Jump to a key chapter
Navigation Algorithms Definition
Understanding navigation algorithms is essential in many fields, including robotics, mobile applications, and geographic information systems. These algorithms are designed to find the optimal path from a starting point to a desired destination.
What are Navigation Algorithms?
Navigation algorithms are mathematical instructions that enable computation of routes or paths. They consider factors like speed, distance, and obstacles to provide an efficient route. Such algorithms are crucial in GPS technology, autonomous vehicles, and even virtual simulations.
Navigation algorithms: These are computational methods used to determine optimized paths or routes between two points, considering factors like distance, time, and obstacles.
Key Components of Navigation Algorithms
Here are some components often involved in navigation algorithms:
- Pathfinding: This involves finding a viable route from point A to point B.
- Cost Evaluation: Calculating the 'cost' or 'weight' of a path based on distance, time, or energy consumption.
- Optimization: Refining the path to minimize costs or satisfy specific constraints.
- Data Structures: Often involve graphs or grids.
Consider an app offering varied routes to a location based on minimal time or economic fuel consumption. The navigation algorithm computes:
- Total travel time using speed limits
- Distance by calculating using geographic coordinates
- Fuel efficiency by assessing different terrains
Math Behind Navigation Algorithms
Mathematics forms the backbone of navigation algorithms. You might encounter:
- Graph Theory: Nodes represent locations, and edges signify possible paths.
- Calculus: Used in optimizing routes by minimizing costs.
- Linear Algebra: Helps in transformations and rotations in coordinate systems.
- \(C\) is the total cost.
- \(d\) is the distance.
- \(s\) is the speed.
- \(f(c)\) represents other costs, like fuel.
Let’s delve into one specific type of navigation algorithm: the Dijkstra’s Algorithm. It is particularly useful for calculating shortest paths in weighted graphs. The primary mechanism involves selecting the node with the smallest tentative distance, updating its neighboring node distances, and repeating until the destination is reached.Algorithm steps:
function Dijkstra(Graph, source): initialize distances, previous_node with Infinity, None distances[source] = 0 create priority_queue while priority_queue not empty: extract_min from queue for each neighbor of extracted node: calculate new_distance if new_distance < distances[neighbor]: update distances and previous_node enqueue neighbor with new_distance return distances, previous_nodeThis algorithm ensures that each node in a graph gets the shortest distance from the source node.
Navigation Algorithms Explained
Navigation algorithms are integral components in the realms of technology and robotics. By designing optimal paths from an origin to a destination, they facilitate a wide range of applications from GPS navigation to autonomous vehicle routing.
Key Elements of Navigation Algorithms
weight of a path by assessing factors such as distance and time.
Navigation algorithms: Algorithms designed to calculate optimal routes or paths considering various factors, including distance and obstacles, used extensively in fields like robotics and geolocation services.
Suppose a navigation app is programmatically assessing routes based on time efficiency. It calculates:
- Total Travel Time: Using speed limits and traffic data.
- Distance: By converting geographic coordinates into a traveled path length.
- Dynamic Constraints: Such as temporary roadblocks or changes in weather conditions.
Mathematical Foundation of Navigation Algorithms
Mathematics forms the core of designing navigation algorithms. Key mathematical concepts include:
- Graph Theory: Locations are modeled as nodes, with paths as edges.
- Calculus: Utilized in optimizing functions to minimize path cost.
- Linear Algebra: Assists in transformations and calculations within coordinate spaces.
- \(C\) represents the total cost.
- \(d\) signifies the distance covered.
- \(s\) indicates speed.
- \(f(c)\) accounts for additional costs, such as fuel efficiency or toll roads.
A deep dive into Dijkstra’s Algorithm provides a glimpse into specific navigation algorithms. Known for computing shortest paths in weighted graphs, it selects nodes with the minimum tentative distance, adjusting the distances of neighboring nodes iteratively.Algorithm steps include:
function Dijkstra(Graph, source): initialize distances, previous_node with Infinity, None distances[source] = 0 create priority_queue while priority_queue not empty: extract_min from queue for each neighbor of extracted node: calculate new_distance if new_distance < distances[neighbor]: update distances and previous_node enqueue neighbor with new_distance return distances, previous_nodeDijkstra's Algorithm ensures each node in a graph receives the shortest possible distance from a designated source node.
Autonomous Navigation Algorithms in Robotics
In the realm of autonomous robotics, navigation algorithms are pivotal. These algorithms empower robots to autonomously navigate their environment, making decisions based on real-time data. They optimize routes and ensure that robots can adapt to dynamic surroundings, facilitating better movement efficiency and obstacle avoidance.
