Jump to a key chapter
Introduction to Using Subqueries in SQL Predicates
Using subqueries in SQL predicates is a powerful technique for harnessing the full potential of Structured Query Language (SQL). As a computer science student, mastering subqueries as part of your learning curve will enable you to extract and manipulate data from databases in a more efficient and flexible manner. Questions will inevitably arise during your learning journey, and this article aims to provide a comprehensive understanding of subqueries in SQL predicates along with their importance in data retrieval.
The Concept of Using Subqueries in SQL Predicates Explained
A subquery is a query nested within another SQL query, allowing you to perform complex operations on the data. SQL predicates, on the other hand, are conditional expressions used in SQL statements to filter the results based on specific criteria. Combining subqueries with SQL predicates provides greater flexibility when manipulating data.
A subquery, also called a nested query or inner query, is an SQL query embedded within the main query, which retrieves intermediate results that are used by the outer query. Subqueries usually appear within the WHERE or HAVING clauses of the main query.
There are two types of subqueries:
- Correlated subqueries: These subqueries execute multiple times depending on the outer query results. Correlation exists when there is a relationship between the outer and inner queries.
- Non-correlated subqueries: These subqueries don't depend on the main query and are executed only once. There's no interaction between inner and outer queries.
Consider an online store with a database containing information about products and their prices. You may use a subquery to fetch the most expensive product(s) as follows:
SELECT * FROM products
WHERE price = (SELECT MAX(price) FROM products);
In this example, the inner query calculates the maximum price and the outer query retrieves the records of the products that have that price.
The Importance of Using Subqueries in SQL Predicates for Data Retrieval
Employing subqueries in SQL predicates allows you to perform advanced data manipulation and retrieval techniques, leading to a more efficient and effective way of handling data in relational databases. The key benefits of using subqueries with SQL predicates include:
- Logical simplification: Subqueries provide a logical structure to complex SQL statements, making them easier to read and understand.
- Data consistency: Subqueries ensure data consistency by encapsulating the query logic within a single statement, reducing the risk of errors when updating or modifying the data.
- Increased flexibility: Subqueries enable you to retrieve intermediate results and perform operations on them before using them in the main query, providing greater flexibility in data manipulation.
- Code reusability: Subqueries allow for modular code that can be reused across multiple queries, increasing efficiency and maintainability of your SQL code.
In addition to these benefits, using subqueries in SQL statements can potentially lead to performance improvements. Database management systems (DBMS) create query execution plans to optimize subquery performance. Modern DBMS can efficiently handle multiple subqueries, reducing the need for multiple round trips to the server.
As a computer science student, understanding the concept and practical applications of using subqueries in SQL predicates is essential for becoming an effective database developer, data analyst, or IT professional in general. It is crucial for you to grasp these concepts and apply them while working with relational databases, ensuring you can effectively manage and retrieve data from your databases.
Examples of Using Subqueries in SQL Predicates
In this section, we will dive into several examples of using subqueries in SQL predicates, focusing on single and multiple conditions. We will also explore the implementation of these techniques in real-life scenarios to help you better understand their practical applications.
Using Subqueries in SQL Predicates Example with Single Condition
Let's begin with a simple single condition subquery example. Suppose we have the following table named 'employees':
id | first_name | last_name | salary |
1 | Alice | Smith | 45000 |
2 | Bob | Jones | 55000 |
3 | Charlie | Johnson | 60000 |
If we want to retrieve employees with salaries higher than the average salary, we can use a subquery in the WHERE clause:
SELECT * FROM employees
WHERE salary > (SELECT AVG(salary) FROM employees);
This SQL statement features a subquery inside the WHERE clause, which calculates the average salary. The outer query then retrieves employees whose salaries are greater than the average salary calculated by the subquery.
