, without having to discard the entire transaction. This helps in partially undoing the transaction operations, providing finer control and flexibility during the transaction execution. You can create a savepoint using the SAVEPOINT statement, followed by a savepoint_name. To rollback to the savepoint, use the ROLLBACK TO savepoint_name statement.
For example, consider an SQL transaction that inserts data into multiple tables in an order processing system. If one of the insert operations fails, you might want to rollback only that part of the transaction and let the other inserts continue. By using a SAVEPOINT before the failing operation, when an error occurs, you can rollback to the savepoint and maintain the consistency of the remaining operations within the transaction.
Understanding how and when to use these commands is vital to control SQL transactions effectively, ensuring that your database operations are consistent, error-free, and maintain the integrity of your data throughout the process.
SQL Transaction Replication
SQL Transaction Replication is a method of distributing and synchronising data across multiple databases in real-time. It ensures that any modifications or changes made to a source database (Publisher) are propagated consistently and accurately to the target databases (Subscribers). This replication technique sets high standards for maintaining data integrity, performance, and scalability in various scenarios such as load balancing, reporting, and disaster recovery.
Transactional Replication in Database Systems
Transactional Replication in database systems is accomplished through a set of components and processes that work together to ensure the changes made to a Publisher are propagated to its Subscribers accurately and consistently. Key components involved in transactional replication are:
- Publisher: The source database that holds the original data and distributes it to the Subscribers
- Subscriber: The target databases that receive and apply the changes from the Publisher
- Distributor: A database that acts as an intermediary between the Publisher and Subscribers, storing metadata and the replication history
The core processes of transactional replication are:
- Capture: The modifications made to the Publisher are captured in real-time by log readers and stored as commands in the distribution database.
- Distribution: The captured commands are transmitted from the distribution database to the Subscribers.
- Application: The Subscriber databases apply the commands received from the Distributor, ensuring that their data is consistent with the Publisher.
Transactional replication offers several advantages:
- Real-time data synchronisation, ensuring up-to-date information across all databases
- Increased performance and scalability, as data can be distributed across multiple servers
- Isolation of read-only workload on Subscriber databases, enabling better load balancing
- Support for heterogeneous database systems, including different DBMS platforms or versions
However, it also comes with certain limitations:
- Increased complexity in configuration and maintenance
- Possible latency in data synchronisation during high workloads or network issues
- Resource consumption on the Publisher, Distributor, and Subscriber systems that might impact overall performance
Setting Up Transaction Replication in SQL
Setting up transaction replication in SQL requires a step-by-step approach to ensure proper configuration, security, and performance.
Here is a systematic guide to establish transaction replication in SQL:
- Designate the components: Identify the databases that will serve as the Publisher, Distributor, and Subscribers. Configure the Publisher and Distributor to enable transaction replication.
- Select the articles: Articles are data objects such as tables, stored procedures, or views that will be replicated. Determine which articles in the Publisher need to be replicated to the Subscribers.
- Create the publication: A publication is a set of articles to be replicated as a single unit. Define the publication on the Publisher, specifying the articles, replication mode, and any necessary filters or transformations.
- Configure the distribution: Define the relationship between the Publisher and the Distributor, specifying database names, server connections, and any necessary securities or configurations.
- Configure the subscriptions: Define the Subscriptions on each Subscriber database, specifying the desired publication, the type of subscription (push or pull), and any necessary configurations and securities.
- Monitor and manage replication: Use SQL Server Management Studio or other monitoring tools to track replication performance, troubleshoot any issues, and modify configurations as needed.
When setting up transaction replication, it is crucial to:
- Consider load balancing configuration to prevent performance bottlenecks
- Optimise network connectivity and bandwidth to reduce latency
- Follow security best practices to protect sensitive data and prevent unauthorised access
- Perform regular monitoring and maintenance to ensure optimal replication performance and data consistency
By following these guidelines and processes, you will be able to set up and manage SQL Transaction Replication effectively, providing efficient data distribution and synchronisation across your database systems.