Navigation Algorithms Robotics Applications
Robotics applications make extensive use of navigation algorithms to enhance functionality and performance. Here are some of the key applications:
- Autonomous Vehicles: Employ complex algorithms to chart the safest and most efficient paths, interpreting data from sensors and GPS.
- Personal Assistive Robots: Use these algorithms to move around in human environments, providing help with daily tasks.
- Industrial Robots: Focus on optimizing paths within constrained environments, such as manufacturing floors.
- Search and Rescue: Utilize detailed maps and continuously updating algorithms to operate in unpredictable terrains.
Consider an autonomous drone tasked with delivering packages. The navigation algorithm:
- Calculates altitude and distance using data from GPS coordinates.
- Adjusts the path in real-time to avoid obstacles like birds or buildings.
- Modifies speed based on wind conditions to optimize energy usage.
- Updates routing based on package weight to maintain balance.
Examples of Navigation Algorithms in Robotics
Various navigation algorithms are employed in robotics to enhance movement and task performance. Some popular ones include:
- A* Algorithm: Well-known for its efficiency in pathfinding and graph traversal, focusing on sampled environments.
- RRT (Rapidly-exploring Random Trees): Useful for navigating complex spaces by exploring random samples.
- Kalman Filters: Applied in navigation systems to estimate states like position, velocity, and orientation.
A deeper look at the A* Algorithm, often used in robotics for path planning:
- It combines features of uniform-cost search and pure heuristic search to efficiently compute a path.
- The algorithm searches for a path by calculating the cost, \( f(x) = g(x) + h(x) \), where:
- \(g(x)\) is the cost to reach the current node.
- \(h(x)\) is the estimated cost from the current node to the goal.
- It employs a priority queue to manage explored nodes, always expanding the least cost node first.
Pseudocode for A*:function A*(start, goal): open_set = priority_queue() open_set.add(start) while open_set not empty: current = open_set.pop() if current is goal: return reconstruct_path(current) for each neighbor of current: tentative_g = g(current) + distance(current, neighbor) if tentative_g < g(neighbor): neighbor.parent = current g(neighbor) = tentative_g f(neighbor) = g(neighbor) + h(neighbor) if neighbor not in open_set: open_set.add(neighbor) return failureThis algorithm is widely used for its robustness and ability to navigate unpredictable environments.
Graph-Based Navigation Algorithms
In the field of navigation, graph-based algorithms are extensively used to compute paths and routes in various applications such as robotics, transportation systems, and geographic information systems. These algorithms harness graph theory to represent the network of pathways, making them extremely efficient for pathfinding tasks.
Understanding Graph Theory in Navigation
Graphs consist of nodes and edges, where nodes represent positions or junction points, and edges signify possible paths between these points. The goal of graph-based navigation algorithms is to find the best path considering constraints like distance, cost, and obstacles.
Nodes and Edges: In graph theory, nodes are the individual intersections of a path, while edges are the connections that represent the pathways between nodes.
A city map can be represented as a graph:
- Nodes: Locations like intersections, bus stops, and landmarks.
- Edges: Streets and pathways connecting these locations.
Algorithms Employed in Graph-Based Navigation
Several algorithms stand out when it comes to graph-based pathfinding:
- Dijkstra’s Algorithm: Efficiently finds the shortest path from a single source to all other nodes in a graph.
- A* Algorithm: Combines heuristic analysis with pathfinding to optimize the search process.
- Breadth-First Search (BFS): Explores all possible paths equally before deciding the optimal route in unweighted graphs.
Examining Dijkstra’s Algorithm offers insights into its function within graph-based navigation:Dijkstra’s Algorithm operates by systematically exploring pathways from a starting node and calculating the shortest possible distance to each subsequent node.It initializes all distances as infinite except for the starting point and iteratively adjusts these until the minimal distances are found.Graph referencing:
function Dijkstra(Graph, source): initialize distances = infinity set distance[source] = 0 priority_queue = empty enqueue source with priority 0 while priority_queue is not empty: current = dequeue for each neighbor of current: distance via current = distance[current] + weight(current, neighbor) if new distance < distance[neighbor]: update distance[neighbor] = new distance enqueue neighbor with new distance return distancesThis algorithm is fundamental in network routing and determining least-cost paths.
Did you know? The A* algorithm's strength lies in its heuristic component, which anticipates the ideal path and speeds up the search process.
