Jump to a key chapter
Recurrent Networks Overview
Recurrent networks, a subclass of neural networks, are designed for sequence prediction. These networks are particularly useful in tasks involving sequential data, such as language translation, speech recognition, and time series forecasting. Their ability to remember past information makes them unique compared to traditional neural networks.
What Are Recurrent Neural Networks?
Recurrent Neural Networks (RNNs) are a type of neural network where connections between units can form cycles, allowing information to persist. In a typical RNN, the output from the previous step is fed as input to the current step, making them ideal for tasks where contexts or sequences are important. These networks are broadly applied in:
- Text Generation: Producing a sequence of words.
- Stock Market Prediction: Analyzing time-series data for trends.
- Machine Translation: Translating languages by understanding sequence context.
The architecture of an RNN is distinct because of its loops, which enable the network to maintain a memory of previous inputs. One of the common variants of RNN is the Long Short-Term Memory (LSTM) network. It was designed to combat the 'vanishing gradient problem' often found in standard RNNs, which can impede learning of long-range dependencies. LSTMs introduce memory cells and gates to control the flow of information, making it easier to remember the long-term context.
import numpy as npimport tensorflow as tffrom tensorflow import kerasmodel = keras.Sequential([ keras.layers.SimpleRNN(50, input_shape=(3, 4)), keras.layers.Dense(1, activation='sigmoid')])print(model.summary())In the above Python example, a simple RNN layer with 50 units is created for a sequence with three steps and four features per step. The final layer has a dense unit for output and utilizes a sigmoid activation.
RNNs are powerful for handling sequential data but can struggle with long-term dependencies. This is where LSTM networks shine.
Recurrent Neural Network Definition
Recurrent Neural Networks (RNNs) can be defined as neural networks with loops that allow them to maintain a memory, which is useful for processing sequences of data and learning temporal patterns.
RNNs are characterized by their feedback connections, making them exceptionally well-adapted for tasks where sequence or temporal characteristics are critical. The key to their power lies in their cyclical architecture:
- Nodes in a cyclical cycle: Enable feedback loops for memory preservation.
- Algorithmic Complexity: Derived from their ability to model and predict complex sequences.
The functionality of an RNN can be mathematically expressed as:For time step \( t \):\( h_t = \mathrm{tanh}(W \cdot x_t + U \cdot h_{{t-1}} + b) \) and\( y_t = V \cdot h_t + c \)Where:
- \(h_t\) is the hidden state.
- \(W, U, V\) are weights.
- \(b, c\) are biases.
- \(x_t\) is the input at time \(t\).
Weights in RNNs are shared across different time steps, which significantly reduces computational load.
Recurrent Neural Network Explained
Recurrent Neural Networks (RNNs) are specialized types of neural networks capable of using their internal states as memory to process sequences of inputs. This unique capability makes them highly suitable for handling tasks involving sequential data, where patterns emerge over time. In the field of engineering, RNNs are particularly valued for their flexibility in adapting to varying input lengths and their ability to model temporal dynamics.
Understanding Recurrent Networks in Engineering
In engineering, understanding and implementing RNNs involves recognizing their suitability for tasks dealing with sequences and time-dependent data. RNNs are widely used in various domains, where processes are naturally sequential. The following aspects are essential for understanding RNNs in this field:
- Data Sequence Processing: Whether for audio processing or predictive maintenance, RNNs excel at identifying patterns over time.
- Feed-back Loops: These allow for past information to be integrated into current inputs, greatly enhancing predictive capabilities.
- Complex Temporal Models: By processing sequences, RNNs can model system behavior and predict outcomes effectively.
The architecture of RNNs is fundamentally designed around carrying information across time steps. Standard RNNs face difficulties with gradients, which is why LSTM and GRU (Gated Recurrent Unit) networks are designed. These variants introduce memory cells and gating mechanisms to control information flow:
- Memory Cells: Allow the network to forget or retain information, addressing the vanishing gradient problem.
- Input and Forget Gates: Control the relevance of incoming and carried-over data.
Keep in mind that RNNs, despite their strengths, require extensive training data to achieve optimal performance in engineering tasks.