Transact SQL Convert
In SQL transactions, it is often necessary to convert data from one data type to another to facilitate proper storage, retrieval, or manipulation of data. Transact-SQL (T-SQL) provides the CONVERT function to perform data type conversion efficiently and accurately. In this section, you will explore data type conversion in SQL transactions, along with examples and best practices for using the Transact SQL CONVERT function.
Data Type Conversion in SQL Transactions
Data Type Conversion is an essential concept in SQL transactions because incompatible data types can result in data loss, truncation, or runtime errors. T-SQL provides specific functions for data type conversion, CONVERT and CAST, with CONVERT being the focus of this discussion.
The CONVERT function syntax is as follows:
CONVERT (target_data_type, expression [, style])
Where:
- target_data_type represents the data type to which the conversion has to be made
- expression is the value that needs to be converted
- style (optional) represents the formatting style, mostly used when converting between date, time, and string data types
To ensure proper data type conversion in SQL transactions, it is important to:
- Understand the compatibility and implicit conversion rules between different data types
- Choose the correct data type for your columns to avoid unnecessary conversion operations
- Keep in mind the impact of NULL values during the conversion process
- Be aware of the risks associated with explicit data type conversion, such as data loss or truncation
- Follow best practices in handling different styles and format settings for date, time, and string data types
Examples and Best Practices for Transact SQL Convert
In this section, you will encounter practical examples of using the Transact SQL CONVERT function along with best practices to guide you while performing data type conversion.
Example 1: Converting an integer value to a character data type:
SELECT CONVERT(VARCHAR, 12345);
In this example, the integer value '12345' is converted to a character data type (VARCHAR) representation.
Example 2: Converting date data type to character data type with a specific format:
SELECT CONVERT(VARCHAR, GETDATE(), 110);
The GETDATE() function returns the current date and time, with the CONVERT function changing the date data type into a VARCHAR representation in MM-DD-YYYY format (style 110).
Example 3: Converting a character data type representing a date into a date data type:
SELECT CONVERT(DATE, '20-10-2021', 105);
In this example, a string in the DD-MM-YYYY format (style 105) is converted to a DATE data type.
Implement these best practices for Transact SQL Convert:
- Use CONVERT function judiciously: Only use the CONVERT function when necessary and not for every operation, as excessive usage can result in performance overheads.
- Select appropriate styles for date formats: Choose the correct style codes for date and time conversions, considering regional settings, culture-specific formats, and consistency across the application.
- Ensure data type compatibility: Verify the compatibility between the original data type and the target data type before performing conversion to prevent data loss or truncation.
- Handle NULL values properly: When performing data type conversions, consider how NULL values may affect the outcome and take appropriate measures to avoid potential issues.
- Minimise performance impact: Keep an eye on any possible performance impact caused by data type conversion operations, monitoring query execution plans and optimising as needed.
By understanding the Transact SQL CONVERT function and following these best practices, you can perform data type conversion efficiently and accurately, ensuring the integrity of your data and smoother execution of SQL transactions.
SQL Transaction - Key takeaways
SQL Transaction: a sequence of database operations that behaves as a single unit of work, ensuring atomic and consistent execution of multiple operations, and maintaining database integrity.
ACID Principles: Atomicity, Consistency, Isolation, and Durability; properties that guarantee correct execution of transactions and maintenance of database integrity.
Begin SQL Transaction: the initiation of transaction comprising of commands like COMMIT, ROLLBACK, and SAVEPOINT for proper control and management of transactions.
SQL Transaction Replication: real-time distribution and synchronisation of data across multiple databases, ensuring data integrity, performance, and scalability.
Transact SQL Convert: a function for data type conversion, efficiently preventing data loss, truncation, and runtime errors due to incompatible data types.
