Jump to a key chapter
Regular Expressions Definition
Regular expressions, often abbreviated as regex or regexp, are sequences of characters that define a search pattern. They are widely used for finding and manipulating text or data.
What are Regular Expressions?
Regular expressions are powerful tools used in programming and text processing to specify search patterns. They match sets of strings and are commonly applied for tasks such as finding specific text patterns, validating input, and replacing text in strings.
Regular Expressions: Special sequences of characters that help in finding, matching, and managing patterns in text.
In practical terms, regular expressions are used to:
- Validate input: Ensuring data like email addresses are formatted correctly.
- Search and replace: Finding specific text patterns and replacing them with alternative text.
- Data extraction: Extracting particular pieces of information from text.
\d+
will match one or more digits in a string.Consider the following regex pattern \b\w{3}\b
. This pattern will match any 3-letter word in a text. Applying this regex to the sentence 'The cat sat on the mat.' will match 'cat', 'sat', and 'mat'.
The syntax of regular expressions can appear cryptic at first, but understanding its structure is crucial for utilizing its full potential. Some of the key components include:
- Characters: Literal characters to match themselves.
- Character classes: Sets of characters like [a-z], [A-Z], [0-9].
- Quantifiers: Indicate numbers of characters or occurrences, such as * (zero or more) and + (one or more).
- Anchors: Symbols like
^
(start of string) and$
(end of string).
History and Evolution of Regular Expressions
The concept of regular expressions traces back to the 1950s when American mathematician Stephen Cole Kleene developed them as a mathematical notion to represent regular languages. Since then, they've gained substantial traction and evolved into an indispensable tool in computer science and various programming domains.
Initially, regular expressions were formalized for theoretical computer science purposes, specifically for automating the pattern matching process in string processing tasks. Over time, with the advent of text editors like ed and utilities such as grep in UNIX, regex found practical applications in software development and administration.
Many modern programming languages like Python, JavaScript, and Java have built-in support for regular expressions, making it easier for developers to implement them in their code.
Regular expressions implemented in UNIX played a significant role in their widespread adoption. Modern-regex implementations have advanced to include features like Unicode support, named capture groups, and complex lookaround assertions, further expanding their utility in various contexts from web development to data validation and more.
Understanding Regular Expressions
Regular expressions, often shortened as regex, are powerful tools used to match patterns within text. Their capability to search, replace, and validate data makes them indispensable in computer science.
Importance of Regular Expressions in Computer Science
In the field of computer science, regular expressions play a crucial role in various applications. They offer an efficient way to:
- Validate user inputs: Ensuring formats of emails, phone numbers, and other data types are correct.
- Search within texts: Identifying specific patterns or info in large datasets.
- Text manipulation: Editing or replacing text based on patterns, which is essential in data cleaning tasks.
An example in Python to demonstrate pattern matching using regex:
import re text = 'Please contact us at contact@example.com for more info.' pattern = r'[\w\.-]+@[\w\.-]+' match = re.findall(pattern, text) print(match)This code will search for email patterns in the given text string and will return the email found in the list: ['contact@example.com'].
Regular expressions are deeply integrated into the UNIX utility grep, and are utilized heavily by developers for scripting. They support diverse functionalities such as grouping, alternation (or), and more, which make them flexible for advanced pattern matching scenarios. Understanding how the engine behind regex works—like backtracking algorithms and how greedy vs non-greedy operations are handled—can elevate your mastery in utilizing regex effectively. Here's a peek at some advanced components:
Lookahead | Asserts that a pattern can be matched, without including it in the result. |
Lookbehind | Asserts that a pattern can be matched before a given point in the text. |
Non-capturing groups | Groups parts of patterns for modifications without backreference numbering, using ?:pattern . |
Key Concepts for Understanding Regular Expressions
While using regular expressions, understanding its basic and advanced key concepts is essential. The fundamental building blocks of regex include:
- Literals: Exact characters for matches.
- Meta Characters: Characters with special meanings, such as
.
,*
, or+
. - Character Classes: Sets of characters, like
[a-z]
or[0-9]
, to match specific types. - Anchors: Start or end of a string denoted by
^
and$
respectively. - Groups and Ranges: Use parentheses for binding parts of a pattern.
For instance, the regex pattern ^(?=.*[0-9])
would match any string that contains at least one number. It demonstrates the use of positive lookahead to ensure condition satisfaction without matching the string.