Applications of Recurrent Networks
Recurrent networks are extensively used in various engineering applications due to their proficiency in sequence modeling and pattern recognition. Below are some noteworthy applications:
- Time Series Analysis: RNNs are commonly used for forecasting future trends based on past data, such as predicting stock prices or weather conditions.
- Natural Language Processing: Tasks such as language translation and voice recognition rely on recurrent networks to maintain context and meaning across sentence structure.
- Predictive Maintenance: In industrial engineering, RNNs assess machinery health by analyzing vibrational patterns or sensor data over time, predicting potential failures before they occur.
Consider a Python implementation to create a simple RNN architecture for a sequence learning task:
import numpy as npimport tensorflow as tffrom tensorflow import kerasmodel = keras.Sequential([ keras.layers.SimpleRNN(64, activation='relu', input_shape=(10, 1)), keras.layers.Dense(1)])model.summary()This code demonstrates a basic RNN in TensorFlow, designed to handle input sequences with ten time steps, each having a single feature. Note how the architecture is structured for sequence processing.
When using RNNs, it's important to prepare your data correctly and ensure sequences are padded or truncated to a uniform length for efficient model training.
Gated Recurrent Neural Network
Gated Recurrent Neural Networks (GRNNs) represent an advanced architecture that addresses some of the limitations of traditional RNNs, such as difficulty in learning long-range dependencies. Gated mechanisms, like those found in Long Short-Term Memory (LSTM) and Gated Recurrent Unit (GRU) networks, help regulate the flow of information in the network, allowing them to capture long-term patterns more effectively.
Importance of Gated Recurrent Networks
Gated Recurrent Networks are crucial in addressing some of the core challenges experienced with traditional RNNs. Their importance can be seen in several key areas:
- Long-Term Dependency Handling: The gates in GRNNs control the input, output, and forget signals, effectively managing dependency over longer sequences.
- Robustness to Vanishing Gradients: By preventing gradients from becoming excessively small, GRNNs maintain their sensitivity to small parameter changes over many time steps.
- Flexibility and Adaptability: GRNNs adapt better to different data types and learning tasks, making them versatile in numerous applications.
The architecture of GRNNs includes components such as the input, forget, and output gates. Each gate is a component of a neural network, utilizing activation functions and mathematical operations to manage data flow:The Forget Gate is described by the equation:\[f_t = \text{sigmoid}(W_f \times [h_{t-1}, x_t] + b_f)\]Where:
- \(f_t\) is the forget gate activation.
- \(W_f\) and \(b_f\) are the weights and biases for the forget gate.
- \(h_{t-1}\) is the hidden state from the previous time step.
- \(x_t\) is the input at the current time step.
import numpy as npimport tensorflow as tffrom tensorflow.keras.layers import GRUmodel = tf.keras.Sequential([ GRU(50, activation='tanh', input_shape=(20, 5)), tf.keras.layers.Dense(1, activation='sigmoid')])model.summary()This code showcases the implementation of a GRU layer with 50 units. The model accepts sequences with 20 time steps, each having 5 features. The final layer is a dense unit with a sigmoid activation for binary classification tasks.
Use GRUs for applications that require learning long-term dependencies efficiently but have limited computational resources. They often require fewer parameters and less training time compared to LSTMs.
How Gated Recurrent Networks Work
Gated Recurrent Networks employ a system of gates within their architecture, which control the flow of information. This gating mechanism facilitates more accurate prediction and pattern recognition in sequence-based data. Here's an overview of how they function:
- Input Gate: Determines which values from the input will update the memory state.
- Forget Gate: Decides what information to discard from the previous cell state.
- Output Gate: Controls the output to the next hidden state.
- \(r_t\) is the reset gate activation for the memory content.
- \(o_t\) is output gate activation.
GRNN architectures like LSTMs are widely implemented in language modeling applications, outperforming basic RNNs due to their enhanced memory cell architecture, which efficiently handles varying input lengths.
Recurrent Networks Engineering
Recurrent networks, a vital component in modern artificial intelligence, excel in sequence prediction by utilizing their ability to remember past information through feedback loops. This makes them indispensable in engineering applications like speech recognition, language translation, and time series forecasting. Rather than processing inputs independently, recurrent networks consider data in sequential contexts.