Using Subqueries in SQL Predicates with Multiple Conditions
Now let's examine a more complex example using subqueries in SQL predicates with multiple conditions. Suppose we have the following two tables named 'orders' and 'order_items':
orders:
order_id | customer_id | date |
1001 | 1 | 2021-07-01 |
1002 | 2 | 2021-07-01 |
1003 | 1 | 2021-07-02 |
order_items:
item_id | order_id | product_id | quantity |
1 | 1001 | 50 | 2 |
2 | 1002 | 50 | 1 |
3 | 1001 | 51 | 3 |
If we want to retrieve all orders placed by a specific customer that include a certain product_id, we can use a subquery with multiple conditions:
SELECT * FROM orders
WHERE customer_id = 1 AND
order_id IN (SELECT order_id FROM order_items
WHERE product_id = 50);
In this example, the subquery retrieves all order_ids from the 'order_items' table that have a product_id of 50. The outer query then retrieves all orders placed by customer_id = 1 that also match the order_ids retrieved by the subquery.
Implementing Using Subqueries in SQL Predicates in Real-Life Scenarios
Effective use of subqueries in SQL predicates can play a vital role in solving real-world database-related challenges. Some real-life scenarios might include:
- Finding the top N items in a category by sales, price, or any other attribute to create business reports or populate dashboards for decision-making.
- Identifying all customers who have purchased a specific combination of products to create targeted marketing campaigns.
- Calculating rankings or percentiles of individual records in comparison to the overall dataset to evaluate employee performance, student results, or product ratings.
- Merging and filtering data from various sources to create consolidated and well-structured reports, making data analysis more efficient and effective.
- Reusable subquery logic can be implemented in stored procedures, functions, and views, increasing the maintainability and modularity of database solutions.
Mastering the use of subqueries in SQL predicates will allow you to tackle a wide range of real-world data manipulation tasks with greater flexibility and efficiency. By understanding how to apply these techniques in multiple conditions and diverse scenarios, you will be better equipped to deliver high-quality database solutions in your future IT career.
Advanced Applications of Subqueries in SQL Predicates
As you progress in your understanding of using subqueries in SQL predicates, you will encounter situations that require more advanced techniques. These can help you solve complex data manipulation challenges and further enhance your ability to optimise database queries. The following sections will elaborate on the implementation of advanced subquery techniques in Oracle databases, as well as on complex subqueries for efficient data management.
Using Subqueries in SQL Predicates in Oracle
Oracle is a popular Relational Database Management System (RDBMS) offering various advanced features for using subqueries in SQL predicates. Let's explore some unique Oracle-specific techniques for creating and managing subqueries:
1. Inline Views: Inline views are subqueries written within the FROM clause, allowing you to use the results as if they were actual tables. This technique can simplify complex queries and provide aliases for easy reference.
Consider a scenario where you have a table called 'sales', and you need to find the top 10 selling products by revenue. You can use the following query:
SELECT product_id, total_revenue
FROM (SELECT product_id, SUM(quantity * unit_price) AS total_revenue
FROM sales
GROUP BY product_id
ORDER BY total_revenue DESC)
WHERE rownum <= 10;
Here, the subquery acts as an inline view in the FROM clause, computing the total_revenue for each product. The outer query then filters the top 10 highest revenue-generating products using the rownum pseudo-column.
2. Scalar Subqueries: Scalar subqueries are single-row, single-column subqueries used in SELECT, WHERE, or HAVING clauses. They return a single value and can be used much like a single expression.
Suppose you have tables 'employees' and 'departments', and you want to retrieve employees with the highest salary in their respective departments. You can use a scalar subquery as follows:
SELECT first_name, last_name, salary, department_id
FROM employees e
WHERE salary = (SELECT MAX(salary) FROM employees
WHERE department_id = e.department_id);
The scalar subquery in the WHERE clause calculates the maximum salary for each department based on the outer query's department_id.
3. The WITH Clause (Common Table Expressions): Oracle allows the use of the WITH clause to define subqueries as common table expressions (CTEs), which act as temporary result sets that can be used multiple times within the main query.
