Jump to a key chapter
Then there's also the intriguing realm of complex function theory, where you will get a glimpse into its interactions and the fascinating link between complex function theory and coding. Finally, you will be introduced to the masterly domain of complex network theory that has made substantial contributions to computer science. Understand the intricacies of complex network theory and how it empowers you to unravel the mysteries of networking. It's a journey you wouldn't want to miss – let's get started.
Understanding Complexity Theory in Computer Science
In the field of computer science, understanding Complexity Theory is integral to mastering the discipline. In essence, Complexity Theory is a broad area of study that centers around classifying computational problems in relation to the amount of resources required to solve them.Complexity Theory is a foundational subfield of computer science that explores the inherent difficulty in solving computational problems and characterises them based on the computational resources they require.
Key Concepts of Complexity Theory
Getting to grips with the key concepts within Complexity Theory will provide you with a deeper understanding of its practical implications in computer science. 1. Problem Instances: The generic version of a problem is called a problem instance. For example, the problem of sorting an array is a problem instance. 2. Problem Size: The problem's size is mostly determined by the number of elements in the input. For instance, the size of a sorting problem would be the number of elements to be sorted. 3. Running Time: This refers to the total time taken in executing an algorithm. Usually, it’s expressed as a function of the problem size. Here's an interesting table, that illustrates the running time of different complexities.Complexity | Rate of Growth |
---|---|
O(1) | Constant |
O(log n) | Logarithmic |
O(n) | Linear |
For instance, in the table above, O(log n) signifies logarithmic time complexity. This means that as the problem size doubles, the running time increases approximately by one extra unit of time. An example of this is binary search, where you repeatedly divide the search space in half, until you find your target element.
Role of Computational Complexity Theory
Computation Complexity Theory plays a crucial role in computer science. It helps computer scientists and programmers to develop efficient algorithms and serves as a tool to analyse the performance of these algorithms under different conditions.In particular, it plays a significant role in cryptography where the security of systems depends on the computational complexity of certain problems. A prominent example of this is the RSA encryption system. Its security is based on the fact that factoring large numbers is computationally intensive, making it difficult for unauthorized parties to decrypt the data.
Suppose you're trying to find the shortest route to visit all the major cities in Europe. This seems like a daunting task, yet given a proposed schedule, you can quickly check whether it's feasible or not. This is an example of an NP-complete problem where the solution is difficult to find but easy to verify.
Diving Deep Into Complexity Theory
Complexity Theory represents a key pillar within computer science. Its depth and breadth cover the intricacies of various computational problems and their solutions. Furthermore, it provides critical knowledge concerning the computational resources required for solving these issues.Applications of Complexity Theory in Computer Science
In the practical world of computer science, Complexity Theory finds application in a multitude of areas. It provides the tools necessary to estimate the computational limits and potentials of particular algorithms, ultimately resulting in the optimisation of problem-solving processes. The area of computer programming hugely benefits from Complexity Theory. Here, it assists programmers in gauging the efficiency of their algorithms. The main concern is to develop solutions that execute quickly, use minimal memory, and have low overhead costs.An algorithm's efficiency can be measured by its time complexity, which defines the amount of computer time needed to run an algorithm, and its space complexity, referring to the amount of memory required to complete execution.
Current Research in Complexity Theory
The landscape of Complexity Theory research is wide and diverse, with several fascinating areas currently being explored. One such area is the intricacies of quantum computing - a computational model based on principles of quantum mechanics. There is active research into how complexity classes, such as \( P \) and \( NP \), are affected in a quantum computing environment. Quantum algorithms for factoring numbers, such as Shor's algorithm, and searching unsorted databases, namely Grover's algorithm, have brought new perspectives to well-studied problem classes.For instance, Shor's algorithm, when executed on a quantum computer, can factor large numbers more efficiently than the best-known algorithm on classical computers. Thus, this development has potential implications for cryptographic systems, particularly those relying on the complexity of factoring large numbers, like RSA.
