Jump to a key chapter
Understanding NP Hard Problems
Cracking the code of NP Hard problems in computer science has always been an engaging yet challenging task. Before delving into this intriguing subject, let's get a good grip on what NP hard problems actually are.
Fundamentals of NP Hard Problems
In basic terms, NP Hard problems belong to the set of problems for which no polynomial time algorithm is known. Solving such problems take an undetermined amount of time and hence, others categorize it under 'difficult' to solve. However, if a solution to an NP Hard problem is given, it can be verified in polynomial time, making them interesting in the computational world.
The abbreviation NP stands for Non-deterministic Polynomial time. An NP problem is one in which if someone gives you a "guess" at the solution, you can verify whether it's correct or not in polynomial time.
As a key concept in computer science, let's have a look at the fundamentals of NP Hard problems:
- It's important to note that all NP Hard problems can be reduced to each other in polynomial time.
- Whenever a polynomial time algorithm is devised for any NP Hard problem, it implies that we also have a polynomial time algorithm for all problems in the NP set.
- However, if it is proved that no polynomial time algorithm exists for any NP Hard problem, then it denotes no polynomial time algorithm exists for any problem in NP.
Let's take an example to illustrate this. Consider the Travelling Salesman Problem (TSP), which is a classic algorithmic problem in the field of computer science and operations research. It focuses on optimization. In this problem, a salesman is given a list of cities, and he/she needs to determine the shortest route to cover all cities once, and return to the original city. This is a well known NP-hard problem.
Basics and Terminologies Related to NP Hard Problems
Coming to the terminologies, it is crucial to understand the following terms:
Term | Meaning |
---|---|
Algorithm | A well-defined, self-contained step-by-step set of operations to be performed for obtaining a solution to a problem. |
Polynomial Time | The set of computational problems for which an algorithm exists that solves the problem in polynomial time. |
Non-deterministic Polynomial Time (NP) | In computability theory, a problem is in NP if the statement that the problem has a solution can be verified in a polynomial time given the right information. |
NP Complete | The set of decision problems to which all other NP problems can be reduced in polynomial time. |
Differentiating Between NP Hard and Other Computation Problems
It's important to distinguish NP Hard problems from other computation problems to further understand their complexity and implications. Other known categories of computational problems include P, NP, and NP-Complete. An important distinction is that a problem can simultaneously be NP-Hard and NP. Problems that are both NP and NP-Hard are often referred to as NP-Complete.
An NP-Complete problem is a problem whose solutions can be verified in polynomial time, and for which a solution can be discovered in polynomial time given a hypothetical non-deterministic Turing machine.
In the landscape of computational complexity, a fundamental question which remains unanswered is the P vs NP problem. In simple terms, it asks whether every problem whose solution can be quickly checked (NP problems) can also be quickly solved (P problems). If P = NP, then the world of cryptography would undergo a significant shift, as most of the currently used cryptographic algorithms will become vulnerable.
Summarized, below are the main differences between NP Hard and other computation problems:
- P Problems: Problems that can be solved and verified in polynomial time. They are often referred to as tractable or "easy" problems in computational terms.
- NP Problems: The result or output can be checked if it is correct in polynomial time. However, finding these solutions could potentially take a very long time.
- NP-Complete Problems: These are the hardest problems in NP. An NP-Complete problem could be transformed into any other problem in NP.
- NP-Hard Problems: These are at least as hard as the hardest problems in NP. They may not necessarily be in NP and their solutions are not guaranteed to be able to be checked in polynomial time.
Understanding these differences thoroughly helps in tackling the complexities of computer science more efficiently. So, keep solving, and unlock the exciting world of NP Hard
Solving NP Hard Problems
Turning to practical aspects, solving NP Hard Problems could seem like a daunting task. However, with appropriate approaches and algorithmic techniques, these can be dealt with successfully. Here, the focus is on understanding methods to address NP Hard Problems.
Techniques for Solving NP Hard Problems
Multiple techniques have been developed over the years to deal with NP Hard problems. These techniques broadly fall into deterministic and non-deterministic methods.
