Jump to a key chapter
Understanding Pushdown Automata in Computer Science
Pushdown Automata are an intricate part of theoretical computer science. As a fundamental aspect of automata theory, it helps to shape your understanding of computational methods and techniques. In this section, you will embark on a comprehensive journey to understand the complexity and beauty of Pushdown Automata within the realm of computer science.Basic aspects of Pushdown Automata
Pushdown Automata is a type of automaton that employs a stack-based memory model. It is commonly used for the representation and design of compilers in computer languages.
Primary function of Pushdown Automata
The primary function of a Pushdown Automaton is to accept a Context-Free Language (CFL). This is achieved through the stack operation which allows the automaton to keep track of the application state dynamically.An illustrative example is your journey through a maze where you take different paths (the input) and place a mark (push into the stack) at each junction. When you reach a dead-end (no available action for the current state), you retreat to the last junction (pop the stack) and try a different path (change state). The maze is solved (input accepted) when an exit path is found (a specific final state is reached).
Essential elements of Pushdown Automata
The Pushdown Automata structure is composed of several essential elements. Let's explore these elements in the table below:Elements | Description |
---|---|
States | Define various operational conditions of the automaton |
Input Symbols | Inputs which the automaton accepts |
Stack Symbols | Symbols that can be pushed and popped in the stack |
Stack | Memory model which holds inputs based on LIFO (Last In, First Out) |
Transition Function | Determines the new state, based on current state, input symbol, and top of the stack |
Initial and Final States | Starting state and the state(s) in which the input string is accepted |
Unpacking Pushdown Automata Theory in Practice
While the theory around Pushdown Automata might seem complicated, practical examples can help illustrate these complex concepts in a more digestible manner.Elucidating Pushdown Automata Theory with examples
Let's assume a scenario where a Pushdown Automata is used to determine if parentheses in an expression are balanced. This is a good example since it involves input symbols, state changes, and stack operations.For example, the expression '((()))' would be accepted by the Pushdown Automata, while the expression '(()()(' would be rejected. This is because for each '(', a corresponding ')' should exist. The Automata pushes every '(' it encounters into the stack. When it encounters a ')', it pops '(‘ from the stack. If the stack is empty when the Automata reads the end of the input, then the string is accepted; else, it is rejected.
Understanding deterministic and non deterministic Pushdown Automata
Pushdown Automata are categorized into two types - deterministic and non-deterministic. A deterministic Pushdown Automata (DPDA) has only one move in each condition, whereas a non-deterministic Pushdown Automata (NPDA) can have multiple moves.Although theoretically, NPDAs are more powerful, most real-world applications use DPDAs because the latter can efficiently process the deterministic context-free languages often found in programming languages and compilers.
Visualising Pushdown Automata through Diagrams
In computer science, theoretical concepts like Pushdown Automata often benefit from visual representation. Diagrams can aid in understanding their operation and functionality. In this section, you will gain a conceptual understanding of Pushdown Automata Diagrams and learn how to read them competently.Introduction to Pushdown Automata Diagram
A Pushdown Automata Diagram is a visual tool used for expressing the operations and state transitions within a Pushdown Automaton. Pushdown Automata Diagrams make use of circles to signify states, arrows to symbolise transitions, and labelled stack functions indicating the actions of pushing or popping from the stack.States in the diagram are represented by circles. Each circle is labelled with a state name. Transitions are depicted as arrows connecting the states. The labels on these arrows represent the conditions for transitions.
Key components in a Pushdown Automata Diagram
The following points outline the crucial components within a Pushdown Automata Diagram:- States: Presented by circles, denoted by distinct labels, with the initial state generally sporting an entry arrow.
- Transitions: Illustrated as arrows linking different states, labelled with conditions on which the transition occurs.
- Stack operations: Featured alongside transition arrows, they specify whether a symbol will be pushed into or popped out of the stack.
- Final state(s): The state(s) where the input string is accepted, often denoted by a double circle.
Reading and understanding a Pushdown Automata Diagram
Reading a Pushdown Automata Diagram involves understanding the moves made based on different conditions. A common condition representation format is \(a, b \rightarrow c\), where \(a\) is the input symbol, \(b\) is the stack's top symbol, and \(c\) is the symbol that replaces the stack's top symbol. If \(c\) is \(\epsilon\), it means the topmost stack symbol is popped.Consider a transition labelled as \(1, Z \rightarrow XY\), where 1 is the input symbol, Z is the state's present stack symbol, and XY is the new stack symbol that replaces Z. It shows that on input 1 and if the stack's top symbol is Z, the Automaton will replace the topmost stack symbol with XY.