Different programming languages might have slight variations in regex syntax. Always check the specific regex dialect for the language you're working on.
Regular Expressions Syntax
Understanding the syntax of regular expressions is crucial for effectively utilizing them in programming. It involves a combination of characters and special symbols to form search patterns.
Basic Syntax Elements
The basic syntax elements of regular expressions are foundational to creating more complex patterns. Common elements include:
- Literals: Characters that match themselves.
- Meta Characters: Symbols that denote special meanings and include
.
(matches any character),*
(zero or more occurrences),+
(one or more occurrences), and?
(zero or one time). - Character Classes: Bracketed lists like
[a-z]
match any lowercase letter from a to z. - Anchors: Symbols that specify a position;
^
indicates the start of a string, while$
signifies the end. - Quantifiers: Specify the number of times an element can occur, like
{2,4}
which means between 2 and 4 times.
For instance, the regex pattern \b\w+\b
matches any whole word in a text. Applying it to the sentence 'Regex is amazing' results in matches for 'Regex', 'is', and 'amazing'.
Meta characters have to be escaped with a backslash (\) if you want to use them as normal characters in a string.
Beyond the basic elements, regular expressions support advanced constructs such as:
- Lookahead and Lookbehind: They match based on what precedes or follows a text and are written as
(?=...)
and(?<=...)
respectively. For example,q(?=u)
matches 'q' only if it's followed by 'u'. - Alternation: Uses the pipe
|
to denote 'or', combining different patterns likecat|dog
to match either 'cat' or 'dog'. - Non-capturing Groups: Group patterns without storing them for backreference using
?:...
.
Syntax Variations Across Different Programming Languages
The core concept of regular expressions remains consistent, yet syntax variations exist across different programming languages. Each language may have its own flavor of regex, introducing unique features or slightly altering standard ones.
Here's a comparison of how regex syntax may vary across different languages:
Language | Specific Syntax Feature |
Python | Uses re module; supports Unicode via \u |
JavaScript | Uses built-in RegExp object; lacks traditional lookbehind support |
Java | Comes with powerful Pattern class, allows extended patterns |
Always refer to the language documentation for regex to understand any unique syntax or behavioral nuances when implementing patterns.
Regular Expressions Examples
Regular expressions are immensely useful in text processing and manipulation. Examples of their application help solidify understanding, especially when exploring their diverse usability across different scenarios.
Simple Regular Expressions Patterns
When first learning about regular expressions, it's helpful to start with simple patterns that perform specific functions. These foundational examples build the skills necessary for more complex regex operations. Here are some basic patterns and their purposes:
1. Matching a simple email pattern:
r'[\w\.-]+@[\w\.-]+'This pattern will match a basic email address structure.
2. Searching for any word starting with 'a':
r'\ba\w*'This regex will scan text to find any words that start with the letter 'a'.
The \b
in regex denotes a word boundary, which is useful for specifying starts or ends of words.
3. Matching a phone number format:
r'\d{3}-\d{2}-\d{4}'This pattern matches a simple phone number format like 123-45-6789.
Understanding how symbols like *
, +
, and ?
operate is crucial, as they refer to the frequency of character matches:
- * denotes zero or more matches.
- + indicates one or more matches.
- ? specifies zero or one match.
Advanced Regular Expressions Usage Cases
Once you're comfortable with basic patterns, advanced regular expression techniques can greatly expand your ability to handle complicated text-processing tasks. Advanced use cases often involve intricate patterns in large datasets.
1. Validating complex email addresses with multiple subdomains, and TLDs:
r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}'This pattern accounts for most valid email formats in a more comprehensive way.
Incorporating advanced features such as lookahead and lookbehind assertions in regex helps in crafting precise criteria for matches without consuming characters in the search results.
2. Password validation that requires at least one letter, one number, and one special character:
r'^(?=.*[A-Za-z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$'This pattern ensures each password contains a mix of required character types.
Advanced regex usage in data science and programming often involves combining patterns using logical OR (|) and parentheses to form subpatterns, for instance:
Pattern | Description |
(cat|dog) | Matches 'cat' or 'dog' |
\d{3,} | Matches any sequence of three or more digits |
*?
), you can optimize the regex engine's operation and manage backtracking more efficiently.Regular Expressions - Key takeaways
- Regular Expressions: Sequences of characters that define a search pattern, used for finding and manipulating text or data.