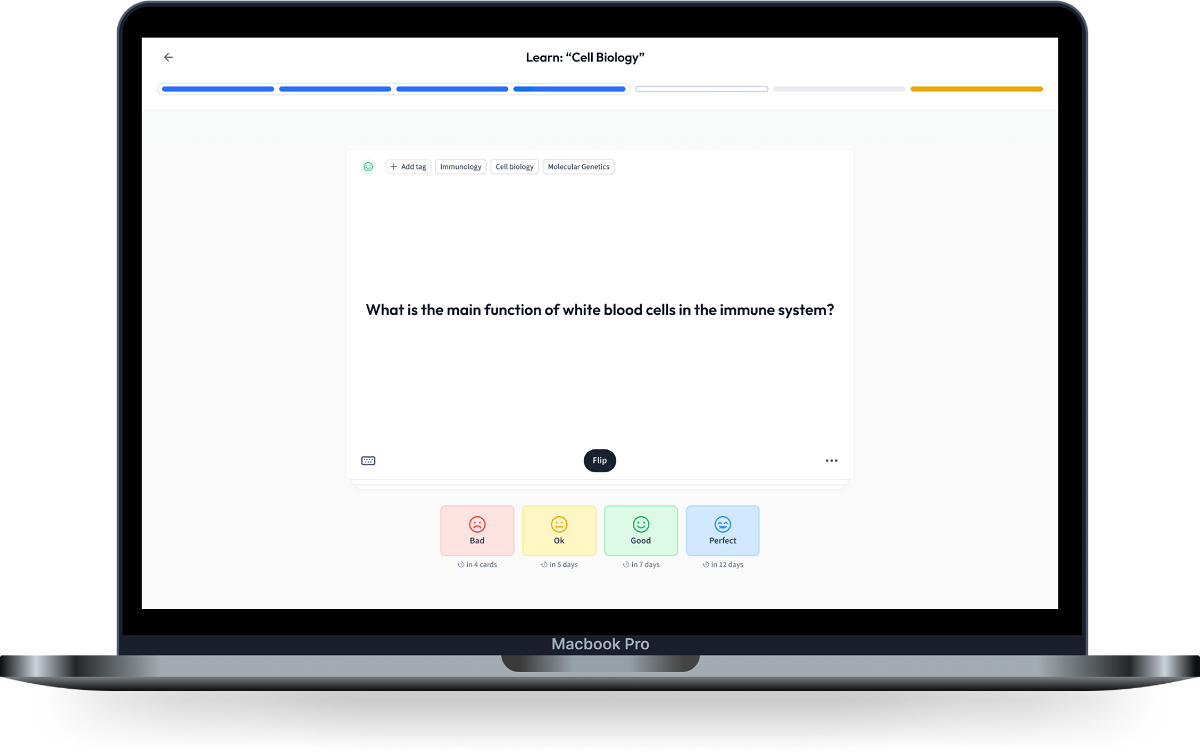
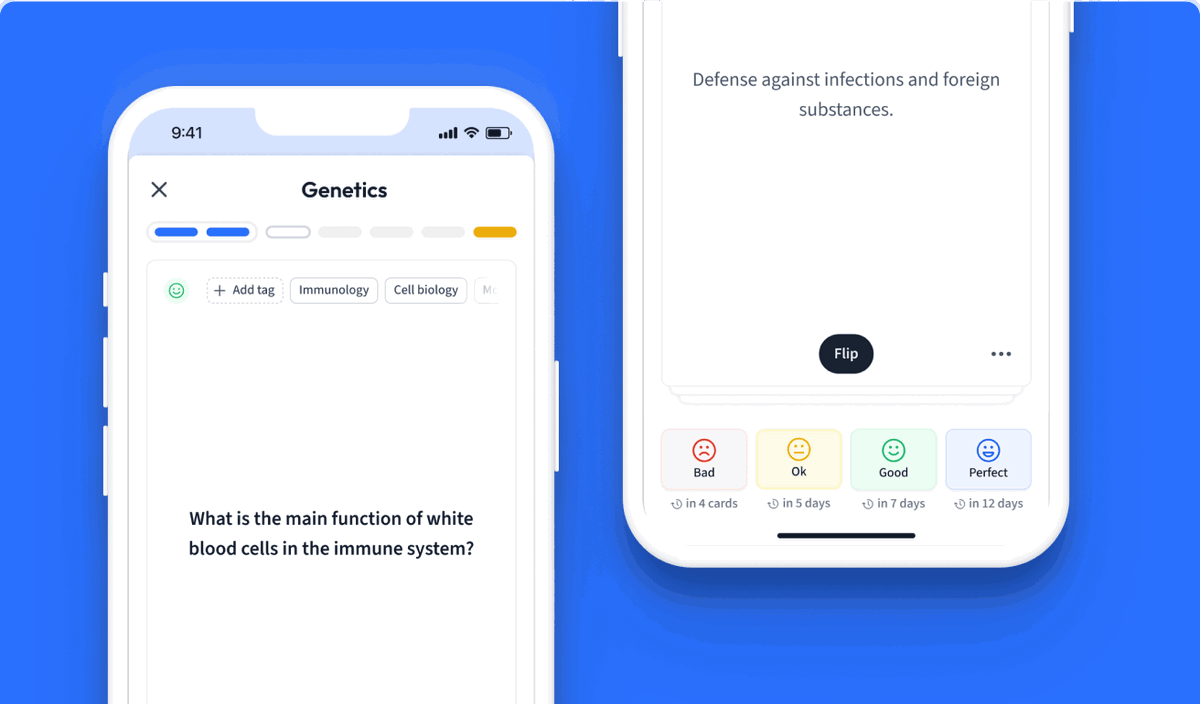
Learn with 13 SQL Transaction flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about