- Final state: Acceptance upon reaching a final state.
- Empty stack: Acceptance when the entire input has been processed, and the stack is empty.
Examples of Pushdown Automata Diagrams
In the spirit of 'a picture paints a thousand words', examining examples can be the most effective way of understanding Pushdown Automata Diagrams.Diagrams illustrating deterministic Pushdown Automata
A deterministic Pushdown Automata Diagram is relatively simple, as it depicts just one state change for any specific input.Consider a DPDA with two states A and B that accepts strings with equal numbers of 0's and 1's. In state A, 0 pushes Z; in state B, 1 pops Z. When all 0's and 1's are balanced, it returns to the state A and accept the string if the last symbol to be popped from the stack is Z (which means all symbols have been matched).
Diagrams demonstrating non deterministic Pushdown Automata
Non deterministic Pushdown Automata Diagrams are more complex and sophisticated as they could have multiple transitions for the same input symbol.Imagine an NPDA that accepts strings where the number of a's are equal or more than the number of b's, like 'aaabb', 'aab', 'ab', etc. There will be multiple pathways from the initial state to the final state, each depending on whether an 'a' is read and pushed onto the stack or whenever a 'b' is read and 'a' is popped from the stack. When all 'b's are accounted for, all remaining 'a's on the stack are popped, and if the string ends with stack symbol Z, this string is accepted.
Exploring Types of Pushdown Automata
Pushdown Automata (PDA) is categorised into primarily two types in computer science: Deterministic Pushdown Automata (DPDA) and Non-Deterministic Pushdown Automata (NPDA). Understanding these categories is crucial for exploring the extensive capabilities and applications of Pushdown Automata.Differentiating between Deterministic and Non-Deterministic Pushdown Automata
It's essential to discern between Deterministic and Non-Deterministic Pushdown Automata. Both types share the primary features of states, input symbols, and stack operations. Yet, their transition functions diverge significantly, affecting how they process input and progress through states.Deterministic Pushdown Automata: Explanations and Examples
Deterministic Pushdown Automata can be defined by six components:A DPDA is a 6-tuple \( (Q, Σ, Γ, δ, q0, F) \) where \( Q \) is a finite set of states, \( Σ \) is an input alphabet, \( Γ \) is a stack alphabet, \( δ \) is the transition function, \( q0 \) is the start state, and \( F \) is the set of accept states.
To illustrate, consider a DPDA that accepts the language of even-length palindromes. When it reads a symbol \( x \), it pushes \( x \) into the stack. If the following input matches the stack top, it pops \( x \). If all inputs are read and the stack is empty simultaneously, the input is accepted.
Non-Deterministic Pushdown Automata: Explanitions and Examples
Non-Deterministic Pushdown Automata shares the same tuple representation as DPDAs. However, as implied by the name, NPDAs can have multiple possible transitions for the same input symbol, depending on the stack’s topmost symbol.An NPDA is a 6-tuple \( (Q, Σ, Γ, δ, q0, F) \) in which all components have the same meaning as in a DPDA. The key difference lies in the transition function \( δ \), allowing more than one next move for a given state, input symbol and stack symbol combination.
An example can illustrate an NPDA's function: imagine an NPDA that accepts the language of palindromes over the alphabet {0, 1}. When it reads a symbol \( x \), it either pushes \( x \) into the stack or enters a state where it attempts to match the remaining inputs with the stack content. In the matching state, if there is an input-match-stack-top scenario, it pops the top. If all inputs are read and the stack is empty simultaneously, the input is accepted.
Other Types of Pushdown Automata
Beyond the primary types, there exist some less common variations of Pushdown Automata, modified to obtain specific capabilities or operating behaviours. Understanding these nuances expands your breadth of knowledge around this intricate subject matter.Learning about less common variations of Pushdown Automata
Some lesser-known variations of Pushdown Automata include:- Visibly Pushdown Automata (VPA) - Push and pop operations explicitly defined in the input symbols.
- Input-driven Pushdown Automata - Stack operation decided by the current input symbol, disregarding the stack's top symbol.
- Counter Automata - Instead of a symbol stack, it uses a counter to record values.
While these are rarely used in mainstream computer science, each offers a unique approach to solve specific computational problems. Understanding their concepts offers an enhanced, comprehensive view of automata theory.
Practical applications of different types of Pushdown Automata
Understanding different types of Pushdown Automata helps in applying them correctly according to situation needs.- DPDAs find applications in designing deterministic context-free programming languages and parsers. Its simplistic and straightforward nature allows for easier debugging and quicker running time.