Take, for example, the lesser-known class \( NEXP \), which notates problems where the solution length is exponentially long, and we want to verify a solution in deterministic polynomial time. This class serves as a basis for some interesting hierarchies and definitions, and subdividing this class further is a topic of active research.
Exploring Complex Adaptive Theory
The field of computer science doesn't just deal with straightforward problems. Sometimes, the challenges you face have multiple interacting elements that adapt and evolve over time, which are better understood within the context of Complex Adaptive Systems (CAS). Broadly speaking, CAS is a system in which a perfect understanding of individual parts does not automatically convey a perfect understanding of the whole system’s behaviour.Relevance of Complex Adaptive Theory in Computer Science
In computer science, Complex Adaptive Systems theory provides you with a new lens through which to view and understand certain multi-part dynamic systems. Here, the significance of this theory is not just found in the academic understanding of problem-solving, but also in the real-world applications where such systems commonly occur.A Complex Adaptive System is a 'complex' system comprising numerous 'adaptive' elements that interact and evolve in response to each other and the environment.
Indeed, understanding CAS can aid in deciphering the complex dynamics between multiple learning agents in a shared environment, as they adapt and evolve their policies in response to each other's behaviour. This becomes crucial when designing multi-agent reinforcement learning systems, playing a fundamental role in areas like robotics, game theory, computer networks, and even in enabling autonomous vehicles to navigate traffic.
Practical Examples of Complex Adaptive Theory
There are several illustrative examples in the field of computer science that demonstrate the principles of Complex Adaptive Systems. Consider the case of self-organising networks, commonly seen in wireless sensor networks, where the computational nodes adapt their roles, typically as relays or data sources, based on a mix of factors like network topology, signal quality, and energy constraints.For instance, in a sensor network deployed to monitor environmental conditions, individual sensor nodes adapt their power and relay strategy based on factors such as battery life, number of neighbouring nodes, and signal-to-noise ratio. The whole network self-organizes to streamline communication, ensuring data transmission to the base station with minimal latency and power consumption. Thus, even if individual sensors fail, the network dynamically adapts to maintain functionality – a characteristic hallmark of Complex Adaptive Systems.
The Swarm Intelligence paradigm, inspired by social insect behaviour, is another compelling example. Here, simple agents following simple rules work together to solve complex tasks. Algorithms like Ant Colony Optimization (used for finding shortest paths) and Particle Swarm Optimization (commonly used for numerical optimization problems) are practical computer science applications reflective of CAS.
In these examples, understanding Complex Adaptive Systems Theory is key to appreciating the emergent global behaviour resulting from the local interactions of the individual agents. This perspective helps unlock novel ways to approach and solve computing problems, adding a valuable tool in your toolbox as a computer scientist.
Foundations of Complex Function Theory
Complex Function Theory, also known as Complex Analysis, is a branch of mathematical analysis specifically dealing with functions of complex numbers. This field has a profound influence on various mathematical disciplines from number theory to engineering, adding depth to the understanding and solving of problems in these areas.Delving into the Interactions in Complex Function Theory
The interactions within Complex Function Theory essentially revolve around several core concepts and principles. Efficient interpretation of these enables a holistic understanding of complex numbers' behaviour and their non-trivial function relationships. Firstly, the fundamental notion of a complex number, which extends the real numbers by adding an imaginary unit, forms the very basis of Complex Function Theory. These numbers can be represented in multiple ways, most commonly Cartesian \(a + bi\) and Polar \(re^{i\theta}\) forms.A complex number is a number in the form \(a + bi\) where \(a\) (real part) and \(b\) (imaginary part) are real numbers and \(i\) is the imaginary unit defined by \(i^2 = -1\).
In particular, an important theorem in complex analysis is the Cauchy-Riemann equations. These equations are a set of two partial differential equations which, along with certain continuity and differentiability conditions, provide a characterisation of analytic functions. They are named after Augustin-Louis Cauchy and Bernhard Riemann.