Deterministic methods include:
- Exact Algorithms: As the name suggests, these are algorithms that find the exact solution for a problem. However, they usually take an exponential amount of time to solve NP Hard problems.
- Polynomial-time approximation algorithms: These are algorithms that provide an approximate solution to the problem in polynomial time. These methods offer a trade-off between running time and the accuracy of the solution - faster running times may lead to slightly less accurate solutions.
Non-deterministic methods include methods such as:
- Probabilistic algorithms: These algorithms employ randomization in their logic and can often find good solutions quickly, but may not always find the optimal solution.
- Heuristic algorithms: These are rule-of-thumb algorithms that can efficiently solve difficult problems, but may not necessarily find the optimal solution. They often involve the making of an educated guess or inferencing.
Choosing the right algorithmic strategy involves understanding the specific problem constraints and choosing a method that offers a reasonable trade-off between computational efficiency and solution accuracy.
Latest Algorithms for NP Hard Problems
Keeping up with the latest algorithms and approaches is crucial to efficiently solving NP Hard problems. These are building blocks on previous methods, with refinements that often make them more applicable to a wider range of problems or make them more effective in solving specific types of issues.
Some of these algorithms include:
- Clever Algorithms: Nature-Inspired Programming Recipes: This is a fantastic resource for heuristic algorithms inspired by processes in the natural world, such as Genetic Algorithms, Swarm Intelligence, and more.
- Ant Colony Optimization (ACO): A technique inspired by the behavior of ant colonies. It repeatedly constructs solutions and updates pheromone trails, which influence the construction of future solutions.
- Jaya: A simple, efficient, and powerful optimization algorithm, applicable for continuous and discrete problems.
- Evolutionary Algorithms (EAs): These methods use mechanisms inspired by biological evolution, such as reproduction, mutation, recombination, and selection. They are effective for solving complex problems where the exact solution methods fail.
Nonetheless, no single algorithm will perform best on all problems. Therefore, the understanding and selection of algorithms must be problem-specific.
Overcoming Challenges While Solving NP Hard Problems
Attempting to solve NP Hard problems can present a few challenges. However, as you understand the problem better, you'll find that these challenges can be navigated and even overcome. Let's walk through a few of the common issues:
Often, the most significant challenge can be that the set of possible solutions to explore is vast, especially for large problem sizes.
The naive approach of exhaustive search would take too much time. However, this can be addressed by:
- Using heuristic or approximation algorithms that find near-optimal solutions much more efficiently than exhaustive search.
- Applying more computational resources, either through parallelization or by using more powerful hardware.
Certain techniques can aid you in overcoming these issues. For instance, when dealing with decision problems, look for certifying algorithms. These are algorithms that, in polynomial time, either produce a witness to prove a 'yes' answer or a counterexample to justify a 'no' answer. In a nutshell, don't give up. Computers and their algorithms are relentless and this trait can be adopted when approaching NP Hard Problems. After all, developing problem-solving skills is as much about tenacity as it is about expertise!
Example of NP Hard Problem
Let's walk you through an example of an NP Hard problem to provide a practical sense of understanding the idea. The Travelling Salesman Problem (TSP) is a classic NP Hard problem wherein the challenge is to find the shortest possible route that allows a travelling salesman to visit every city once and return back to the original city.
Step-by-Step Guide to an NP Hard Problem Example
To understand the nature of NP Hard problems, it's beneficial to break down an established problem and go through it step by step. Let's consider the Travelling Salesman Problem (TSP), which is a well-known NP Hard problem.
Step 1: Define the Problem
The Traveling Salesman Problem is often defined as follows:
Given a list of cities and the distances between each pair of cities, find the shortest possible route that visits each city exactly once and returns to the original city.
Step 2: Understand the Problem Complexity
Due to its combinatorial nature, TSP becomes complex as the number of cities (n) increases. The number of possible solutions (or tours) for the TSP can be given by \( (N-1)! \) where \( N \) is the number of cities. This explains why the problem belongs to the category of NP Hard problems.