Let's assume you have a hierarchical table called 'employees', with a manager_id column referencing the id column. If you want to retrieve the total number of direct and indirect reports for each manager, you can use a recursive CTE as follows:
WITH reports_cte (manager_id, emp_id, depth) AS (
SELECT manager_id, id, 1
FROM employees
WHERE manager_id IS NOT NULL
UNION ALL
SELECT r.manager_id, e.id, r.depth + 1
FROM employees e, reports_cte r
WHERE e.manager_id = r.emp_id
)
SELECT manager_id, COUNT(*) AS total_reports
FROM reports_cte
GROUP BY manager_id;
This query creates a CTE called reports_cte, which recursively calculates the number of direct and indirect reports for each manager. The main query then returns the count of total_reports for each manager_id.
Understanding these Oracle-specific techniques and proper implementation of subqueries in SQL predicates will help you optimise your database queries and achieve better performance.
Complex Using Subqueries in SQL Predicates for Efficient Data Management
Working with subqueries in SQL predicates in more complex scenarios can prove to be very effective in data management. Here are some complex techniques that will help you make the most of subqueries for efficient data manipulation and handling.
1. Multiple Subqueries: You can use more than one subquery within your main query to work with multiple result sets, which can provide improved flexibility when handling data in different scenarios.
Assume you have tables 'orders' and 'order_items', and you want to retrieve all customers who have placed orders containing products from two specified product categories. You can use multiple subqueries like this:
SELECT customer_id FROM orders
WHERE order_id IN (SELECT order_id FROM order_items
WHERE product_id IN (SELECT product_id FROM products
WHERE category_id = 1))
AND order_id IN (SELECT order_id FROM order_items
WHERE product_id IN (SELECT product_id FROM products
WHERE category_id = 2));
Two subqueries are executed, one for each category_id, with intermediate results used in the main query.
2. Use of INTERSECT, UNION and EXCEPT: When using subqueries, you can combine the output of different queries using the INTERSECT, UNION, and EXCEPT operators to further filter and manipulate the result set.
Considering the same scenario as the previous example, we can use the INTERSECT operator to retrieve customers who have placed orders containing products from both specified categories:
SELECT customer_id FROM orders
WHERE order_id IN (SELECT order_id FROM order_items
WHERE product_id IN (SELECT product_id FROM products
WHERE category_id = 1))
INTERSECT
SELECT customer_id FROM orders
WHERE order_id IN (SELECT order_id FROM order_items
WHERE product_id IN (SELECT product_id FROM products
WHERE category_id = 2));
The subqueries return two sets of customer_ids who have ordered from the specified categories. The INTERSECT operator then retrieves the customer_ids common to both sets.
3. EXISTS and NOT EXISTS: You can use the EXISTS and NOT EXISTS operators in combination with a subquery to apply conditions based on whether the subquery returns any rows.
Following the example of the 'orders' and 'order_items' tables, if you want to find all orders that do not contain items from a specific category, you can use a subquery with the NOT EXISTS operator:
SELECT * FROM orders o
WHERE NOT EXISTS (SELECT 1 FROM order_items oi
JOIN products p ON oi.product_id = p.product_id
WHERE o.order_id = oi.order_id AND p.category_id = 1);
This query uses a correlated subquery with the NOT EXISTS operator to filter out orders containing items from the specified category.
By mastering these complex techniques, you will become adept at using subqueries in SQL predicates for efficient data management and simplify the handling of your database-related tasks.
Using Subqueries in SQL Predicates - Key takeaways
Using Subqueries in SQL Predicates: A powerful technique for complex data manipulation by embedding one query within another, usually within the WHERE or HAVING clauses.
Correlated and Non-correlated Subqueries: Two types of subqueries in SQL. Correlated subqueries rely on the outer query results, while non-correlated subqueries are independent.
Subqueries in Real-Life Scenarios: Practical applications include business reporting, targeted marketing campaigns, performance evaluation, and data consolidation for efficient analysis.
Advanced Oracle Techniques: Inline Views, Scalar Subqueries, and Common Table Expressions (CTEs) offer unique Oracle-specific methods for creating and managing subqueries.