Link between Complex Function Theory and Coding
Complex Function Theory isn't just confined to pure mathematical explorations. It also establishes myriad connections with other areas, including coding, thereby enriching the understanding and effectiveness of these fields. Coding theory, in particular, relies heavily on algebraic structures, and many problems in this area can be translated or analysed using tools from Complex Function Theory. The cornerstone of this link is the understanding of error correction codes, such as Reed-Solomon codes, which are utilised commonly in digital communications to correct errors in data transmission. These are often defined over finite fields, which are algebraic structures related to complex numbers.A Reed-Solomon code is a type of error-correcting code that works by oversampling a polynomial constructed from the data and sending slightly more than the minimum possible information.
If you are trying to send four packets of data 4, 5, 6, 7, you can think of this data as a degree 3 polynomial \(p(x) = 4x^3 + 5x^2 + 6x + 7\). The Reed-Solomon coding would involve evaluating this polynomial at several points \(x = 1, 2, 3, 4\) and sending these values. If one point gets corrupted in transmission, you can use the remaining points to reconstruct the original polynomial and hence recover your original data.
Mastering Complex Network Theory
The sphere of computer science is replete with a multitude of networks, communicating, sharing and interpreting data. Complex Network Theory dives into the study of these networks, their characteristics, behaviour, and the principles governing them. This rich theory, entrenched in mathematics and computer science, begins to unravel when you comprehend the fundamental concepts and correlates they house.Insights into Complex Network Theory and Its Contributions to Computer Science
Complex Network Theory is a vibrant field of study dedicated to deciphering the structure and dynamics of networks that populate real-world systems. It revolves around revealing patterns of connectivity and understanding how these patterns influence the functionality and behaviour of the networked system. At the heart of Complex Network Theory, lies the concept of graph theory:Networks can be represented mathematically using the constructs of graph theory, where a graph is formed of nodes (vertices) representing the elements of the system and edges (links) representing interactions or connections between elements.
- Degree distribution: One primary feature of a network is the degree of a node, which is the total number of connections it has. Understanding the statistics of how these degrees are distributed across all nodes in the network provides significant insight into the network's structure and robustness.
- Clustering coefficient: This measures the tendency of nodes to cluster together. High clustering is often a signature of real-world networks.
- Centrality measures: These provide an indication of the most 'important' or 'central' nodes in the network, based on various metrics like degree, closeness, and betweenness.
Intricacies of Complex Network Theory
Delving deeper into Complex Network Theory, you would encounter some fascinating phenomena and structures ingrained in these networks. A core concept is that of network models, including random, small-world, and scale-free networks, each exhibiting unique arrangements and behaviours. Random networks, as the name suggests, randomly connect nodes, leading to a Poisson degree distribution. Small-world networks, on the other hand, are characterised by high clustering and short average path lengths, capturing the small-world phenomenon often seen in social networks. Scale-free networks, which emerge in many real-world situations, are distinguished by a degree distribution that follows a power law. This means that a few nodes (hubs) have a disproportionately high number of connections.An example of a scale-free network is the internet, where some websites like Google have a vast number of links pointing towards them, while most others have very few. Such networks are robust to random failures but vulnerable to targeted attacks on the hubs.
Understanding these models provides an invaluable toolset to analyse the wide range of networks manifesting in computer science, from data structures and algorithms to distributed computing and beyond. Mathematically, network analysis often boils down to exploring properties of the adjacency matrix, defined as the matrix \(A\) where \(A_{ij} = 1\) if nodes \(i\) and \(j\) are connected and \(0\) otherwise.
Another exciting facet of Complex Network Theory is network dynamics, mainly how networks change and evolve over time. This involves scenarios like nodes and links being added or removed, weights of links changing, or even the process of information or traffic flowing over the network. A profound understanding of Complex Network Theory arms you with an ability to interpret the complex web of connections that form the backbone of many computer science applications. It enhances your ability to design efficient algorithms, recognise network vulnerabilities, and predict the behaviour of complex networked systems.
Complexity Theory - Key takeaways
Complexity theory in computer science is key to enhancing computational skills and problem-solving techniques, and involves understanding the inherent difficulty in solving computational problems.
Computational complexity theory sets benchmarks in the measurement of complexity, ranging from simple algorithms to intricate processes.