Step 3: Formulate It as an Optimization Problem
Next, specify the objective function and constraints mathematically. For the TSP, the objective is to minimize the total traveling distance. If \( d_{ij} \) represents the distance from city i to city j, and \( x_{ij} \) is a binary variable that equals 1 if the path from i to j is part of the tour, the objective can be expressed as:
\[ \text{Minimize } \sum_{i=1}^{N} \sum_{j\neq i,j=1}^{N} d_{ij}x_{ij} \]Subject to the constraints that each city is visited exactly once and the tour is connected.
Step 4: Try Solving the Problem
Depending on the number of cities and computational resources, different techniques can be applied. Exact solvers can be used for smaller scale problems. For larger scale instances, heuristic or approximate algorithms can be implemented. Common methods include greedy algorithms, 2-opt, simulated annealing, and genetic algorithms.
Step 5: Analyze the Results
Analyze the obtained route and total distance. If the applied method does not guarantee an optimal solution, the quality of the solution can be estimated by comparing it with a proven optimal solution (if available) or lower bounds.
Step 6: Adjust and Innovate
If the current approach doesn’t offer satisfactory results, consider tweaking the current method or testing alternative algorithms. Variable neighborhood search or ant colony optimization are other techniques to consider.
Learning from NP Hard Problem Scenarios
To better tackle NP Hard problems, it can be handy to learn from different problem scenarios and solutions. Analyzing different methods used in varied contexts can also boost the learning process, granting a versatile perspective on tackling such problems. Let's take two scenarios for understanding different approaches:
Scenario 1: Travelling Salesman Problem with small number of cities
For a small number of cities (for instance, lesser than 15), an exact method such as exploring all permutations can be viable due to low computational cost. The brute force method, though computationally expensive for larger instances, can be an apt solution for small-scale instances.
- Generate all permutations of the cities.
- Compute the total distance for each permutation.
- Find the permutation with the minimum total distance.
Scenario 2: Travelling Salesman Problem with many cities
As the problem size grows, it becomes untenable to use the brute force approach due to its factorial time complexity. In such cases, heuristic, probablistic or approximation algorithms can be a wiser choice:
- The nearest neighbour heuristic can be a starting point, which builds the tour by repeatedly visiting the nearest unvisited city.
- Metaheuristics like simulated annealing or genetic algorithms can be applied for more sophisticated approaches.
- Approximation methods like the 2-approximation algorithm based on Minimum Spanning Trees can be used to deliver a solution within a factor of the optimum.
Remember, it's not about getting a perfect solution every time, but about understanding and learning the efficient algorithm, manipulating it appropriately as per the problem scale, and coming up with a satisfactory resolution. That's the essence of learning from NP Hard problems scenarios!
Exploring NP Hard Problems Lists
The world of computer science is replete with a multitude of NP Hard problems. They constitute a significant part of combinatorial optimization problems, revealing fascinating characteristics of computational complexity. This complex nature has led to the creation of various lists comprising different NP Hard problems.
A Comprehensive List of NP Hard Problems
In the arena of computational theory, there exist an array of problems that are categorized as NP Hard. By examining these problems, you can appreciate the vast expanse of this particular category in computer science.
Notably:
Being classified as NP hard indicates that a problem is at least as hard as the most difficult problems in NP. Any problem in NP can be reduced to any NP hard problem with a polynomial time algorithm.
Let's take a closer look at an assortment of well-established NP Hard problems:
- Travelling Salesman Problem (TSP): This problem involves finding the shortest possible route for a travelling salesman who needs to visit a set of cities and return to the original city, visiting each city only once.
- Knapsack Problem: Here, one is given a set of items, each with a weight and a value, and a knapsack of limited capacity. The problem is to determine the most valuable combination of items that fit into the knapsack.
- Bin Packing Problem: Given a set of items with different volumes and a collection of bins, the goal is to pack the items into the fewest bins possible.
- Job Shop Scheduling: This problem entails scheduling jobs on machines in a job shop environment where each job has a specific order of operations and each operation has a specified machine it needs to run on.
