Jump to a key chapter
The Role of UPDATE in SQL
When working with an SQL (Structured Query Language) database, there are several important operations that developers use to manage and manipulate data. One of the critical operations is the UPDATE command. In this section, you will understand the primary role of the UPDATE command in SQL databases and how it helps to maintain up-to-date and accurate information within the database.
UPDATE in SQL is an operation used to modify the existing records in a database. It allows the developers to change specific columns in a row or a set of rows with new information, thus keeping the records updated and accurate.
In SQL database systems, data is organised into tables, consisting of rows and columns. These tables are used to store and retrieve data. Sometimes, the information in a table needs to be updated to ensure that the data remains accurate and relevant. This is where the UPDATE command comes into play, as it allows you to modify the value(s) of one or more columns for a specific row or a set of rows based on a set of conditions.
UPDATE in SQL explained: Modifying data records
Using UPDATE allows you to alter the values of existing records within the table. The syntax for implementing UPDATE in SQL is as follows:
UPDATE table_name SET column1 = value1, column2 = value2, ... WHERE condition;
Here is a breakdown of the syntax components:
- UPDATE: The SQL keyword used to indicate a modification of existing records
- table_name: The name of the table containing the records that you want to modify
- SET: The SQL keyword used to specify the columns to be updated and their new values
- column1, column2, ...: The names of the columns that you want to update with new values
- value1, value2, ...: The new values to assign to the specified columns
- WHERE: The SQL keyword used to define a condition that determines which records to update
- condition: A filter, based on which the specified rows will be updated
Essential UPDATE in SQL commands and syntax
In addition to the basic syntax, there are some additional commands and clauses that you can use with the UPDATE statement for more complex data modifications and scenarios.
Three important SQL clauses that can be used with the UPDATE command are:
- FROM: You can use the FROM clause to reference other tables when updating records in multiple related tables, utilising the JOIN keyword.
- ORDER BY: You can use the ORDER BY clause to sort the rows and apply a sequence for updating the records.
- LIMIT: You can use the LIMIT clause to limit the number of records that should be updated, which is particularly helpful when working with large datasets.
UPDATE in SQL example: Practical illustrations
Let's assume that you have a table called 'employees' with the following columns: 'id', 'first_name', 'last_name', 'salary', and 'job_title'. You want to update the salary of an employee with an id of 5 and set their new salary to 5000.
Here's the UPDATE statement to update the salary for that specific employee:
UPDATE employees SET salary = 5000 WHERE id = 5;
Next, you want to update the job_title for all employees with the 'job_title' as 'Sales Representative' to 'Sales Executive'. The example below demonstrates this:
Here's the UPDATE statement to update the job_title for all Sales Representatives:
UPDATE employees SET job_title = 'Sales Executive' WHERE job_title = 'Sales Representative';
In short, manipulating data records in SQL becomes a breeze with the UPDATE command, ensuring that the data in your database is consistent and up-to-date at all times. Familiarise yourself with the syntax, commands, and examples above to become proficient in the crucial skill of updating records using SQL.
Executing UPDATE in SQL Server
When working with SQL Server, it is essential to understand the critical aspects of configuring and managing the UPDATE process to ensure efficient and accurate data manipulation. This section will delve deep into the critical factors such as transaction management, isolation levels and working with constraints and triggers during the execution of the UPDATE command in SQL Server.
- Transaction management: While executing the UPDATE command, it is crucial to ensure data integrity and consistency. You can achieve this by using transactions. A transaction is a sequence of SQL statements treated as a single, indivisible unit of work in SQL Server. If any part of the transaction fails, the whole transaction is rolled back, ensuring no incomplete data modifications.
- Isolation levels: SQL Server supports several isolation levels, which determine how data interacts with other transactions. The isolation level helps control the transaction's visibility and locking behaviour to avoid problems such as dirty reads, non-repeatable reads, and phantom reads. You can set the appropriate isolation level with the SET TRANSACTION ISOLATION LEVEL statement before executing the UPDATE command.