Complex adaptive theory influences how countless systems in computer science function with its practical applications.
Complex function theory provides an understanding of its interactions and the link between complex function theory and coding.
Complex network theory contributes substantially to computer science, aiding in the understanding of the intricacies of complex network theory.
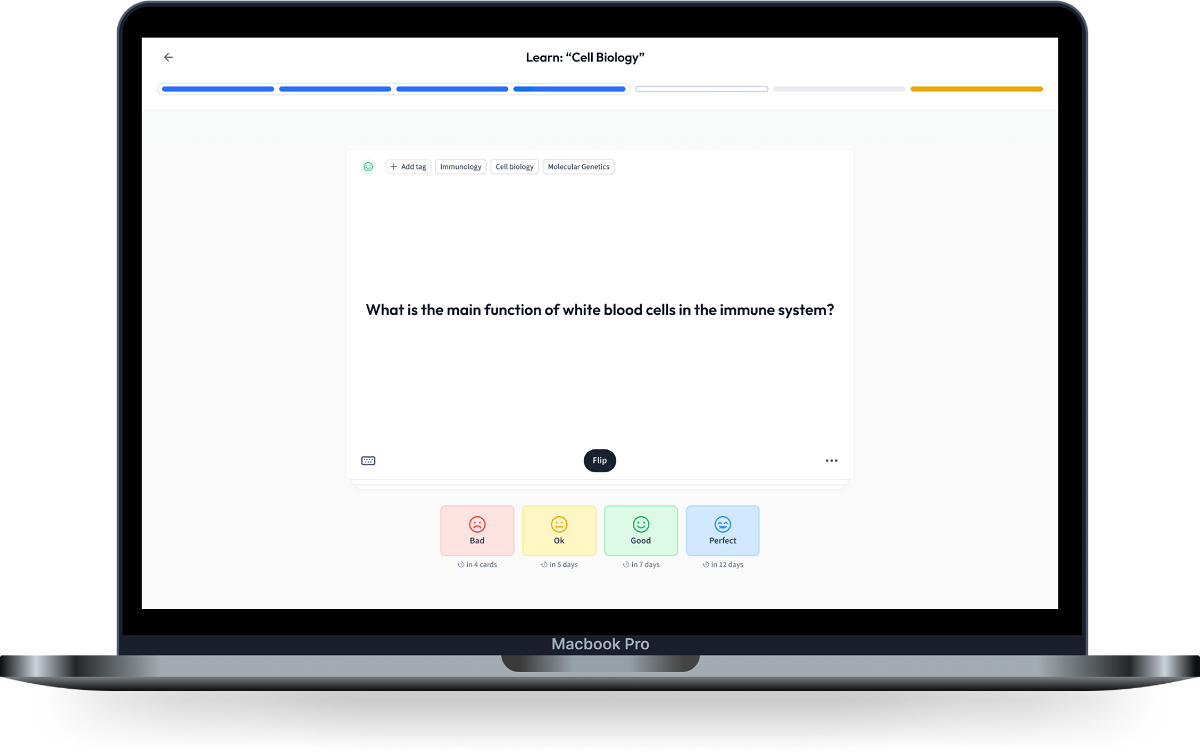