- Graph Colouring: The problem here is to assign colours to vertices of a graph such that no two adjacent vertices have the same colour, with the aim to minimize the number of colours used.
- Vehicle Routing Problem: Similar to the Travelling Salesman Problem, but includes multiple vehicles (or salesmen) starting and ending at a depot (or home city).
These problems highlight the broad range of applications that NP Hard problems cater to. Despite their seeming complexity, these problems offer profound insights into problem-solving skills and algorithm design.
Understanding Different Types of NP Hard Problems
Peeling back another layer of complexity, NP Hard problems can be further categorized into different types based on certain attributes. This categorization, while not officially acknowledged, serves as an effective way to comprehend the versatility of NP Hard problems and devise apt solutions.
To begin, the common types of NP Hard problems include:
- Decision Problems: These problems pose a question that has a simple 'yes' or 'no' answer. Not all decision problems are NP Hard, but many NP Hard problems are decision problems. Examples include 'Does there exist a travelling salesman tour of a given length?' or 'Can the items be packed into the knapsack without exceeding its capacity?'.
- Optimization Problems: Unlike decision problems, optimization problems seek the best possible solution. An optimization problem could be finding the shortest travelling salesman tour or the most value-packed combination of items for the knapsack.
- Search Problems: Search problems involve finding a solution that meets a certain criteria. For instance, the Vertex Cover Problem, an NP hard problem, requires finding a subset of vertices in a graph such that each edge has at least one of its endpoints in the subset.
It is worth noting that most optimization and search problems have associated decision problems, and these problems are usually related in terms of their computational complexity.
Consider the Travelling Salesman Problem (TSP) as an example. The decision version of TSP asks - 'does there exist a tour of length less than or equal to K?' If you can solve this decision problem in polynomial time, you can also solve the optimization problem in polynomial time simply by doing a binary search over the possible values of K. So, if the decision problem is NP Hard, the optimization problem is also considered NP Hard.
By analyzing different types of NP Hard problems, one gains insight into the characteristics of NP Hard problems and ways to approach these problems systematically, enhancing problem-solving skills in the computational landscape.
NP Hard Optimization Problem
In computer science, NP hard optimization problems occupy an intriguing intersection, where the decision-making landscape of NP Hard Problems melds with the mission for achieving the best possible solution that characterizes optimization. It forms a dynamic class of problems that are both challenging due to their inherent complicatedness and rewarding because they produce the best possible outcomes within given constraints.
The Nexus between NP Hard Problems and Optimization
The relationship between NP Hard problems and optimization offers intriguing insights. Essentially, an NP Hard problem deals with decision problems where the existence of a solution can be verified in polynomial time, yet finding the solution can potentially take a very long time. On the other hand, optimization refers to the process of finding the best solution from all feasible solutions. Therefore, NP Hard optimization problems lie at the intersection of these two areas: you are looking for the best solution (optimization), but that best solution is difficult to find due to the computational complexity (NP Hard).
It's essential to understand that not all optimization problems are NP Hard, and not all NP Hard problems are optimization problems. However, the overlap forms a category of problems that generally deals with getting the optimal solution in a situation where the solution space is exceedingly large and difficult to explore.
An NP Hard optimization problem is an optimization problem for which no known algorithm can find a globally optimal solution in polynomial time, but if a solution is provided, its optimality can be verified in polynomial time.
To grasp this intersection, let's examine a few notable NP Hard optimization problems:
- Travelling Salesman Problem (Optimize the total distance or travel time)
- Knapsack Problem (Maximize the total value within the capacity limit)
- Vehicle Routing Problem (Minimize the total distance travelled by all vehicles)
- Job Shop Scheduling (Minimize the makespan, total tardiness or other objectives)
Consider the Classic Travelling Salesman Problem (TSP). As an optimization problem, it focuses on minimizing the tour’s total distance. As an NP Hard problem, it implies that if you were given a tour, you can quickly compute its total distance and verify if it is less than or equal to a given value. However, finding a tour with minimum distance can take a lot of time due to the exponential number of possible tours.