- NPDAs are utilised in the design of compilers and syntax checkers where multiple transitions for the same condition might occur, offering flexibility and increased computing power.
- VPAs and Input-driven PDAs are used in analysis and verification of recursive program execution.
- Counter Automata are used in modelling and analysing systems with a finite number of repetitive processes.
Pushdown Automata - Key takeaways
Pushdown Automata is a type of automaton that uses a stack-based memory model and is widely applied in the representation and design of compilers within computer languages.
Pushdown Automata characteristically contains an extra stack component that holds a string of inputs, upon which push and pop operations occur subject to certain rules.
The operational Purpose of Pushdown Automata is to accept a Context-Free Language (CFL) via stack operations, thus dynamically keeping track of the application state.
Pushdown Automata is composed of several key components - States, Input Symbols, Stack Symbols, Stack, Transition Function, and Initial/Final States.
Two major types of Pushdown Automata are deterministic and non-deterministic, with a deterministic Pushdown Automata (DPDA) having only one move per condition and a non-deterministic Pushdown Automata (NPDA) capable of multiple moves.
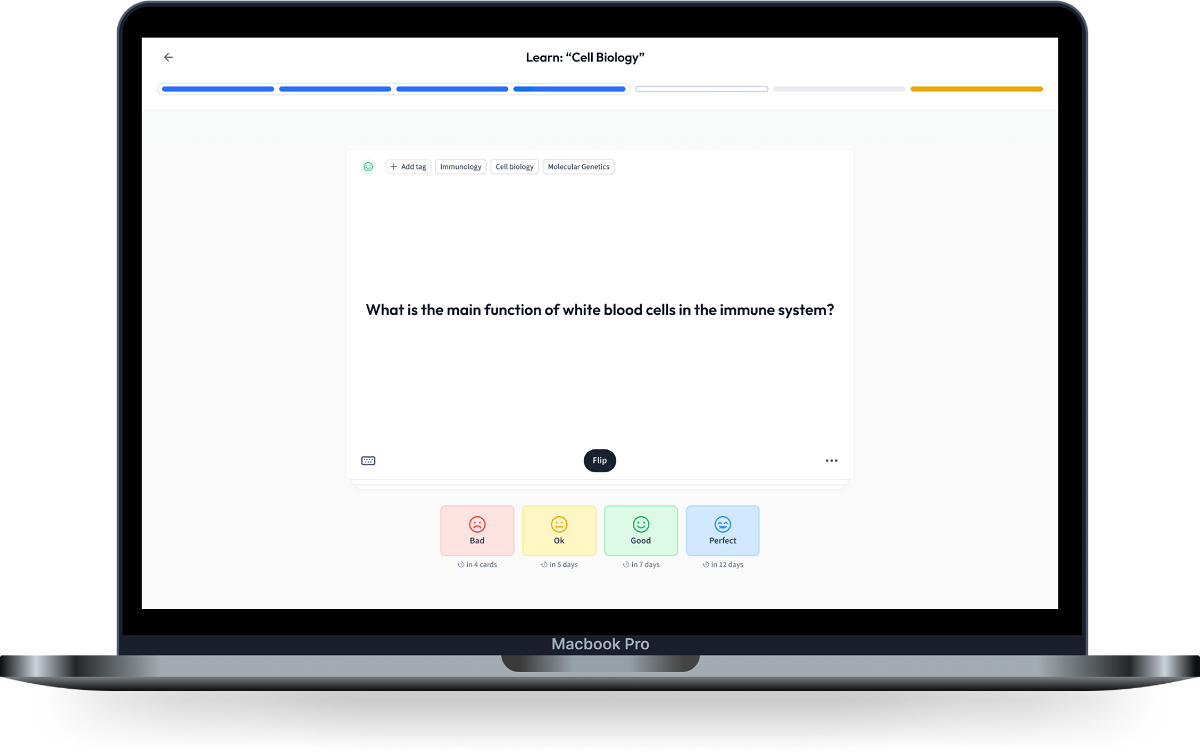
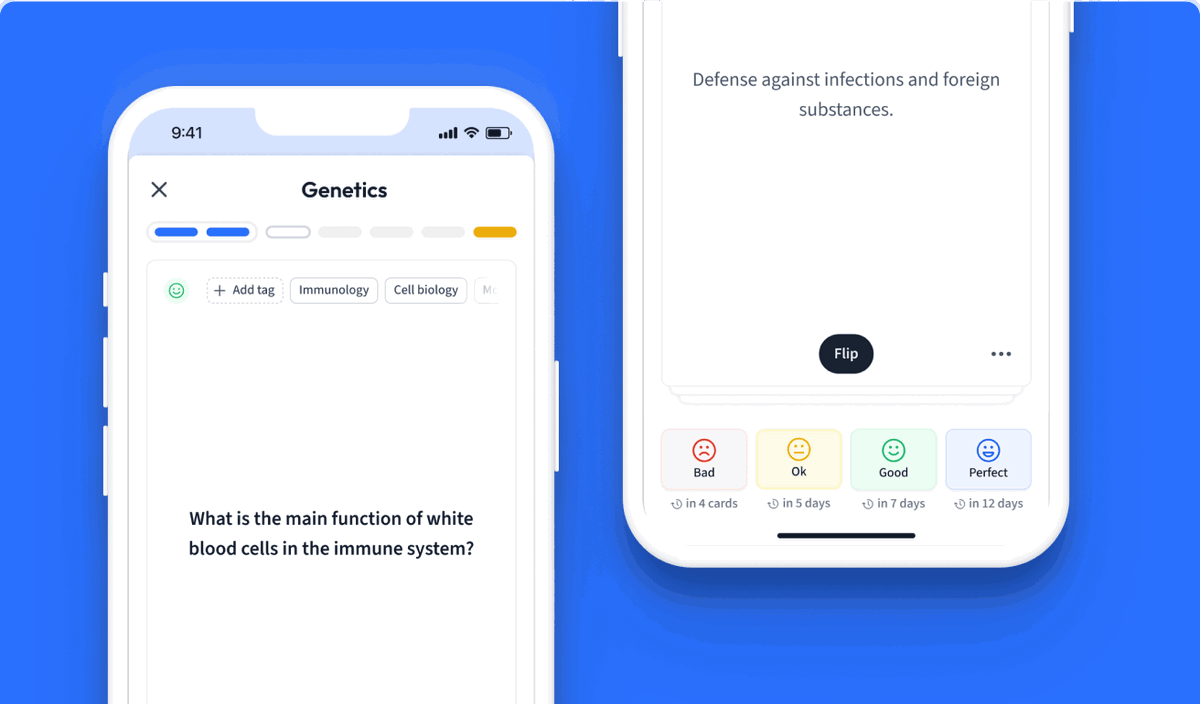
Learn with 15 Pushdown Automata flashcards in the free StudySmarter app
Already have an account? Log in
Frequently Asked Questions about Pushdown Automata
What is a pushdown automata?
How to construct a pushdown automata?
Can you use pushdown automata for regular languages?
How is a pushdown automata different from a finite automata?
Why do we use pushdown automata?
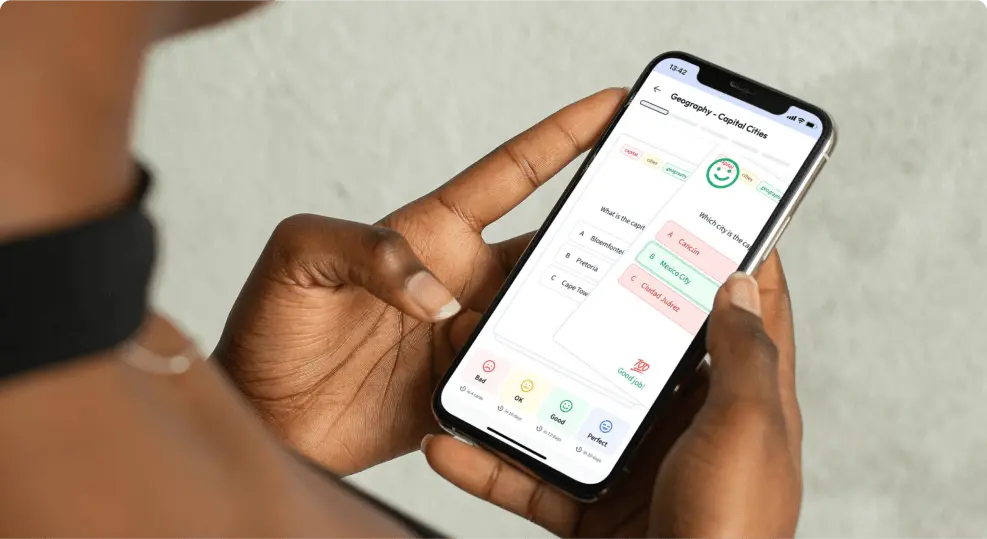
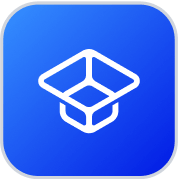
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more