- Constraints and Triggers: During the update process, you might encounter constraints, such as primary key, foreign key, check, and unique constraints. SQL Server needs to validate these constraints before applying the changes. Additionally, when an UPDATE command is executed, it may activate triggers defined on the table being updated. These triggers perform additional actions defined by the user (such as auditing, data validation, or cascading updates). It is essential to understand and manage these extra validation checks and trigger executions while performing updates in SQL Server.
UPDATE in SQL Server: Performance considerations and best practices
When working with SQL Server, it is important to consider the performance aspects of the UPDATE command execution. This requires understanding different performance optimisation techniques and best practices to avoid locking issues, table scans, and other performance bottlenecks. Below are some key performance considerations and best practices when executing UPDATE commands in SQL Server:
- Optimising the WHERE clause: An efficient WHERE clause minimises the number of rows affected by the update process, speeding up the operation. Using selective filters and efficient indexes can help achieve this.
- Minimising lock contention: Prolonged locks can reduce the system's overall performance by causing contention. Strategies to minimise lock contention include using optimised queries, row versioning and lower isolation levels, or using the SQL Server locking hints, such as NOLOCK, READ COMMITTED, and READ UNCOMMITTED.
- Avoiding unnecessary updates: Updating a column with the same value it already holds can cause performance issues and trigger re-execution. Verify whether the new value is different from the current value before executing an UPDATE statement.
- Using indexes wisely: Indexes can speed up the search process in SQL Server but can also slow down updates if not managed correctly. Try to create selective indexes, only include essential columns and avoid using too many indexes on heavily updated tables.
- Bulk updating: When updating a large number of rows, consider using batch processing techniques with a set number of UPDATE statements or a bulk update method using the BULK INSERT statement or a utility like the bcp utility in SQL Server.
Troubleshooting UPDATE issues in SQL Server
Occasionally, you may face issues with UPDATE commands execution in SQL Server. Identifying and addressing these issues is a critical part of maintaining a healthy and efficient database environment. Below are some common issues you might encounter and how to troubleshoot them:
- Constraint violations: When updating a row, you may accidentally violate a table constraint (such as a foreign key constraint). In this case, carefully review the data changes you are trying to apply and ensure they comply with the existing constraints.
- Trigger issues: If an update causes a trigger to execute and results in an error or undesired outcome, investigate the trigger code for any bugs or incorrect logic specific to the UPDATE command.
- Concurrency conflicts: When multiple users are attempting to update the same data simultaneously, you may experience conflicts. Consider implementing row versioning, optimistic concurrency control techniques or utilising the READ COMMITTED isolation level to address these issues.
- Performance issues: If the UPDATE command is executing slower than expected, identify any potential bottlenecks using SQL Server Profiler, Execution Plans or other SQL Server performance monitoring tools. Apply appropriate techniques, as discussed in the performance considerations and best practices section, to resolve these issues.
- Permission errors: If the UPDATE command results in a permission error, ensure that the user executing the update has the required privileges on the target table. The user might need the UPDATE permission or any other specific permissions to carry out the desired operation.
By understanding and applying the discussed configuration aspects, best practices, and troubleshooting techniques, you can efficiently and accurately execute UPDATE commands in SQL Server to maintain an up-to-date and high-performing database environment.
Mastering UPDATE SQL with JOIN and SELECT
Executing the UPDATE command in SQL becomes more powerful when combined with JOIN and SELECT statements, as it allows you to modify rows in multiple related tables based on sophisticated conditions. This technique is particularly useful for managing complex-relational databases where data is spread across several tables and requires synchronisation during updates.
There are two primary ways to use JOIN and SELECT with UPDATE:
- Performing an UPDATE based on data from another table using SELECT
- Updating multiple related tables using JOIN clauses
UPDATE with SELECT, as the name suggests, involves using the result of a SELECT statement to update the values of specific fields in a table. This method is useful in situations where you need to update a table based on the values in another table or a subquery.