How to Approach an NP Hard Optimization Problem
Tackling an NP Hard optimization problem can seem intimidating at first glance due to its inherent complexity. However, a systematic, methodical, and innovative approach can be effective in dealing with these challenges.
Assuming the NP Hard Problem is well-defined and understood, the initial step could be determining if the problem size is small enough to consider applying exact algorithms, despite the potentially exponential time complexity. It is feasible for some problems where the problem size is tiny and thus the solution space is small, and it's possible to exhaustively explore all potential solutions.
When dealing with large problem sizes, this approach is not suitable due to prohibitive computational costs.
Instead, heuristic or approximation algorithms are often the tools of choice in such scenarios. This includes, for instance, greedy algorithms, local search algorithms, and metaheuristics. Each algorithm has strengths and weaknesses, so selecting the appropriate one is crucial and dependent on the problem specifics.
A Heuristic Algorithm makes decisions based on the current 'best' available choice, rather than considering all possible options, making approximations for faster solutions. Meanwhile, Approximation Algorithms might not give the best solution, but guarantee a solution that is close to the best possible one. Metaheuristics, on the other hand, are strategies that guide the search process to explore the solution space more efficiently.
Using NP Hard optimization problems can also allow the application of techniques from mathematical programming and operations research. For instance, Integer Programming formulations are possible for many NP Hard problems, and Commercial Integer Programming solvers often use heuristic methods to provide good solutions to these problems.
Finally, don't shy away from innovation. Novel approaches like combing various methods, adjusting parameters, devising new ideas can yield promising results and drive learning to new frontiers. Remember, tackling NP Hard optimization problems is not just about finding the solution but also enhancing understanding about the inherent complexity of problems and various computational methods.
One way to innovate is by using hybrid algorithms. For Example, Genetic Algorithm combined with Local Search in a method known as Memetic Algorithm, which provides an excellent balance between exploration (searching new areas) and exploitation (searching around the current best area). An approach like this could be beneficial when conventional methods alone are unable to yield a satisfactory solution.
Common Algorithms for NP Hard Problems
As you delve deeper into the labyrinth of NP Hard Problems, it's essential to become familiar with the range of algorithms suitable for attending to these complex challenges. Algorithms represent systematic approaches that help address NP Hard Problems efficiently and offer a robust structure for navigating the complex landscapes of such problems.
When it comes to NP Hard problems, several potent algorithms have been used to tackle these puzzling conundrums. These algorithms range from deterministic techniques that systematically explore the solution space to probabilistic or heuristic algorithms that make educated guesses or approximations to efficiently find good solutions.
Let's delve into some of the widely used algorithms for solving NP Hard problems:
- Deterministic algorithms: As the name suggests, these algorithms operate in a predictable manner and provide the exact solution to the problem. If the problem size is relatively small, deterministic algorithms like Brute Force or Divide and Conquer can find the exact solution in a reasonable time. However, these algorithms are typically not feasible for large-scale NP Hard problems.
- Approximation algorithms: Approximation algorithms deliver solutions that are close to the optimal but not necessarily the best solution. These algorithms have a guaranteed worst-case performance, expressed as the ratio between the value of the solution produced by the algorithm and the optimum value. Examples include the Greedy Algorithm and the Primal-Dual Schema.
- Heuristic algorithms: Heuristics utilize rules of thumb to find good enough solutions within reasonable timeframes. Although they cannot guarantee an optimal solution, they are often used for sizeable NP Hard problems due to their efficiency. Classical examples of heuristics include Hill Climbing, Tabu Search, and Simulated Annealing.
- Metaheuristic algorithms: Metaheuristics represent higher-level heuristic strategies that guide other heuristics to find optimised solutions by exploring and exploiting the solution landscape. Genetic Algorithms, Ant Colony Optimization, and Particle Swarm Optimization are popular metaheuristics for dealing with NP Hard problems.