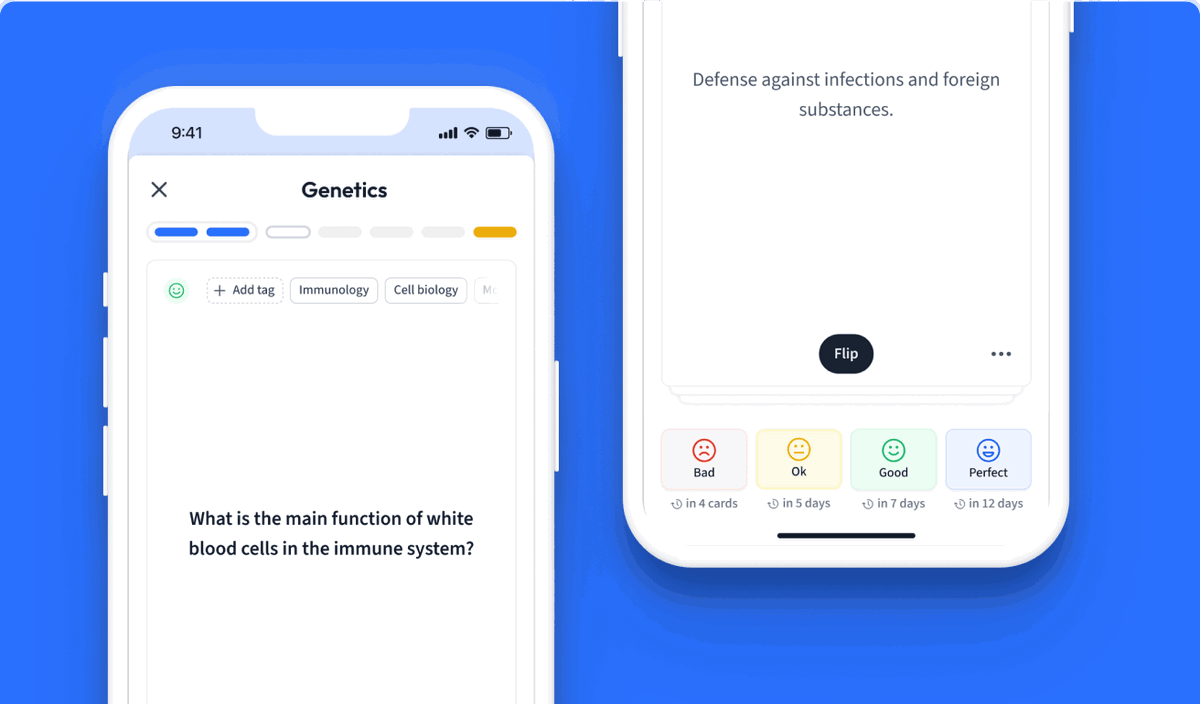
Learn with 15 Complexity Theory flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Complexity Theory
What is computatiional complexity theory?
Who created complexity theory?
Where does complexity theory come from?
What is complexity theory?
What is complex systems theory?
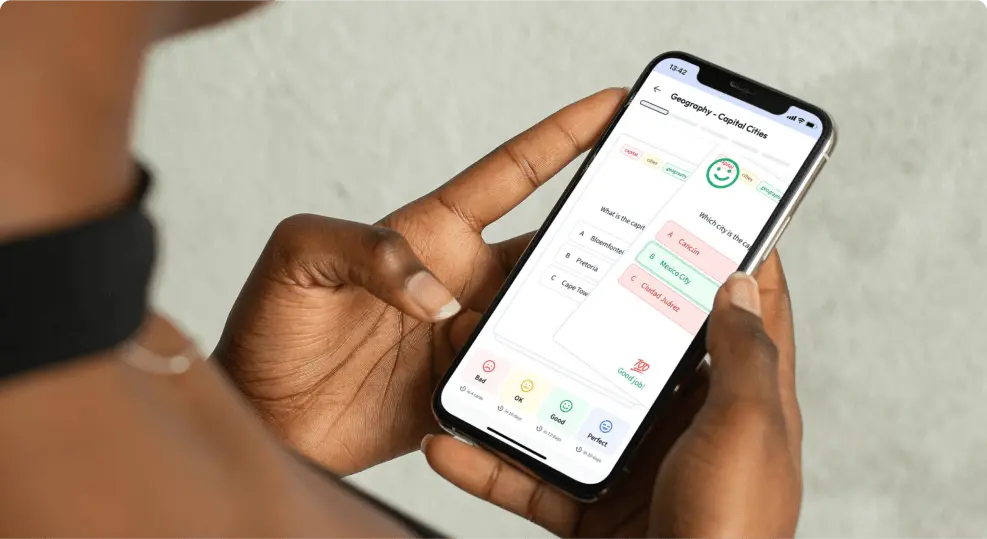
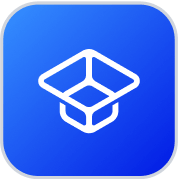
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more