Here's an example of an UPDATE statement using SELECT:
UPDATE employee_salary SET salary = (SELECT new_salary FROM salary_updates WHERE employee_id = employee_salary.id) WHERE EXISTS (SELECT 1 FROM salary_updates WHERE employee_id = employee_salary.id);
This UPDATE statement modifies the salary of each employee in the 'employee_salary' table based on the corresponding 'new_salary' value from the 'salary_updates' table, utilising the INNER SELECT statement to filter the records.
UPDATE with JOIN statements, on the other hand, involves modifying related tables by using a JOIN clause to reference multiple tables during the update process, allowing you to update multiple tables simultaneously. This method is convenient when updating data related to primary and foreign key relationships in the database.
Here's an example of an UPDATE statement using JOIN:
UPDATE employee_salary JOIN salary_updates ON employee_salary.id = salary_updates.employee_id SET employee_salary.salary = salary_updates.new_salary;
In this UPDATE statement, the 'employee_salary' table is updated using a JOIN clause based on the matching 'employee_id' and 'id' fields from the related 'salary_updates' table. The salary values are then updated according to the 'new_salary' column from the 'salary_updates' table.
UPDATE in SQL with SELECT: Applying conditions and filters
When using UPDATE with SELECT in SQL, you can apply various conditions and filters to update only specific records based on more complex criteria. The SELECT statement acts as a source of information for updating the target table, ensuring that you modify only the necessary rows.
Conditions and filters can be added to SELECT statements using clauses such as:
- WHERE: Limit the columns or rows selected based on specified conditions
- GROUP BY: Group rows that have the same values in specified columns
- HAVING: Filter the groups based on a specified condition
- ORDER BY: Sort the selected records based on specified columns
Here's an example of using UPDATE in SQL with a SELECT statement with conditions and filters:
UPDATE products SET price = price * 1.1 WHERE EXISTS (SELECT 1 FROM orders WHERE orders.product_id = products.id AND orders.date > '2022-01-01');
In this example, the product prices are updated by increasing them by 10% only for those products that have been ordered after '2022-01-01', using the EXISTS clause and an INNER SELECT statement with a WHERE condition.
UPDATE in SQL with JOIN and SELECT: Benefits and use cases
Combining UPDATE with JOIN and SELECT provides several benefits and expands SQL's capabilities for handling different use cases. These advantages include:
- Flexibility: Employing JOIN and SELECT with UPDATE enables you to deal with complex data updating scenarios easily, providing flexibility in managing your database.
- Data consistency: Using SELECT and JOIN clauses with UPDATE maintain data consistency by ensuring that the updated values are in sync across all related tables in the database.
- Performance optimisation: When updating large datasets, JOIN and SELECT statements can help optimise the performance of your UPDATE operations by allowing you to apply conditions and filters that focus on specific records.
- Better control: Incorporating JOIN and SELECT gives you better control over the modification process by enabling you to reference multiple tables and update intricate relationships more accurately.
Ultimately, mastering the use of UPDATE with JOIN and SELECT in SQL allows you to take full advantage of the possibilities afforded by SQL for managing complex database requirements and maintaining vital data consistency. This proficiency will enable you to efficiently and accurately modify related tables, ensuring the data in your database remains up-to-date and synchronised always.
UPDATE in SQL - Key takeaways
UPDATE in SQL: an operation used to modify existing records in a database, allowing developers to change specific columns in a row or set of rows based on conditions.
Basic UPDATE syntax:
UPDATE table_name SET column1 = value1, column2 = value2, ... WHERE condition;
UPDATE SQL with JOIN: used for simultaneously updating multiple related tables, typically based on primary and foreign key relationships.
UPDATE in SQL with SELECT: uses the result of a SELECT statement to update the values of specific fields in a table, typically based on conditions or filters.
Configuring and managing UPDATE in SQL Server: involves aspects such as transaction management, isolation levels, constraints, triggers, performance considerations, and troubleshooting.