- Hybrid algorithms: Hybrid algorithms involve combining two or more algorithms for problem-solving, capitalizing on the strengths of each to achieve better performance. An instance of this includes the integration of local search heuristics into Genetic Algorithms to form Memetic Algorithms.
While different algorithms come with their unique strengths and weaknesses, the choice largely depends on the specific problem requirements and constraints, offering a balance between computational efficiency and solution quality.
Guide to Implementing Algorithms for NP Hard Problems
If you're gearing to tackle NP Hard problems, here's a practical guide to implementing algorithms for these problems. Remember that implementing these algorithms involves understanding the problem, choosing an appropriate algorithmic strategy, implementing it, and interpreting the results. These steps can be iterative until the solution meets the requirements.
Step 1: Understand the Problem
This step involves getting a robust grasp of the problem you're aiming to solve. Clarify the problem constraints, the feasible solution space, and the performance measures to assess the quality of the solution.
Step 2: Choose an Appropriate Algorithm
Depending on the problem complexity and size, choose an algorithm that suits your problem best. Consider the algorithm's strengths and shortcomings, computational complexity, and potential to meet your problem constraints.
Step 3: Implement the Algorithm
At this stage, you put your hands to work and translate the chosen algorithm into computer code. If you're using a popular algorithm, chances are there are already library functions or packages available in programming languages like Python, Java, or C++. If you're implementing the algorithm from scratch, ensure it follows the algorithm’s steps accurately.
Coding Example |
---|
python # Python code implementing a greedy algorithm for the knapsack problem def greedy_knapsack(weights, values, capacity): # Step 1: Compute value-to-weight ratios ratios = [v/w for v, w in zip(values, weights)] # Step 2: Sort items by value-to-weight ratio in descending order sorted_indices = sorted(range(len(ratios)), key=ratios.__getitem__, reverse=True) # Step 3: Add items to the knapsack until it becomes full total_value = 0 for i in sorted_indices: if weights[i] <= capacity: capacity -= weights[i] total_value += values[i] return total_value |
Step 4: Test the Algorithm
Test your algorithm on various test cases to ensure that it works correctly. You may start with simpler cases (e.g., small instances of the problem) to ascertain the algorithm’s correctness. Thereafter, shift focus to more complex cases or edge cases to examine its robustness and reliability.
Step 5: Analyse the Results
Interpret the results of your computation. Keep in mind the performance measures defined in Step 1. If the solution doesn’t meet your requirements, tweak your implementation or algorithm, or even try a better-suited algorithm. Remember, it's not always about finding the 'best' solution but finding a solution that meets the problem constraints in a reasonable amount of time.
What's pivotal here is continuous learning and adaptive thinking. As you gain experience implementing various algorithms for different NP Hard problems, you'll start developing an intuitive sense of picking suitable strategies and foreseeing potential challenges.
NP Hard Problems - Key takeaways
NP Hard Problems are a set of problems in computer science, for which no polynomial time algorithm is known. Their solution can take an undetermined amount of time, making them challenging to solve. However, if a solution to an NP Hard problem is provided, it can be verified in polynomial time.
The abbreviation NP stands for Non-deterministic Polynomial time. It refers to a problem wherein if someone provides a "guess" at the solution, this solution can be verified in polynomial time.
Key facts about NP Hard Problems: They can be reduced to each other in polynomial time. If a polynomial time algorithm is devised for any NP Hard problem, it implies that similar algorithms exist for all problems in the NP set. Conversely, if it's proved that no polynomial time algorithm exists for any NP Hard problem, then no polynomial time algorithm exists for any problem in NP.
The Travelling Salesman Problem (TSP) is a classic example of an NP Hard problem. It involves a salesman needing to determine the shortest route to cover all cities once and return to the original city.
Main differences between NP Hard and other computation problems: P Problems can be solved and verified in polynomial time, whereas NP Problems can be verified in polynomial time but may take a long time to solve. NP-Complete Problems are among the hardest problems in NP set, and can be transformed into any other problem in NP. Finally, NP-Hard Problems are at least as hard as the hardest problems in NP, and their solutions are not guaranteed to be verifiable in polynomial time.