Recurrent Networks in AI Development
In the realm of AI development, recurrent networks provide the architecture needed to model time-dependent sequences. These networks are engineered to address a variety of sequence-oriented AI tasks by maintaining a form of memory through feedback within the network.
- Language Processing: RNNs are used to analyze sequences of words in language models, effectively translating and generating text.
- Sequential Data Analysis: They model the dependencies in sequential data such as stock prices and climate patterns.
- Dynamic System Predictions: In robotics, they help predict outcomes based on a series of environmental inputs.
import tensorflow as tffrom tensorflow.keras.layers import LSTM, Densemodel = tf.keras.Sequential([ LSTM(50, activation='relu', input_shape=(10, 1)), Dense(1)])model.compile(optimizer='adam', loss='mse')print(model.summary())This example illustrates an LSTM model construction for sequence tasks, accepting sequences of 10 time steps each. The output layer uses a single neuron for regression tasks, compiled with Adam optimizer and mean squared error loss.
Developing RNNs in AI applications links physics-based insights with stochastic machine learning approaches, quantifying temporal patterns in data. Advanced engineering techniques include creating hybrid models that combine RNNs with transformers, capitalizing on RNNs' temporal strengths and transformers' contextual modeling power. This can be instrumental in handling varied data types across sectors like healthcare diagnostics and natural language understanding.Moreover, recurrent networks contribute significantly to reinforcement learning, where they aid in decision-making processes by predicting future rewards based on sequence inputs, allowing AI systems to learn strategies over longer horizons.
Combining RNNs with convolutional layers can improve performance in video processing tasks by capturing both spatial and temporal features effectively.
Challenges in Recurrent Networks Engineering
Despite their advantages, the engineering of recurrent networks presents inherent challenges, particularly concerning the stability and efficiency of model training. The primary issues include:
- Vanishing and Exploding Gradients: This occurs when updates become too small or too large, impairing effective learning and weight updates during training.
- Computational Complexity: RNNs, especially those with numerous layers or units, require substantial computational resources, impacting scalability.
- Data Dependency: RNNs need large amounts of labeled sequential data for effective training, which can be resource-intensive to compile and annotate.
To manage the vanishing gradient issue, consider using advanced activation functions like rectified linear units (ReLU) instead of traditional sigmoid functions.
Addressing RNN challenges often resorts to hybridizing architectures or leveraging the strengths of different network types. For instance, engineers might integrate attention mechanisms or incorporate transfer learning to manage resource constraints effectively.Beyond technical optimizations, interdisciplinary approaches are gaining traction. By combining insights from cognitive sciences with computational engineering, it's possible to craft RNNs that are not only efficient but more closely aligned with human learning processes, offering improvements in areas such as adaptive learning algorithms and the creation of more interactive AI systems.
recurrent networks - Key takeaways
- Recurrent Networks: A subclass of neural networks designed for sequence prediction, particularly useful in tasks like language translation and speech recognition due to their ability to remember past information.
- Recurrent Neural Network (RNN) Definition: Neural networks with loops that allow them to maintain a memory, facilitating the processing of sequences and learning of temporal patterns.
- RNN Architecture: Characterized by loops and feedback connections, enabling memory retention across time steps; common variants include Long Short-Term Memory (LSTM) and Gated Recurrent Unit (GRU) networks.
- Long Short-Term Memory (LSTM): A type of RNN that addresses the 'vanishing gradient problem'; uses memory cells and gates to aid in maintaining long-term dependencies.
- Gated Recurrent Networks: Advanced RNN architectures like LSTM and GRU use gating mechanisms to regulate information flow, improving long-term pattern capture and mitigating issues like vanishing gradients.
- Recurrent Networks Engineering: Involves leveraging RNNs' sequential processing capabilities in AI development, with applications in language processing, time-series analysis, and dynamic system predictions.
Learn faster with the 12 flashcards about recurrent networks
Sign up for free to gain access to all our flashcards.