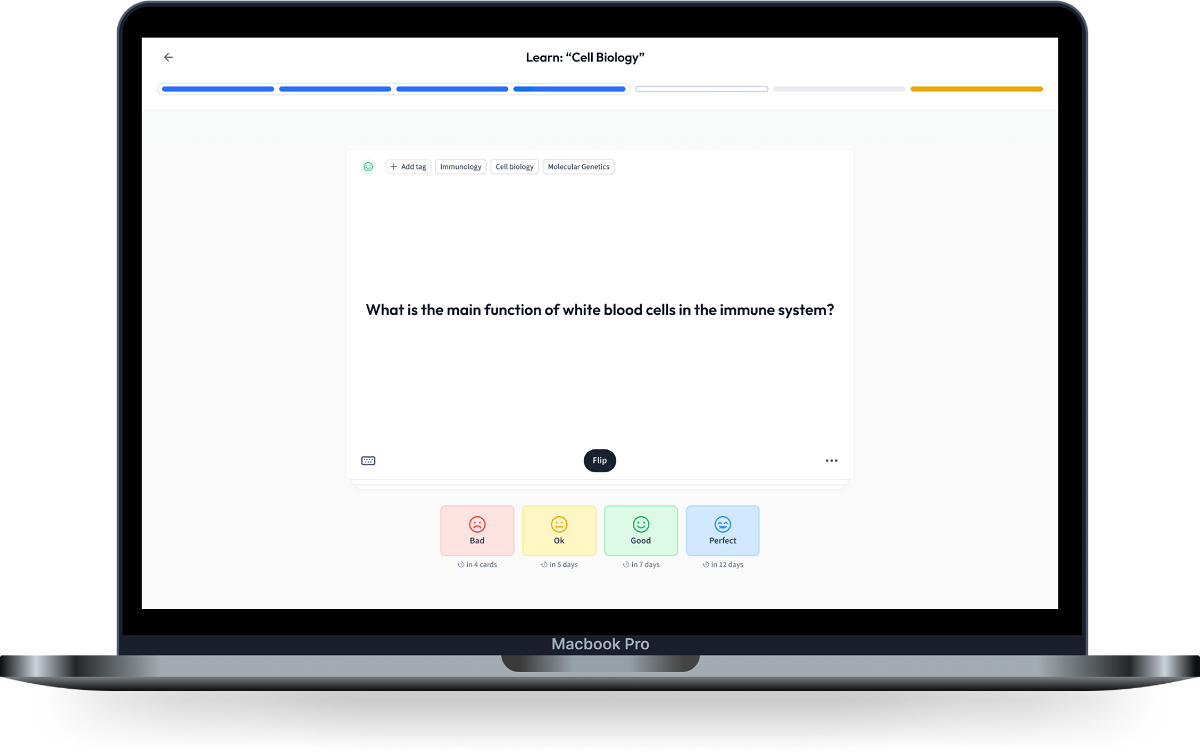
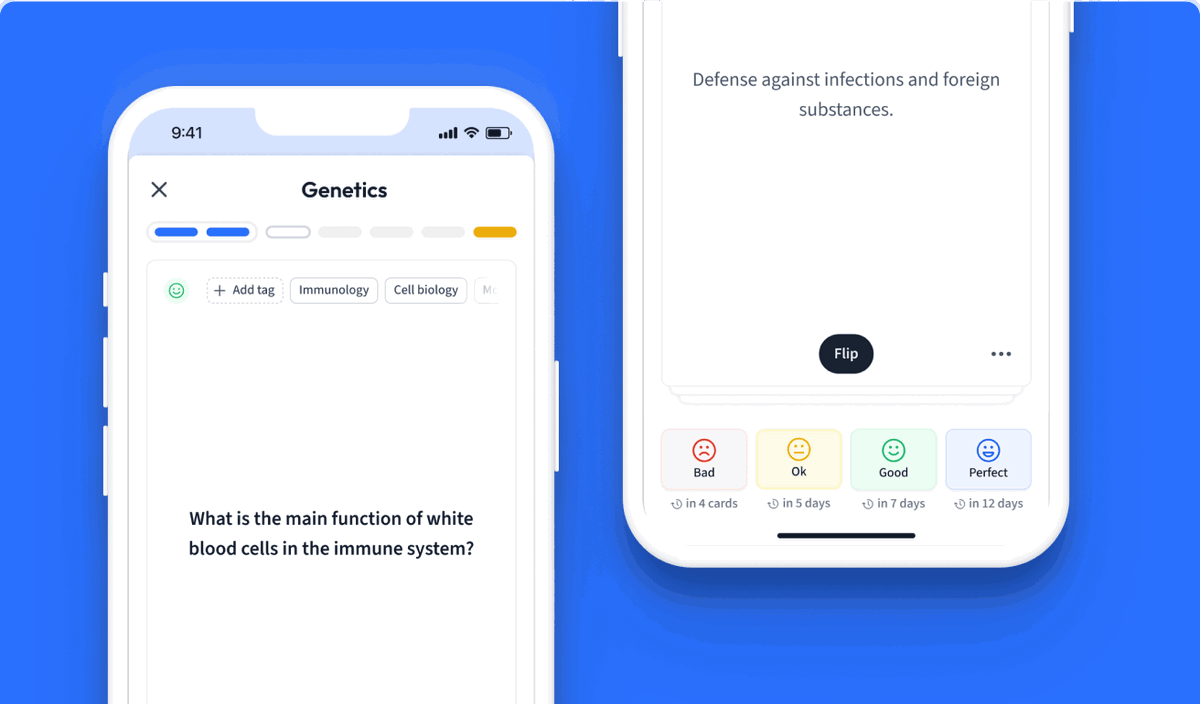
Learn with 15 UPDATE in SQL flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about UPDATE in SQL