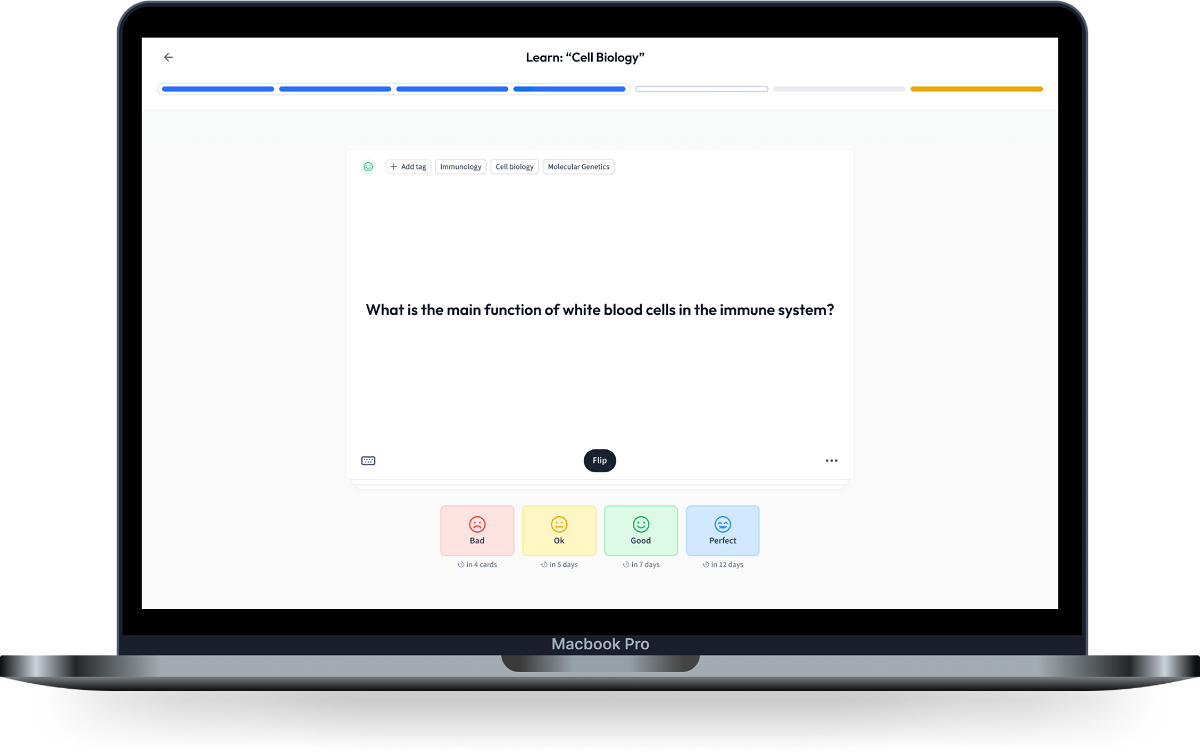
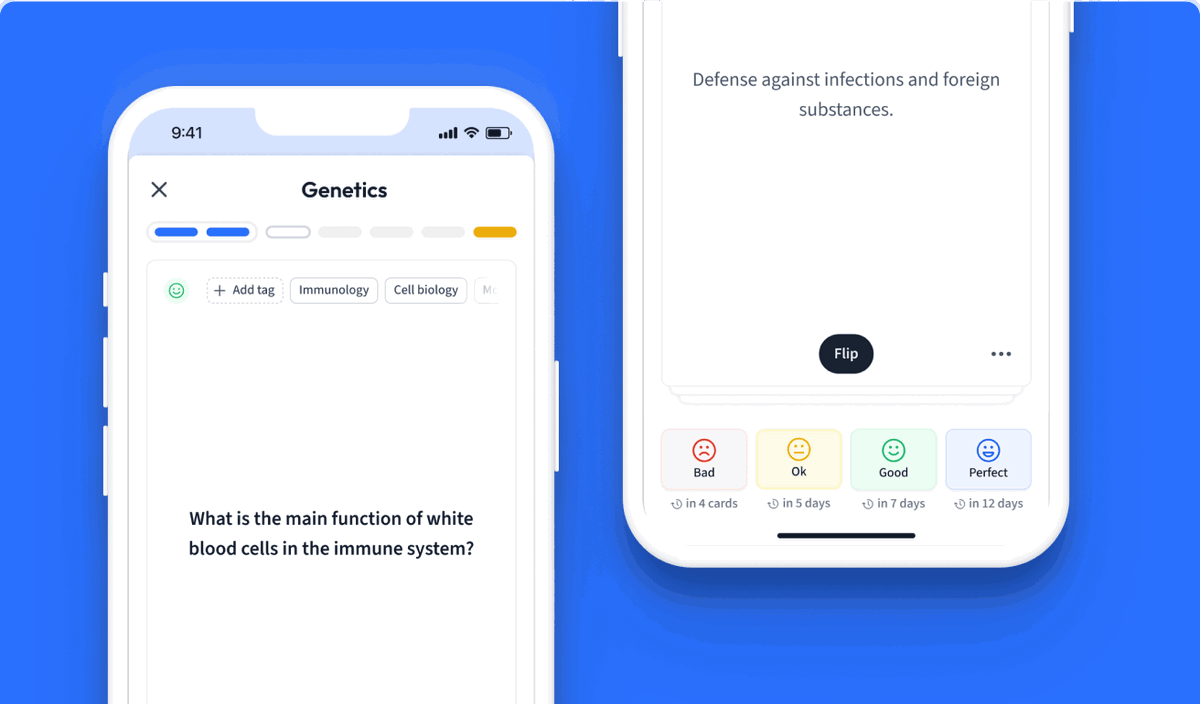
Learn with 18 NP Hard Problems flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about NP Hard Problems
What are np hard problems?
Are all np complete problems np hard?