SQL Transaction
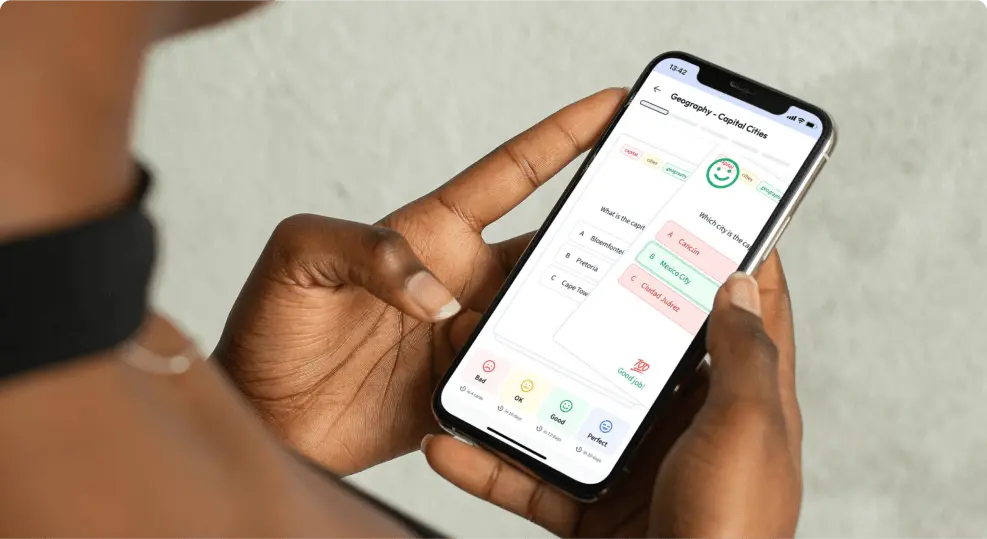
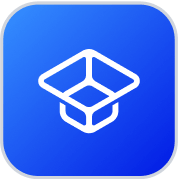
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more