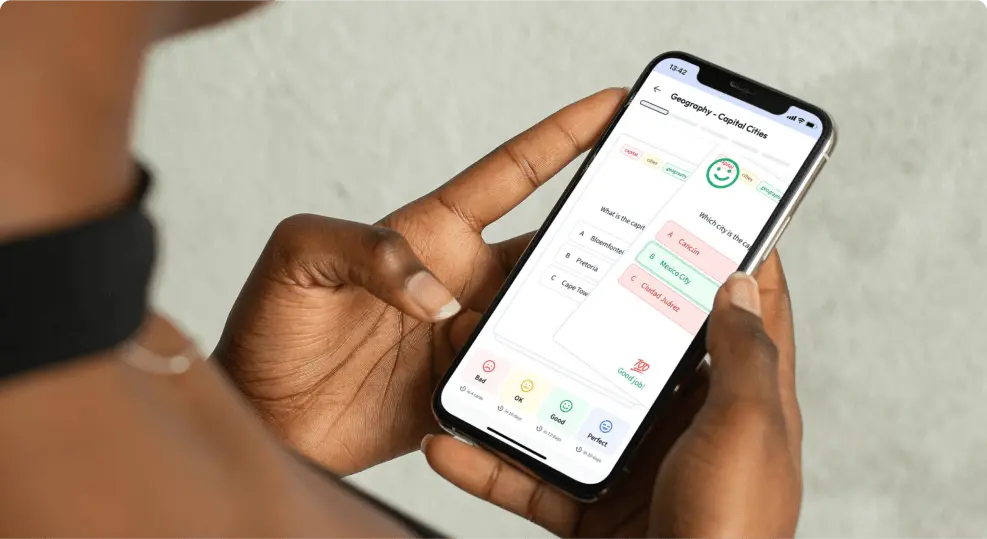
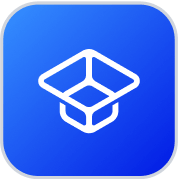
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more