Mathematics in Graph-Based Algorithms
Mathematics plays a crucial role in graph-based navigation:
- Cost Functions: Calculate the weighted costs of different paths. Example:\[f(x) = g(x) + h(x)\]Where \(g(x)\) is the cost from the start node, and \(h(x)\) is the estimated cost to the goal node.
- Matrix Representations: Graphs are often represented as adjacency matrices or lists, with entries indicating the presence and weight of edges.
navigation algorithms - Key takeaways
- Navigation Algorithms Definition: Computational methods for determining optimized paths, considering factors like distance, time, and obstacles.
- Components of Navigation Algorithms: Include pathfinding, cost evaluation, optimization, and the use of data structures like graphs or grids.
- Examples in Robotics: A* Algorithm, RRT, and Kalman Filters; used to enhance movement and task performance in robots.
- Graph-Based Navigation Algorithms: Utilize graph theory, where nodes represent locations and edges represent paths; key algorithms include Dijkstra’s and A*.
- Mathematical Foundation: Graph theory, calculus, and linear algebra are critical for route optimization and path cost calculation.
- Autonomous Navigation Algorithms: Enable robots to autonomously navigate environments with efficient route optimization and real-time adaptability.
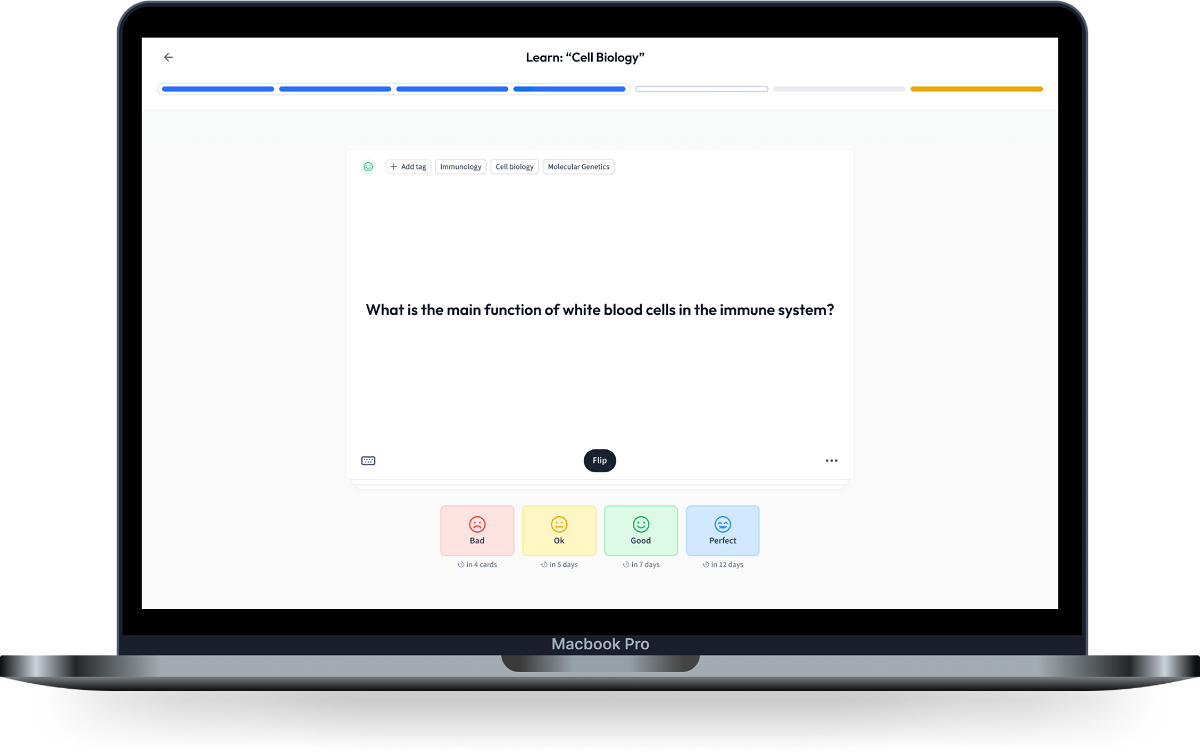
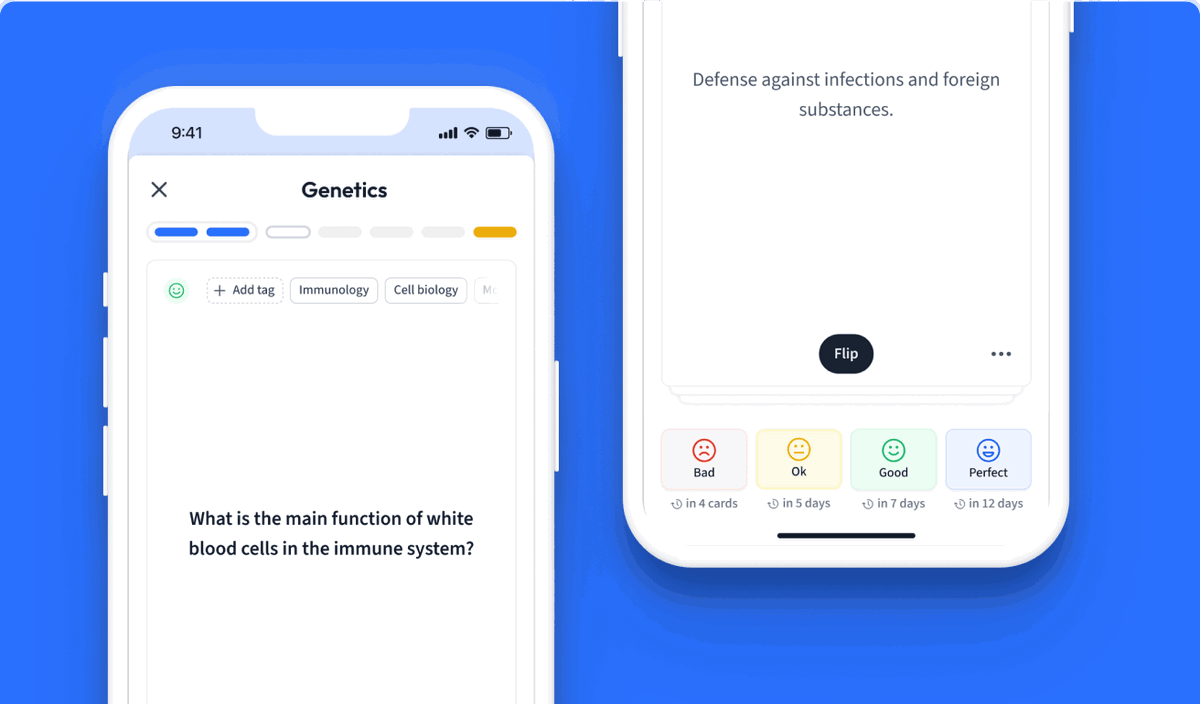
Learn with 12 navigation algorithms flashcards in the free StudySmarter app
We have 14,000 flashcards about Dynamic Landscapes.
Already have an account? Log in
Frequently Asked Questions about navigation algorithms
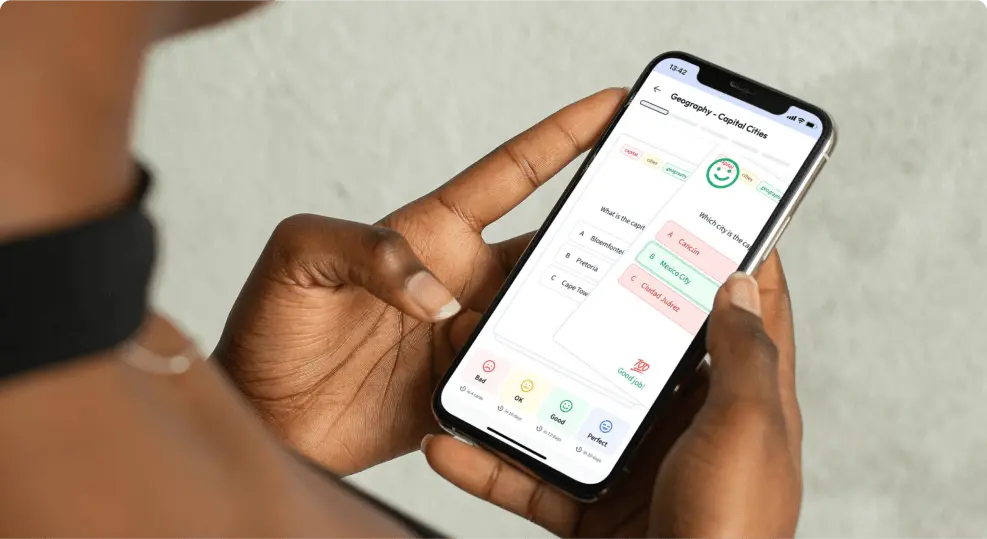
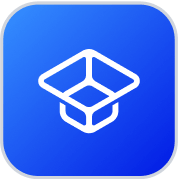
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more