How to solve np hard problems?
What is np hard problem in algorithm?
Can np hard problems be solved?
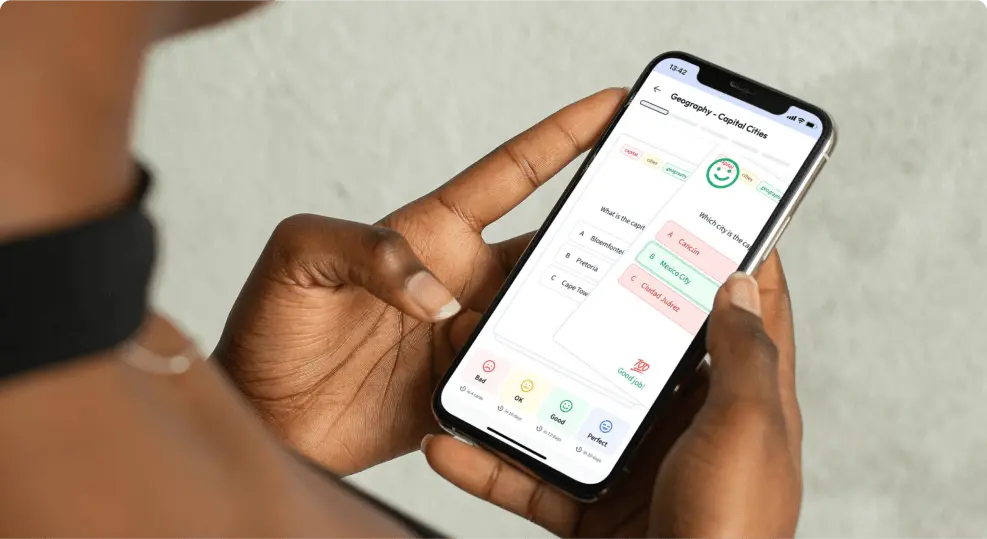
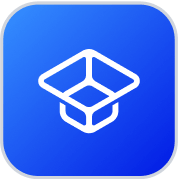
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more