- Regular Expressions Examples: Patterns like
\d+
match one or more digits, and\b\w{3}\b
matches any 3-letter word. - Understanding Regular Expressions: They match text patterns and are crucial for data validation, search, and replace operations in programming.
- Regular Expressions Syntax: Include literals, meta characters (like
*
and+
), character classes ([a-z]
), and anchors (^
,$
). - Advanced Use Cases: Incorporate features like lookaheads and lookbehinds, and non-capturing groups for complex pattern matching.
- Regular Expressions Evolution: Originating in the 1950s from Stephen Cole Kleene and evolving with UNIX tools to become integral in programming languages like Python, JavaScript, and Java.
Learn faster with the 28 flashcards about Regular Expressions
Sign up for free to gain access to all our flashcards.
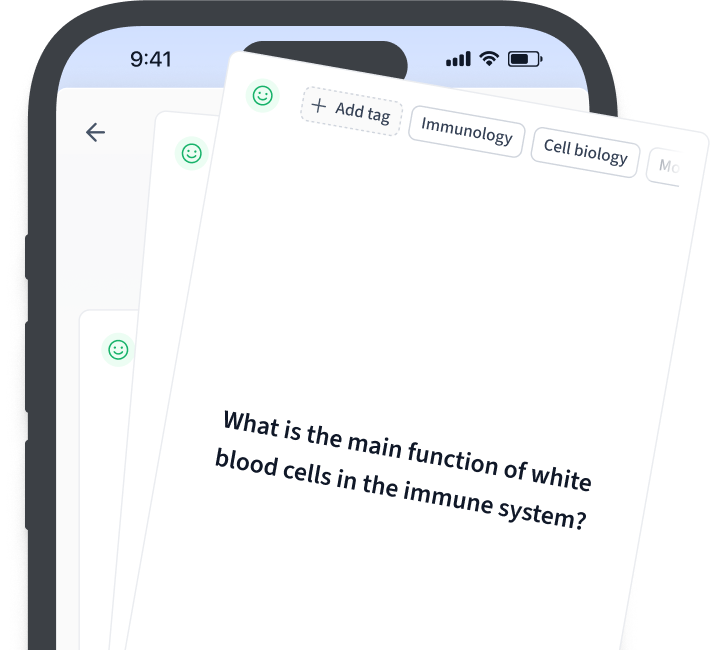
Frequently Asked Questions about Regular Expressions
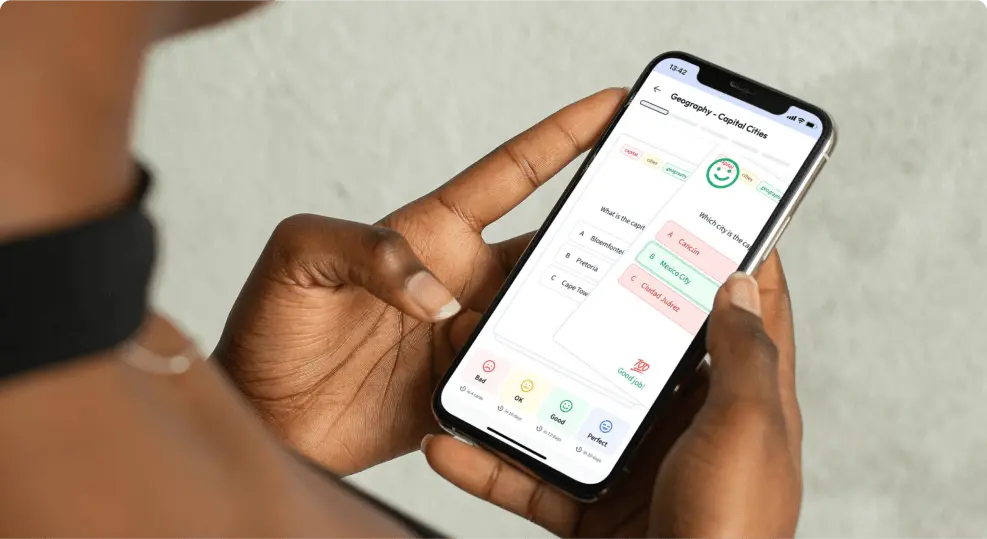
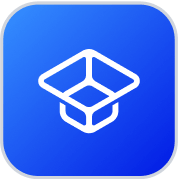
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more