Complex Subquery Techniques: Multiple Subqueries, use of INTERSECT, UNION, and EXCEPT, and EXISTS and NOT EXISTS operators enable efficient and flexible data management in diverse scenarios.
Learn faster with the 15 flashcards about Using Subqueries in SQL Predicates
Sign up for free to gain access to all our flashcards.
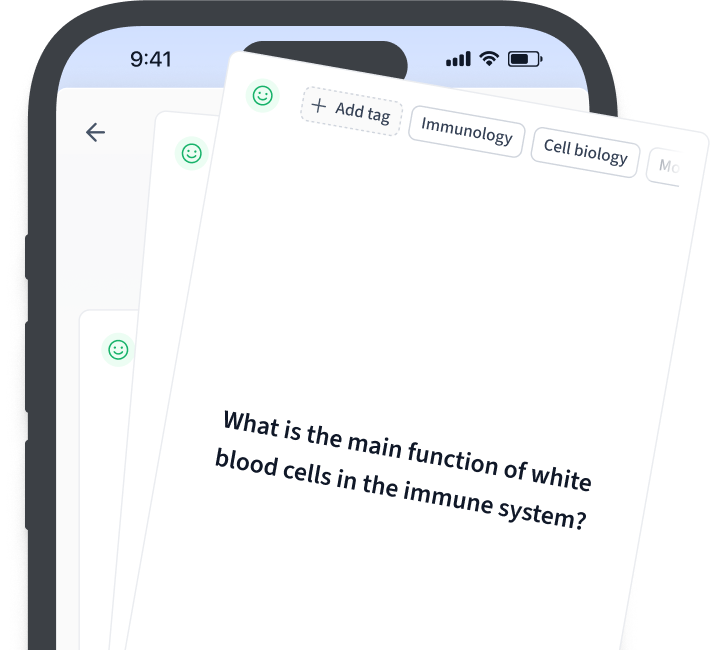
Frequently Asked Questions about Using Subqueries in SQL Predicates
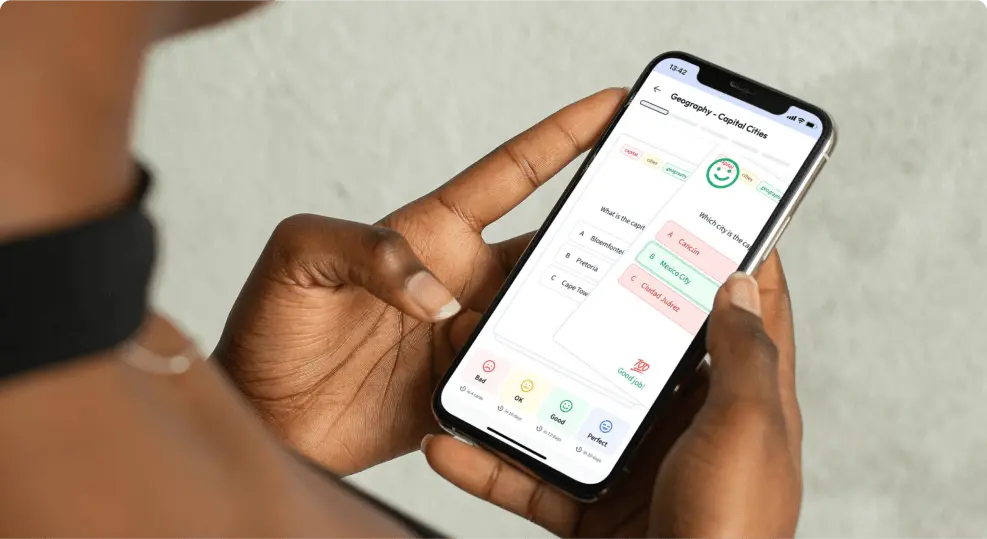
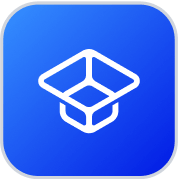
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more