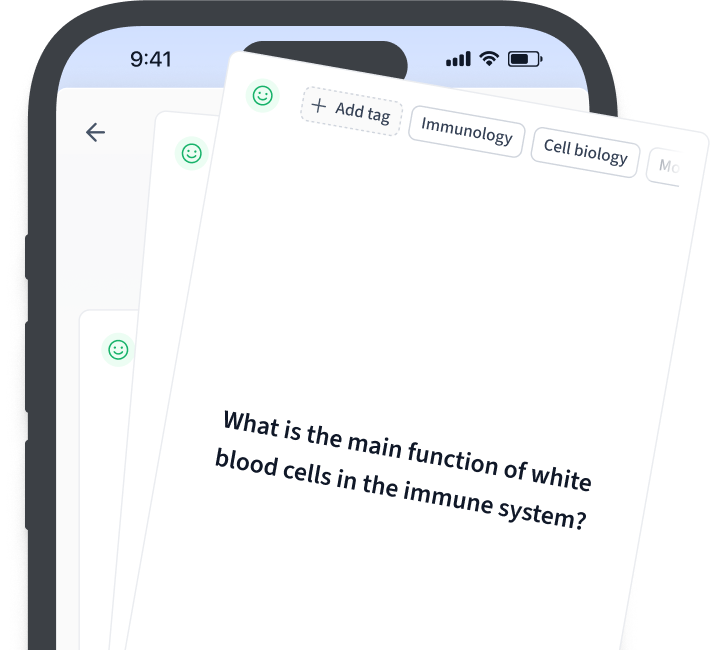
Frequently Asked Questions about recurrent networks
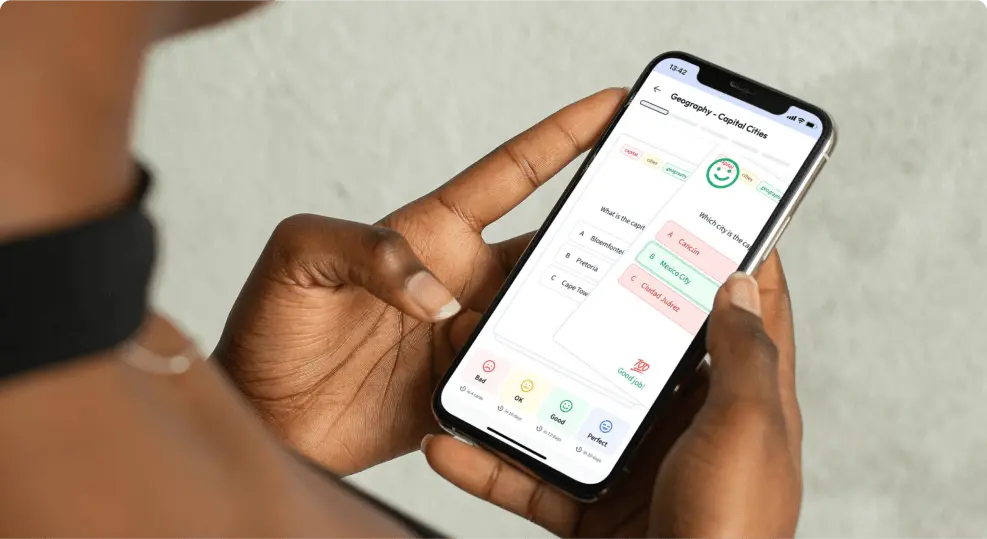
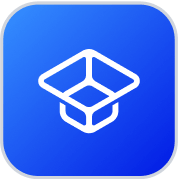
About StudySmarter
StudySmarter is a globally recognized educational technology company, offering a holistic learning platform designed for students of all ages and educational levels. Our platform provides learning support for a wide range of subjects, including STEM, Social Sciences, and Languages and also helps students to successfully master various tests and exams worldwide, such as GCSE, A Level, SAT, ACT, Abitur, and more. We offer an extensive library of learning materials, including interactive flashcards, comprehensive textbook solutions, and detailed explanations. The cutting-edge technology and tools we provide help students create their own learning materials. StudySmarter’s content is not only expert-verified but also regularly updated to ensure accuracy and relevance.
Learn more